(<< Back <<) Typescript Matt Pocock lectures (<< Back <<)
https://www.totaltypescript.com/, https://github.com/total
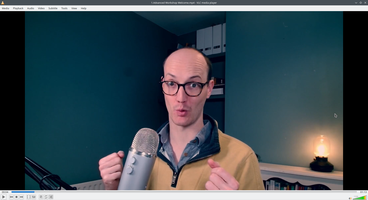
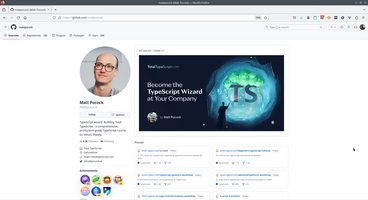
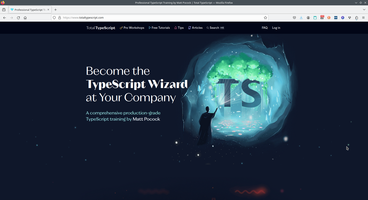
1. Advanced TypeScript Patterns
- 1.Advanced Workshop Welcome
- 2.What is a Branded Type?
- 3.Form Validation with Branded Types
- 4.Assigning Branded Types to Values
- 5.Using Branded Types as Entity Ids
- 6.Add Branded Types to Functions and Models
- 7.Creating Reusable Validity Checks with Branded Types and Type Helpers
- 8.Combine Type Helpers with Branded Types
- 9.Creating Validation Boundaries with Branded Types
- 10.Using Branded Types to Validate Code Logic
- 11.Using Index Signatures with Branded Types
- 12.Indexing an Object with Branded Types
- 13.TypeScript's Global Scope
- 14.Add a Function to the Global Scope
- 15.Add Functionality to Existing Global Interfaces
- 16.Use Declaration Merging to Add Functionality to the Global Window
- 17.Add Types to Properties of Global Namespaced Interfaces
- 18.Typing process.env in the NodeJS Namespace
- 19.Colocating Types for Global Interfaces
- 20.Solving the Colocation Problem with Globals
- 21.Filtering with Type Predicates
- 22.Use a Type Predicate to Filter Types
- 23.Checking Types with Assertion Functions
- 24.Ensure Valid Types with an Assertion Function
- 25.Avoiding TypeScript's Most Confusing Error
- 26.Declare Assertion Functions Properly to Avoid Confusing Errors
- 27.Combining Type Predicates with Generics
- 28.Filtering with Type Predicates and Generics
- 29.Combining Brands and Type Predicates
- 30.Checking for Validity with Brands and Type Predicates
- 31.Combining Brands with Assertion Functions
- 32.Validate Types with Brands and Assertions
- 33.Classes as Types and Values
- 34.Using Classes in TypeScript
- 35.Dive into Classes with Type Predicates
- 36.Simplifying TypeScript with Type Predicates
- 37.Assertion Functions and Classes
- 38.Leverage Assertion Functions for Better Inference in Classes
- 39.Class Implementation Following the Builder Pattern
- 40.TRPC's Creator on the Builder Pattern
- 41.Create a Type Safe Map with the Builder Pattern
- 42.Getters and Setters in the Builder Pattern
- 43.Debugging the Builder Pattern
- 44.Default Generics in the Builder Pattern
- 45.Building Chainable Middleware with the Builder Pattern
- 46.The Power of Generics and the Builder Pattern
- 47.Subclassing in Zod
- 48.Where Do External Types Come From?
- 49.Extract Types to Extend an External Library
- 50.Retrieve Function Parameters from an External Library
- 51.Navigating Lodash's Type Definitions
- 52.Finding Proper Type Arguments and Generics with Lodash
- 53.Passing Type Arguments with Lodash
- 54.Navigating Express's Type Definitions
- 55.Add Query Params to an Express Request
- 56.Make an Express Request Function Generic
- 57.Browsing Zod's Types
- 58.Create a Runtime and Type Safe Function with Generics and Zod
- 59.Infer Runtime Arguments from a Zod Schema
- 60.Override External Library Types
- 61.Create a Declarations File to Override Types
- 62.Identity Functions as an Alternative to the `as const`
- 63.Narrowing in an Identity Function for Better Inference
- 64.Add Constraints to an Identity Function
- 65.Constraining and Narrowing an Identity Function
- 66.Specifying Where Inference Should Not Happen
- 67.Fix Inference Issues with F.NoInfer
- 68.Find the Generic Flow of an Identity Function
- 69.Avoid Duplicate Code in an Identity Function with Generics
- 70.Reverse Mapped Types
- 71.Inference Inception in an Identity Function
- 72.Merge Dynamic Objects with Global Objects
- 73.Add Objects to the Global Scope Dynamically
- 74.Narrowing with an Array
- 75.Narrowing with Arrays and Generics
- 76.Create a Type-Safe Request Handler with Zod and Express
- 77.Type-Safe Request Handlers with Zod and Express
- 78.Building a Dynamic Reducer
- 79.Dynamic Reducer with Generic Types
- 80.Custom JSX Elements
- 81.Adding Custom Elements to JSX.IntrinsicElements
2. TypeScriptGenerics
- 1.TypeScript Generics Workshop Welcome
- 2.Typing Functions with Generics
- 3.Replace the unknown Type with a Generic
- 4.Restricting Generic Argument Types
- 5.Add Constraints to a Generic
- 6.Typing Independent Parameters
- 7.Use Multiple Generics with a Function
- 8.Approaches for Typing Object Parameters
- 9.Approaches for Typing Object Parameters: Solution
- 10.Generic Functions in Excalidraw
- 11.Generics in Classes
- 12.Add Types to a Class
- 13.Generic Mapper Function
