Typescript Learning StartPoint
- 1. What is Typescript at all?
- 2. Install Typescript compiler and runtime.
- 3. Install debugger and developer services.
- 4. Run Typescript code in various mode.
- 5. TypeScriptlang.org
- 6. How to start WebDevelopment on Typescript with Lite-server and Webpack server
- 7. Max Typescript Lecture
- 8. Typescript brief on Angular lectur
- 9. Typescript Colt lecture
- 10. Typescript Matt Pocock lecture
- 11. DigitalOcean.com
- 12. Egghead.io
- 13. Related pages
This is my start point for learning Typescript (without Angular) https://github.com/AAlex-11/TypescriptLearningStartPoint/tree/main.
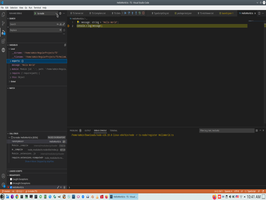
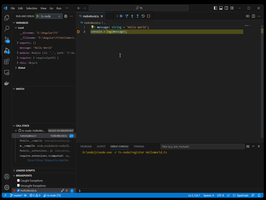
1. What is Typescript at all?
Globally TS is no more than package of JS extension, main future of this extension is adding Types to type absent JavaScript. To understand this look to this 5 files https://github.com/Alex-1347/TsTypesSelfTest
2. Install Typescript compiler and runtime.
1. Install Node.JS and check version.
# node -v
2. Install typescript locally to project.
# npm i typescript --save-dev
Two files will appears after installation
+ package.json + package-lock.json
3. Create ini typescript compiler file
# npx tsc --init
File with compiler options appear.
+ tsconfig.json
4. Install ts-node compiler.
# npm i ts-node --save-dev
5. There are alternative compiler, this is comparison https://github.com/privatenumber/ts-runtime-comparison, between https://github.com/TypeStrong/ts-node and https://github.com/esbuild-kit/tsx.
The main difference is that tsx transpile all the file according to your tsconfig. Instead, ts-node will start from the entry file and transpile the file step by step through the tree based on the import/export.
6. Also for 99% program need more packages, for example.
# npm i @types/node --save-dev
3. Install debugger and developer services.
https://marketplace.visualstudio.com/items?itemName=kakumei.ts-debug or other, after installation file .vscode\launch.json appear. After that we can use VS Code debugger as Run Debug -> Ts-Node.
Also there are so called AstViewer for TypeScript, there are extension for VS Code https://marketplace.visualstudio.com/items?itemName=saber2pr.ts-ast-viewer, and the same service there is online https://ts-ast-viewer.com/, https://astexplorer.net/. How to Read JavaScript Source Code, Using an AST
4. Run Typescript code in various mode.
After installation we can try to start TypeScript code, there are two node - simple command line and on server code. For simple command line code we can start with debugger (Run Debug -> Ts-Node) or with simple command line
For Linux debugging debug commands look as
# /home/admin/Downloads/node-v16.19.0-linux-x64/bin/node -r ts-node/register HelloWorld.ts
For Windows:
# D:\nodejs\node.exe -r ts-node/register HelloWorld.ts
Pay attention that console output and commands appears on "VS Code debug console" windows. Alternatively we can start TS application as console aplication.
# npx ts-node HelloWorld
Alternative way is install Express package and run, this is way to start Typescript code as server code.
- https://github.com/VinitGurjar/REST-API-Node.js/blob/main/Node-Express-REST-Boilerpalte/recipe.js
- https://www.digitalocean.com/community/tutorials/setting-up-a-node-project-with-typescript
We can start ONE Ts file as server Node.JS code with npx ts-node or start all project according tsconfig.json npx tsc, or start ONE TS file as server code npx tsc HelloWorld.
