(<< Back <<) How to start WebDevelopment on Typescript with Lite-server and Webpack server (<< Back <<)
I like Webpack, I use it in many my projects. This is small example, on screen below you can see my webpack.config.js, packages I used in that project, source code and target code after Webpack.
1: require("dotenv").config();
2: const path = require("path");
3: const { DefinePlugin } = require("webpack");
4: const NodePolyfillPlugin = require("node-polyfill-webpack-plugin");
5:
6: module.exports = {
7: entry: path.resolve(__dirname, "index.js"),
8: target: "webworker",
9: output: {
10: filename: "worker.js",
11: path: path.resolve(__dirname, "dist"),
12: },
13: mode: "production",
14: resolve: {
15: fallback: {
16: fs: false,
17: },
18: },
19: plugins: [
20: new NodePolyfillPlugin(),
21: new DefinePlugin({
22: BOT_TOKEN: JSON.stringify(process.env.BOT_TOKEN || ""),
23: SECRET_PATH: JSON.stringify(process.env.SECRET_PATH || ""),
24: }),
25: ],
26: performance: {
27: hints: false,
28: },
29: };
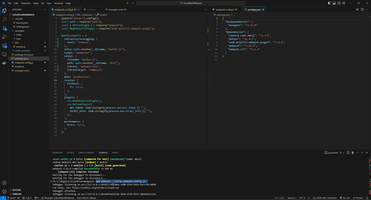
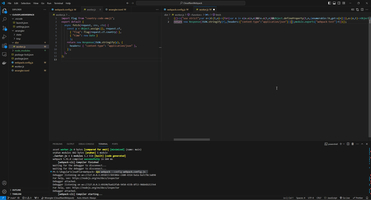
Alternative way to Webpack is just use Require.JS after compilation TS to JS, see example on My RxJs learning conspectus
Now I want to learning deeper, working with Typescript and using TS with Webpack. Typescript is not Angular, Angular is SPA-page framework and allow to change view on one page (except NativeScript Angular where each one component present one separate page with separate URL) , Typescript allow create JS for each site page. This is lecture about tuning Typescript developer environment.
1. Vite
First simplest way of Typescript development is using Vite. Compare source and target:
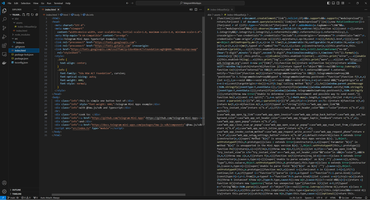
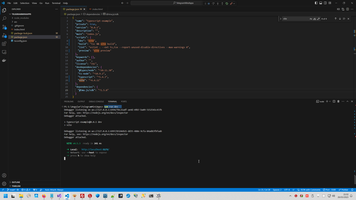
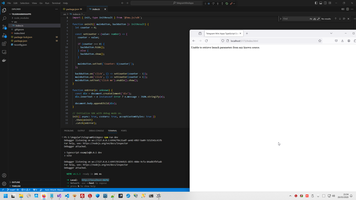
And this is usual package.json
"scripts": { "dev": "vite", "build": "tsc && vite build", "lint": "eslint . --ext ts,tsx --report-unused-disable-directives --max-warnings 0", "preview": "vite preview" },
This means npm run dev perform vite and npm run build perform tsc && vite build
2. Lite-server
1. A Simple Project Setup.mp4 2. Working With The DOM.mp4 3. The Lib Compiler Option.mp4 4. TypeScript's Non-Null Assertion Operator.mp4 5. Type Assertions.mp4 6. Type Assertions With the DOM.mp4 7. Working With Events.mp4 8. Building The Todo List.mp4 9. Adding in an Interface.mp4 10. Connecting to LocalStorage.mp4 11. Todo List Finishing Touches.mp4
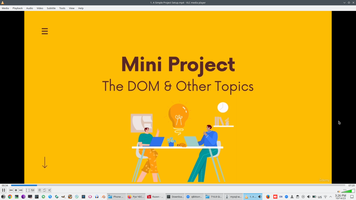
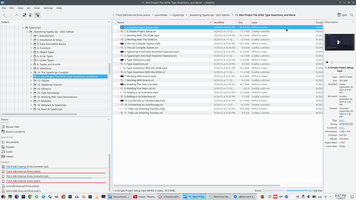
Initialize typescript compiler "tsc --init", create "tsconfig.json"
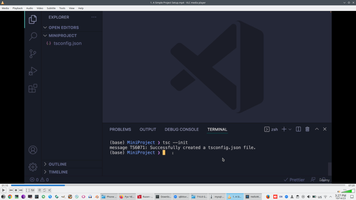
Create project
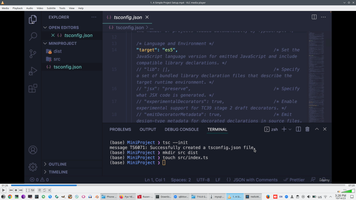
Tune tsconfig.json - set "outDir","skipLibCheck" and "include" folder with source files.
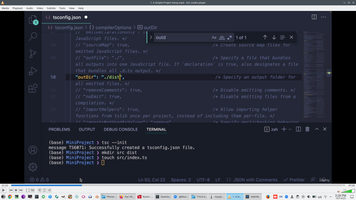
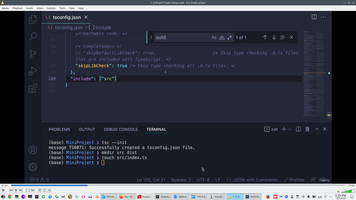
Try to start - receive .JS file
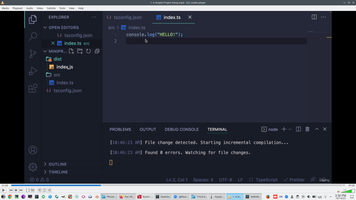
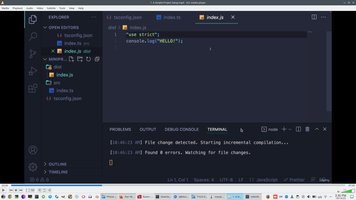
Try ro run .JS file on Node.js
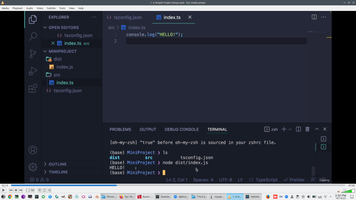
Create *.HTML file with *.JS file and try to start view it on Browser.
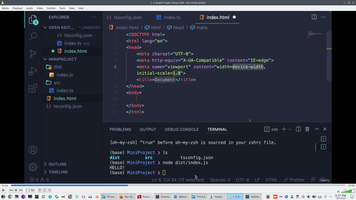
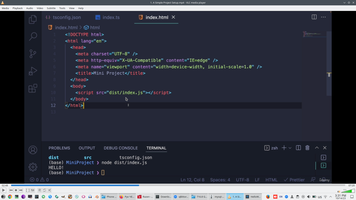
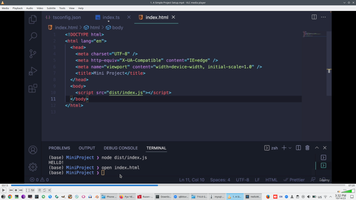
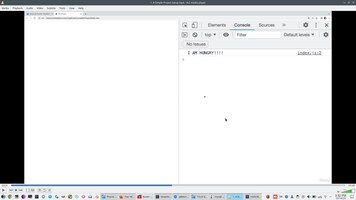
Initialize Node.JS config - "package.json"
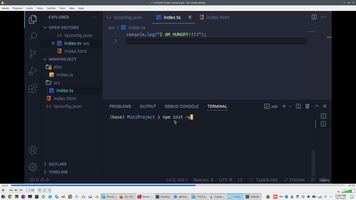
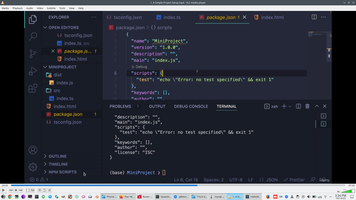
Install lite-server "npm i lite-server"
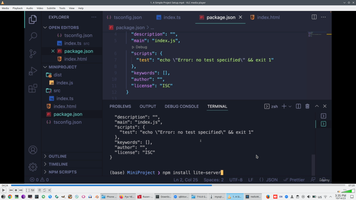
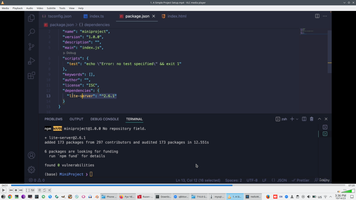
Tune script section on "package.json" - "npm start" now will start lite-server on current folder.
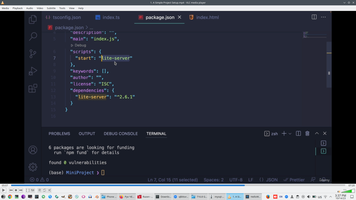
Start lite-server, check page on browser and GET/POST request on console.
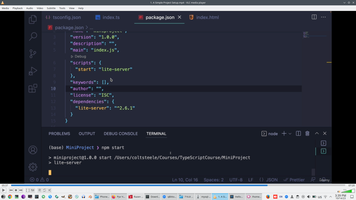
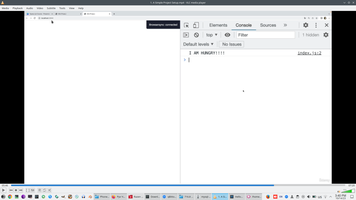
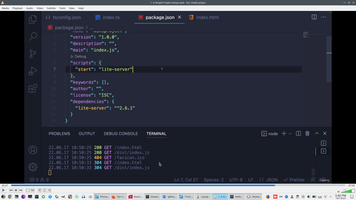
Check hot reloading when Typescript code changing.
