How to Read URLs from Firefox tabs by UI Automation.
I want to create simple .NET wrapper around Firefox for testing purposes. Firstly I create code in bottom this page How to SendText to specific field in another application by Win32 API. But it work extremely slow, in my environment it worked at least 2 minutes. Therefore I try to learn structure of Firefox deeper by my own Reflection Object dumper.
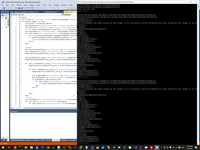
And finally I found this version of code to search URL. This is full version of code with my testing efforts but without code of Reflection Object dumper
1: Imports System.Windows.Automation
2:
3: Module Module1
4:
5: Sub Main()
6: Dim Firefox As Process() = Process.GetProcessesByName("firefox")
7: If Firefox.Length > 0 Then
8: Dim Parent = Firefox(0).Parent
9: Dim RootElement As AutomationElement = AutomationElement.FromHandle(Parent.MainWindowHandle)
10: Dim ControlTypeCondition As Condition = New PropertyCondition(AutomationElement.ControlTypeProperty, ControlType.Custom)
11: Dim CustomControl_Level_1 As AutomationElement = GetAllNextLevelCustomControl(RootElement, ControlTypeCondition)
12: DumpProperty(CustomControl_Level_1, "Level_1")
13:
14: For Each CustomControl_Level_2 As AutomationElement In CustomControl_Level_1.FindAll(TreeScope.Children, ControlTypeCondition)
15: DumpProperty(CustomControl_Level_2, "Level_2")
16:
17: For Each CustomControl_Level_3 As AutomationElement In CustomControl_Level_2.FindAll(TreeScope.Children, ControlTypeCondition)
18: DumpProperty(CustomControl_Level_3, "Level_3")
19:
20: GetDocumentFromControl(CustomControl_Level_3)
21:
22: Next
23: Next
24: End If
25: End Sub
26:
27: Private Function GetDocumentFromControl(CustomControl As AutomationElement)
28: Dim condDocument As Condition = New PropertyCondition(AutomationElement.ControlTypeProperty, ControlType.Document)
29: Dim Document1 As AutomationElement = CustomControl.FindFirst(TreeScope.Children, condDocument)
30: If Document1 IsNot Nothing Then
31: For Each pattern As AutomationPattern In Document1.GetSupportedPatterns()
32: If TypeOf Document1.GetCurrentPattern(pattern) Is ValuePattern Then Console.WriteLine((TryCast(Document1.GetCurrentPattern(pattern), ValuePattern)).Current.Value.ToString() + Environment.NewLine)
33: Next
34: End If
35: End Function
36:
37: Private Function GetAllNextLevelCustomControl(ByVal rootElement As AutomationElement, ByVal condCustomControl As Condition) As AutomationElement
38: Return rootElement.FindAll(TreeScope.Children, condCustomControl).Cast(Of AutomationElement)().ToList().Where(Function(x) x.Current.BoundingRectangle <> System.Windows.Rect.Empty).FirstOrDefault()
39: End Function
40:
41: Sub DumpProperty(Control As AutomationElement, Title As String)
42: Dim D As New ObjectDumper
43: Console.WriteLine("===========" & Title & "===========")
44: D.Dump(Control, 5)
45: End Sub
46:
47: End Module
And this is something another my universal extension function to search parent process, because this is firefox structure of tasks.
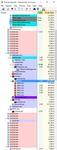
1: Imports System.Runtime.CompilerServices
2:
3: Module ProcessExtensions
4: Private Function FindIndexedProcessName(ByVal pid As Integer) As String
5: Dim processName = Process.GetProcessById(pid).ProcessName
6: Dim processesByName = Process.GetProcessesByName(processName)
7: Dim processIndexdName As String = Nothing
8:
9: For index = 0 To processesByName.Length - 1
10: If index = 0 Then
11: processIndexdName = processName
12: Else
13: processIndexdName = processName & "#" & index
14: End If
15:
16: Dim processId = New PerformanceCounter("Process", "ID Process", processIndexdName)
17:
18: If CInt(processId.NextValue()) = pid Then
19: Return processIndexdName
20: End If
21: Next
22:
23: Return processIndexdName
24: End Function
25:
26: Private Function FindPidFromIndexedProcessName(ByVal indexedProcessName As String) As Process
27: Dim parentId = New PerformanceCounter("Process", "Creating Process ID", indexedProcessName)
28: Return Process.GetProcessById(CInt(parentId.NextValue()))
29: End Function
30:
31: <Extension()>
32: Function Parent(ByVal process As Process) As Process
33: Return FindPidFromIndexedProcessName(FindIndexedProcessName(process.Id))
34: End Function
35: End Module
And this is finally result of this test (if controls class dump is commented).
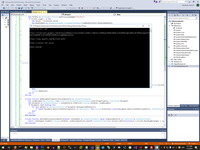
Comments (
)

Link to this page:
//www.vb-net.com/ReadFirefoxUrls/index.htm
|