# npx tsc HelloWorld
Alternatively we can start TS code on browser by various way, first way is create bundle with WebPack OR compile TS to JS and start JS on Browser with Require.JS, see example on may page My RxJs learning conspectus
Alternatively we can use Vite local web server with HMR
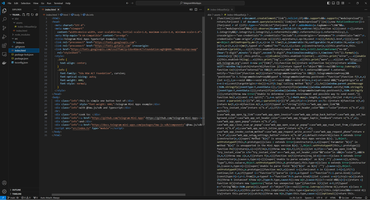
prepare this package.json
"scripts": { "dev": "vite", "build": "tsc && vite build", "lint": "eslint . --ext ts,tsx --report-unused-disable-directives --max-warnings 0", "preview": "vite preview" },
And call Typescript code as
#npm run dev #npm run build #npm run preview
5. TypeScriptlang.org playground
- Handbook https://www.typescriptlang.org/docs/handbook/declaration-files/introduction.html
- 4 page summary https://www.typescriptlang.org/cheatsheets
- JavaScript Essentials
- Functions with JavaScript
- Working With Classes
- Classes 101
- This
- Generic Classes
- Mixins
- Modern JavaScript
- External APIs
- TypeScript with Web
- TypeScript with React
- TypeScript with Deno
- TypeScript with Node
- TypeScript with WebGL
- Primitives
- Type Primitives
- Meta-Types
- Language
- Language Extensions
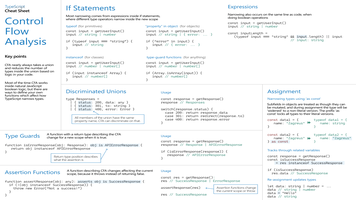
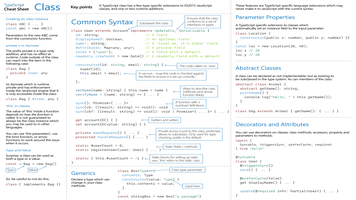
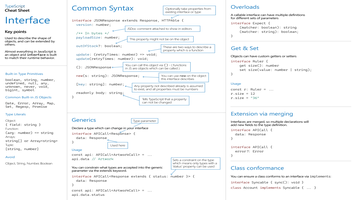
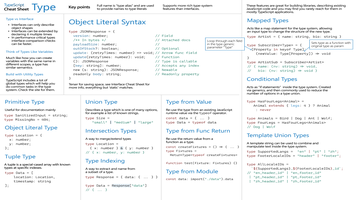
6. How to start WebDevelopment on Typescript with Lite-server and Webpack server
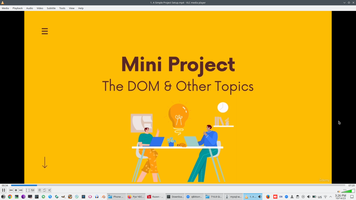
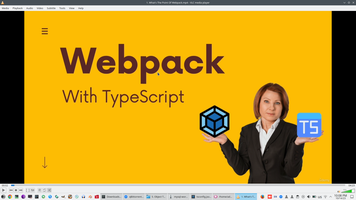
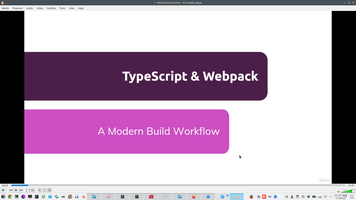
7. Max Typescript Lecture
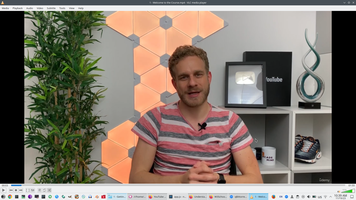
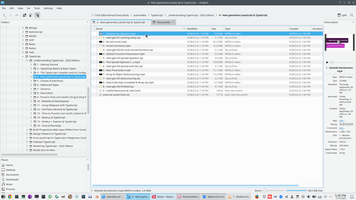
All TS future is accesible on Compilation time, on Runtime we receive just only JS We can create JS TypeOf TypeGuard just based on simple JS types, We can use JS TypeOf with Number or String, but don't use with Date, because JS DateType is missing JS Runtime not check variable type, On TS we can avoid to use type Any, any this is fallback TS intercept all possible issue in Compilation time - 1,2 + 1,2 = 2; 1,2 + 1.2 = 3.2; +'1.2' + 1.2 = 2.4; '1.2' + 1.2 = "1.21.2"; 1,2 + '1,2' = "21,2"; 1,2 + +'1.2'= 3.2; 1.2 + +'1,2'=NaN; 1.2 ++'1,2' = invalid increment/decrement operand; 1.2 || 1,2 = 2; 1.2 || '1,2' = 1.2; '1.2' || '1,2' = "1.2"; '1.2' && '1,2' = "1.2"; '1.2' && 1,2 = 2; '1.2' && 1.2 = 1.2; '1.2' && '1,2' = "1,2"; 1,2 ?? '1,2' = 2; 1.2 ?? 1.2 = 1.2; '1.2' ?? 1.2= "1.2"; +'1.2' ?? '1.2' = 1.2; '+' is opposite to ToString(), convert to Number We can use keyword 'Object' and '{}' for TS Object, Object type separate property with ';', but object with ',' Array, TS Array need type Any[] to support different type item, OR we can use Union Type String | Number Tuple declaration, Push to Tuples look strange Convention for Enum is started with UpperCase, We can start assign Enum from any value Literal type, Literal type (with Union type) Type Alias Void type, JS don't know what is Void, TS Void produced JS Undefined. Ts Undefined is not the same that TS Void TS Function type - reference to JS Function, use Function name without '()' On TS we omit type declaration, we use explicit type declaration instead implicit Definition Anonymous function and pass it as parameter, Definition Callback function as parameter, Pass Anonymous function as Callback TS Unknown is more restricted that TS Any, We can avoid Any and use Union type as possible TS Never type. We used Never usually if function finish with Throw
Class, On JS ES6 we see the same code as on TS, but on ES5 class implements as Prototypes Property, Default property value, Constructor, Methods (default is Public), Private value, This Instantiate with New and without New with {} Shortcut - declare private variables on Constructor Private constructor, Super Singleton, GetInstance Extends - Class Inheritance Static methods, Abstract class and Abstract some method - force user to implement that one method Interface look as Abstract class, but without implementation. Interface vs Custom type, Custom type is more flexible (we can use Union type), but we can share interface to many class and class can implement multiple interfaces. Interface definition can not be Public/Private (it's always public), but can be Readonly Class can implements many interface, Interface can extends one another Custom Type the same as interface. "!", and "?" - mark property and method as optional Alternate way to make parameter optional - set default value