- 14.Add Object Property Constraints to a Generic
- 15.The Importance of Generics in TypeScript
- 16.Add Type Parameters to a Function
- 17.Pass Type Arguments to a Function
- 18.Defaults in Type Parameters
- 19.Specify a Default Value
- 20.Infer Types from Type Arguments
- 21.Infer from the Type Arguments of a Class
- 22.Strongly Type a Reduce Function
- 23.Pass Type Arguments to a Reduce Function
- 24.Avoid any Types with Generics
- 25.Use Generics to Type a Fetch Request
- 26.Passing Type Arguments in cal.com
- 27.Improving Code Maintainability
- 28.Generics at Different Levels
- 29.Represent Generics at the Lowest Level
- 30.Typed Object Keys
- 31.Two Approaches for Typing Object Keys
- 32.Make a Generic Wrapper for a Function
- 33.Constrain a Type Argument to a Function
- 34.Understand Literal Inference in Generics
- 35.Understand Generic Inference When Using Objects as Arguments
- 36.Inferring Literal Types from any Basic Type
- 37.Accepting Multiple Literal Types
- 38.Infer the Type of an Array Member
- 39.Constrain to the Array Member, Not the Array
- 40.Generics in a Class Names Creator
- 41.Two Approaches to Working with Class Names
- 42.Generics in React Query
- 43.Generics with Conditional Types
- 44.Ensure Runtime Level & Type Level Safety with Conditional Types
- 45.Fixing Errors in Generic Functions
- 46.Fixing the "Not Assignable" Error
- 47.Generic Function Currying
- 48.Fix Type Inference in Curried Functions
- 49.Generic Interfaces with Functions
- 50.Understanding Generics at Different Levels of Functions
- 51.Spotting Useless Generics
- 52.Refactoring Functions with Unnecessary Type Arguments
- 53.Spotting Missing Generics
- 54.Improving Type Inference with Additional Generics
- 55.How tRPC Handles Inheritable Generics
- 56.Refactoring Generics for a Cleaner API
- 57.Create a Factory Function to Apply Type Arguments to All Child Functions
- 58.The Partial Inference Problem
- 59.A Workaround for The Lack of Partial Inference
- 60.What is a Function Overload?
- 61.Understanding Function Overloads
- 62.Function Overloads vs. Conditional Types
- 63.Match Return Types with Function Overloads
- 64.Debugging Overloaded Functions
- 65.Specifying Types for an Overloaded Function
- 66.Function Overloads vs. Union Types
- 67.When to Use Overloads and Unions
- 68.Generics in Function Overloads
- 69.Typing Different Function Use Cases
- 70.Solving an Inference Mystery
- 71.The Inference Mystery Solved
- 72.Use Function Overloads to Infer Initial Data
- 73.Split Functions Into Two Different Call Signatures
- 74.The "Instantiated with Subtype" Error
- 75.Handling Default Arguments with Function Overloads
- 76.Make An Infinite Scroll Function Generic with Correct Type Inference
- 77.Introduce a Type Parameter to Ensure Type Consistency
- 78.Create a Function with a Dynamic Number of Arguments
- 79.Use a Tuple to Represent a Dynamic Number of Arguments
- 80.Create a Pick Function
- 81.Extracting Object Properties with Reduce and Generics
- 82.Create a Form Validation Library
- 83.Add Strong Typing and Proper Error Handling to a Form Validator
- 84.Improve a Fetch Function to Handle Missing Type Arguments
- 85.Modify a Generic Type Default for Improved Error Messages
- 86.Typing a Function Composition with Overloads and Generics
- 87.Using Overloads and Generics to Type Function Composition
- 88.Build an Internationalization Library
- 89.Extract Types from Strings for an Internationalization Library
3. TypeTransformations
- 1.Type Transformations Workshop Welcome: Explainer
- 2.Get the Return Type of a Function
- 3.Use a Utility Type to Extract a Functions Return Type
- 4.Typeof Keyword, and Type Level
- 5.Extract Function Parameters Into A Type
- 6.Use a Utility Type to Extract Function Parameters
- 7.Extract The Awaited Result of a Promise
- 8.Use Utility Types To Extract a Promise's Result
- 9.Create a Union Type From an Objects Keys
- 10.Create Unions from Objects Using Two Operators
- 11.Understand The Terminology Around Unions
- 12.Union Terminology Examples
- 13.Extracting Members of a Discriminated Union
- 14.Extract From A Union Using a Utility Type
- 15.Excluding Parts of a Discriminated Union
- 16.Use a Utility Type to Remove a Single Member of a Union
- 17.The Power of Union Types in TypeScript: Explainer
- 18.Extract Object Properties into Individual Types
- 19.Use Indexed Access Types to Extract Object Properties
- 20.Extract the Discriminator from a Discriminated Union
- 21.Simple Syntax Used to Access Parts of a Discriminated Union
