Modern Javascript books
Javascript
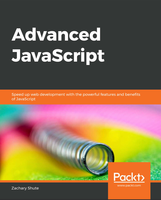
Introducing ECMAScript 6 Introduction Beginning with ECMAScript Understanding Scope Function Scope Function Scope Hoisting Block Scope Exercise 1: Implementing Block Scope Declaring Variables Exercise 2: Utilizing Variables Introducing Arrow Functions Exercise 3: Converting Arrow Functions Arrow Function Syntax Exercise 4: Upgrading Arrow Functions Learning Template Literals Exercise 5: Converting to Template Literals Exercise 6: Template Literal Conversion Enhanced Object Properties Object Properties Function Declarations Computed Properties Exercise 7: Implementing Enhanced Object Properties Destructuring Assignment Array Destructuring Exercise 8: Array Destructuring Rest and Spread Operators Object Destructuring Exercise 9: Object Destructuring Exercise 10: Nested Destructuring Exercise 11: Implementing Destructuring Classes and Modules Classes Exercise 12: Creating Your Own Class Classes – Subclasses Modules Export Keyword Import Keyword Exercise 13: Implementing Classes Transpilation Babel- Transpiling Exercise 14: Transpiling ES6 Code Iterators and Generators Iterators Generators Exercise 15: Creating a Generator Activity 1: Implementing Generators Summary
Asynchronous JavaScript Introduction Asynchronous Programming Sync Versus Async Synchronous versus Asynchronous Timing Introducing Event Loops Stack Heap and Event Queue Event Loops Things to Consider Exercise 16: Handling the Stack with an Event Loop Callbacks Building Callbacks Callback Pitfalls Fixing Callback Hell Exercise 17: Working with Callbacks Promises Promises States Resolving or Rejecting a Promise Using Promises Exercise 18: Creating and Resolving Your First Promise Handling Promises Promise Chaining Promises and Callbacks Wrapping Promises in Callbacks Exercise 19: Working with Promises Async/Await Async/Await Syntax Asnyc/Await Promise Rejection Using Async Await Activity 2: Using Async/Await Summary
DOM Manipulation and Event Handling Introduction DOM Chaining, Navigation, and Manipulation Exercise 20: Building the HTML Document from a DOM Tree Structure DOM Navigation Finding a DOM Node Traversing the DOM DOM Manipulation Updating Nodes in the DOM Updating Nodes in the DOM Exercise 21: DOM Manipulation DOM Events and Event Objects DOM Event Event Listeners Event Objects and Handling Events Event Propagation Firing Events Exercise 22: Handling Your First Event Custom Events Exercise 23: Handling and Delegating Events JQuery jQuery Basics jQuery Selector jQuery DOM Manipulation Selecting Elements Traversing the DOM Modifying the DOM Chaining jQuery Events Firing Events Custom Events Activity 3: Implementing jQuery Summary
Testing JavaScript Introduction Testing Reasons to Test Code Test-driven Development TDD Cycle Conclusion Exercise 24: Applying Test-Driven Development Types of Testing Black Box and White Box Testing Unit Tests Exercise 25: Building Unit Tests Functional Testing Integration Tests Building Tests Exercise 26: Writing Tests Test Tools and Environments Testing Frameworks Mocha Setting Up Mocha Mocha Basics Exercise 27: Setting Up a Mocha Testing Environment Mocha Async Mocha Hooks Activity 4: Utilizing Test Environments Summary
Functional Programming Introduction Introducing Functional Programming Object-Oriented Programming Functional Programming Declarative Versus Imperative Imperative Functions Declarative Functions Exercise 28: Building Imperative and Declarative Functions Pure Functions Same Output Given Same Input No Side Effects Referential Transparency Exercise 29: Building Pure Controllers Higher Order Functions Exercise 30: Editing Object Arrays Shared State Exercise 31: Fixing Shared States Immutability Immutability in JavaScript Side Effects Avoiding Side Effects Function Composition Activity 5: Recursive Immutability Summary