"&" Type intersection operator, This will be the same intersection if we create two interface and Extends new interface from both of they Type Guard choice 1 (only for JS simple type) - TypeOf,W e can not use this approach to TS Types and Interface, because JS known nothing about its Type Guard choice 2 (check if property is exist) - IN Type Guard choice 3 (check if class instantiated) - InstanceOf, this way can not be used to TS Type and TS Interface, because JS know nothing about they Type Guard choice 4 - discriminated union, add implicit type as property. We working with "DOM" library on configuration, therefor we have access to any HtmlElement. First way of Type casing is cast by angular brackets <>. And we need to use "!" exclamation mark. Second way of Type casing is casting by AS (this way also working with React) Or we can delete exclamation mark with manually checking presentation Html element Indexed property - we don't need specify exactly property name, we will declare just property count and type. Interface X {[prop:string]:string]} Empty, or any property with string name."1" will be interpreted as string in this case. Also ee will declare property name with string array Function overloading One way in typecasting Result with AS Second way - create declaration for function overloading, function definition only without cali-brackets {}, Ts engine will be understand this overloading. Optional chaining operator - "?" We can check property one-by-one on this way (if job is undefined second part after "&&" never run) Or simple use optional chaining "||" Nullish coalescing operator - is JS future, but on JS this is "??" "''", "undefined", "null" look as False and variable with "||" assign with "Default" value. If we want to use exactly "null" or "undefined" (but not "''" empty) - we need to use "??" Nullish coalescing operator
If we use union type or complex types or less precise type we have a number of way to clarify type and use more precise type - this is type narrowing. 1. JS Typeof Guard 2. Truthiness guard (check by IF). These values {0, NaN, "" (the empty string), 0n (the bigint version of zero), null, undefined} - always get False on IF checking. Other get True on IF checking. 3. Equality narrowing. Comparing without transformation to one type (===, !==) or with transformation to one type (==, !=). 4. IN narrowing - check if object of prototype has property 5. InstanceOf narrowing - check prototype chain, and object created with New 6. Type predicates AS - TypeScript will narrow that variable to that specific type if the original type is compatible 7. Discriminated unions - store literal as one of property 8. Using Never type to receive exception if type is wrong 9. Also type can be checking by Assertion Functions, NodeJs generate AssertionError if checking by Assert function generate exception, JS has only Console.Assert function
Generic Array (with "<" ">" angular brackets) and Predefined Array of string Define empty array with square brackets "[" "]", we can initialize in this way any kind of array. But correct definition allow us working with type safe Array<string> We have no information what data return JS Promise, But we can define return datatype Promise<string> Object.Assign is JS method what allow copy all property from Source to Target object, But TS not sure that property exists on target object, We can type cast of result object with "AS" Or create Generic object Result have intersection of type U "&" T, because for this function input parameters "T & U" explicitly setup output type as interception "T & U" Constraint - limitation or restriction, we want to protect from call this function with simple value, because this function working only with Objects. Extends keyword help us. In this case function return tuple (value with description), Length property must bu exist, Also we can specify implicit output type KeyOf Constraint - this is simple JS function, but this is not working on TS, Because TS can not guarantee that key exist in object U extends KeyOf T - guarantee that U property exist in T object Generic utility types - Partial and Readonly - fix all object property of make it optional (locked ot open to modification) Partial mean that all property is optional, therefore we can create empty object firstly and than step-by-step adding property. And finally casting to clear type with "AS". How to make array locked? Readonly forbid changing any property Union types vs Generic types. Union types allow story mixed value, buy Generic types allow stre only one object type. Generic type allow lock one certain type.
