Javascript tests
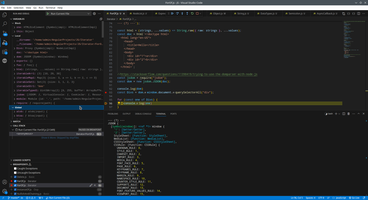
Array
- Array/Array.js
- Array/ArrayLikeObjects.js
- Array/ArrayLoop.js
- Array/ArrayTyped.js
Ar#1 length Ar#2 removes the last element from an array Ar#3 adds a new element at the end of array Ar#4 removes the first array element Ar#5 adds a new element at the beginning of array Ar#6 Changing Elements Ar#7 changing from iterating Ar#8 make element undefined Ar#9 concat array Ar#10 Merging an Array with Values Ar#11 Flattening an array is the process of reducing the dimensionality of an array Ar#12 used to add new items, The first parameter (2) defines the position where new elements should be added, The second parameter (0) defines how many elements should be removed. Ar#13 Using splice() to Remove Elements Ar#14 slices out a piece of an array into a new array. Ar#15 toString() Ar#16 sort Ar#17 New Ar#18 Arrays are a special type of objects Ar#19 foreach Ar#20 map Ar#21 keys Ar#22 iteration for keys Ar#23 array from Ar#24 Transforming a Javascript iterable into an array Ar#25 implicit string converstion Ar#26 changing each array item Ar#27 Array methods are always generic Ar#28 length property, length always ends as an integer Ar#29 Create a string from an array Ar#30 Find the index of an item in an array Ar#31 Check if an array contains a certain item Ar#32 Remove multiple items from the end of an array Ar#33 Truncate an array down to just its first N items Ar#34 Remove the first item from an array Ar#35 Remove multiple items from the beginning of an array Ar#36 Add a new first item to an array Ar#37 concat Ar#38 jagged Ar#39 three way copy Ar#40 deep copy Ar#41 print two-dimensional array Ar#42 Sparse array https Ar#43 forEach.call https Ar#44 create range array with with function and expression
Aj#1 array-like objects, Aside from true arrays, there are also array-like objects that have a length property and properties with all-digits names: NodeList instances, HTMLCollection instances, the arguments object, etc. Aj#1 use for-of with host-provided array-like objects Al#2 use Array.prototype functions with host-provided array-like objects Al#3 Create a true array Al#4 Use spread syntax (...) Al#5 Use the slice method of arrays
Al#1 encient stype Al#2 for Al#3 forEach Al#4 for in Al#5 for iterator Al#6 (6) map Al#7 iterate over Array with iterator
Type of TypedArray At#1 Different way to create Int9Array At#2 example to access data At#3 set up Array and access to it as octet At#4 Multiple views on the same data At#5 TextDecoder At#6 FromCharCode At#7 Conversion to normal arrays At#8 DataView method (+ArrayBuffer and Array.From)
Async
- Async/Async.js
- Async/AsyncCallback.js
- Async/AsyncFunction.js
- Async/AsyncFunctionAsterisk.js
- Async/Await.js
- Async/Fetch.js
- Async/ForAwaitOf.js
- Async/Promise.js
- Async/PromiseAsPrm.js
- Async/PromiseChain.js
- Async/PromiseLocalFS.js
- Async/PromiseWaitAll.js
- Async/PromiseWaitAny.js
- Async/SyncCallback.js
- Async/Timeout.js
As#1 if async function return simple value, that value will wrap to Promise As#2 any Asyc function return Promise, As#3 wait result with then As#4 top-level await with IIFE As#5 parallel executing
Ac#1 callback stack Ac#2 serius of callback. A middleware function will return another function, and a terminator function will invoke the callback.
Af#1 Await is Promise Af#2 Async functions and execution order Af#3 always Use Promise.all instead awaiting many results
Aa#1 simple example with: yield await Promise Aa#2 Async generator functions always produce promises of results — even when each yield step is synchronous. Aa#3 Using an async generator function to read a series of files
Aw#1 The for await...of statement creates a loop iterating over async iterable objects as well as sync iterables. Aw#2 Thenables Aw#3 Awaiting a promise to be fulfilled Aw#4 Thenable objects are resolved just the same as actual Promise objects. Aw#5 Thenable objects can also be rejected: Aw#6 demonstrate how the microtask queue is processed when each await expression is encountered.
Ft#1 simple fetch with JSON Ft#2 Async/Await fitch Ft#3 async IIFE Ft#4 pass result of async function to then Ft#5 error handle
Ao#1 Iterating over async iterables Ao#2 Iterating over async generators Ao#3 Iterating over sync iterables and generators Ao#4 yielding rejected promises from a sync generator - In such case, for await...of throws when consuming the rejected promise and DOESN'T CALL finally blocks within that generator.