The JavaScript Ecosystem Introduction JavaScript Ecosystem Frontend JavaScript Command-Line Interface Mobile Development Backend Development Nodejs Setting Up Nodejs Node Package Manager Loading and Creating Modules Exercise 32: Exporting and Importing NPM Modules Basic Nodejs Server Exercise 33: Creating a Basic HTTP Server Streams and Pipes Types of Streams Writeable Stream Events: Readable Stream Events: Filesystem Operations Express Server Exercise 34: Creating a Basic Express Server Routing Advanced Routing Middleware Error Handling Exercise 35: Building a Backend with Nodejs React Installing React React Basics React Specifics JSX ReactDOM ReactComponent State Conditional Rendering List of Items HTML Forms Activity 6: Building a Frontend with React Summary Appendix
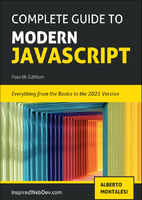
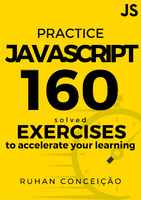
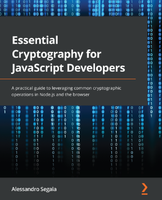
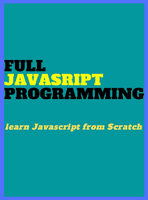
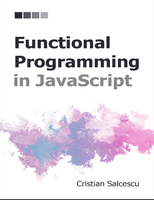
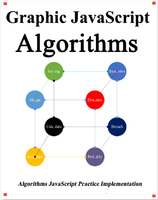
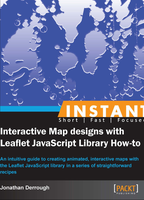
9.Interactive Data Visualization for the Web. An Introduction to Designing with D3-O’Reilly (2017).pdf
.png)
.png)
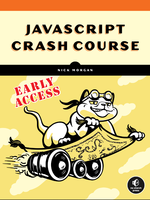
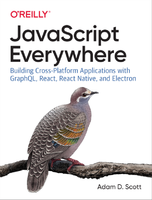
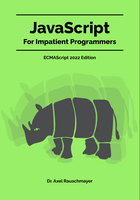
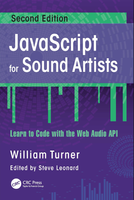
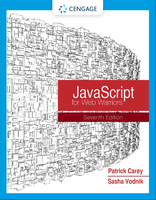
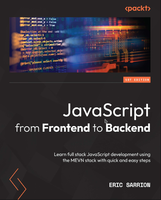
17.JavaScript From Zero to Hero - The Most Complete Guide Ever, Master Modern JavaScript Even If.epub
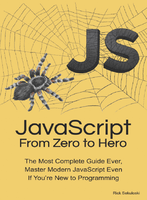
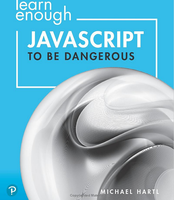
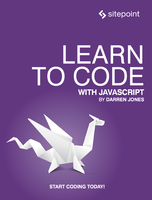
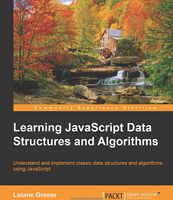
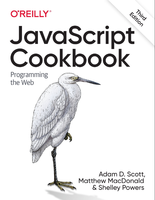
Html/Css
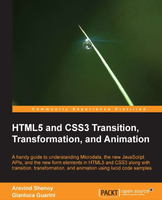
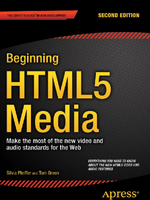
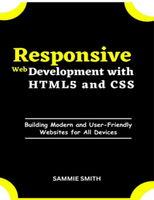
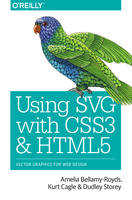
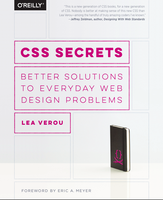
Firebase
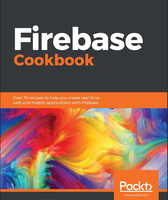
Chapter 1: Firebase - Getting Started Creating your first Firebase application Adding Firebase to an existing frontend project Integrating Firebase into the backend Integrating Firebase in Android applications Integrating Firebase in iOS applications
Chapter 2: Firebase Real-Time Database Saving and serving data in and from our Realtime Database Updating and deleting data from our Realtime Database Structuring data within our Realtime Database Implementing offline capabilities support
Chapter 3: File Management with Firebase Storage Creating file storage references Implementing file upload Implementing file serving and downloading Implementing file deletion Implementing file metadata updates Firebase file storage error handling
Chapter 4: Firebase Authentication Implementing email/password authentication Implementing anonymous authentication Implementing Facebook login Implementing Twitter login Implementing Google Sign-in Implementing user metadata retrieval Implementing the linking of multiple authentication providers
Chapter 5: Securing Application Flow with Firebase Rules Configuring the Firebase Bolt language compiler Configuring database data security rules Configuring database user data security rules Configuring storage files security rules Configuring user storage files security rules
Chapter 6: Progressive Applications Powered by Firebase Integrating Node-FCM in a NodeJS server Implementing service workers Implementing sending/receiving registration using Socket.IO Implementing sending/receiving registration using post requests Receiving web push notification messages Implementing custom notification messages
