How to change image Exif metadata
If you have Photoshop editable image, it write tag "Photoshop" to Exif information and change another Exif tag. Even you save image without Photoshop metadata, it means only absent XMP tag in result image.
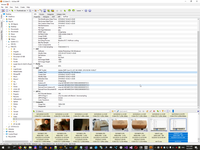
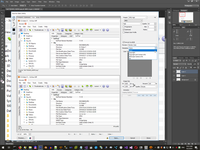
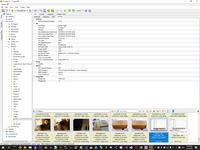
To restore original exif metadata you need to use some programs and steps.
- (0) The best program to show and evaluate you Exif in GUI mode is XnView MP, screen of this program you can see above. XnView MP has two opportunity to change Exif - (1) Clear it at all, (2) changing timestamp. Any other operation with Exif need to more deep understanding..
- (1) To Show Exif and edit it in symbolic view or in hexadecimal view by HexEdit is useful a project ExifLibrary for .NET. Also this project contains a lot information about Exof metadata.
- (2) Best way to simple copy one Exit information to another is own code contained only some simple lines. Come below copy all exif information from IMG_0000.jpg to IMG_0001.jpg with excluding Trumbnail image.
1: Imports System.Drawing
2: Imports System.Drawing.Imaging
3: Imports System.Text
4:
5: Module Module1
6:
7: Sub Main()
8: Dim SourceImg As New Bitmap("E:\My-1\IMG_0000.jpg")
9: Dim TargetImg As New Bitmap("E:\My-1\IMG_0001.jpg")
10: For Each One As PropertyItem In SourceImg.PropertyItems
11: Dim Str1 As New StringBuilder()
12: Str1.Append(String.Format("{0:x2} :", One.Id))
13: For i = 0 To One.Len - 1
14: Str1.Append(String.Format("{0:x2}", One.Value(i)))
15: Next
16: If String.Format("{0:x2}", One.Id) <> "501b" Then
17: TargetImg.SetPropertyItem(One)
18: End If
19: Console.WriteLine(Str1.ToString)
20: Next
21: TargetImg.Save("E:\My-1\IMG_00011.jpg")
22:
23: End Sub
24:
25: End Module
And in this screen I compare information from this my copier and ExifLibrary for .NET from CodeProject.
- (3) There are one way to change Exif (except changing timestamp by Xnview MP) - this is ExifTool by Phil Harvey,
ExifTool by Phil Harvey. But this is command line utilities with big and difficult usage. This is common help of this utils exiftool-help.txt, and this is list of exif tag it supported exit-tag.txt.
- Main problem after Photoshop edition, it changing JFIF tags in Exif metagata. For example before Photoshop I was 300 DPI, after edition 72 DPI.
Therefore after copying information to editable image you have to use at least one ExifTool command "C:\Program Files\exiftool.exe" -JFIF:*Resolution=300 IMG_0001.jpg
- You also can delete all tail of you Photoshop editing. But simple command usually don't working.
Ned to delete one-by-one all Photoshop tags (-xmp:CreatorTool=,-xmp:DerivedFrom=,-xmp:DocumentID=,-xmp:InstanceID=) by this way exiftool.exe -xmp:CreatorTool= ImagePath
- Another small addition issue need to solved is DateTime for image access. There are two way to solve this problem.
(1) There are GUI utilities Attribute Changer
(2) By own codes. This small function collect information about image to database and than change image timestamp.
1: Imports System.Drawing
2: Imports System.Linq
3: Imports System.Text
4: Imports System.IO
5:
6: Class Module1
7:
8: Dim Db1 As VbNetDataContext
9: Public Sub New()
10: Db1 = New VbNetDataContext()
11: End Sub
12:
13: Public Sub SetImageDate(SiteRoot As String, httpPrefix as string)
14: Dim DirName As String
15: Dim Count1 As Integer = 0, Count2 As Integer = 0
16: For Each One As Entrance In Db1.Entrances.OrderByDescending(Of Integer)(Function(X) X.i).AsEnumerable
17: Debug.Print(One.URL)
18: Console.WriteLine(One.URL)
19: Dim RelPath As String = One.URL.Replace("httpPrefix", "")
20: DirName = IO.Path.GetDirectoryName(RelPath)
21: For Each OneImage In Db1.Images.Where(Function(X) X.Folder.ToLower = DirName.ToLower).Where(Function(X) CDate(X.ArticleDate).AddDays(30) < X.Accessed Or CDate(X.ArticleDate).AddDays(30) < X.Created Or CDate(X.ArticleDate).AddDays(30) < X.Modified).AsEnumerable
22: Try
23: Dim ImagePath As String = (SiteRoot & OneImage.Folder & "\" & OneImage.Name).Replace("\\", "\").Replace("/", "\")
24: Debug.WriteLine(ImagePath)
25: If IO.File.Exists(ImagePath) Then
26: Console.WriteLine(ImagePath)
27: IO.File.SetCreationTime(ImagePath, One.CrDate)
28: IO.File.SetLastAccessTime(ImagePath, One.CrDate)
29: IO.File.SetLastWriteTime(ImagePath, One.CrDate)
30: Count1 += 1
31: Else
32: Console.WriteLine("Not found " & ImagePath)
33: Count2 += 1
34: End If
35: Catch ex As Exception
36: Console.WriteLine(ex.Message)
37: End Try
38: Next
39: Next
40: Console.WriteLine(Count1.ToString & " image modified." & vbCrLf & Count2.ToString & " image not found.")