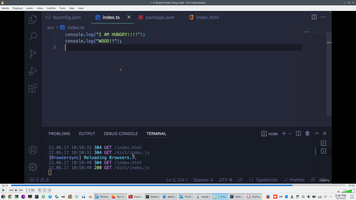
Add button to page
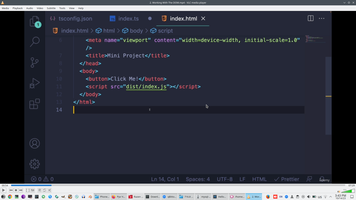
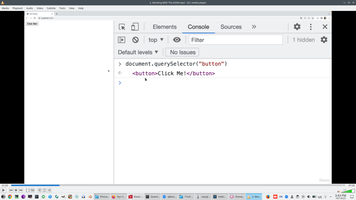
Type of HTML document id Document, Typescript automatically understand this type.
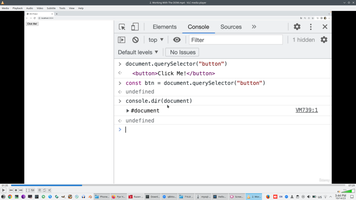
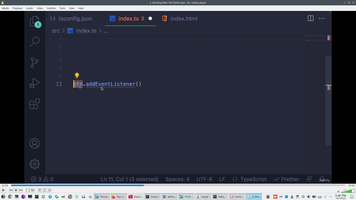
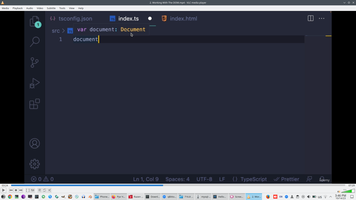
We can click to Document definition and look to Document Interface
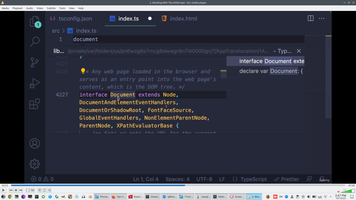
Because compiler used needed models from linked library, look list of linked library on https://www.typescriptlang.org/tsconfig#lib
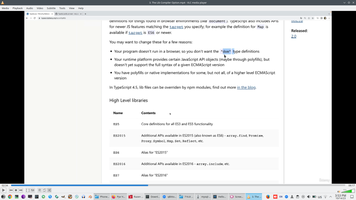
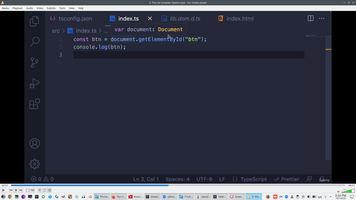
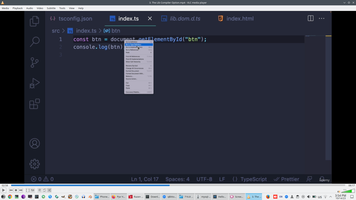
"lib.dom.d.ts" contains definition of current version of DOM model.
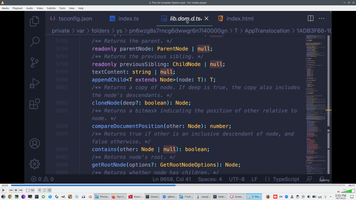
But without Library we can not use repated property, for example "replaceAll" method present only on DOM version start from "2021" version
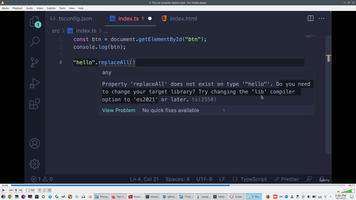
Therefor we need bind project with ES5 Javascript to needed DOM, in this case version "2021".
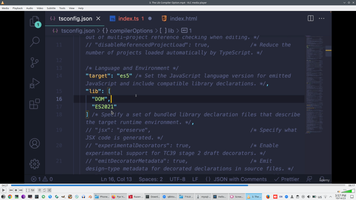
And replaceAll method is appear.
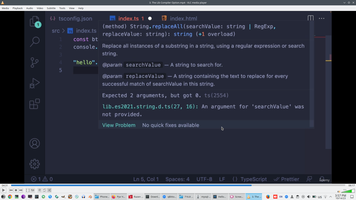
This is list of all available DOM on https://github.com/microsoft/TypeScript/tree/main/src/lib related to JS version
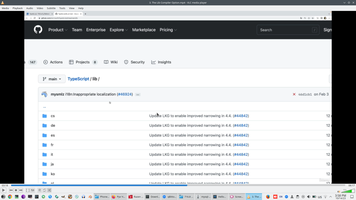
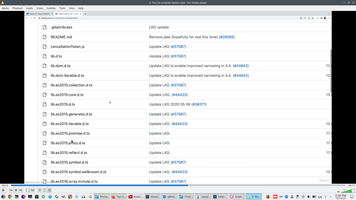
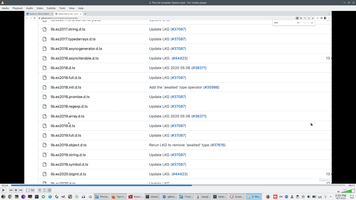
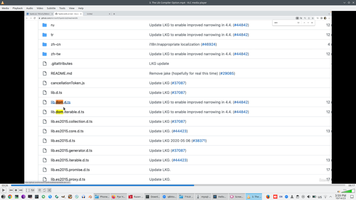
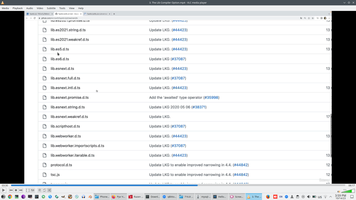
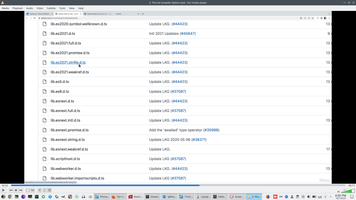
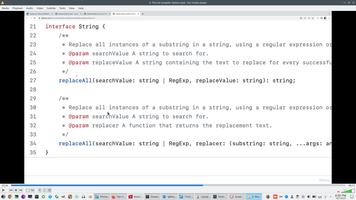
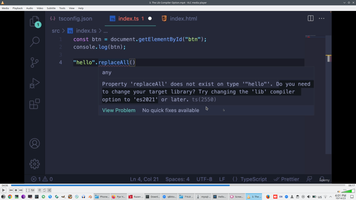
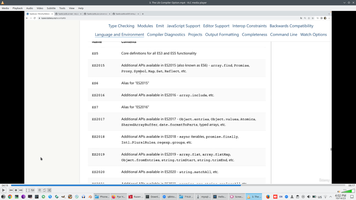
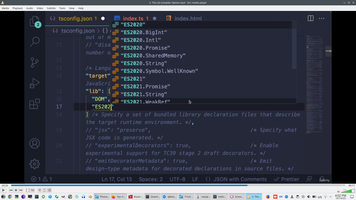
We also can change "target" Javascript version on Typescript compiler, in this case we select "2021" version.
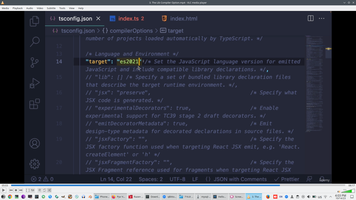
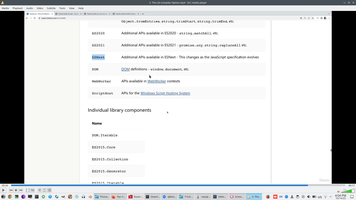
But we can not use Button on Typescript, it has only common HTML property
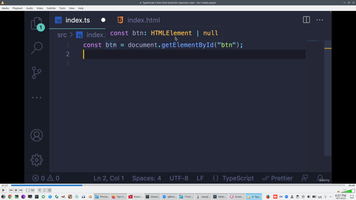
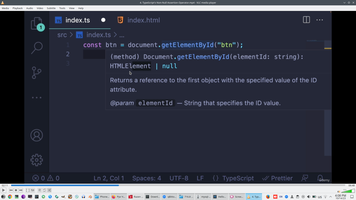
How we can understand it Type? Look on prototope on Browser.
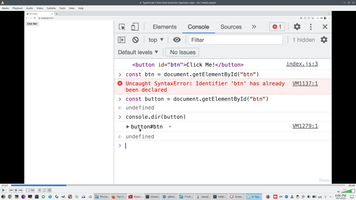
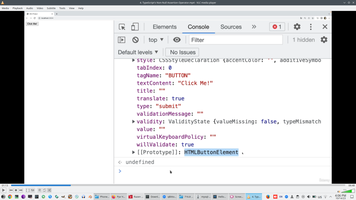
HtmlButton can be undefined, this is obstacle to Typescript programming.
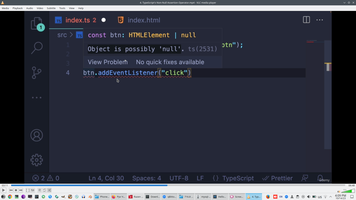
One wey to solve it to use JS approach - use "?".
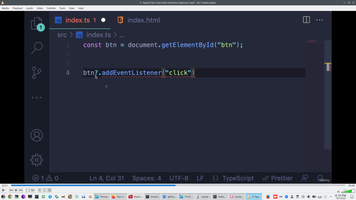
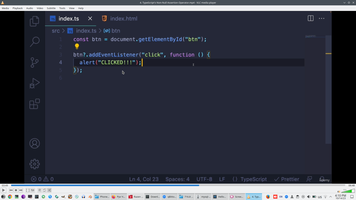
But if we sure that button name present we can use "!" - Typescript approach.