Pm#1 fist call executor function creates finalstate Pm#2 correct syntax Pm#3 min access to Promise result from onFulfilled function Pm#4 and onRejected
Pb#1 this function accept promise as prm Pb#2 create Promise from any value Pb#3 created rejected Promise
Ph#1 Promise onFulfill and onReject functions Ph#2 capture error in next chian Ph#3 pass Error to second chain Ph#4 pass promise to second chain Ph#5 pass wrong promise with error to second chain
Lf#1 synchronous reading node specific Lf#2 wrap synchronous reading to promise task Lf#3 standard asynchronous reading
Pw#1 setTimeut return Promise Pw#2 working even simple value instead Promise Pw#3 handle reject Pw#4 unhaddled rejection Pw#5 imediate rejection
Pa#1 start on Promise WaitAll
Sc#1 async function show the same time because it invoke synchronously
Tt#1 Promise timeout merge to one
Basic
- Basic/Clone.js
- Basic/Compare.js
- Basic/Delete.js
- Basic/DestructuringAssignment.js
- Basic/Eval.js
- Basic/In.js
- Basic/NullishAndChaining.js
- Basic/Object.js
- Basic/PredefinedFunctions.js
- Basic/Property.js
- Basic/StrictModeOff.js
- Basic/Xhr2.js
Cl#1 Shallow_copy - object is a copy whose properties share the same references Cl#2 deep clone with structuredClone and Object.assign() Cl#3 Transferring values = clone an array and transfer its underlying resources to the new object. On return, the original uInt8Array.buffer will be cleared Cl#4 use ArrayBuffer and then clone the object it is a member of, transferring the buffer. Cl#5 Object.assign() copies all enumerable own properties from one or more source objects to a target object. It returns the modified target object. Cl#6 But can not use for deep clone like structuredClone() If the source value is a reference to an object, it only copies the reference value. Cl#7 Copying symbol-typed properties Cl#8 Copying accessors alternate way to deap copy on Ar#40 (JSON)
Cm#1 compare value and type Cm#2 compare bigint (0n) with other Cm#3 This use case demonstrates an instance of the Liskov substitution principle Cm#4 Similar to same-value equality, but +0 and -0 are considered equal. Cm#5 Object.is() and NaN
Dl#1 delete and the prototype chain Dl#2 delete global this (don't working in strict mode)
Da#1 assignment like x = y = 5 Da#2 Assignment with destructuring, Unpacking values from a regular expression match Da#3 common syntax of destructing Assignment Da#4 Swapping variables Da#5 Using a binding pattern as the rest property Da#6 unpacking from any iterable Da#7 Unpacking properties from objects passed as a function parameter Da#8 unpacking object Da#9 inside for-of Da#10 the prototype chain is looked up when the object is deconstructed
Ev#1 eval(script), script - A string representing a JavaScript expression, statement, or sequence of statements. Direct call eval version Ev#2 For if, it would be the last expression or statement evaluated. Ev#3 If you assign multiple values then the last value is returned. Ev#4 eval() as a string defining function requires "(" and ")" as prefix and suffix. Function declaration vs function expression Ev#5 Indirect call version using the comma operator to return eval Ev#6 Uses global scope, throws because x is undefined Ev#7 strict mode Ev#8 source eval string is in strict mode Ev#9 eval new.target Ev#10 var-declared variables and function declarations would go into the surrounding scope if the source string is not interpreted in strict mode — for indirect eval, they become global variables. Ev#11 Context is strict, but this is indirect eval and the source string is not strict Ev#12 indirect eval - Simply using indirect eval and forcing strict mode. The two code snippets above may seem to work the same way, but they do not; the first one using direct eval suffers from multiple problems. Ev#13 The Function() constructor is very similar to the indirect eval Ev#14 Using bracket accessors to access properties dynamically. Ev#15 the same with descendant properties obj.a.b.c
In#1 The in operator returns true if the specified property is in the specified object or its prototype chain In#2 In on array used as property (index or other) In#3 Using the in operator with deleted or undefined properties In#4 If you set a property to undefined but do not delete it, the in operator returns true for that property. In#5 Inherited properties object - hasOwn In#6 Using the in operator to implement branded checks In#7 You can also implement this as a @@hasInstance [Symbol.hasInstance] method of the class, so that you can use the instanceof operator to perform the same check
Nc#1 logical nullish assignment operator, only evaluates the right operand and assigns to the left if the left operand is nullish (null or undefined). Nc#2 Nullish coalescing operator ?? Nc#3 || Optional chaining Nc#4 Assigning a default value to a variable Nc#5 Short-circuiting
Ob#1 common Object syntax and initializer Ob#2 you should directly call() the Object.prototype method on your target object instead, to prevent the object from having an overriding property that produces unexpected results. Ob#3 create object from NULL prototype with Object.create(null) Ob#4 creating from NULL prototype can be interesting, this function working wrong Ob#5 but this working correctly Ob#6 Making your sensitive object not inherit from Object.prototype also prevents prototype pollution attacks. Ob#7 Constructing empty objects Ob#8 create Boolean objects Ob#9 Modifying the object prototype property Ob#10 get keys and value from Object Ob#11 JSON Indented format (usefull function) Ob#12 Detecting an undefined object property with hasOwnProperty or with ==== Ob#13 object methods create/defineProperty/getOwnPropertyDescriptor/getOwnPropertyNames/getPrototypeOf/keys Ob#14 protection object methods preventExtensions/isExtensible/seal/isSealed/freeze/isFrozen Ob#15 serialise Obj with circular reference
Pf#1 The decodeURI() / encodeURI() method encodes a Uniform Resource Identifier (URI) by replacing each instance of certain characters by one, two, three, or four escape sequences representing the UTF-8 encoding of the character Pf#2 The decodeURIComponent()/ encodeURIComponent() method encodes a Uniform Resource Identifier (URI) component by replacing each instance of certain characters by one, two, three, or four escape sequences representing the UTF-8 encoding of the character Pf#3 redirect standard console.log by redefine function console.log to variable also look to Html Folder to list of all global function on Node.JS and Browser
Pr#1 computed property names like [`foo${++i}`] Pr#2 computed property names like [Array] Pr#3 computed property names like [variables] Pr#4 Spread object properties to merged object with ...