Namespaces, Export, Export Default, /// reference, Html 'Script' tag with defer (async) (Type=amd on compiler options) with bundle all JS to one, Html 'Script' Type=Module (ES6 on compiler options), Import variation (omit {}, alias 'as', *)
install @types/xxx --save-dev, Lodash, Validators, using class-transformer and import 'reflect-metadata', declare external variables from Html level (setting)
TS development environment (Lite-server, tsc -w) default TS library var/const/let arrow function syntax (with variation, omit () and {}) '...' spread operator (4 type of usage) Destructuring assignment
@C @D class Person {} the same as C(D(class Person())). Allow decorator in TS config. Constructor:Function - this is decorator factory, Function return Function - in this case we can use Decorator as Function. Pass parameters to Decorator. Bind DIV ID from HTML template to Decorator function. "_" underscore parameters. Changing constructor type to Any allow to create new constructor. Use "!" exclamation mark to guarantee as HTML element present. By Decorator Angular framework to pass HTML template to code. Multiple decorator. Two types of decorators - Logger factory and Template factory. Decorator to Method Accessor, Class, Property, Method Parameter - only interface has difference. Common decorator interface (with Symbol). Order of multiple Decorator. Any Decorator working when JS engine faced with class, not when class instantiated. Class extended Constructor and we will used Super (MyBase). New Constructor function will replace original constructor function with new extra logic. Generic '<T extends { new (... args: any []): () } >' allow accept any parameters. Anonymous class with Anonymous method. Generic - decorated must have property Text. If we don't instantiate class, Template decorator don't working (because document not exists and don't rendered), When we call Super we save original function, and we save original class but we replace original class with new extra logic. Decorator can return anything, but TS will ignore what decorator return. We can change options of Method of Accessor, for example Configurable, Enumerable. JS Bind() and AutoBound decorator. This is different on EventListener and Class. Create decorator Antobind() what change context from Button.EventHandler to Class exemplar. Parameters of Method decorator, (1) Target is Prototype because we use Instance method, (2) MethodName or PropertyName, (3) ProertyDescriptor, Validation decorator. Text to Digital by '+. TS validations with decorators.