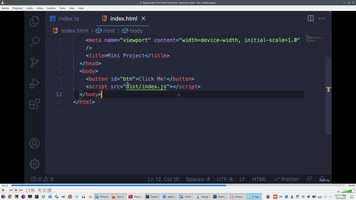
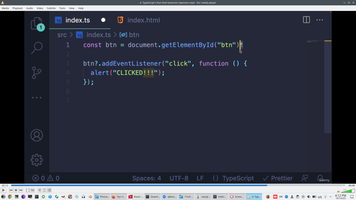
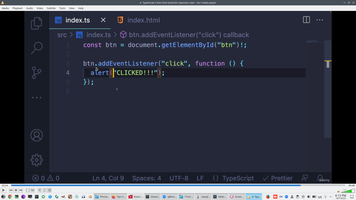
Button and string still has no specific value, to access all specific DOM value we need to use "Type assertion". Look to prototype on Browser, Check DOM and add type assertion "as".
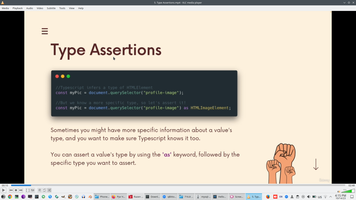
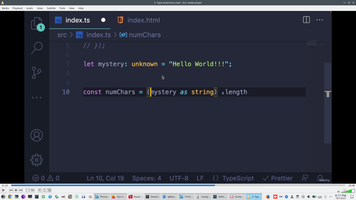
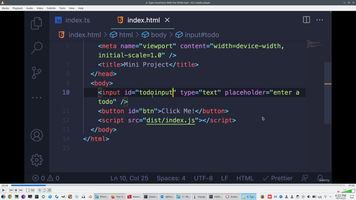
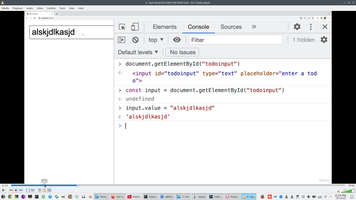
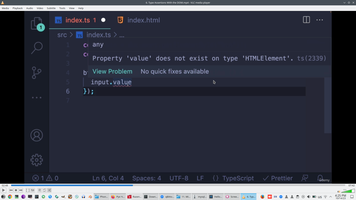
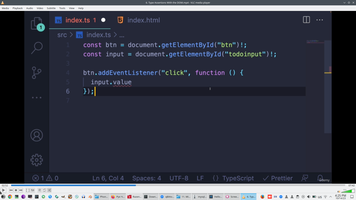
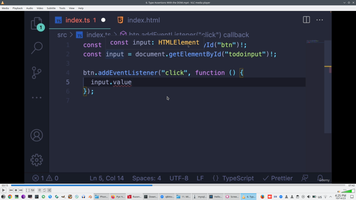
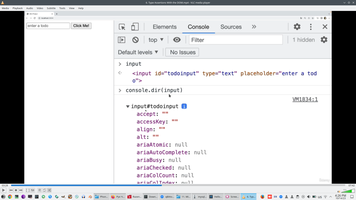
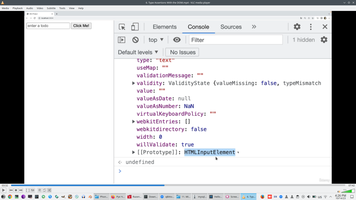
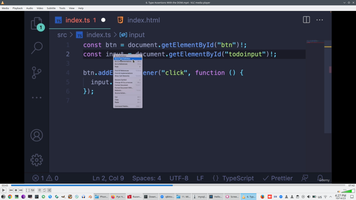
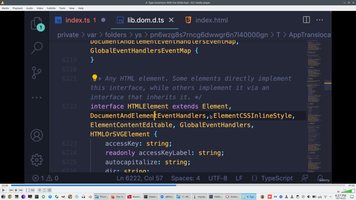
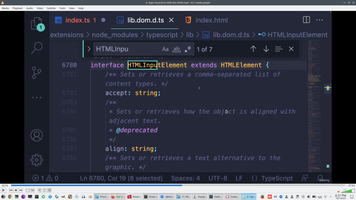
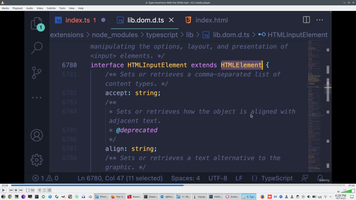
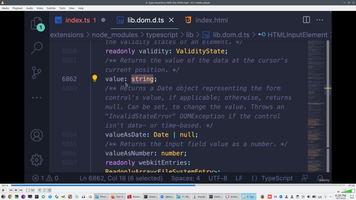
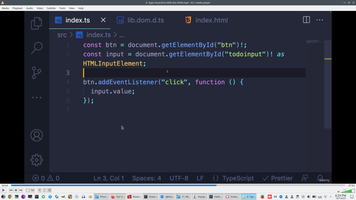
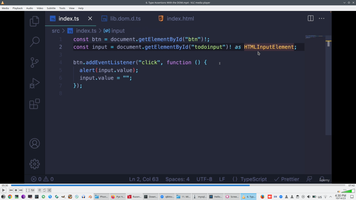
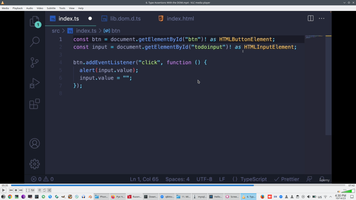
At common Typescript allow alternative way to setup Type assertion, but this way will not working on React.
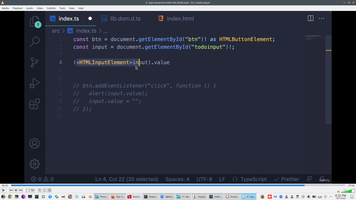
Now we can add <FORM> element and start working with Events.
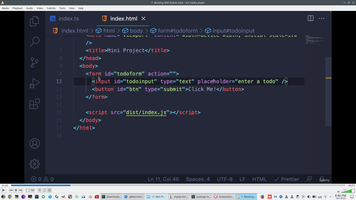
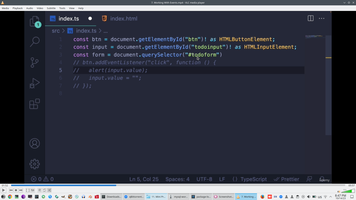
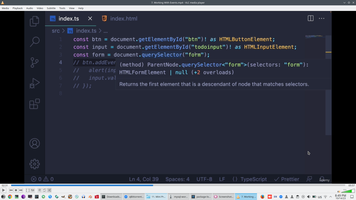
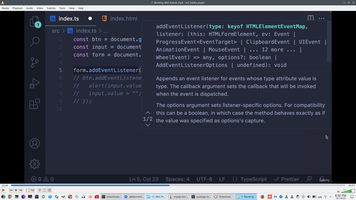
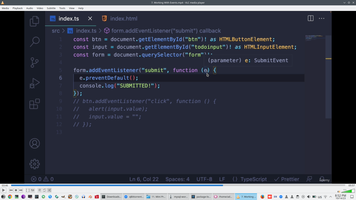
But if we can not fixed "e.preventdefault" event not captures on <FORM> and going to high level HTML element.
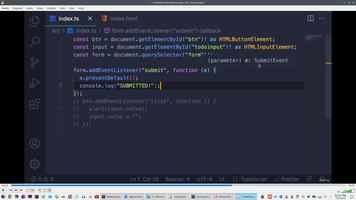
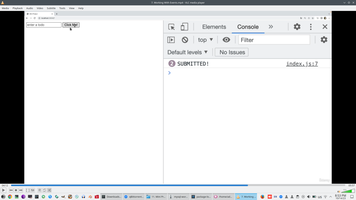
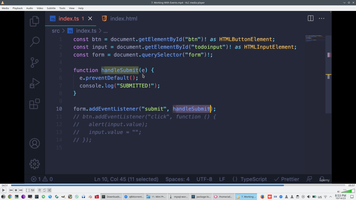
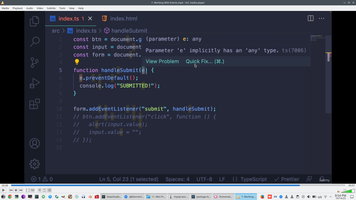
We need to specify Event type.
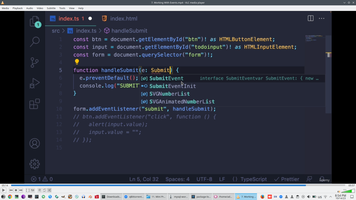
Since than all development working free and without troubles. For example this demo primer build dynamic list.
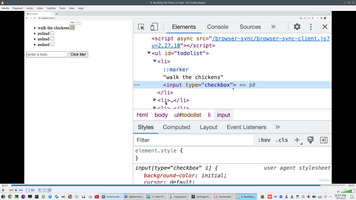
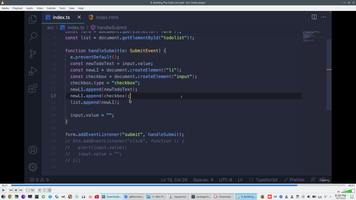
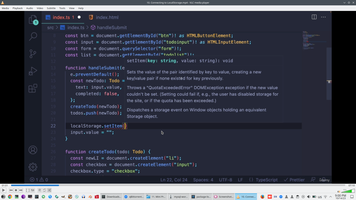
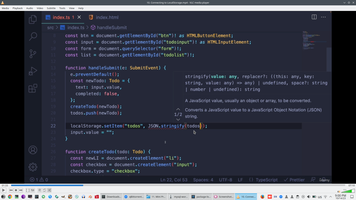
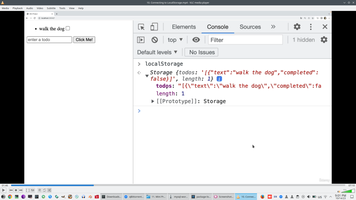
3. Typescript with WebPack
1. What's The Point Of Webpack.mp4 2. Setting Up a Project.mp4 3. Installing Webpack Dependencies.mp4 4. Basic Webpack Config.mp4 5. Adding Source Maps.mp4 6. Webpack Dev Server.mp4 7. Production Configuration.mp4
At common WebPack replace a lot of server requests to only one.