So#1 error because variable is not declared So#2 This, Only for demonstration — you should not mutate built-in prototypes like Number.prototype.getThis === globalThis So#3 compare with This.js - currently this 5 functions return false, with strict mode on - all of that comparing return true So#4 function local scope (only the code inside the function is in strict mode): So#5 Deleting a variable (or object) is not allowed in strict mode So#6 Octal numeric literals are not allowed in strict mode So#7 Octal escape characters are not allowed in strict mode So#8 Writing to a read-only property is not allowed in strict mode So#9 get-only property is not allowed in strict mode So#10 Deleting an undeletable property is not allowed in strict mode So#11 eval, arguments, implements, interface, let, package, private, protected, public, static, yield - can not use as identifier in strict mode So#12 The with statement is not allowed in strict mode So#13 variable can not be used before it is declared in strict mode So#14 eval() can not declare a variable in strict mode
Xr#1 use XMLHttpRequest
ControlFlow
- ControlFlow/DoWhile.js
- ControlFlow/Goto.js
- ControlFlow/Other.js
Dw#1 do while Dw#2 while Dw#3 const html = (strings, ...values) => String.raw({ raw: strings }, ...values); Dw#4 create createNodeIterator on dom.window.document Dw#5 Many style guides recommend putting additional parentheses as a grouping operator () around the assignment in While - while ((currentNode = iterator.nextNode())) {}
Gt#1 Goto on Continue Gt#2 Goto on Break for
Ot#1 nested ternary operator Ot#2 Break on block {} Ot#3 switch Ot#4 for-each loop
Function
- Function/ArrowFunction.js
- Function/ExecuteFunctionAsLiteral.js
- Function/Function.js
- Function/FunctionApplyBindCall.js
- Function/FunctionCallback.js
- Function/FunctionClosure.js
- Function/FunctionPrototype.js
- Function/FunctionReference.js
- Function/ImmediatelyInvokedFunction.js
- Basic/NewFunction.js
- Function/UseArguments.js
Fa#1 syntax cases Fa#2 code inside braces ({}) is parsed as a sequence of statements, where foo is a label, not a key in an object literal. To fix this, wrap the object literal in parentheses: Fa#3 you may put the line break after the arrow or use parentheses/braces around the function body Fa#4 Arrow function expressions should only be used for non-method functions because they do not have their own this Fa#5 this inside the arrow function's body will correctly point to the instance (or the class itself, for static fields). However, because it is a closure, not the function's own binding, the value of this will not change based on the execution context Fa#6 Arrow functions do not have their own arguments object. Fa#7 Cannot be used as constructors Fa#8 With arrow functions, function is essentially created on the globalThis (global) scope, it will assume this is the globalThis Fa#9 this on array.forEach with ordinary and arrow functions, all arrow functions lexically bind the this
Fl#1 execute function as literal
Fu#1 Constructor new Function Fu#2 Declaration Fu#3 Function expressions; the function is anonymous but assigned to a variable Fu#4 Expression; the function has its own name Fu#5 Arrow function Fu#6 define Method and Get/Set function in object Fu#7 Object, Default and Array (with Spread syntax) as function parameters Fu#8 obj as parameters Fu#9 Invoking a Function with a Function Constructor NEW Fu#10 Named function expression
Fb#1 Using call, bind, and apply Fb#2 Function Borrowing with the bind() method, an object can borrow a method from another object. Creates a bound function that has the same body as the original function. The this object of the bound function is associated with the specified object, and has the specified initial parameters. Fb#3 Call() Fb#4 Call with parameters Fb#5 apply() Fb#6 apply can used for calculate Max Method on Arrays Fb#7 Using call() and apply(), you can pass the value of this as if it's an explicit parameter. Fb#8 f.bind(someObject) creates a new function with the same body and scope as f,
Fk#1 callback function on map Fk#2 Callback with Rest parameters
Fc#1 Nested Functions and closure, see more in Closure Fc#2 closure on self-invoking functions (arrow function working in global context) Fc#3 This point to global context in arrow function Fc#4 arrow function fn2()() === globalThis is true in non-strict mode Fc#5 Nested functions and closures Fc#6 Name conflict in Closure
Fp#1 The function with custom prototype
Fe#1 function can apply to any array elements, pass function reference as parameter
Fi#1 Immediately Invoked Function Expression Fi#2 IIFE with arrow Function Fi#3 Async IIFE
Fn#1 Function constructor Fn#2 The function constructor can take in multiple statements separated by a semicolon. Function expressions require a return statement with the function's name
Fm#1 apply arguments for function parameters Fm#2 arguments look as array-like object, but this is not array!
