Combine Javascript and VB.NET to one application.
1. Integrate JavaScript code .NET framework.
Combine Javascript and VB.NET to one application is possible by 3 ways:
:- 1. Basic integration.
- 2. Use a .NET JavaScript Engine:
- • Mechanism: .NET provides ways to embed and run JavaScript code. You'd use a JavaScript engine (like ChakraCore or V8, which can be accessed through .NET libraries) within your Windows Forms application.
- • Implementation: Your .NET code would host the JavaScript engine, pass data to your JavaScript functions, and receive results back from the engine. This method requires writing C# code to interact with the engine API and handle data marshaling between the .NET and JS environments.
- • Advantages: No external dependencies beyond the .NET framework, potentially better performance than IPC.
- • Disadvantages: More complex to implement, requires proficiency in both VB.NET and JavaScript, error handling needs careful consideration for both the .NET and JS sides. Might only be suitable if the JS portions are small and relatively self-contained.
- 3. Create a Separate Node.js Service (and IPC):
- • Mechanism: Create a separate Node.js service (a small server application) that exposes the JS functionality via HTTP or some other IPC method. Your .NET WinForms application would act as a client, sending requests to the Node.js service and receiving responses. This approach is similar to the IPC method previously described but doesn't use direct inter-process communication within the same machine.
- • Implementation: This approach is more flexible for larger JS codebases. Use a framework like Express.js in your Node.js server and network APIs in .NET. This is also the best choice if the JS portion might eventually need to run on a different machine.
- • Advantages: Cleaner separation of concerns. Easier to test and maintain the JS code independently.
- • Disadvantages: Adding network overhead (if HTTP is used), adds more complexity to the architecture.
- 4. Use a COM Object (look more detail in pages ComObject Tag ):
- • Mechanism: If your JavaScript code is wrapped as a COM (Component Object Model) object, you can potentially access it from your .NET WinForms application. This is an advanced technique best suited for applications that were designed from the outset to use COM.
- • Implementation: You would need to create a COM server from your JavaScript code (a significant undertaking). Then you would need to use COM interop in your .NET code to use the server.
- • Advantages: Could offer tighter integration if COM is a feasible option.
- • Disadvantages: Very complex to set up, COM is not the preferred interoperability method in most modern contexts.
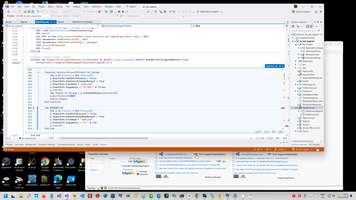
Or something like this:
1: Public Async Function RunNodeJsModule(projectPath As String, scriptName As String, ParamArray args As String()) As Task(Of String)
2: Dim ModulePath As String = Path.Combine(projectPath, scriptName)
3: Dim Arguments As String = String.Join(" ", args)
4: Dim StartInfo As New ProcessStartInfo("node", $"{ModulePath} {Arguments}")
5: StartInfo.RedirectStandardOutput = True
6: StartInfo.UseShellExecute = False
7: StartInfo.WorkingDirectory = projectPath
8: Using Process As New Process With {.StartInfo = StartInfo}
9: Process.Start()
10: Dim Output As String = Await Process.StandardOutput.ReadToEndAsync()
11: Process.WaitForExit()
12: If Process.ExitCode <> 0 Then
13: Dim ErrorMessage As String = Await Process.StandardError.ReadToEndAsync()
14: Throw New Exception($"Node.js script exited with error code {Process.ExitCode}: {ErrorMessage}")
15: End If
16: Return Output
17: End Using
18: End Function
2. What is Inter-Process Communication (IPC)
Look more details to page My workable project template for Angular Electron) and look to this project Rebuilding my ancient API factory on modern way (Cryptor, Windows services, IIS management, Windows Task Scheduler, TcpListener, WCF, gRPC, Port Sharing service)
- 1. Inter-Process Communication (IPC) using Named Pipes or Sockets:
- • Mechanism: Your .NET WinForms app and your Electron app would each run as separate processes. They would communicate using named pipes or sockets. This is a common, robust approach for inter-process communication, even across different programming languages.