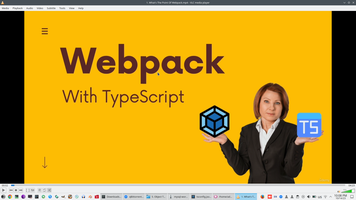
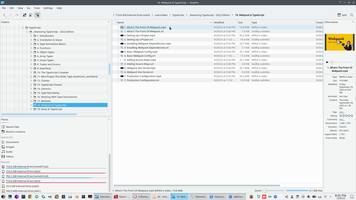
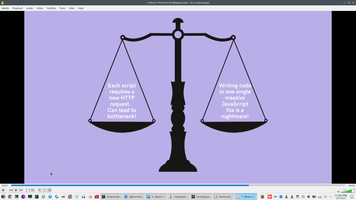
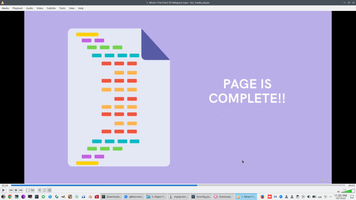
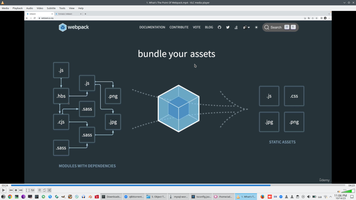
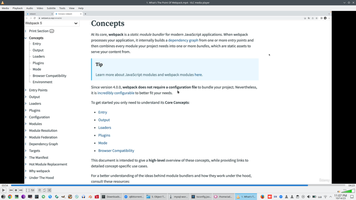
To start development we need to initialize Typescript compliler "tsc --init" and Node.js "npm init -y".
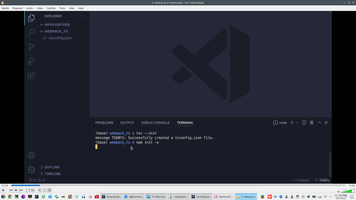
Firstly need to check project working with Lite server "npm i lite-server"
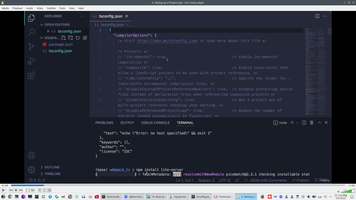
Setup "outDir", "target" JS version, and "include" - source project dir.
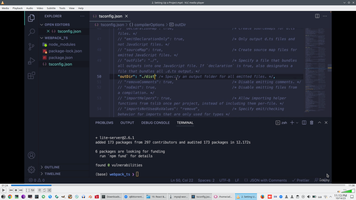
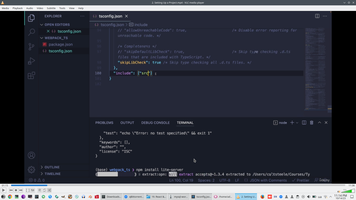
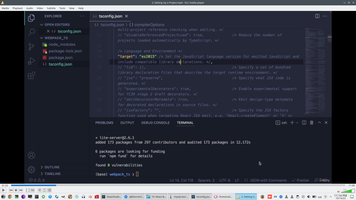
Create project with Index.ts, Utils.ts, Class.ts and import Utils and Class to Index.ts
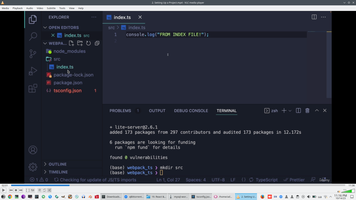
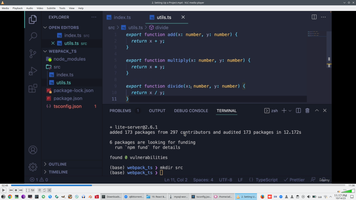
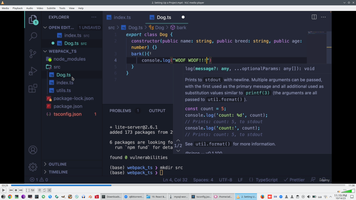
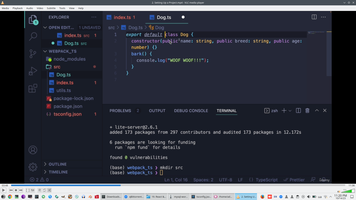
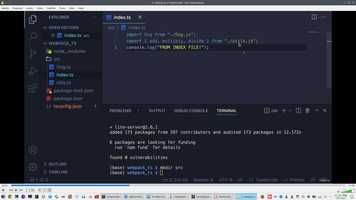
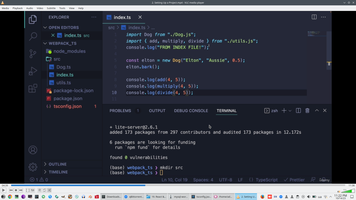
Create nested class extend first class. Use "super" (MyBase on VB.NET)
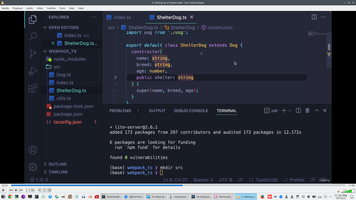
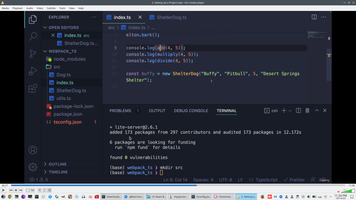
We still working with /dist/index.js and lite-server.
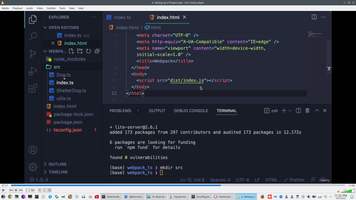
Look to result of Typescript compilation.
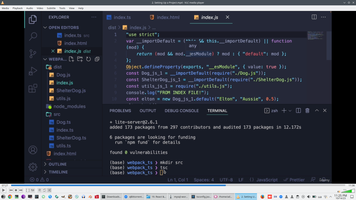
We can compile TypeScript to "Module" and in this case we need to import compilation result as <script type="module"
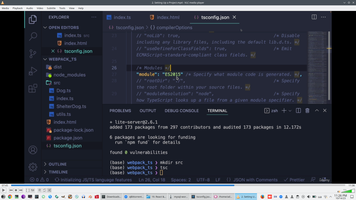
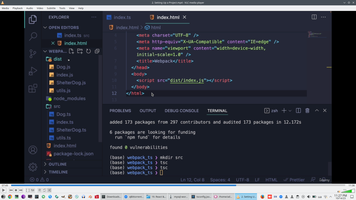
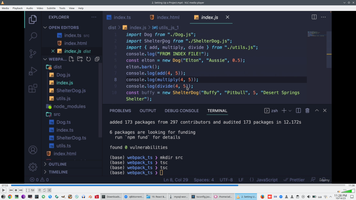
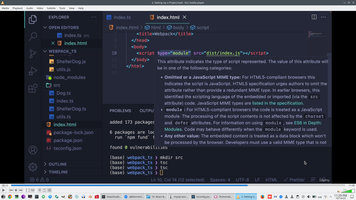
Start project and see result, see each web request in console, project working correctly.
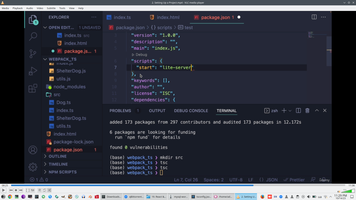
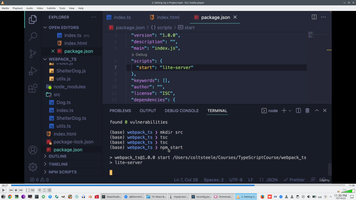
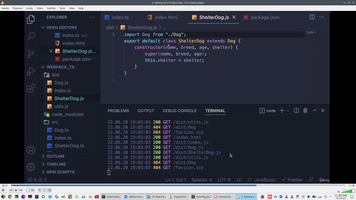
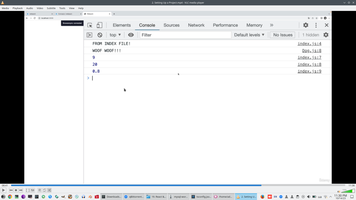
Each script and resource generate separate request to server.
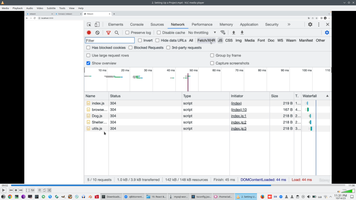
Install lodash and lodash types"npm i lodash @types/lodash", https://lodash.com/ (Lodash makes JavaScript easier by taking the hassle out of working with arrays, numbers, objects, strings).