41: End Sub
42:
43: Public Sub UpdateImageDateInDB(SiteRoot)
44: For Each OneImage In Db1.Images.Where(Function(X) CDate(X.ArticleDate).AddDays(30) < X.Accessed Or CDate(X.ArticleDate).AddDays(30) < X.Created Or CDate(X.ArticleDate).AddDays(30) < X.Modified).AsEnumerable
45: Try
46: Dim ImagePath As String = (SiteRoot & OneImage.Folder & "\" & OneImage.Name).Replace("\\", "\")
47: If IO.File.Exists(ImagePath) Then
48: Debug.Print(ImagePath)
49: Console.WriteLine(ImagePath)
50: Dim CreationTime As Date = IO.File.GetCreationTime(ImagePath)
51: Dim LastAccessTime As Date = IO.File.GetLastAccessTime(ImagePath)
52: Dim LastWriteTime As Date = IO.File.GetLastWriteTime(ImagePath)
53: OneImage.Accessed = LastAccessTime
54: OneImage.Created = CreationTime
55: OneImage.Modified = LastWriteTime
56: Else
57: Console.WriteLine("Not found " & ImagePath)
58: End If
59:
60: Catch ex As Exception
61: Console.WriteLine(OneImage.Folder & "\" & OneImage.Name & vbCrLf & ex.Message)
62: End Try
63: Next
64:
65: Db1.SubmitChanges()
66: End Sub
67:
68: Public Sub InitialCollectImageToDB(SiteRoot)
69:
70: Dim Files = Directory.GetFiles(SiteRoot, "*.*", SearchOption.AllDirectories).
71: Where(Function(X) X.EndsWith(".jpg") Or X.EndsWith(".gif") Or X.EndsWith(".png") Or X.EndsWith(".jpeg"))
72:
73: For Each OneFile In Files
74: Dim DirName As String
75: Dim FileName As String
76: Try
77: Dim RelPath As String = OneFile.Replace(SiteRoot, "")
78: DirName = IO.Path.GetDirectoryName(RelPath)
79: FileName = IO.Path.GetFileName(RelPath)
80: Dim Img As Drawing.Bitmap = New Bitmap(OneFile)
81: Dim CreationTime As Date = IO.File.GetCreationTime(OneFile)
82: Dim LastAccessTime As Date = IO.File.GetLastAccessTime(OneFile)
83: Dim LastWriteTime As Date = IO.File.GetLastWriteTime(OneFile)
84: Dim Exist = (Db1.Images.Where(Function(X) X.Name = FileName And X.Folder = DirName)).FirstOrDefault
85: If Exist Is Nothing Then
86: Db1.Images.InsertOnSubmit(New Image With {.Folder = DirName, .Name = FileName, .X = Img.Width, .Y = Img.Height, .Accessed = LastAccessTime, .Created = CreationTime, .Modified = LastWriteTime})
87: End If
88: Catch ex As Exception
89: Db1.Images.InsertOnSubmit(New Image With {.Name = FileName, .Folder = DirName})
90: Console.WriteLine(OneFile & vbCrLf & ex.Message)
91: End Try
92: Next
93:
94: Db1.SubmitChanges()
95: End Sub
96:
97: End Class
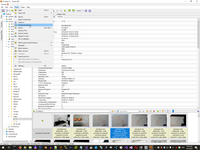
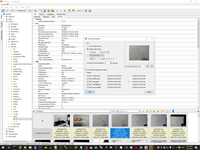
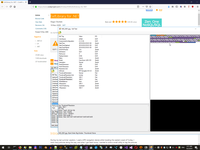
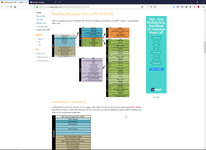

<SITEMAP> <MVC> <ASP> <NET> <DATA> <KIOSK> <FLEX> <SQL> <NOTES> <LINUX> <MONO> <FREEWARE> <DOCS> <ENG> <CHAT ME> <ABOUT ME> < THANKS ME> |