- • Implementation: In .NET, you'd use the NamedPipeServerStream (for pipes) or a socket library. In your Electron app (using Node.js), you'd use the net module (for sockets) or a library that simplifies named pipe interaction. Each process would listen for and send messages over the designated communication channel.
- • Data Transfer: You'd need to serialize data (convert it to a common format like JSON) before sending it between processes.
- • Advantages: Relatively simple to implement, good performance for most use cases.
- • Disadvantages: Requires more manual coding for IPC handling, error handling needs to be considered carefully for each process.
- 2. Shared Memory:
- • Mechanism: This approach is more complex but potentially offers better performance for large amounts of data exchange. Your .NET and Electron apps would share a designated region of memory. Changes in one application would be visible to the other.
- • Implementation: In .NET, you'd use memory-mapped files or other shared memory mechanisms. In Node.js, you'd need a library that allows interaction with shared memory (these can be tricky to use). This requires careful synchronization to avoid race conditions and data corruption.
- • Advantages: Potentially very fast data transfer.
- • Disadvantages: Significantly more difficult to implement correctly. Requires careful synchronization to avoid concurrency problems. Not recommended unless you have a strong understanding of shared memory programming.
- 3. Using a Message Queue (e.g., RabbitMQ, Redis):
- • Mechanism: A message queue acts as an intermediary. Your .NET and Electron apps would send messages to the queue and receive messages from it asynchronously. This is particularly useful if there's a need for loose coupling and asynchronous communication.
- • Implementation: You would need to install and configure a message queue system (like RabbitMQ or Redis). Then, you would use client libraries for both .NET and Node.js to interact with the queue.
- • Advantages: Loose coupling, asynchronous communication, more scalable.
- • Disadvantages: Adding an external dependency (the message queue). Requires more setup and configuration.
Passing Parameters make regardless of the IPC method, you'll need a structured format for parameter passing. JSON is almost universally compatible:
- • .NET (sending): Serialize parameters as JSON using JsonConvert.SerializeObject().
- • Electron (receiving): Parse JSON received from .NET using JSON.parse().
- • Electron (sending): Serialize parameters as JSON using JSON.stringify().
- • .NET (receiving): Parse JSON using JsonConvert.DeserializeObject < YourDataType > () (replace YourDataType with a corresponding VB.NET class).
For most scenarios, named pipes are the easiest and most practical approach to combine your .NET WinForms and Electron applications. It provides a good balance of simplicity, performance, and maintainability. If you expect high throughput or extremely low latency communication, then look at shared memory. But make sure to handle the complexity correctly. Message queues are best suited for situations requiring high scalability and loose coupling between the processes.
Robust error handling is crucial for inter-process communication. You'll need to handle potential connection issues, serialization/deserialization errors, and other problems that can arise when two applications communicate.
3. Jint - .NET JavaScript Engine templates.
Jint (https://github.com/sebastienros/jint) of course, don't allow to run Node.js modules within a Windows desktop application using Jint. Jint is a .NET library that provides an embedded JavaScript interpreter. It emulates a browser-like environment, not a Node.js environment. Node.js modules rely heavily on Node's built-in modules (like fs, path, etc.) and the Node.js event loop, which Jint does not provide. However in some case this can be useful:
1: Imports Jint ' Install the Jint NuGet package
2: Imports System
3: Imports System.Windows.Forms
4:
5: Partial Public Class Form1
6: Inherits Form
7:
8: Public Sub New()
9: InitializeComponent()
10: End Sub
11:
12: Private Sub Button1_Click(sender As Object, e As EventArgs) Handles Button1.Click
13: ' Create a Jint engine
14: Dim engine = New Engine()
15:
16: ' Define a JavaScript function (can be more complex)
17: Dim jsFunction As String = "
18: function add(a, b) {
19: return a + b;
20: }
21: "
22:
23: ' Execute the JavaScript code
24: engine.Execute(jsFunction)
25:
26: ' Call the JavaScript function from VB.NET
27: Dim result = engine.Invoke("add", 10, 5) ' Invoke the JS function
28:
29: ' Display the result in a TextBox (or other UI element)
30: TextBox1.Text = result.ToString()
31:
32:
33: ' Accessing .NET objects from Javascript (more advanced):
34: ' You can expose .NET objects to your JS code.