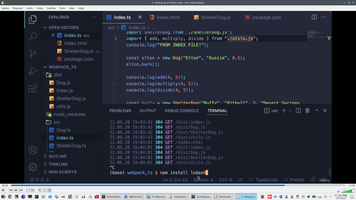
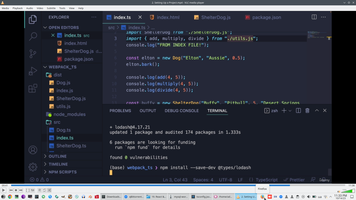
Install Webpack, webpack-cli, typescript, ts-loader. All need to install locally '--save-dev' to store current Typescript version to future development.
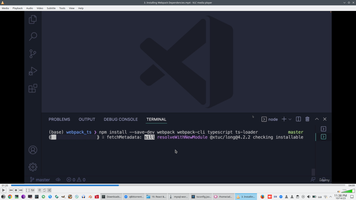
What dependency we can receive on config after installation.
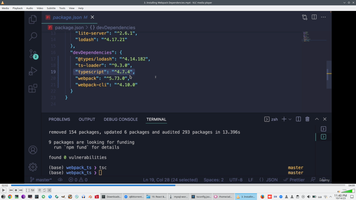
What is Webpack at all, https://www.npmjs.com/package/webpack, what is Webpack loaders https://webpack.js.org/loaders/.
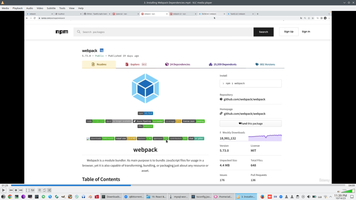
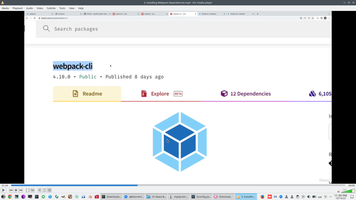
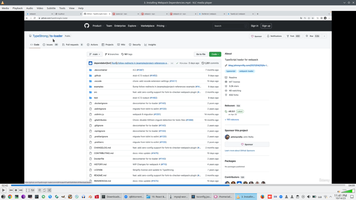
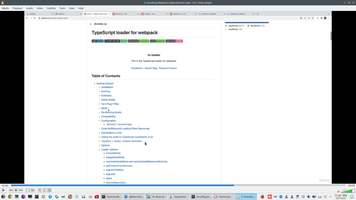
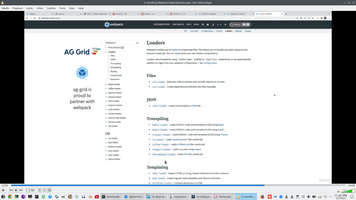
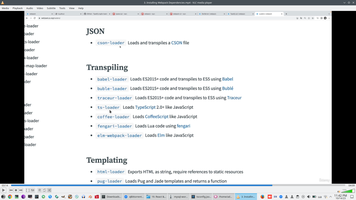
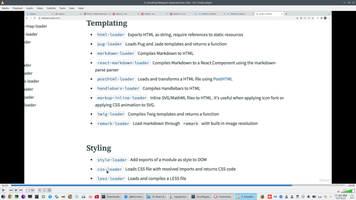
Try to first recompile Typescript code.
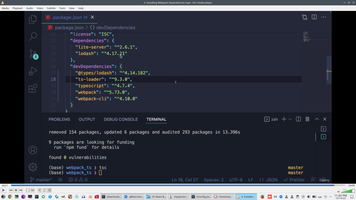
Main future of Webpack - setup Webpack.config.js
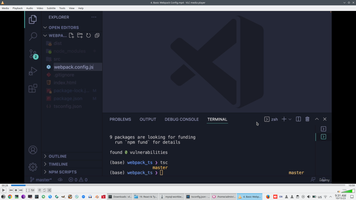
Instruction how to setup Webpack.config for Typescript.
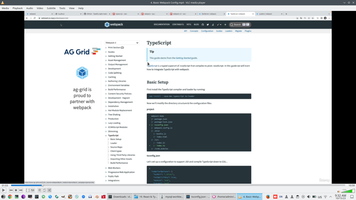
Webpack.config.js will be JS module.
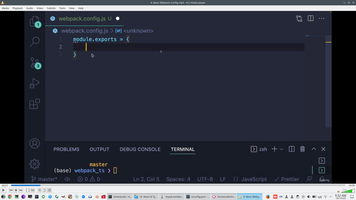
As start point we can simple copy config from Webpack decumentation.
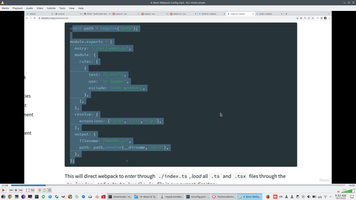
Currently we will processing *.TS files, but if we will working with React we will processing *.TSX files.
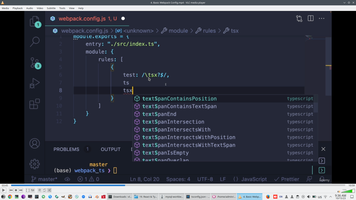
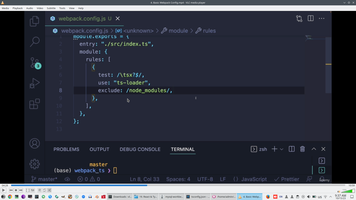
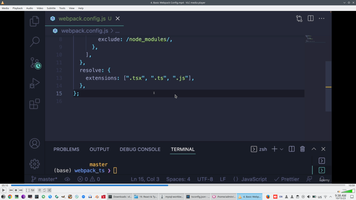
Important point is place from we will import *.TS files and result file name. For working with directory we will use "path" function.
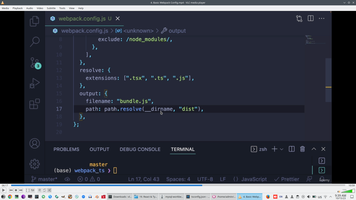
And we need to import this function to Webpack config.
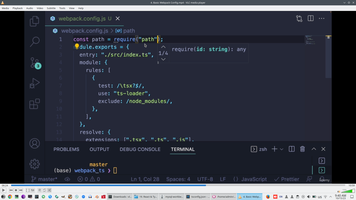
Next step is configure NPM command - "Start" will be working with Lite-server, "Build" will be working with Webpack server.
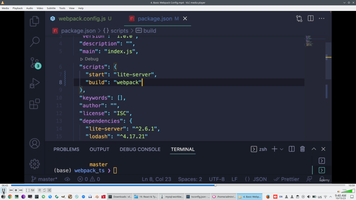
First start unsuccessful, because for Webpack we will working with *.TS files and we need update imports without extension.
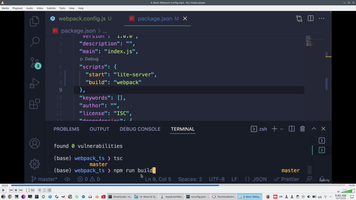
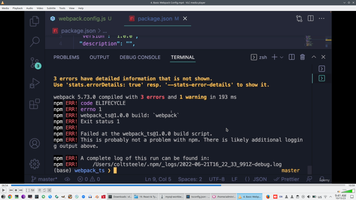
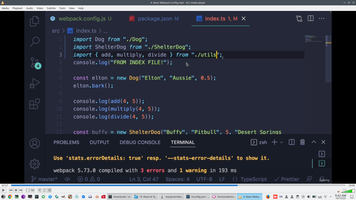
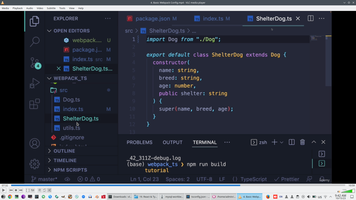
Also we need to change qualifier of class visibility from public.
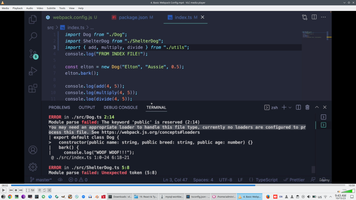
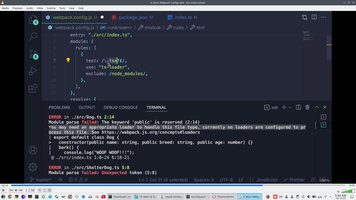
And after fix this two issue first package .TS files is happens. Bundle.JS is result of Webpack.
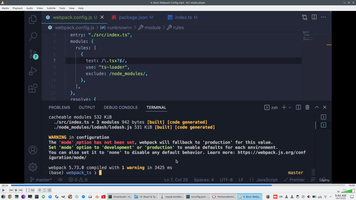
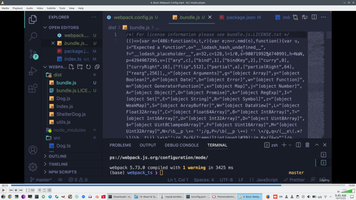
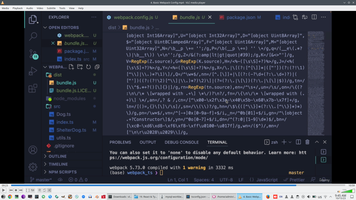
Time to delete "module" on script tag and change "index.js" to "bundle.js".
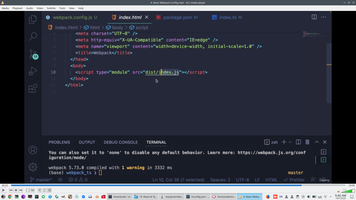
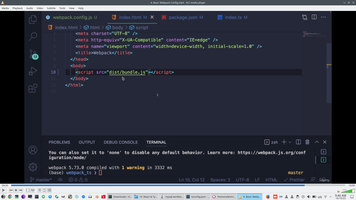
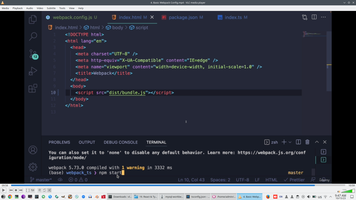
Look to Browser, all working fine, and Bundle.js loaded as one file.
