Encrypt sensitive data in DB by Rijndael symmetric algorithm.
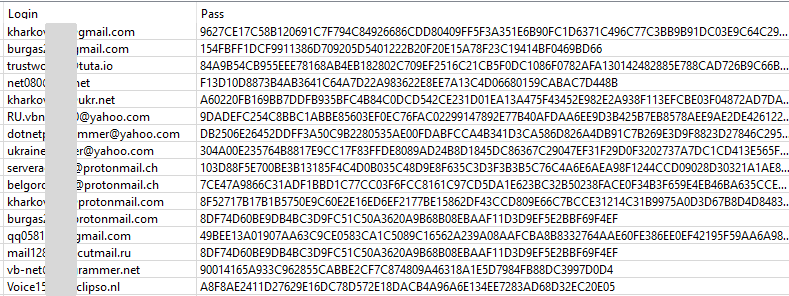
This is simple and fast algorithm, and below you can see my workable implementation of this crypto algorithm.
1: Class Symmertric
2:
3: Dim RMCrypto As New Security.Cryptography.RijndaelManaged()
4: Public Property Key As Byte()
5: Public Property IV As Byte()
6:
7: Public Sub CreateKey()
8: RMCrypto.GenerateIV()
9: IV = RMCrypto.IV
10: RMCrypto.GenerateKey()
11: Key = RMCrypto.Key
12: End Sub
13:
14: 'выдает на выход сгененрированный ключ, IV и выходным параметром - криптованную строку
15: Public Function EnCryptString(ByVal InputStr As String) As String
16: Try
17: Dim InputArr() As Byte = (New System.Text.UnicodeEncoding).GetBytes(InputStr)
18: Dim MemStream As New IO.MemoryStream(InputArr)
19: Dim CryptStream1 As New Security.Cryptography.CryptoStream(MemStream, RMCrypto.CreateEncryptor(Key, IV), Security.Cryptography.CryptoStreamMode.Read)
20: Dim CryptoArrLength As Integer = (InputStr.Length + 32) * 2 'размер буфера (примерный)
21: Dim OutArr(CryptoArrLength) As Byte, I As Integer, OneByte As Byte
22: While True
23: Try
24: OneByte = CryptStream1.ReadByte
25: Catch ex As System.OverflowException
26: Exit While
27: End Try
28: OutArr(I) = OneByte
29: I += 1
30: End While
31: MemStream.Close()
32: CryptStream1.Close()
33: ReDim Preserve OutArr(I - 1)
34: Dim OutBuilder As New System.Text.StringBuilder
35: For j As Integer = 0 To OutArr.Length - 1
36: OutBuilder.AppendFormat("{0:X2}", OutArr(j))
37: Next
38: Return OutBuilder.ToString
39: Catch ex As Exception
40: MsgBox(ex.Message)
41: End Try
42: End Function
43:
44: 'кушает на входе ключ, IV, криптованную строку и выдает расшифрованную строку
45: Public Function DeCryptString(ByVal InputStr As String) As String
46: Try
47: Dim CryptoArrLength As Integer = (InputStr.Length + 32) * 2 'размер буфера (примерный)
48: Dim InputArr(CryptoArrLength) As Byte, I As Integer, OneByte As Byte
49: For I = 0 To InputStr.Length / 2 - 1
50: OneByte = Byte.Parse(InputStr.Substring(I * 2, 2), Globalization.NumberStyles.AllowHexSpecifier)
51: InputArr(I) = OneByte
52: Next
53: ReDim Preserve InputArr(I - 1)
54: Dim MemStream As New IO.MemoryStream(InputArr)
55: Dim CryptStream1 As New Security.Cryptography.CryptoStream(MemStream, RMCrypto.CreateDecryptor(Key, IV), Security.Cryptography.CryptoStreamMode.Read)
56: Dim OutArr(CryptoArrLength) As Byte, j As Integer
57: If OutArr.Length = 0 Then
58: Return "" 'не расшифровало ничего вообще
59: Else
60: While True
61: Try
62: OneByte = CryptStream1.ReadByte
63: Catch ex As System.OverflowException
64: Exit While
65: End Try
66: OutArr(j) = OneByte
67: j += 1
68: End While
69: MemStream.Close()
70: CryptStream1.Close()
71: ReDim Preserve OutArr(j - 1)
72: Return (New System.Text.UnicodeEncoding).GetString(OutArr)
73: End If
74: Catch ex As Exception
75: If ex.GetType.FullName = "System.Security.Cryptography.CryptographicException" Then
76: Return "@?@" 'не расшифровало, сменились Key,IV - OutArr из нулей
77: Else
78: MsgBox(ex.Message)
79: End If
80:
81: End Try
82: End Function
83: End Class
Usually code to save data to DB is look as below - to Decrypt data.
1: Public Class EditForm
...
5: Dim Crypter As New Symmertric
...
12: With CurEditRow
13: LoginTextBox.Text = .Login
14: PassTextBox.Text = IIf(Crypter.DeCryptString(.Pass) = "@?@", "", Crypter.DeCryptString(.Pass))
And to Encrypt data.
32: Dim R As Site = Db.Rows.Where(Function(X) X.I = CurEditRow.I).FirstOrDefault
33: R.Login = LoginTextBox.Text
34: R.Pass = Crypter.EnCryptString(PassTextBox.Text)
...
49: Db.DbSaveChange()
Comments (
)

Link to this page:
//www.vb-net.com/Rijndael-symmetric/index.htm
|