35: ' For Example:
36: engine.SetValue("myDotNetObject", New MyDotNetClass())
37: engine.Execute("
38: var result2 = myDotNetObject.DotNetMethod(5);
39: console.log('Result from DotNetMethod: ' + result2);
40: ") ' This will execute the DotNetMethod
41:
42: End Sub
43:
44: ' Example of a .NET class exposed to javascript
45: Public Class MyDotNetClass
46: Public Function DotNetMethod(x As Integer) As Integer
47: Return x * 2
48: End Function
49: End Class
50: End Class
4. Node.js Service (and IPC using HTTP):
1: Imports System
2: Imports System.Net.Http
3: Imports System.Net.Http.Headers
4: Imports System.Text
5: Imports System.Text.Json ' For JSON serialization
6: Imports System.Threading.Tasks
7: Imports System.Windows.Forms
8:
9:
10: Partial Public Class Form1
11: Inherits Form
12:
13: Private Async Sub Button1_Click(sender As Object, e As EventArgs) Handles Button1.Click
14: ' Data to send to Node.js
15: Dim data = New With {.numbers = New Integer() {1, 2, 3, 4, 5}}
16: Dim json = JsonSerializer.Serialize(data)
17:
18: Using client = New HttpClient()
19: client.BaseAddress = New Uri("http://localhost:3000/") ' Your Node.js Server address
20: client.DefaultRequestHeaders.Accept.Clear()
21: client.DefaultRequestHeaders.Accept.Add(New MediaTypeWithQualityHeaderValue("application/json"))
22:
23: Dim content = New StringContent(json, Encoding.UTF8, "application/json")
24: Dim response = Await client.PostAsync("processdata", content)
25:
26: If response.IsSuccessStatusCode Then
27: Dim responseJson = Await response.Content.ReadAsStringAsync()
28: Dim responseObject = JsonSerializer.Deserialize(Of Object)(responseJson) ' Use Object or a specific type if known
29: TextBox1.Text = responseObject.result.sum.ToString() ' Accessing sum assuming the structure is known
30: Else
31: TextBox1.Text = $"Error: {response.StatusCode}"
32: End If
33: End Using
34: End Sub
35: End Class
And this is Node-based service:
1: const express = require('express');
2: const app = express();
3: app.use(express.json()); // For parsing JSON bodies
4:
5: app.post('/processdata', (req, res) => {
6: try {
7: const data = req.body;
8: // Process the data using your JavaScript functions
9: const result = processData(data); //Your processing logic here. This will be specific to your application.