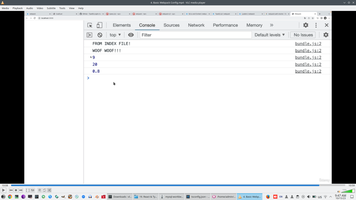
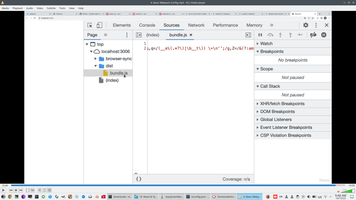
For receive access to source *.TS file we need to produce SourceMap by Typescript compiler and include it to Webpack config..
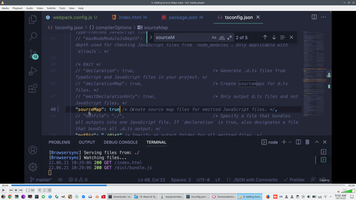
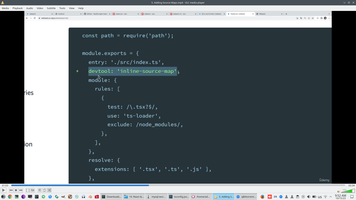
In this case we can see Typescript source on Browser.
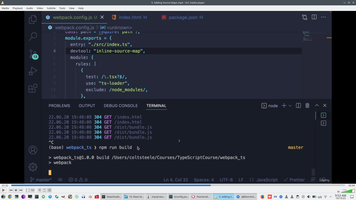
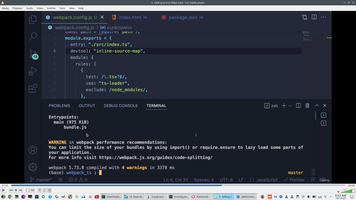
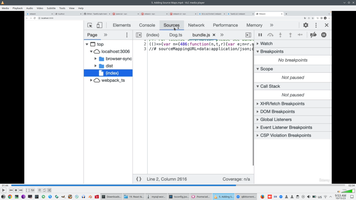
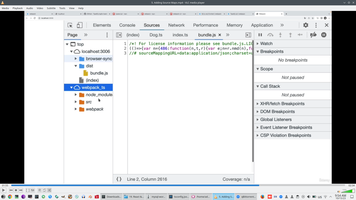
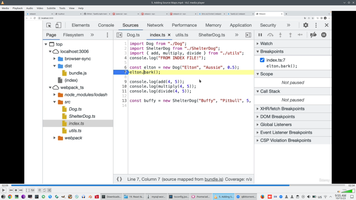
Start using development and production mode, now will add Webpack development server. In development mode we can see source of Bundle.js
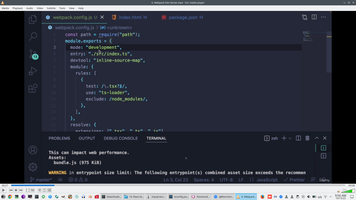
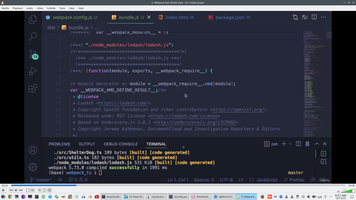
Start local installation "webpack-dev-server". Replace "lite-server" to "webpack serve" (working in memory only), mode of Webpack development server.
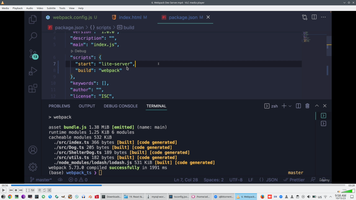
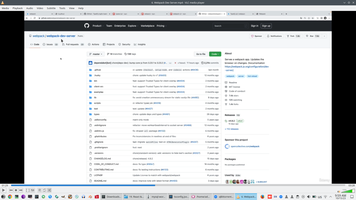
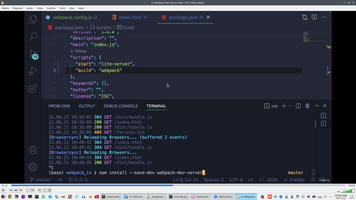
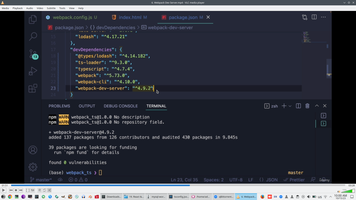
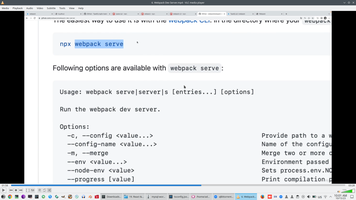
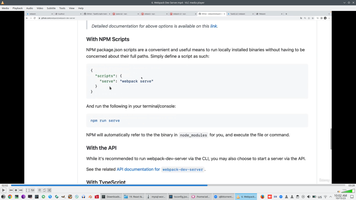
And now "npm run serve" will start Webpack development server with parameter "server, "npm run build" without it https://webpack.js.org/api/cli/#commands
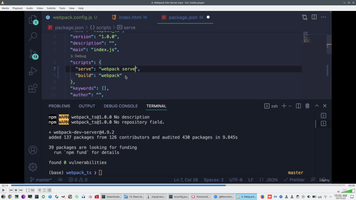
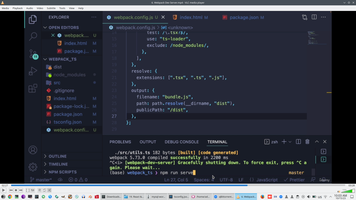
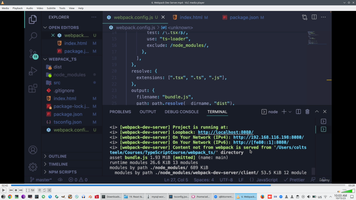
Now project successfully working on webpack-dev-server.
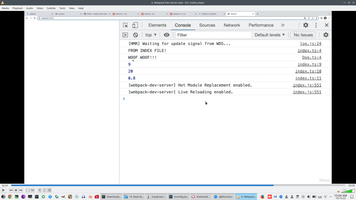
On development server we can see bundle.js clearly.
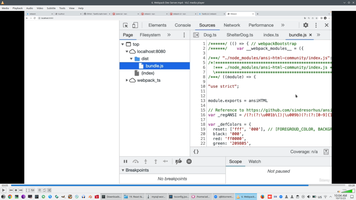
Development server working on port 8080.
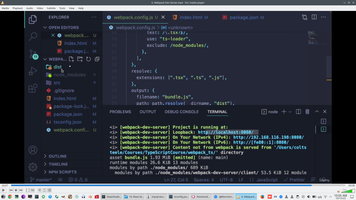
Try to start "npm start build" and look clear bundle.js as file.
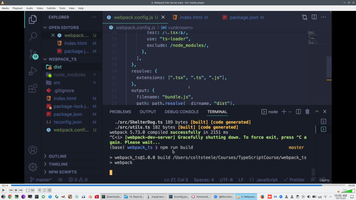
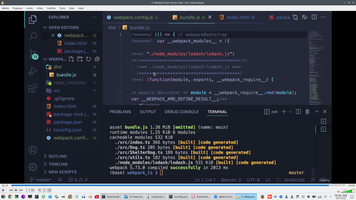
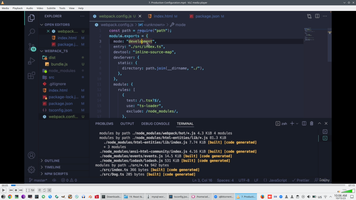
Create production copy of webpack config "webpack.prod.js" and look to result on disk.
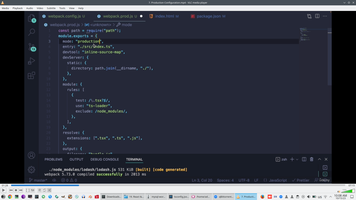
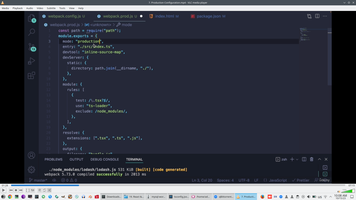
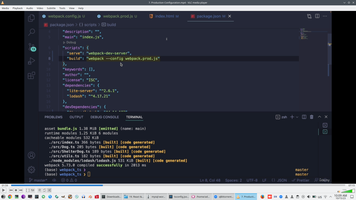
setuo poduction config to "npm run build command"
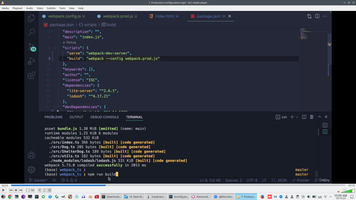
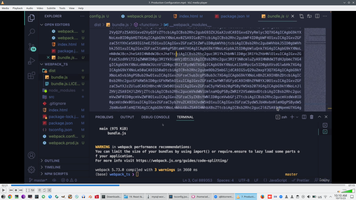
Create versioned bundle.js, add hash prefix.
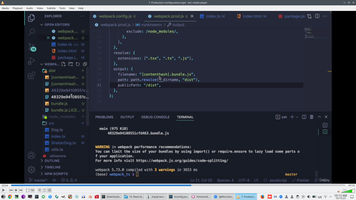
Add package to clean /dist directory from previous version - clean-webpack-plugin.