- 22.Resolve an Objects Values as Literal Types
- 23.The Annotation Used to Infer an Object's Values as Literal Types
- 24.Create a Union From an Object's Values
- 25.Extract Specific Members From A Union with Indexed Access
- 26.Get All of an Objects Values
- 27.Use Two Operators With Indexed Access to Get All of an Object's Values
- 28.Create Unions out of Array Values
- 29.Methods Used to Create Unions out of Array Values
- 30.Only Allow Specified String Patterns
- 31.Template Literal with Strings
- 32.Extract Union Strings Matching a Pattern
- 33.Extracting String Pattern Matches with Template Literals
- 34.Create a Union of Strings with All Possible Permutations of Two Unions
- 35.Passing Unions Into Template Literals
- 36.Splitting A String into a Tuple
- 37.Using S From ts-toolbelt to Split a String Into a Tuple
- 38.Create an Object Whose Keys Are Derived From a Union
- 39.Use a Utility Type to Create An Object From A Union
- 40.Transform String Literals To Uppercase
- 41.Manipulate String Literals Using Type Helpers
- 42.Template Literals in Mattermost: Explainer
- 43.Introducing Type Helpers
- 44.Create Functions that Return Types
- 45.Creating a Maybe Type Helper
- 46.The Unconstrained Maybe Type Helper
- 47.Ensure Type Safety in a Type Helper
- 48.Use Constraints to Limit Type Parameters
- 49.Create a Reusable Type Helper
- 50.Add Support for Multiple Types in a Type Helper
- 51.Optional Type Parameters in Type Helpers
- 52.Set a Default Type Value in a Type Helper
- 53.Functions as Constraints for Type Helpers
- 54.Support Function Type Constraints with Variable Arguments
- 55.Constraining Types for Anything but null or undefined
- 56.Exclude null and undefined from the Maybe Type
- 57.Constraining Type Helpers to Non-Empty Arrays
- 58.Enforce a Minimum Array Length in a Type Helper
- 59.Explainer: Type Helpers in Redux
- 60.Add Conditional Logic to a Type Helper
- 61.Compare and Return Values with Extends and the Ternary Operator
- 62.Refine Conditional Logic in a Type Helper
- 63.Prevent Unwanted Type Scenarios from Happening
- 64.How TypeScript Added Conditional Types: Explainer
- 65.Introducing infer for Conditional Logic
- 66.Infer Elements Inside a Conditional with Infer
- 67.Extract Type Arguments to Another Type Helper
- 68.Use infer with Generics to Extract Types from Arguments
- 69.Extract Parts of a String with a Template Literal
- 70.Pattern Matching on Template Literals with Infer
- 71.Template Literal Types Were Nearly Regexes: Explainer
- 72.Extract the Result of an Async Function
- 73.Optionally Infer the Return Type of a Function
- 74.Extract the Result From Several Possible Function Shapes
- 75.Two Methods for Extracting the Result of Multiple Possible Functions
- 76.Distributivity in Conditional Types
- 77.Using Generic Context to Avoid Distributive Conditional Types
- 78.Map Over a Union to Create an Object
- 79.Use Mapped Types to Create an Object from a Union
- 80.Mapped Types with Objects
- 81.Map Over the Keys of an Object
- 82.Transforming Object Keys in Mapped Types
- 83.Remapping Object Keys in a Mapped Type
- 84.How Excalidraw uses Mapped Types to Save Lines of Code: Explainer
- 85.Conditionally Extract Properties from Object
- 86.Selective Remapping with Conditional Types and Template Literals
- 87.Map a Discriminated Union to an Object
- 88.Two Techniques for Mapping a Discriminated Union to an Object
- 89.Map an Object to a Union of Tuples
- 90.Create a Union of Tuples by Reindexing a Mapped Type
- 91.Transform an Object into a Union of Template Literals
- 92.Map an Object to a Union of Template Literals
- 93.Transform a Discriminated Union into a Union
- 94.Iteratively Map and Remap to Transform Types
- 95.Transform Path Parameters from Strings to Objects
- 96.Extract from String with Mapped Types, Template Literals, and infer.
- 97.Transform an Object into a Discriminated Union
- 98.Create a Discriminated Union through Intermediary Transformations
- 99.Transform a Discriminated Union with Unique Values to an Object
- 100.Create an Object using Mapped Types, Conditional Types, and TypeScript Keywords
- 101.Construct a Deep Partial of an Object
- 102.Use Recursion and Mapped Types to Create a Type Helper
4. Updates
- Add Constraints to an Identity Function
- Constraining and Narrowing an Identity Function
- Identity Functions as an Alternative to the `as const`
- Using const type parameters For Better Inference
Related page:
Comments (
)

Link to this page:
http://www.vb-net.com/TsMatt/Index.htm
|