DataTypes
- DataTypes/Map.js
- DataTypes/RegExp.js
- DataTypes/Symbol.js
Mp#1 The Map object holds key-value pairs and remembers the original insertion order of the keys. Mp#2 create function and function call more readable Mp#3 converting string to array Array.prototype.map.call Mp#4 can transformed to Array with entries() - IterableIterator Mp#5 Reformatting Array to Objects
Rg#1 Matching duplicate words with text.match Rg#2 Assignment with destructuring, Unpacking values from a regular expression with exec
Sm#1 Every Symbol() call is guaranteed to return a unique Symbol. Sm#2 Symbol wrapper around object, TypeOf symbol and typeof wrapper Sm#3 Symbol.for(tokenString) takes a string key and returns a symbol value from the registry, while Symbol.keyFor(symbolValue) takes a symbol value and returns the string key corresponding to it. Sm#4 typeof operator with Symbols, Symbols(object), Symbol.iterator Sm#5 Symbols and for...in iteration Sm#6 Symbols and JSON.stringify() Sm#7 Symbol wrapper objects as property keys
Html
- Html/AllDomExample.txt
- Html/AllHtmlExample.html
- Html/Angular.txt
- Html/Canvas.html
- Html/CookieProxy.html
- Html/CustomControl.html
- Html/DetectUniqueBrowser.html
- Html/GlobalContext-Chrome.txt
- Html/GlobalContext-Firefox.txt
- Html/GlobalContext-Node.txt
- Html/Global.html
- Html/Html5.html
- Html/HtmlDom1.js
- Html/HtmlDom2.js
- Html/IIFE-SortTable.html
- Html/Index.html
- Html/LocalStorage.txt
- Html/PostForms.html
- Html/Transitions.txt
- Html/VideoAudio.txt
- Html/WebGL.txt
- Html/Webworker.txt
Dm#1 Create Html dom with jsdom
Dm#2 Create Html dom with html-element, return Promise
Iterator
- Iterator/ForIn.js
- Iterator/ForOf.js
- Iterator/Generator.js
- Iterator/Iterator.js
- Iterator/Spread.js
Fr#1 for...in loop only iterates over enumerable, non-symbol properties. Fr#2 we can use of Object.hasOwn(): the inherited properties are not displayed. Fr#3 Shadowed properties are only visited once: Fr#4 Deleting a property during iteration: Fr#5 Changing the prototype during iteration: Fr#6 Difference between for...of and for...in - The for...in statement iterates over the enumerable string properties of an object, while the for...of statement iterates over values that the iterable object defines to be iterated over.
Ff#1 Iterating over an Array Ff#2 Iterating over a string Ff#3 Iterating over a TypedArray Ff#4 Iterating over a Map Ff#5 Iterating over a Set Ff#6 Iterating over the arguments object Ff#7 Iterating over a NodeList - dom.window.document.querySelectorAll Ff#8 Iterating over a user-defined iterable - Iterating over an object with an @@iterator [Symbol.iterator]() method that returns a custom iterator Ff#9 Iterating over an object with an @@iterator generator method *[Symbol.iterator]() Ff#10 Iterable iterators (iterators with a [@@iterator]() method that returns this) are a fairly common technique to make iterators usable for for...of Ff#11 Iterating over a generator function* Ff#12 Execution of the break statement in the first loop causes it to exit early. The iterator is not finished yet, so the second loop will continue from where the first one stopped at. Ff#13 Difference between for...of and for...in
Gn#1 function generator function* gen() { yield* [];} gen().next() Gn#2 Generator Function Gn#3 GeneratorFunction.prototype.constructor return instance of GeneratorFunction Gn#4 infinite generator Gn#5 async generator function async function* [yield await Promise] Gn#6 operators yield* vs yield
It#1 @@iterator the same is [Symbol.iterator]() looks as ForEach(), simplest iterator over array items - array.keys() It#2 For of It#3 Transforming a Javascript iterable into an array array.entries() It#4 Array.prototype[@@iterator]()] - check typeOf iterable parameter It#5 iterable iterator (function* () { yield {}; }) (), It#6 array and parameters spreading ... from *[Symbol.iterator]() {} It#7 spreading chars from string It#8 desctructing assignment from set It#9 create User-defined iterables It#10 unpack uder defined iterables to array It#11 and read unpacked value with array.map It#12 for-of over User-defined iterables It#13 pass break to iterables perform return() instead next() It#14 Defining custom iterator function from array, index in Iterators are stateful It#15 Defining an iterable property (Simple Class As Array with index) It#16 You can redefine the iteration behavior by supplying our own @@iterator It#17 create createNodeIterator on dom.window.document
allows an iterable, such as an array or string. used in (1) function argument, (2) array, (3) literal and (4) object Sp#1 1. Replace apply() in Function arguments list Sp#2 2. Spread in array Sp#3 make a shallow copy of an array Sp#4 Apply for new operator - When calling a constructor with new, it's not possible to directly use an array and apply(), because apply() calls the target function instead of constructing it, which means, among other things, that new.target will be undefined, therefore only this way is possible Sp#5 concatenate arrays instead Array.prototype.concat() Sp#6 Conditionally adding values to an array Sp#7 3. Spread in object Sp#8 Conditionally adding properties to an object, no key at all with Object.keys(). Sp#9 Object.assign() can be used to mutate an object, whereas spread syntax can't.