10: res.json({ result });
11: } catch (error) {
12: console.error("Error processing data:", error);
13: res.status(500).json({ error: error.message });
14: }
15: });
16:
17:
18: function processData(data) {
19: // Example processing:
20: let sum = 0;
21: for (let i = 0; i < data.numbers.length; i++){
22: sum += data.numbers[i];
23: }
24: return {sum: sum}
25: }
26:
27: const port = 3000; // Or any available port
28: app.listen(port, () => console.log(`Server listening on port ${port}`));
NetCoreBackend context:
- (2024) Combine Javascript and VB.NET to one application. #NetCoreBackend #WinDesktop
- (2024) Example of my Node Express server code to read image and save it to Amazon S3 #NodeBackend #Cloud
- (2024) My ticket lottery engine based on SQL CTE procedure #Sql #NodeBackend #JavascriptProjects
- (2024) Common Node.js backend logic (plus some of my YUP filters) #NodeBackend
- (2024) My workable Node mailing templates (with Brevo and Resend Post servers, on JS and TS with Dotenv, with native packages and directly with fetching API, with sync/async/IIFE templates). #Mailing #NodeBackend
- (2024) Test project with Nest.js and Sequelize ORM #JavascriptProjects #NodeBackend
- (2024) Migration ASP.NET to NET Core 8 (including EF6 to EF Core) #AspNetMvc #NetCoreBackend
- (2024) C# EF code first. How to create DB context and deploy DB in Net Core 8 #NetCoreBackend #EfCodeFirst
- (2023) [Angular mosaic 3] Standard Interceptor and JWT injector, Login page, Auth service, and Base form with FormGroup. #NetCoreBackend #Angular
- (2023) [Angular mosaic 5] Net Core SignalR Client (ReactiveX/rxjs, Configure Angular injector, Abstract class, Inject SignalR service, Configure SignalR hub - forbid Negotiation, setUp Transport, URL, LogLevel, Anon or AU socket connection), Configure server Hub (MapHub endpoint, WithOrigins on CORS-policy, LogLevel, JWT AU) #NetCoreBackend #Angular
- (2023) List of my small freelance project 2023 #Browser #WinDesktop #NetCoreBackend #AspClassic #Css #PaymentGateway #EfCodeFirst #Voip
- (2023) NET Core 6 project template with Serilog, example of services and write to PostgreSQL by ExecuteSqlRaw #NetCoreBackend
- (2023) Notes about most interesting project in my life. #Crypto #Blazor #Angular #Kvm #Linux #Ssl #NetCoreBackend #Servers #VmWare #Docker
- (2022) BackendAPI (Net Core 5) project template with Custom Attribute, Service and Controller Example, MySQL database with masked password in config, SwaggerUI, EF Core and extension to EF Core #NetCoreBackend #EfCodeFirst
- (2022) CheckDBNull, RawSqlQuery, ExecRDR, RawSqlQueryAsync, ExecNonQueryAsync (with transaction)- amazing extension for working with data. #Sql #NetCoreBackend
- (2022) Working with MySQL Blob #NetCoreBackend #Sql
- (2022) Asp Net Core DI container - services, service instances, service parameters list and service parameters value, service resolver for services with the same type. #NetCoreBackend
- (2022) Asp Net Core Routing, Conventions, DefultUI. Site roots - ContentRootPath/StaticFilesRoot/WebRootPath/WebRootFileProvider/RazorPagesOptions.RootDirectory. #AspNetMvc #NetCoreBackend
- (2022) My TDD Technique for Backend API development with Xunit (Custom Attribute, WebClient GET/POST, JWT auth, Fact, Theory, InlineData, ClassData iterator function, Inject Log, Txt parsers for console output) #Testing #NetCoreBackend
- (2022) Asynchronous MultiThreaded SSH engine for Web (Net Core 6, Linux) - Part 1,2 (Database and SSH client) StreamReader/ReadToEndAsync, Extension/Inherits/Overloads, BeginExecute/EndExecute/IAsyncResult/IProgress(Of T)/Async/Await/CancellationToken/Task.Run/Thread.Yield. #Task #NetCoreBackend #Linux #Yield
