[Angular mosaic 1] My fast style, Intercept routing events (router-outlet onActivate event, @Input, extends base page).
This page contains a small fast notes for myself, because I have no big experience with Angular and I need some notes to switching to Angular faster from other my programmer's environment.
- 1. My fast style (background, NAV menu, header) for my various technical sites.
- 2. Intercept and analyzing routing events (router-outlet onActivate event).
- 3. Pass parameters from one component to another (@Input, extends base page).
- 4. Simplest shared service (@Injectable, Subject, Injectable, LocalStorage, Store).
- 5. UIkit Modal Futures (uk-modal tag, Modal from routed page and from Menu, Modal Toggle, Modal Show, Bind by On, MouseOver Modal, Modal Alert, Modal Prompt, Close button).
- 6. Standard Interceptor and JWT injector, Login page, Auth service, and Base form with FormGroup.
- 7. Fill select/options (FormsModule, ngModel, ngModelChange, ngValue, http.get Json from Assets, LocalStorage)
- 8. Angular connection service (Enable/Disable Heartbeat, Heartbeat URL, Heartbeat Interval/RetryInterval)
- 9. [Angular mosaic 5] Net Core SignalR Client (ReactiveX/rxjs, Configure Angular injector, Abstract class, Inject SignalR service, Configure SignalR hub - forbid Negotiation, setUp Transport, URL, LogLevel, Anon or AU socket connection), Configure server Hub (MapHub endpoint, WithOrigins on CORS-policy, LogLevel, JWT AU)
1. My fast style (background, NAV menu, header) to my various technical sites.
There are a lot of various free Angular site styles, for example, look to https://github.com/coreui/coreui-free-angular-admin-template, but I use my own template.
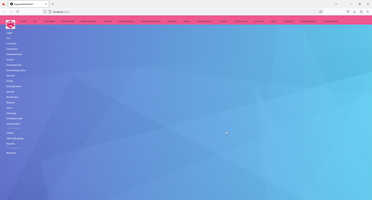
2. Intercept and analyzing routing events (router-outlet onActivate event)
Router module has a couple of very important events.
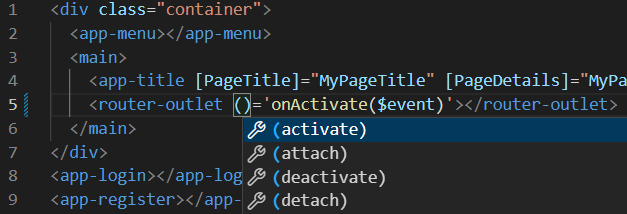
We can intercept this events
1: <div class="container">
2: <app-menu></app-menu>
3: <main>
4: <app-title></app-title>
5: <router-outlet (activate)='onActivate($event)'></router-outlet>
6: </main>
7: </div>
And analyze it (menu.component.ts).
1: import { Component, OnInit } from '@angular/core';
2: import { Router } from '@angular/router';
3: import { takeUntil } from 'rxjs';
4: import { Subject } from 'rxjs/internal/Subject';
10: ...
11: @Component({
12: selector: 'app-menu',
13: templateUrl: './menu.component.html',
14: styleUrls: ['./menu.component.css']
15: })
16: export class MenuComponent implements OnInit {
17:
18: public ShowLoginButton: boolean = true;
19: private destroyed$ = new Subject();
20:
21: constructor(private router: Router) {
22: this.router.events
23: .pipe(takeUntil(this.destroyed$))
24: .subscribe(event => {
25: console.log(event);
26: });
re>
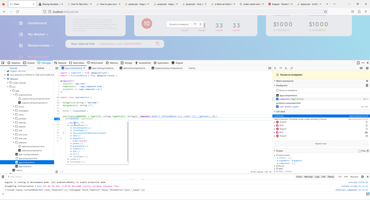
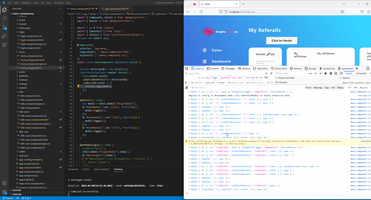
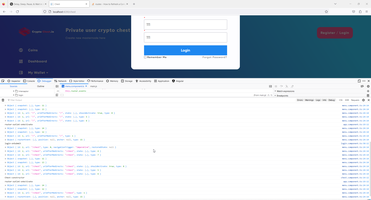
3. Pass parameters from one component to another (@Input, extends base page)
First main Angular future is pass parameters from one component to another. This is a pattern to do this (app.component.html).
1: <div class="container">
2: <app-menu></app-menu>
3: <main>
4: <app-title [PageTitle]="MyPageTitle" [PageDetails]="MyPageDetails"></app-title>
5: <router-outlet></router-outlet>
6: </main>
7: </div>
In this case component app-title accept input two parameters.
1: <section id="main_info">
2: <div class="main_info">
3: <div class="main_info__title">
4: <h1>{{PageTitle}}</h1>
5: <p>
6: {{PageDetails}}
7: </p>
8: </div>
9: <app-title-user *ngIf="ShowCabinet">
10: </app-title-user>
11: <app-title-anon *ngIf="!ShowCabinet">
12: </app-title-anon>
13: </div>
14: </section>
1: import { Component, Input, OnInit } from '@angular/core';
6: ...
7: @Component({
8: selector: 'app-title',
9: templateUrl: './title.component.html',
10: styleUrls: ['./title.component.css']
11: })
12:
13: export class TitleComponent implements OnInit {
14:
15: @Input() public PageTitle: string = "";
16: @Input() public PageDetails: string = "";
18: ...;
19: constructor(
23: ...
And each page has base page routed-component.ts.
1: export class RoutedComponent {
2: PageTitle: string="";
3: PageDetails: String="";
4: }
And extends base page.
1: import { Component, OnInit } from '@angular/core';
2: import { RoutedComponent } from '../routed-component';
5: ...
6: @Component({
7: selector: 'app-settings',
8: templateUrl: './settings.component.html',
9: styleUrls: ['./settings.component.css']
10: })
11: export class SettingsComponent extends RoutedComponent implements OnInit {
12: constructor() {
13: super();
14: this.PageTitle="Settings"
15: this.PageDetails = "User profile settings"
16: console.log("Settings-constructor");
17: }
Than route interceptor need to transfer this values from base page to app-title component (app.component.ts).
1: import { Component } from '@angular/core';
2:
3: @Component({
4: selector: 'app-root',
5: templateUrl: './app.component.html',
6: styleUrls: ['./app.component.css']
7: })
8:
9: export class AppComponent {
10:
11: MyPageTitle:string = "";
12: MyPageDetails: string ="";
13:
14: title = 'CryptoChest';
15:
16: onActivate(component: { PageTitle: string; PageDetails: string}){
17: console.log("router-outlet-onActivate");
18: if(component.PageTitle){
19: this.MyPageTitle = component.PageTitle;
20: this.MyPageDetails = component.PageDetails;
21: }
22: }
23: }

|