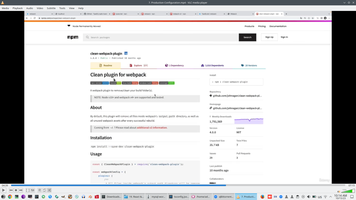
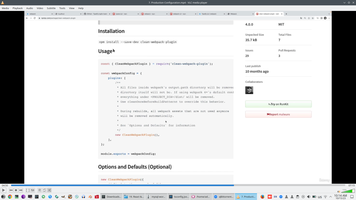
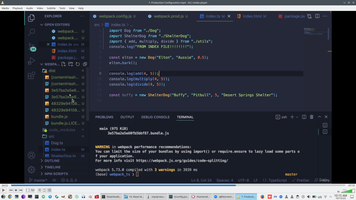
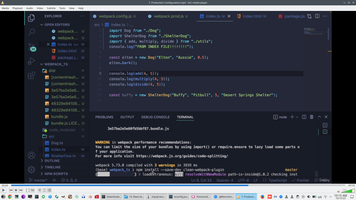
Add pluging to production config.
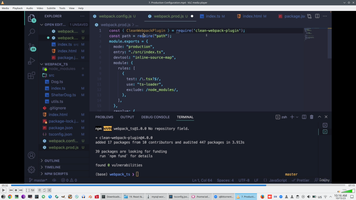
4. Typescript & WebPack
1 - Module Introduction.mp4 2 - What is Webpack & Why do we need it.mp4 4 - Installing Webpack & Important Dependencies.mp4 5 - Adding Entry & Output Configuration.mp4 6 - Adding TypeScript Support with the ts-loader Package.mp4 7 - Finishing the Setup & Adding webpack-dev-server.mp4 8 - Adding a Production Workflow.mp4 9 - Wrap Up.mp4
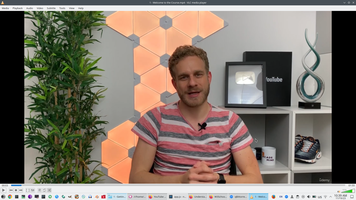
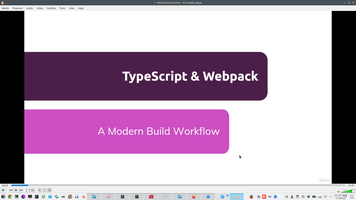
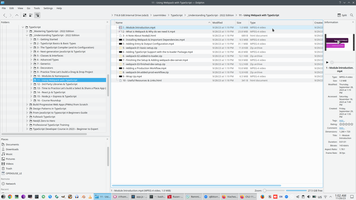
We will refactoring project with ES6 module and now we see a bunch of Http request
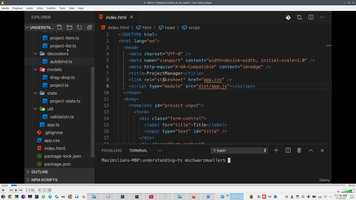
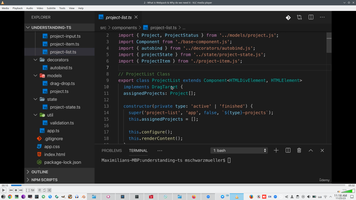
On server we have a lot of latency for each request, we need reduce Http request
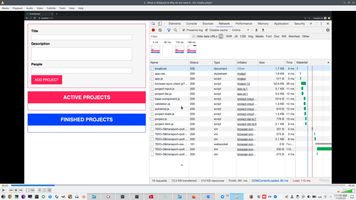
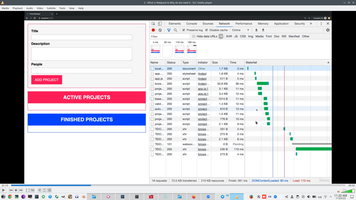
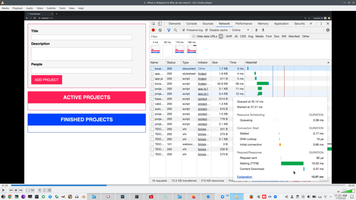
Webpack is tools what help us bundle all request together
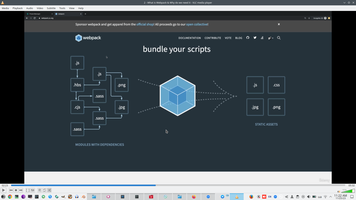
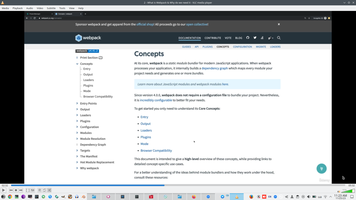
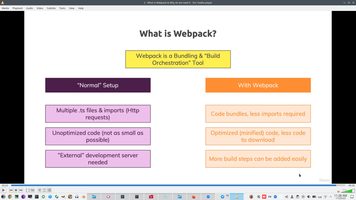
Installation, we need to install all 5 packages. Lite server allow automatically reload site, npm i "--save-dev" mean developer only
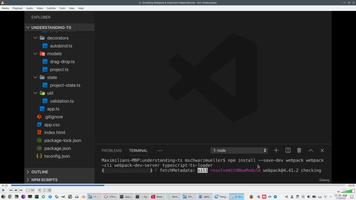
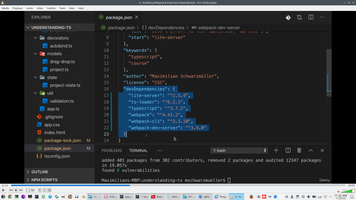
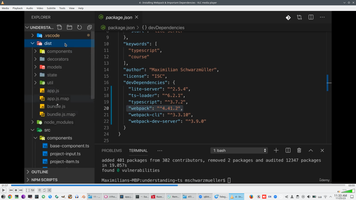
Good practice is lock TS version locally on project.
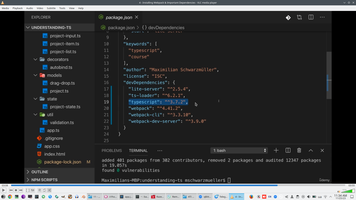
We have ES5 modules on Dist folders. TsConfig configuration (1`) Target ES5 or ES6, (2) Module - 2015, (3) OutputDir, other configuration of WebPack.Config.JS
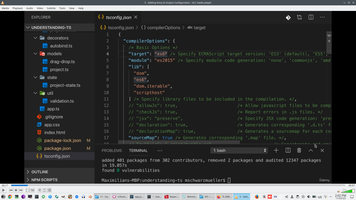
Firstly need to remove all *.JS extension in all Imports
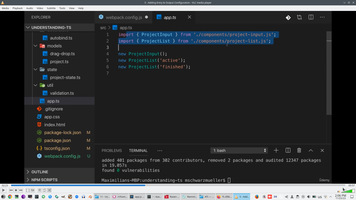
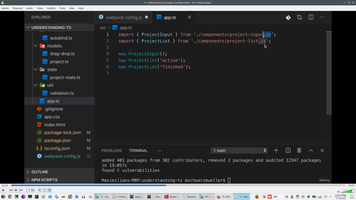
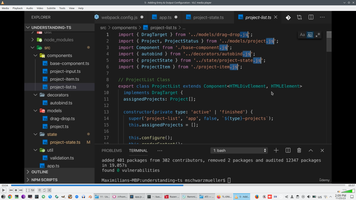
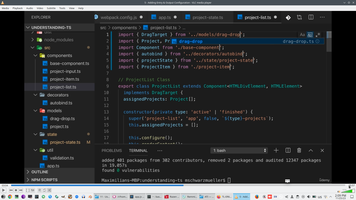
Start configure WebPack.Config.JS
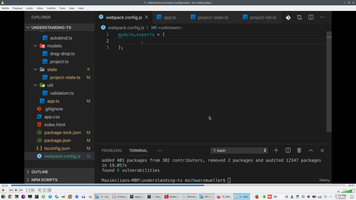
test file with *.TS extension from folder, output can be with Hash ("[..]" is dynamic path)or without dynamic pat
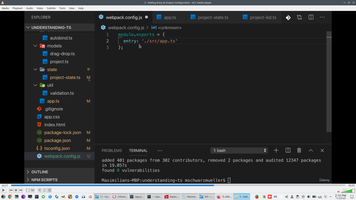
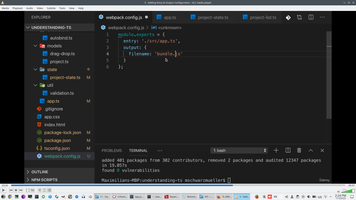
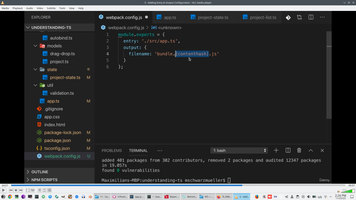
Import Path module and resolve output folder
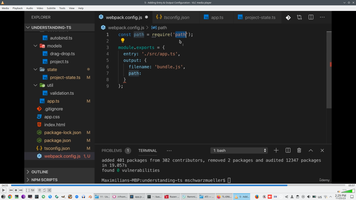
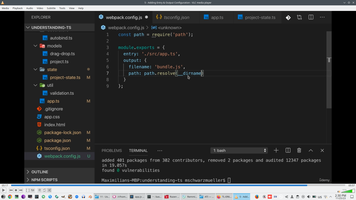
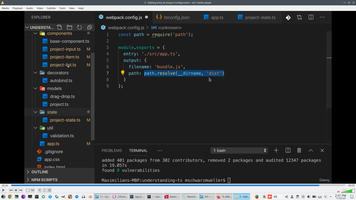