- (2022) Asynchronous MultiThreaded SSH engine for Web (Net Core 6, Linux) - Part 3,4 (CryptoAPI/CryptoService and Database Access). Protect password in DB and config, Task.Run for Async DB access, Expand POCO classes, Service lifetime list, Linq and Iterator/Yield functions. #Task #NetCoreBackend #Linux #Yield
- (2022) Asynchronous MultiThreaded SSH engine for Web (Net Core 6, Linux) - Part 5,6 (Crypt/Encrypt JWT Auth header, Middleware, User scoped service, custom AU attribute, custom HttpClient and Typed SignalRHub with saving ConnectionID to Singleton service). #Task #NetCoreBackend #Linux
- (2022) Asynchronous MultiThreaded SSH engine for Web (Net Core 6, Linux) - Part 7,8 (QuartzService/Jobs and Singleton CacheService). ConcurrentDictionary, Interlocked.Increment, SyncLock, Closure variable for MultiThreading, Cron Configuration. #Task #NetCoreBackend #Linux #Kvm
- (2022) Asynchronous MultiThreaded SSH engine for Web (Net Core 6, Linux) - Part 9,10 (BackendAPI for NotificationController and my technique to testing this engine with xUnit). #Task #NetCoreBackend #Linux #Kvm
- (2022) Split code and view to different projects with ASP.NET Core 6 #AspNetMvc #NetCoreBackend #Authentication
- (2022) Processing absent files by custom middleware #NetCoreBackend
- (2022) MySQL Kvm hosting management Db with tracking changing by triggers. #NetCoreBackend #Ssl #Kvm
- (2022) Asp.Net Core 6 new initialization - Startup.vb #NetCoreBackend
- (2022) Simplest way to create tracing and authorization layers in Backend API. Special attribute to arrange API for authorization layers. #NetCoreBackend
- (2022) Key future of RPAT backend project #Excel #Cloud #Browser #EfCodeFirst #TimeSchedule #NetCoreBackend #Angular
- (2022) Scaffolding Angular Client From Swagger.json #Angular #NetCoreBackend
- (2022) Notes about singleton service. #NetCoreBackend
- (2022) Json Path more examples. #NetCoreBackend #Xml
- (2022) Processing Backend result in Angular frontend as typed data #Angular #NetCoreBackend
- (2021) How to build application server based on push notification from Firebase #Google #Android #NetCoreBackend
- (2021) Deploy .NET Core on Ubuntu, demonize Kestrel, run Nginx as reversy proxy, separate Backend and Frontend #Linux #NetCoreBackend
- (2021) Linux console app (.NET Core, EF DB first, CamelCase file and dir rename, calc MD5, RegExpression, change and check link). #NetCoreBackend #Linux #EfCodeFirst
- (2021) KWMC - description the features of a typical ASP.NET MVC project. #Mailing #Google #Css #AspNetMvc #NetCoreBackend
- (2021) Linux shell parser (Renci.SshNet.SshClient, MySqlConnector.NET, RSA encription, Console password, XDocument/XPath, Tuple, Regex, Buffer.BlockCopy, Stream, Base64String, UTF8.GetBytes, ToString("X2")) #Linux #NetCoreBackend
- (2020) NET Core 3.1 test project for Oauth2 with new IndentityModel 4.2 #Authentication #NetCoreBackend
- (2020) How Linux Net Core 3.1 daemon (in VB.NET) can read/write data from/to ancient MS SQL 2005 #NetCoreBackend #Sql #WebServiceClient #WebApiServer
- (2020) How to debug SignalR application in Net Core 3.1 #DevEnvironment #NetCoreBackend
- (2020) Transform application from ancient MVC Web API technology to Net Core 3.1 (step-by-step guide) #NetCoreBackend #AspNetMvc
- (2020) Deploy ASP.NET Core application from scratch (install and tune SSH/VNC/FTP/NGINX/Daemon) #NetCoreBackend #Linux #Servers
- (2020) My last public project templates in Microsoft marketplace #Blazor #Bot #Testing #NetCoreBackend #WebServiceClient