Chapter 7: Firebase Admin SDK Integrating the Firebase Admin SDK Implementing user account management by fetching users Implementing user account management by creating accounts Implementing user account management by deleting accounts Implementing notification sending
Chapter 8: Extend Firebase with Cloud Functions Getting started with Cloud Functions Implementing data manipulation Implementing data-change monitoring Welcoming users upon account creation Implementing account email confirmation Sending re-engagement emails to inactive Firebase users
Chapter 9: We’re Done, Let’s Deploy Deploying our application to Firebase Customizing the Firebase hosting environment
Chapter 10: Integrating Firebase with NativeScript Starting a NativeScript project Adding the Firebase plugin to our application Pushing/retrieving data from the Firebase Realtime Database Authenticating using anonymous or password authentication Authenticating using Google Sign-In Adding dynamic behavior using Firebase Remote Config
Chapter 11: Integrating Firebase with Android/iOS Natively Implementing the pushing and retrieving of data from Firebase Realtime Database Implementing anonymous authentication Implementing password authentication on iOS Implementing password authentication on Android Implementing Google Sign-in authentication Implementing Facebook login authentication Facebook authentication in Android Facebook authentication in iOS Generating a crash report using Firebase Crash Report Adding dynamic behavior using Firebase Remote Config in Android Adding dynamic behavior using Firebase Remote Config in iOS
Chapter 12: Hack Application's Growth Implementing sending/receiving app invite in Android/iOS Implementing topic subscription in Android/ iOS
Chapter 13: Adding Analytics and Maximizing Earnings Integrating Firebase analytics into Android/iOS applications Implementing event logging on Android/iOS Implementing user properties for data and audience filtering Integrating Firebase AdMob with Android/iOS applications Implementing Firebase AdMob banner ads on Android/iOS Implementing Firebase AdMob native ads express on Android/iOS Implementing AdMob ads targeting
Chapter 14: Firebase Cloud FireStore
RxJs
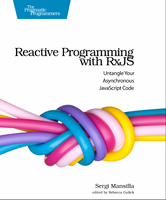
1. The Reactive Way . . . . . . . . . . . . What’s Reactive? Of Observers and Iterators The Rx Pattern and the Observable Creating Observables Wrapping Up
2. Deep in the Sequence . . . . . . . . . . Visualizing Observables Basic Sequence Operators Canceling Sequences Handling Errors Making a Real-Time Earthquake Visualizer Ideas for Improvements Operator Rundown Wrapping Up
3. Building Concurrent Programs . . . . . . . . Purity and the Observable Pipeline RxJS’s Subject Class Spaceship Reactive! Ideas for Improvements Wrapping Up
4. Building a Complete Web Application . . . . . . Building a Real-Time Earthquake Dashboard Adding a List of Earthquakes Getting Real-Time Updates from Twitter Ideas for Improvements Wrapping Up
5. Bending Time with Schedulers . . . . . . . . Using Schedulers Scheduling for Animations Testing with Schedulers Wrapping Up
6. Reactive Web Applications with Cycle.js . . . . . Cycle.js Installing Cycle.js Our Project: Wikipedia Search Model-View-Intent Creating Reusable Widgets Ideas for Improvements Wrapping Up
Node
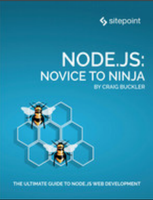
Electron
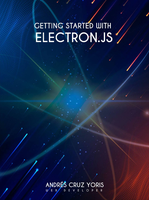
Chapter 1: Chat application, building the first application in Electron.js What is Electron? About Electron Technical requirements Create an app in Electron Sample application Create a detail page Bootstrap 5 Install Bootstrap 5 Define columns Application debugging in Chrome Web design Contacts Chat Text's box Create contacts based on an array of objects Create chats based on an array of objects