Meta
- Meta/MapKeyValuePair.js
- Meta/ProxyReflect.js
- Meta/Set.js
- Meta/Week.js
Kv#1 Map is KeyValue set Kv#2 Map Object Kv#3 NaN Kv#4 Iteration with For [key, value] of myMap Kv#5 iteration Map.forEach((value, key) => {} Kv#6 Relation with Array objects new Map(Array), Array.from(Map), [...Map] Kv#7 Map can clone Kv#8 Map can Merge Kv#9 Map can megre with array Kv#10 create from Array Kv#11 add item Kv#12 Being able to use objects as keys is an important Map feature. Kv#13 for-of iteration Kv#14 Keys
Pl#1 Proxy object can intercept and redefine fundamental operations for that object. The Reflect method still interacts with the object through object internal methods — it doesn't "de-proxify" the proxy if it's invoked on a proxy. Pl#2 Before Reflect, you typically use the Function.prototype.apply() method to call a function with a given this value and arguments provided as an array (or an array-like object). Pl#3 With Reflect.apply this becomes less verbose and easier to understand
St#1 JavaScript Set is a collection of unique values. Each value can only occur once in a Set. A Set can hold any value of any data type. St#2 Set looks as ordinary Ilist of data St#3 Set.add St#4 set from string St#5 Set.forEach St#6 Set.values() St#7 Set.keys() St#8 A Set has no keys, entries() returns [value,value] pairs instead of [key,value] pairs on Map. St#9 Sets are Objects, typeof Set, instanceof Set St#10 Iterating sets St#11 Keys, Value, entries St#12 Convert Set object to an Array object, with Array.from St#13 Iteration with fileter St#14 Use to remove duplicate elements from an array
Wk#1 A WeakSet is a collection of garbage-collectable values, including objects and non-registered symbols. Example 1. Wk#2 example 2 Wk#3 example 3 Wk#4 A WeakMap is a collection of key/value pairs whose keys must be objects or non-registered symbols, with values of any arbitrary JavaScript type, Example 1 Wk#5 WeakMap Example 2
Node
- Node/AsyncHook.js
- Node/AsyncStateManagement.js
- Node/CalcScriptTime.js
- Node/ConsoleRead.js
- Node/EventEmitter.js
- Node/EventLoop.js
- Node/GlobalThis.js
- Node/JsonParse.js
- Node/LocalFS.js
- Node/LongCalc.js
- Node/Process.js
- Node/Server.js
- Node/SimplePropertyRetriever.js
- Node/WebWorker.js
- Node/WorkerThreads.js
Ah#1 node:async_hooks, If Workers are used, each thread has an independent async_hooks interface, and each thread will use a new set of async IDs. Ah#2 Asynchronous context example. The context tracking use case is covered by the stable API AsyncLocalStorage.
