How to intercept exception & console output & debug trace and show it in textbox.
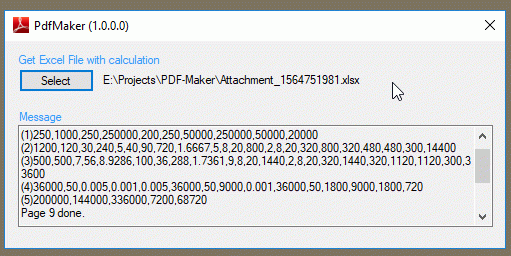
This is a common pattern to catch up all message of exception, console output and debug trace in windows desktop application and show all of it in textbox.
...
5: Public Class StartForm
...
15: Private Sub StartForm_Load(sender As Object, e As EventArgs) Handles Me.Load
16: Text &= " (" & My.Application.Info.Version.ToString & ")"
17: Try
...
26: Dim TextBoxListener = New TextBoxTraceListener(MsgBox1)
27: Trace.Listeners.Add(TextBoxListener)
28: Dim ConsoleWriter = New ConsoleWriter()
29: AddHandler ConsoleWriter.WriteEvent, AddressOf consoleWriter_WriteEvent
30: AddHandler ConsoleWriter.WriteLineEvent, AddressOf consoleWriter_WriteLineEvent
31: Console.SetOut(ConsoleWriter)
...
35: If Not My.Computer.FileSystem.FileExists(IO.Path.Combine(TempDirectory, Inkscape)) Then
36: MsgBox1.Text = "This program use Inkscape library, please install Inkscape firstly."
37: MsgBox1.WriteLog("https://inkscape.org/")
38: Else
39: MsgBox1.Text = "Start " & Now
40: MsgBox1.Select(0, 0)
41: End If
42: Catch ex As Exception
43: MsgBox1.WriteLog(ex.Message)
44: End Try
45: End Sub
46:
47: Private Sub consoleWriter_WriteLineEvent(sender As Object, e As ConsoleWriterEventArgs)
48: MsgBox1.WriteLog(e.Value)
49: End Sub
50:
51: Private Sub consoleWriter_WriteEvent(sender As Object, e As ConsoleWriterEventArgs)
52: MsgBox1.WriteLog(e.Value)
53: End Sub
54:
This is module to intercept write to trace log, usually as debug.print
1: Public Class TextBoxTraceListener
2: Inherits TraceListener
3:
4: Private _target As TextBox
5: Private _invokeWrite As StringSendDelegate
6:
7: 'Delegate to invoke on the text box
8: Private Delegate Sub StringSendDelegate(ByVal message As String)
9:
10: 'Create a new text box trace listener that writes to the
11: Public Sub New(ByVal target As TextBox)
12: _target = target
13: _invokeWrite = New StringSendDelegate(AddressOf SendString)
14: End Sub
15:
16: 'Writes a string to the textbox
17: Public Overrides Sub Write(ByVal message As String)
18: _target.Invoke(_invokeWrite, New Object() {message})
19: End Sub
20:
21: 'Writes a string followed by a new line character to the textbox
22: Public Overrides Sub WriteLine(ByVal message As String)
23: _target.Invoke(_invokeWrite, New Object() {message & Environment.NewLine})
24: End Sub
25:
26: 'Method passed for invokation by the text box
27: Private Sub SendString(ByVal message As String)
28: _target.Text += message
29: End Sub
30: End Class
This is module to intercept writing to console in windows desktop application.
1: Imports System.IO
2: Imports System.Text
3:
4: Public Class ConsoleWriterEventArgs
5: Inherits EventArgs
6:
7: Public Property Value As String
8:
9: Public Sub New(ByVal value As String)
10: value = value
11: End Sub
12: End Class
13:
14: Public Class ConsoleWriter
15: Inherits TextWriter
16:
17: Public Event WriteEvent As EventHandler(Of ConsoleWriterEventArgs)
18: Public Event WriteLineEvent As EventHandler(Of ConsoleWriterEventArgs)
19:
20: Public Overrides ReadOnly Property Encoding As Encoding
21: Get
22: Return Encoding.UTF8
23: End Get
24: End Property
25:
26: Public Overrides Sub Write(ByVal value As String)
27: RaiseEvent WriteEvent(Me, New ConsoleWriterEventArgs(value))
28: MyBase.Write(value)
29: End Sub
30:
31: Public Overrides Sub WriteLine(ByVal value As String)
32: RaiseEvent WriteLineEvent(Me, New ConsoleWriterEventArgs(value))
33: MyBase.WriteLine(value)
34: End Sub
35:
36:
37: End Class
And this is main extension function to add text and scroll is to end of log.
1: Imports System.Runtime.CompilerServices
2:
3: Module Log
4:
5: <Extension>
6: Public Sub WriteLog(T As TextBox, TXT As String)
7: T.Text &= vbCrLf & TXT
8: T.Refresh()
9: T.SelectionStart = T.TextLength
10: T.ScrollToCaret()
11: End Sub
12:
13: End Module
Comments (
)

Link to this page: //www.vb-net.com/TextBoxLog/index.htm
|