Chapter 2: Main modules in Electron Enable integration with Node Communication between processes through events Interprocess Communication: Send messages Main Process to Render Process Render process to main process Events and messages Introduction to menus in Electron Custom options Predefined roles Keyboard shortcuts: Shortcut Windows Windows identifier Additional windows Static methods for working with windows Close windows
Chapter 3: Chat application, communication with Electron.js Communication between processes Upload contacts and chats Load contacts by selection Chapter 4: Build the application for production Chapter 5: Editor application Editor app and inter-process communication menu structure Create and open files menu options
Chapter 6: Tasks app with Vue Prepare the environment Vue Router Instalation Project settings Component Creation Configure Electron.js Basic task CRUD with Vue List Create tasks Delete tasks Edit tasks Using Electron.js modules from Vue Basic Task CRUD with Vue and Electron.js List Rest CRUD operations Create task Edit task Delete task Electron.js and Electron DB Install ElectronDB Scheme to use List Create task Edit task Delete task Configure a Rest API on Node with Express Install required dependencies Configure the Rest API Configure CORS List Configure Electron DB Create task Edit task Delete task Reload the listing Configure the Rest Api with Express and MySQL Install dependencies in the project Create SQL Create connection Get all records Create task Edit task Delete task Extra: Protect Rest Api by request origin Conclusion Basic user module Create SQL Register a user Login Window to register Login window Messages and redirects Close windows Authentication token Register and consume the auth token from a file Obtain data from the user authenticated by the token Send event to main window when authenticating user Detect wrong login Sign off Include Bootstrap 5 in the project in Vue Container List Navbar Form Login and Registration window Optional: Adapt Vue app for the web Token handling Debug mode in routes Registration and login links Login and Cookies Sign off Checkin Build the app for production Install Electron builder Other services Generate application build in Vue Vue application on server Application in Vue as part of the build
Chapter 7: Environment variables and reload in the main process Environment Variables Reload/reload in the main process electron reloader Nodemon Electron reloader
Pwa
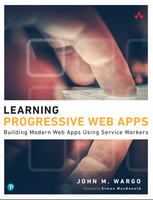
1 Introducing Progressive Web Apps First, a Little Bit of History Making a Progressive Web App PWA Market Impact PWAs and App Stores Wrap-Up
2 Web App Manifest Files Save to Home Screen Making a Web App Installable Anatomy of a Web App Manifest Setting the App Name Setting App Icons Configuring Display Mode Setting the Installed App’s Start URL Setting App Options Additional Options Controlling the Installation Experience Preparing to Code Node.JS Git Client Visual Studio Code App Installation in Action Adding a Service Worker Adding a Web Manifest File Running the App Enhancing the Installation Process Troubleshooting Manifest Generation and More Wrap-Up
3 Service Workers PWA News Introducing Service Workers Preparing to Code Prerequisites Navigating the App Source Configuring the Server API Starting the Server Registering a Service Worker Service Worker Scope The Service Worker Lifecycle Forcing Activation Claiming Additional Browser Tabs Observing a Service Worker Change Forcing a Service Worker Update Service Worker ready Promise Wrap-Up
4 Resource Caching Service Worker Cache Interface Preparing to Code Caching Application Resources Cache Management Return a Data Object on Error Adding an Offline Page Implementing Additional Caching Strategies Cache-Only Network First, Then Cache Network First, Update Cache Wrap-Up