Tm#1 accuracy measure script working time with setTimeout(() => console.timeEnd())
Cr#1 console input on node
Ee#1 emit events with EventEmitter.emit and process events Ee#2 pass arguments
El#1 JavaScript runtime collecting events, and executing queued sub-tasks of accumulated event El#2 how Node call simple thread JS-program El#3 IIFE function executed immediatelly, but callaback with setTimeout after all main stack calculation finished
print All Node.js global objects Th#1 globalThis-getOwnNonenumerables Th#2 globalThis-getOwnEnumerables Th#3 globalThis-getPrototypeNonenumerables Th#4 globalThis-getPrototypeEnumerables
Fs#1 Tab Session Manager parser
Fs#2 local file read/write sync and async
Tm#2 long calculation on worker_threads (separate threads)
Tp#1 print Node process list
Ex#1 simple Express API REST server on port 3000
Rw#1 Enumerability all properties
Ww#1 worker threading
Wt#1 start longcalculations and measure time console.end
Oop
- Oop/Abstract.js
- Oop/Class.js
- Oop/Extend.js
- Oop/ExtendHTML.js
- Oop/InstanceOf.js
- Oop/NewTarget.js
- Oop/Private.js
- Oop/Prototype.js
- Oop/Super.js
- Oop/tracePrototypeChainOf.js
Ab#1 Abstract Method Ab#2 Abstract Class
Cl#1 encapsulation private field Cl#2 get/set Accessor fields Cl#3 Bound methods in classes this.x.bind(this), The value of 'this' in methods depends on their caller https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Operators/this also Example of Static constructor and instanceof / @@hasInstance see on In.js , Super.js, Function.js
Ex#1 private fields Ex#2 A class's constructor can return a different object, which will be used as the new this for the derived class constructor. also see Prototype to extend
Eh#1 #masket from external using
Tn#1 objects, defining constructor and check prototype chain with instanceof Tn#2 Re-assign `constructor.prototype` (you should rarely do this in practice) and check prototype chain with instanceof Tn#3 string leieral vs String object Tn#4 Objects created using Object.create() and check instanceof Tn#5 Object with null prototype Tn#6 Overriding the behavior of instanceof - static [Symbol.hasInstance] https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Operators/instanceof#overriding_the_behavior_of_instanceof Tn#7 extends class with custom instanceof method and check class chain
Nt#1 The new.target meta-property lets you detect whether a function or constructor was called using the new operator. new.target is guaranteed to be a constructable function value or undefined. Nt#2 use new.target to check that class instantiated with new Nt#3 extend Map (KeyValuePair from lib.es2015.collection.d.ts) with operation InsertOrUpdate
Pv#1 You can use the in operator to check whether an externally defined object possesses a private property. Pv#2 #privateField from the ClassWithPrivateField base class is private to ClassWithPrivateField and is not accessible from the derived Subclass Pv#3 A class's constructor can return a different object, which will be used as the new this for the derived class constructor. Pv#4 Simulating private constructors
Pt#1 Objects Pt#2 Array Pt#3 Function Pt#4 Constructor Pt#5 Access to prototype getPrototypeOf/setPrototypeOf, __proto__ keyword Pt#6 set prototype using Object.create Pt#7 Using a prototype constructor with Object.assign Pt#8 Own property Pt#9 To build longer prototype chains, we can set the [[Prototype]] of Constructor.prototype via the Object.setPrototypeOf() function. Pt#10 In class terms, this is equivalent to using the extends syntax. Pt#11 With constructor functions Pt#12 Object.create() creates a new object. The [[Prototype]] of this object is the first argument of the function: Pt#13 The instanceof operator tests to see if the prototype property of a constructor appears anywhere in the prototype chain of an object
Sp#1 super (MyBase in VB) Sp#2 Setting super.prop sets the property on this instead Sp#3 non-writable property Sp#4 ReferenceError to super constructor
Pc#1 Trace Prototype Chain
Puppeteer
- Puppeteer/Start.js
Pp#1
SimpleDataTypes
- SimpleDataTypes/Bigint.js
- SimpleDataTypes/DataTypes.js
- SimpleDataTypes/Date.js
- SimpleDataTypes/Numbers.js
- SimpleDataTypes/ParseIntAndFloat.js
- SimpleDataTypes/TemplateString.js
- SimpleDataTypes/TypeConversrion.js
- SimpleDataTypes/TypeOf.js
- SimpleDataTypes/Void.js
Bg#1 bigint created by appending n to the end of an integer literal, or by calling the BigInt() function (without the new operator), Only use a BigInt value when values greater than 2^53 are reasonably expected. Bg#2 Operation with Bigint - most do not permit operands to be of mixed types — both operands must be BigInt Bg#3 compare Bigint with zero Bg#4 comparisons with Object-wrapped BigInt Bg#5 logical compare with Bigint Bg#6 BigInt values aren't serialized in JSON by default, Bg#7 However, JSON.stringify() has toJSON() method Bg#8 custom parser function of BigInt object Bg#9 Transform string to BigNumber value on Json Bg#10 example calculation with Bigint
Dt#1 Variables in JavaScript are not directly associated with any particular value type, and any variable can be assigned (and re-assigned) values of all types: Dt#2 conversion of week types Dt#3 NULL Dt#4 See more in TypeOf.js Dt#5 Hexadecimal escape sequences Dt#6 Unicode escape sequences See more in TypeOf.js , TemplateString
De#1 Date object is slightly smaller than the maximum safe integer (Number.MAX_SAFE_INTEGER, which is 9,007,199,254,740,991). A Date object can represent a maximum of ±8,640,000,000,000,000 milliseconds De#2 now and New Date return milliseconds De#3 Get formatting parts of date (local and UTC), the same methods to Set De#4 Date.parse string De#5 Date time get formatting string result De#6 measure interval
Nm#1 JavaScript Numbers are Always 64-bit Floating Point Nm#2 limits Nm#3 JavaScript BigInt is a new datatype (ES2020) that can be used to store integer values that are too big to be represented by a normal JavaScript Number. Nm#4 The JavaScript interpreter works from left to right. Nm#5 NaN - Not a Number Nm#6 typeof NaN returns number: Nm#7 operation with NaM Nm#8 Infinity (or -Infinity) is the value JavaScript will return if you calculate a number outside the largest possible number. Nm#9 hexadecimal preceded by 0x. Nm#10 toString() method to output numbers from base 2 to base 36. Nm#11 JavaScript Numbers as Objects Nm#12 Note the difference between (x==y) and (x===y). Nm#13 Comparing two JavaScript objects always returns false. Nm#14 toFixed Nm#15 Number ParseInt Nm#16 Number ParseFloat Nm#17 function determines whether a value is NaN or not. Note: coercion inside the isNaN function has interesting rules. You may alternatively want to use Number.isNaN() to determine if the value is Not-A-Number. Nm#18 The global isFinite() function determines whether the passed value is a finite number. If needed, the parameter is first converted to a number. More robust Number.isFinite()
Pi#1 The parseInt() parses a string argument and returns an integer of the specified radix (the base in mathematical numeral systems). Does not handle BigInt values and see Math.Trunc() Pi#2 The parseFloat() function parses a string argument and returns a floating point number.