Ts_Loader also uses WebPack.Config.JS
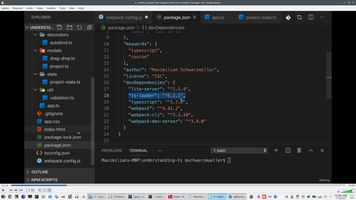
Configure Ts_Loader - this is Regular expression
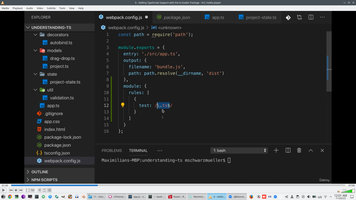
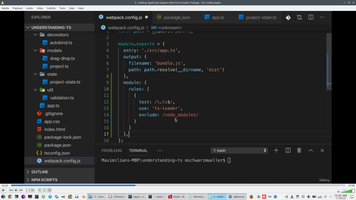
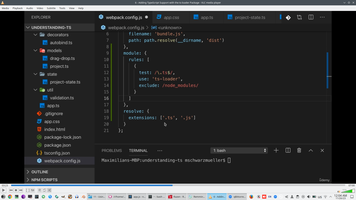
SourceMap need to debugging
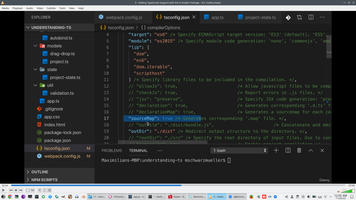
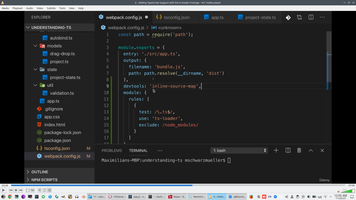
Script on Package.Json - how to call WebPack by NPM
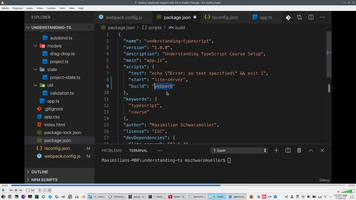
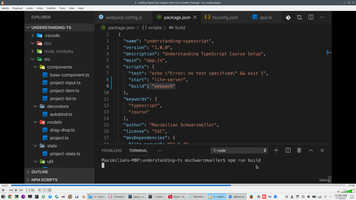
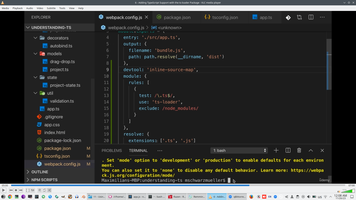
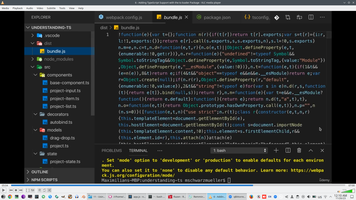
Ref to WebPack output file from Html
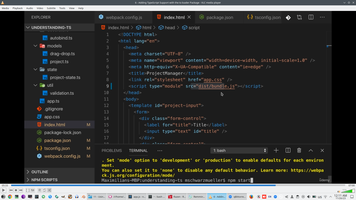
And test how it working, SorceMap included therefore souse TS file is accessible to show and breakpoints
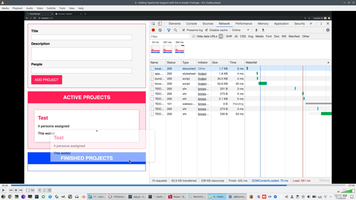
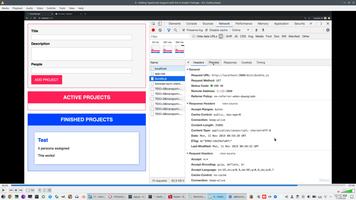
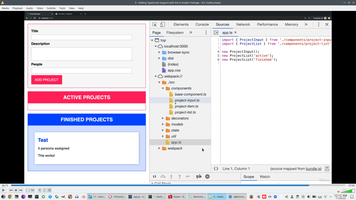
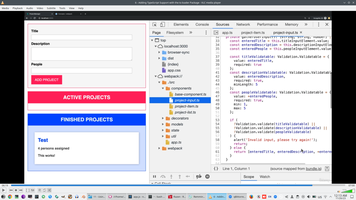
Hot refresh not supportws by Web-Pack-Dev-Server because WebPack working on memory, need to add additional parameter Path
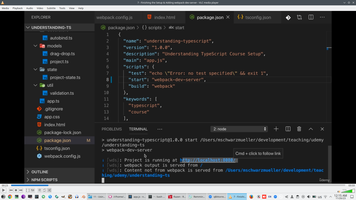
And now Hot refreash and reload supported
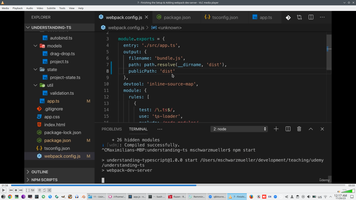
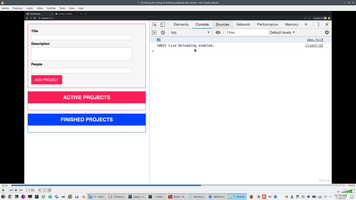
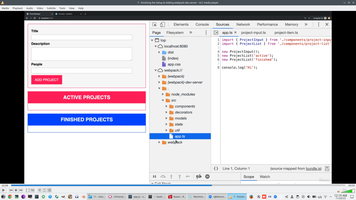
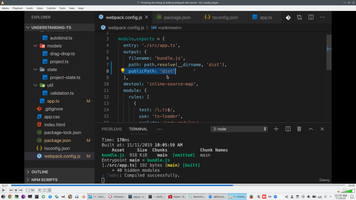
This was Development mode WebPack and now we will create Production mode config
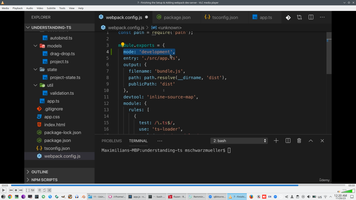
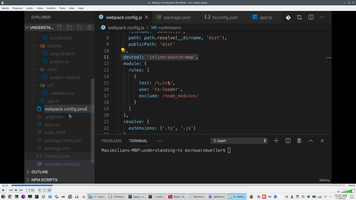
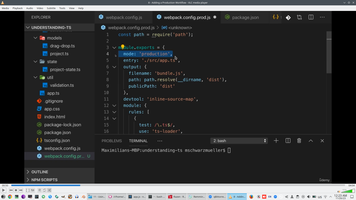
DevTools set node
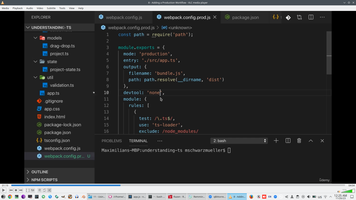
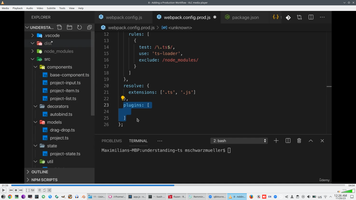
WebPack server has a lot of Plugins, most usable is cleaner output folder plugin
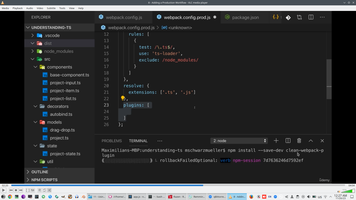
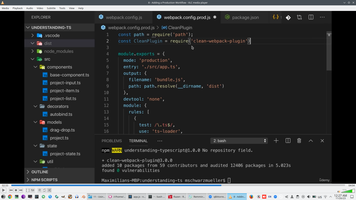
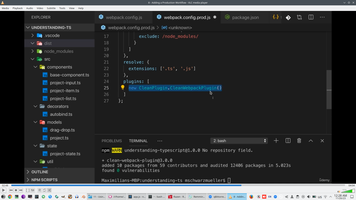
On production mode WebPack server will work instead WebPack-Dev-Server
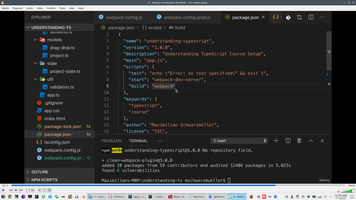
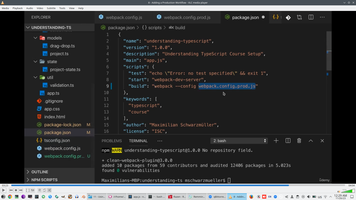
And Link production config to WebPack server
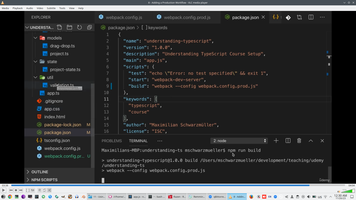
Result is fine
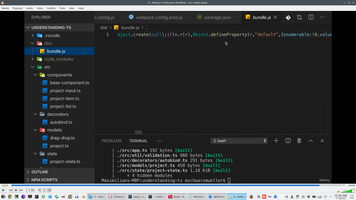
5. Related page:
- (2023) CloudflareWorker and Supabase
- (2022) NativeScript, AndroidBooks
- (2022) JS, Css
- (2022) Typescript, Webpack
- (2022) Angular, RxJs, Firebase, MongoDb
- (2022) Node, NestJs, Electron, Pwa, Telegram
- (2022) React, Redux, GraphQL, NextJs
- (2022) Angular/Typescript, JS books

<SITEMAP> <MVC> <ASP> <NET> <DATA> <KIOSK> <FLEX> <SQL> <NOTES> <LINUX> <MONO> <FREEWARE> <DOCS> <ENG> <CHAT ME> <ABOUT ME> < THANKS ME> |