5 Going the Rest of the Way Offline with Background Sync Introducing Background Sync Offline Data Sync Choosing a Sync Database Create Database Create Store Add Data Delete Objects Iterating through Data Using Cursors Preparing to Code Enhancing the PWA News Application Preparing the Service Worker for Background Sync Updating the Web App to Use Background Sync Finishing the Service Worker Dealing with Last Chances Wrap-Up
6 Push Notifications Introducing Push Notifications Remote Notification Architecture Preparing to Code Generating Encryption Keys Validating Notification Support Checking Notification Permission Getting Permission for Notifications Local Notifications Notification Options Subscribing to Notifications Unsubscribing from Notifications Remote Notifications Dealing with Subscription Expiration Sending Notifications to Push Services Wrap-Up
7 Passing Data between Service Workers and Web Applications Preparing to Code Send Data from a Web App to a Service Worker Send Data from a Service Worker to a Web App Two-Way Communication Using MessageChannel 171 Wrap-Up
8 Assessment, Automation, and Deployment Assessing PWA Quality Using Lighthouse Preparing to Code Using the Lighthouse Plugin Using the Lighthouse Tools in the Browser Using the Lighthouse Node Module PWABuilder Using the PWABuilder UI Creating Deployable Apps Using the PWABuilder CLI PWABuilder and Visual Studio PWAs and the Microsoft Store Wrap-Up
9 Automating Service Workers with Google Workbox Introducing Workbox Generating a Precaching Service Worker Add Precaching to an Existing Service Worker Controlling Cache Strategies 218 Wrap-Up
React/Redux
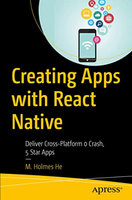
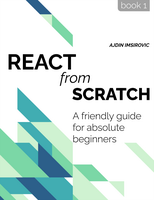
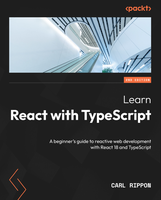
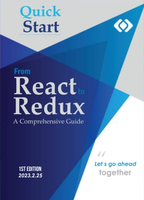
Related page:
- (2023) CloudflareWorker and Supabase
- (2022) NativeScript
- (2022) Typescript, Webpack
- (2022) JS, Css
- (2022) Node, Electron, Pwa
- (2022) Angular, RxJs, Firebase, MongoDb
- (2022) React, Redux, GraphQL, NextJs
- (2022) Angular/Typescript, JS books
- 2023 year: Magic deployment Android application with NS Preview.
- 2023 year: 5 way to create modal windows with Angular (Angular material, UIKit with toggle tag, UIKit with custom function, jQuery UI modal, Bootstrap modal).
- 2023 year: [Angular mosaic 1] My fast style, Intercept routing events (router-outlet onActivate event, @Input, extends base page).
- 2023 year: [Angular mosaic 2] Simplest shared service (@Injectable, Subject, Injectable, LocalStorage, Store), UIkit Modal Futures (uk-modal tag, Modal from routed page and from Menu, Modal Toggle, Modal Show, Bind by On, MouseOver Modal, Modal Alert, Modal Prompt, Close button).
- 2023 year: [Angular mosaic 3] Standard Interceptor and JWT injector, Login page, Auth service, and Base form with FormGroup.
- 2023 year: [Angular mosaic 4] Fill select/options (FormsModule, ngModel, ngModelChange, ngValue, http.get Json from Assets, LocalStorage), Angular connection service (Enable/Disable Heartbeat, Heartbeat URL, Heartbeat Interval/RetryInterval).
- 2023 year: [Angular mosaic 5] Net Core SignalR Client (ReactiveX/rxjs, Configure Angular injector, Abstract class, Inject SignalR service, Configure SignalR hub - forbid Negotiation, setUp Transport, URL, LogLevel, Anon or AU socket connection), Configure server Hub (MapHub endpoint, WithOrigins on CORS-policy, LogLevel, JWT AU)
- 2023 year: NativeScript and Android troubleshooting.
- 2023 year: Simple AngularFirebaseUploader as Typescript console application - npx ts-node (function main).
Comments (
)

<00>
<01>
<02>
<03>
<04>
<05>
<06>
<07>
<08>
<09>
<10>
<11>
<12>
<13>
<14>
<15>
<16>
<17>
<18>
<19>
<20>
<21>
<22>
<23>
Link to this page:
http://www.vb-net.com/ModernJsBooks/Index.htm
<SITEMAP> <MVC> <ASP> <NET> <DATA> <KIOSK> <FLEX> <SQL> <NOTES> <LINUX> <MONO> <FREEWARE> <DOCS> <ENG> <CHAT ME> <ABOUT ME> < THANKS ME> |