Ts#1 escape char and long string Ts#2 Raw strings allows without processing escape sequences. Ts#3 template litheral on String.raw Ts#4 string array join Ts#5 escape a backtick and dollar Ts#6 Use backtick to create multiline string Ts#7 String interpolation Ts#8 Nesting templates Ts#9 Tagged templates Ts#10 The tag does not have to be a plain identifier. Ts#11 Nesting templates literal syntax and closures Ts#12 For any particular tagged template literal expression, the tag function will always be called with the exact same literal array, no matter how many times the literal is evaluated. Ts#13 passes literal array to String.raw Ts#14 format this literal's content as HTML Ts#15 Tagged templates and escape sequences \unicode
Cn#1 All type coercion algorithms look up this symbol on objects for the method that accepts a preferred type and returns a primitive representation of the object, before falling back to using the object's valueOf() and toString() methods Cn#2 An object with Symbol.toPrimitive property. Cn#3 there's no strong preference for what the actual type should be. This is usually when a string, a number, or a BigInt are equally acceptable . Objects are converted to primitives by calling its [@@toPrimitive]() (with "default" as hint), valueOf(), and toString() methods, in that order. Cn#4 Unary plus performs number coercion on its operand, which, for most objects without @@toPrimitive, means calling its valueOf()
Tf#1 typeof Numbers Tf#2 typeof bigint Tf#3 typeof Strings Tf#4 typeof Booleans Tf#5 typeof Symbols Tf#6 typeof Undefined Tf#7 typeof Objects, Array, new Date, new Boolean, new Number, new Strin Tf#8 Functions Tf#9 use Array.isArray or Object.prototype.toString.call to differentiate regular objects from arrays Tf#10 Object.prototype.toString.call
Vd#1 The void operator evaluates the given expression and then returns undefined. Vd#2 void console.log Vd#3 iife console.log Vd#4 void block executing function at all
Syntax
- Syntax/Brackets.js
- Syntax/Comma.js
- Syntax/Expression.js
- Syntax/Group.js
- Syntax/Jsdoc.js
- Syntax/Semicolon.js
Ev#14 Using bracket accessors to access properties dynamically. Pr#1 computed property names Ob#1 common Object syntax and initializer Mp#5 Reformatting Array Objects Sm#5 Symbols and for...in iteration Sm#7 Symbol wrapper objects as property keys Rg#2 Assignment with destructuring, Unpacking values from a regular expression with exec Da#6 unpacking from any iterable It#6 (5) array and parameters spreading ... from *[Symbol.iterator]() {}
Co#1 assignment expression, not a declaration, previous expression before comma is discarded Co#2 x = y = 3 - x value assign from y value, what assign from 3 Co#3 printing the values of the diagonal elements in the array - used string expression plus cycle with two variable, in this case coma is declaration Co#4 Processing and then returning last value (x += 1, x) the same as ++x Co#5 Comma and grouping operation in arrow function [].map((x) => ((sum += x), x * x)); Co#6 the grouping operator still returns the reference, the comma operator returns a new value Co#7 The comma operator always returns the last expression as a value instead of a reference.