- (2019) ASP.NET Core Backend with MailKit/IMAP (Async/Await, Monitor, ConcurrentQueue, CancellationTokenSource) #Task #NetCoreBackend #Mailing
- (2018) Proxy-handler for graphhopper.com. #WebServiceClient #NetCoreBackend #JavascriptProjects
- (2018) My learning ASP.NET Core (Common features, Clean Architecture, CQRS pattern, RabbitMQ, Microservices, Core+Angular+VB.NET) #NetCoreBackend
- (2010) GoogleTranslate - англо-русский онлайн переводчик #Linux #NetCoreBackend #Microsoft
WinDesktop context:
- (2024) Combine Javascript and VB.NET to one application. #NetCoreBackend #WinDesktop
- (2024) How to create full list of Google API #Google #WinDesktop
- (2024) Simple windows desktop app. #WinDesktop
- (2024) Simplest keylogger based on Win32 API SetWindowsHookExA #WindowsApi #WinDesktop
- (2024) Demo application with DataGridView and random data #WinDesktop
- (2023) Desktop application event handler (ThreadException, UnhandledException, NetworkAvailabilityChanged) and High DPI manifest. #WinDesktop
- (2023) List of my small freelance project 2023 #Browser #WinDesktop #NetCoreBackend #AspClassic #Css #PaymentGateway #EfCodeFirst #Voip
- (2023) Asynchronous MultiThreaded performance test of Confluent Kafka (Net Core 6) #Cloud #Task #WinDesktop
- (2023) Rebuilding my ancient API factory on modern way (Cryptor, Windows services, IIS management, Windows Task Scheduler, TcpListener, WCF, gRPC, Port Sharing service) #TimeSchedule #WindowsApi #WinDesktop
- (2023) Two my PostgreSQL project in this year #Cloud #Sql #WinDesktop
- (2022) VB6 to ASPNET converter #Vb6 #WinDesktop
- (2022) Access to SqlLite converter. VB example of Generic, Func, Extension. Using MS.ACE.OLEDB provider and SQLite. #WinDesktop #Access #Sql
- (2022) EventLogger - example of Task.Factory.FromAsync, Task.Run, ReaderWriterLock, Interlocked.Increment, Stream.BeginWrite, SyncLock, Timeout, AsyncCallback, IAsyncResult, String.Format, Timespan. #WinDesktop #Task
- (2022) Windows timers investigation (API Timer, Stopwatch Timer, Multimedia Timer). #WinDesktop #TimeSchedule #Task
- (2021) Zebra barcode 2D scanner as USB HID Keyboard working fine #Device #WinDesktop
- (2021) Airport Security System (based on Zebra scanner) #WinDesktop #Device
- (2021) Windows service example #WinDesktop
- (2021) SQLServerTxtDump - my typical windows console utility in VB.NET. Example of System.Data.SqlClient, GetSchemaTable, DataTable/DataRow, Action/Func, Linq, Tuple, IEnumerable, Iterator, Yield, Byte.ToString("x2"), DBNull, Array of Object. #WinDesktop #Yield
- (2019) Tesseract OCR Project template. #WinDesktop
- (2019) Handling SVG (Scalable Vector Graphics) files. #Device #WinDesktop
- (2019) Multi Languages Spell Checker for webmaster. Part 1. Install and tune NHunspell engine. #WinDesktop
- (2019) Multi Languages Spell Checker for webmaster. Part 7. Other project components. #WinDesktop
- (2019) Multi Languages Spell Checker for webmaster. Part 5. Multilang asynchronous spell checker with NHunspell. #WinDesktop #Task
- (2019) Multi Languages Spell Checker for webmaster. Part 4. TheArtOfDev.HtmlRenderer. #WinDesktop #Browser
- (2019) 3.1. My typical VB.NET desktop application. How to overcome VS Designer lost link to controls. #WinDesktop
- (2019) Multi Languages Spell Checker for webmaster. Part 2. Main MDI-form. #WinDesktop
- (2019) 3.3. My typical VB.NET desktop application. Fill TreeView and ListView without binding. #WinDesktop
- (2019) Multi Languages Spell Checker for webmaster. #WinDesktop
- (2019) 4.1. My typical VB.NET desktop application. Parse message body by MailKit/MimeKit and save attachment. #WinDesktop