Ep#1 function call like [x].forEach(y) Ep#2 template literal `${x}` Ep#3 Assignment operators like [x, ...y] = z Ep#4 increment operator like x++ or ++x Ep#5 Delete statement Ep#6 Import statement Ep#7 yield generator function Ep#8 yield* operator
Gr#1 In an arrow function expression body (one that directly returns an expression without the keyword return), the grouping operator can be used to return an object literal expression Gr#2 since when the parser sees the left parenthesis, it knows that what follows must be an expression instead of a declaration. Gr#2 using for without block Gr#3 Empty statement Gr#4 Assign all array values to 0 with empty statement, Using a block statement to encapsulate data Gr#5 Many style guides recommend putting additional parentheses as a grouping operator around the assignment: Gr#6 code inside braces ({}) is parsed as a sequence of statements, where foo is a label, not a key in an object literal. To fix this, wrap the object literal in parentheses:
Ab#2 jsdoc comment
Sl#1 Automatically insert semiclon. This code return undefined, because a semicolon is inserted directly after the return keyword, without evaluating a + b
ThisContext
- ThisContext/Closure.js
- ThisContext/Scope.js
- ThisContext/This.js
Cs#1 What is closure Cs#2 var are either function-scoped or global-scoped. This can be tricky, because blocks with curly braces do not create scopes Cs#3 let and const allow you to create block-scoped variables Cs#4 makeAdder is a function factory, the function factory creates two new functions—one that adds five to its argument, and one that adds 10. Cs#5 Emulating private methods with closures, in this example each closure had its own lexical environment Cs#6 The shared lexical environment is created in the body of an anonymous function, which is executed as soon as it has been defined (also known as an IIFE). Cs#7 Every closure has three scopes: Local scope (Own scope), Enclosing scope (can be block, function, or module scope), Global scope Cs#8 the same without anonimous functions Cs#9 Closures can capture variables in block scopes and module scopes as well, For example, the following creates a closure over the block-scoped variable y Cs#10 Closures over modules can be more interesting.....
Se#1 what is scope Se#2 var declarations are globally scoped or function/locally scoped. Se#3 let is block scoped, Unlike var which is initialized as undefined, the let keyword is not initialized Se#4 let can be updated but not re-declared. Se#5 const declarations are block scoped, const cannot be updated or re-declared
Ti#1 What is this? Ti#2 this in strict mode (compare result with StrictModeOff) Ti#3 this will be a primitive value as well — but only if the function is in strict mode. Ti#4 Callbacks are typically called with a this value of undefined (calling it directly without attaching it to any object), which means if the function is non–strict Ti#5 Callbacks with parameters Ti#6 In arrow functions when evaluating an arrow function's body, the language does not create a new this binding Ti#7 In arrow function this is bound to what it was when the function was created Ti#8 bind() method has to be used to prevent losing this. Ti#9 this with a getter or setter
JS project context:
Some examples of my projects related to Javascript/Typescript/Node/Angular:
- I made a number of projects with Angular (server side, client side and mobile development on Angular), this is some examples:
- This is project with Nest.JS and TypeORM, just a couple of screens Server Angular (NestJs) learning.
- About Node backend Typescript ORM: Test project with Nest.js and Sequelize ORM.
- A couple of years I have rebuild Blazor project to Angular, this is some page from that job [Angular mosaic 1] My fast style, Intercept routing events (router-outlet onActivate event, @Input, extends base page).
- A lot of small project, for example Simple AngularFirebaseUploader as Typescript console application - npx ts-node (function main).
- A couple of investigation about modal popup windows on Angular 5 way to create modal windows with Angular (Angular material, UIKit with toggle tag, UIKit with custom function, jQuery UI modal, Bootstrap modal).
- This is my Mobile application on Angular NativeScript and Angular form
- About my Angular frontend what I made from scratch Processing Backend result in Angular frontend as typed data
- This is another ASP.NET Core project with Angular frontend, I just made refactoring of this project Key future of RPAT backend project.
- I have created a couple of Node.JS projects without Angular, this is my top projects:
- I made some changing in this project Brief of real workable NestJs application.
- Node backend project (clone of bountycompetitions) My ticket lottery engine based on SQL CTE procedure.
- Node backend project to support this blog Node project for support this blog.
- I made a lot of various project for WebWorker, for example:
- My Telegram bots Telegram bots and Telegram MiniApps.
- My Cloudflare proxy Cloudflare proxy with special CORS policy.
- Project for himself Project to move my blog from private VM with Cloudflare VPN to Google Drive and Cloudflare R2 storage
- I made number of projects with Electron:
- Angular Electron project: My workable project template for Angular Electron.
- My Electron video player Electron video player with cashing and CSP policy.
- I made number of projects with clear JS, for example:
- RxJs and animation RxJs animation
- Also I try to write lectures about Javascript, please look its to this page:
- My JS Lecture My lecture about Javascript (Ukrainian language).
- And, of course, I made a lot of ASP.NET Classic and ASP.NET MVC especially focused to javascript frontend, for example:
ES6 context:
- (2025) Basic workflow to working with Images (CSP, CORS), (Temporary URL as blob, Encoding image as data:image), (Fetch, Browser FileReader, Node-Fetch), (ArrayBuffer, TypedArray, DataView, HexConversion), DataStream (Concatenate Chunks, Convert data inside stream), (Image server, Image Proxy server). #Browser #FrontLearning #ES6
- (2024) Notes about JS Closures. #ES6
- (2024) Notes about Javascript asynchronous programming. #ES6
- (2022) Modern Javascript books #ES6 #Doc
- (2021) JS learning start point #ES6
- (2021) Maximilian Schwarzmüller Javascript lecture #ES6
- (2021) Javascript interview question from Happy Rawat #ES6
- (2021) Javascript tests #ES6
- (2016) New unique features of Javascript (updated). #ES6
Comments (
)

Link to this page:
http://www.vb-net.com/JsTest/Index.htm
|