- (2019) 1.2. My typical VB.NET desktop application. MDI environment. (Login screen, Form menu, Accessibility, Windows management). #WinDesktop
- (2019) Multi Languages Spell Checker for webmaster. Part 6. TextEditor with highlighting, searching, line numbering and FTP-uploading. #WinDesktop
- (2019) Samantha - my typical VB.NET desktop application. #WinDesktop #WinDesktop #Mailing #Access
- (2019) Multi Languages Spell Checker for webmaster. Part 3. Directory observer on NET Reactive extension. #Task #WinDesktop
- (2019) 3.5. My typical VB.NET desktop application. HtmlPanel - browser for desktop application. #WinDesktop #Browser
- (2019) 3.4. My typical VB.NET desktop application. Navigate in DataGrid and TreeView. #WinDesktop
- (2019) 1.1. My typical VB.NET desktop application. Starter. (Catch up non GUI exceptions and load embedded font to managed memory). #WindowsApi #WinDesktop
- (2019) Generic VB.NET function for loading ExcelFile to DataBase (EF6 CodeFirst) with Reflection and avoiding Linq-to-Entity Linq.Expressions (use Linq-to-Object IEnumerable) #EfCodeFirst #Excel #WinDesktop
- (2019) 3.2. My typical VB.NET desktop application. Make form resizable. #WinDesktop
- (2019) 2.1. My typical VB.NET desktop application. (EF DB First and ADO NET Typed Dataset, Tree ways to Binding GridView and ComboBox, Set Timeout, Asynchronous fill table by data) #WinDesktop #Task
- (2018) Template to save SQLiteDB (EF6) to Temporary Location (automatically and user selected). #WinDesktop #Installer
- (2018) Trap unhandled exception in windows application. #WindowsApi #WinDesktop
- (2018) CefSharp.Winforms.ChromiumWebBrowser minimal example on VB.NET (with cookies collector and script executor). #Browser #WinDesktop
- (2018) DownloadHandler for CefSharp.Winforms.ChromiumWebBrowser. #Browser #WinDesktop
- (2018) SetProxy for CefSharp.Winforms.ChromiumWebBrowser. #Browser #WinDesktop
- (2018) How to create Slideshow and VideoConverter by FFmpeg MediaToolkit. #Task #WinDesktop #Video
- (2018) Processing Office Open XML (xlsx) files by EPPlus. #Excel #WinDesktop #Xml
- (2018) How to SendText to specific field in another application by Win32 API. #WindowsApi #WinDesktop
- (2018) Small .Net Wrapper Around Firefox #Browser #WinDesktop
- (2017) Simplest MarkDowm parser for publishing ASP.NET documentation and samples (snapshot 2017). #WinDesktop
- (2017) ImageMagick.NET file converter. #Pdf #WinDesktop
- (2017) Шаблон кода для роботи з DataGridView. #WinDesktop
- (2017) Шаблон кода для роботи з MS Excel. #Excel #WinDesktop
- (2017) How to parse JSON by Newtonsoft.Json (on example of FireFox Tab Session Manager and decrypted JwtSecurityToken) #WinDesktop #Browser
- (2016) TreeView FileSelector by ReactiveNET and TheArtOfDev.HtmlRenderer (SpellChecker project). #Browser #WinDesktop #Yield #Task
- (2016) Mutlithreading InfoMessagBox by ConcurrentQueue, Interlocked and Timer. #Task #Delegate #WinDesktop
- (2016) Проги під заказ і проги по натхненню. #WinDesktop
- (2016) Опис двадцяти моїх дрібних фрілансерских проєктів 2016-го року. #PaymentGateway #WebApiServer #Video #Voip #AspNetClassic #Servers #WinDesktop
- (2016) Building TreeView by Reactive Extensions NET (Recursive observe directory, Iterator function with Yield, Windows native thread). #Task #WinDesktop #Yield
- (2016) How to refactoring old Access application. #Access #WinDesktop
- (2015) Конструктор PDF-схем.. #Pdf #WinDesktop
- (2015) Типовое windows-приложение с базой данных. #Job #WinDesktop
- (2015) FinancialBroker - MDI application with EF code first database. #EfCodeFirst #WinDesktop
- (2015) ListView and TabControl Custom Drawing. #WinDesktop #Mailing
- (2015) DataGridView-редактор параметрів програми. #WinDesktop
- (2014) Простий сервіс реестра для зберігання вводу користувача десктопних прог. #WinDesktop
- (2014) Protect program by password. #WinDesktop
- (2014) My web scrapper with asyncronous web request and visual proxy availability detection. #WinDesktop #Vpn #WebServiceClient #Task
- (2014) Collection and processing information about your system. #WinDesktop #Wsh #Sql
- (2013) DragAndDrop DataGridView Rows #WinDesktop
- (2013) Оновлення StatusLabel з потоку BackGroundWorker - приклад застосування Action, Delegate, Invoke, AddressOf, Extension, Expression. #WinDesktop #Delegate #Task
- (2013) Set HandCursor for all ToolStripButton by Linq, Extension, Delegates, Lambda Expression and Anonymous Action. #Delegate #WinDesktop
- (2012) WinDump - снимок состояния системы с помощью WMI. #Wsh #WinDesktop
- (2012) How to intercept exception & console output & debug trace and show it in textbox. #Excel #WinDesktop #Pdf
- (2012) SoundCreator - пакетная озвучка английского текста (вторая версия) #WinDesktop #Google #WebServiceClient #English
- (2012) RichTextBox Editor for various purposes with row numbering and searching. #WinDesktop
- (2012) ExcelPrice Converter - скачка и преобразование документов Excel в нужный формат за три клика мышкой. #Excel #WinDesktop
- (2011) CSV_Spliter - разрезка больших CSV-файлов. #WinDesktop
- (2009) ExchangeLogAnalyzer - OpenSource парсер журнала MS Exchange Server. #Mailing #WinDesktop
- (2009) Xping - утилита контроля качества связи. #WinDesktop
- (2009) WebDownloader_UltraLite - ваш личный поисковик по рунету с особыми возможностями поиска. #WinDesktop
- (2009) ScriptManager - Менеджер MS SQL сервера - дополнение MS SMS для работы с большими скриптами. #WinDesktop #Sql
- (2009) ComDetector - утилита поиска COM-оборудования #Device #WinDesktop #NetCommon
- (2009) RemoveOldFile - утилита удаления устаревших файлов. #WinDesktop
- (2007) Скачка, раззиповка, перекодирование, парсинг и укладка в базу ЖД-расписания из АСУ Экспресс-3. #Ssl #WinDesktop
- (2006) SiteChecker - утилита оптимизация сайта с открытым исходным текстом. #WinDesktop
- (2006) WinDump - утилита фиксации состояния системы с открытым исходным текстом. #WinDesktop
- (2005) Outline - утилита загрузки прайс-листов с группировкой в SQL с открытым исходным текстом. #Excel #WinDesktop
- (2005) DumpExe - утилитка дампирования структуры NET-сборок с открытым исходным текстом. #Binary #WinDesktop
- (2005) Альтернативная оболочка к SQL2005 #Sql #WinDesktop
- (2005) Редактируемое дерево с меняющимся контекстным меню #WinDesktop
- (2005) Работа с классами и коллекциями #WinDesktop #Task
- (2005) SQLBath - утилита работы с SQL-пакетами с открытым исходным текстом. #WinDesktop #Ssl
- (2005) Типизируемый тип столбца в GridView. #WinDesktop
- (2005) ProjectExplorer #WinDesktop
- (2005) Выгрузка данных из 1С #WinDesktop
- (2005) Мои проекты для ООО Сириус-СЦ #Vb6 #Job #AspNetClassic #WinDesktop #NetCommon
- (2004) OutLookUnload - утилитка разгрузки почты OutLook в SQL с открытым исходным текстом. #WinDesktop
- (2004) Связь NET-COM #WinDesktop #ComObject #NetCommon
- (2004) Drag-and-Drop #WinDesktop #NetCommon
- (2004) DirMSI - утилитка просмотра содержимого MSI-файла с открытым исходным текстом. #Installer #WinDesktop
- (2004) Save password to Registry. #WinDesktop
- (2003) Remoting-программирование #WinDesktop #WebServiceClient #NetCommon
- (2002) FRX-парсер - моя утилитка для выкусывания рисунков из шестерочного FRX-файла. #WinDesktop #Vb6 #Binary
- (2002) Regedit - моя утилитка для создания ярлычков к ключам реестра. #WinDesktop

|