8. Typescript brief on Angular lecture
- 460 Module Introduction
- 461 What & Why_
- 462 Installing & Using TypeScript
- 463 Base Types & Primitives
- 464 Array & Object Types
- 465 Type Inference
- 466 Working with Union Types
- 467 Assigning Type Aliases
- 468 Diving into Functions & Function Types
- 469 Understanding Generics
- 470 Classes & TypeScript
- 471 Working with Interfaces
- 472 Configuring the TypeScript Compiler
9. Typescript Colt lecture
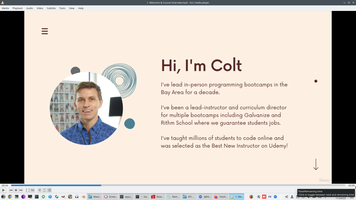
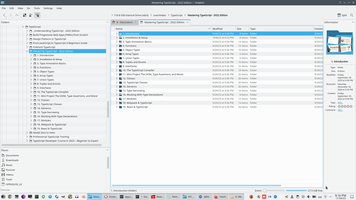
10. Typescript Matt Pocock lecture
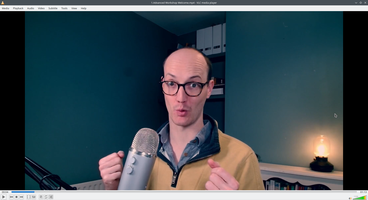
11. DigitalOcean.com
- Benefits of Using TypeScript(January 14, 2019)
- Getting Started With Angular Using the Angular CLI(May 6, 2022)
- How To Build a Bookstore Landing Page with Gatsby and TypeScript(July 22, 2021)
- How To Build a REST API with Prisma and PostgreSQL(November 8, 2022)
- How To Build a Type-Safe URL Shortener in NodeJS with NestJS(August 23, 2022)
- How To Create Custom Types in TypeScript(March 25, 2021)
- How To Deploy Load-Balanced Web Applications on DigitalOcean with CDK for Terraform and TypeScript(July 5, 2022)
- How To Run End-to-End Tests Using Playwright and Docker(August 19, 2022)
- How To Run TypeScript Scripts with ts-node(March 23, 2022)
- How To Set Up a Gatsby Project with TypeScript(July 14, 2021)
- How To Set Up a New TypeScript Project(May 9, 2022)
- How To Use Basic Types in TypeScript(March 16, 2021)
- How To Use Classes in TypeScript(July 9, 2021)
- How To Use Decorators in TypeScript(September 17, 2021)
- How To Use Enums in TypeScript(June 14, 2021)
- How To Use Functions in TypeScript(April 13, 2021)
- How To Use Generics in TypeScript(November 16, 2021)
- How To Use Generics in TypeScript(November 23, 2020)
- How To Use Interfaces in TypeScript(October 1, 2021)
- How To Use Modules in TypeScript(February 7, 2022)
- How To Use Namespaces in TypeScript(February 1, 2022)
- How To Use Type Aliases in TypeScript(December 17, 2020)
- How To Use Typescript with Create React App(April 2, 2021)
- Interface Declaration Merging in TypeScript(August 23, 2019)
- Introduction to Enums in TypeScript(February 3, 2017)
- Introduction to Interfaces in TypeScript(January 17, 2017)
- Module Augmentation in TypeScript(September 5, 2019)
- Object Rest and Spread in TypeScript(December 9, 2016)
- Polymorphic this Type in TypeScript(August 20, 2019)
- String Literal Types in TypeScript(December 5, 2016)
- TypeScript Enum Declaration and Merging(September 4, 2019)
- TypeScript Mixins(September 1, 2021)
- TypeScript Tuples(August 12, 2019)
- Union Types in TypeScript(November 28, 2016)
12. Egghead.io
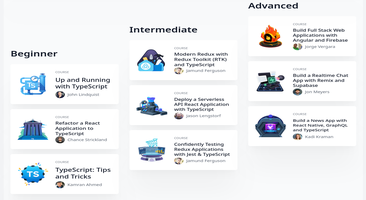
- Up and Running with TypeScriptJohn Lindquist
- Refactor a React Application to TypeScriptChance Strickland
- TypeScript: Tips and TricksKamran Ahmed
- Modern Redux with Redux Toolkit (RTK) and TypeScriptJamund Ferguson
- Deploy a Serverless API React Application with TypeScriptJason Lengstorf
- Confidently Testing Redux Applications with Jest & TypeScriptJamund Ferguson
- Build Full Stack Web Applications with Angular and FirebaseJorge Vergara
- Build a Realtime Chat App with Remix and SupabaseJon Meyers
- Build a News App with React Native, GraphQL and TypeScriptKadi Kraman
- Full Stack with React and AppwriteColby Fayock
- Build a Realtime Chat App with Remix and SupabaseJon Meyers
- Up and Running with TypeScriptJohn Lindquist
- Build a Twitter Clone with the Next.js App Router and SupabaseJon Meyers
- Maintainable CSS using TypeStyleBasarat Ali Syed
- Confidently Testing Redux Applications with Jest & TypeScriptJamund Ferguson
- Build a Modern User Interface with Chakra UILazar Nikolov
- Modern Redux with Redux Toolkit (RTK) and TypeScriptJamund Ferguson
13. Related pages
- Angular documentation
- Typescript documentation
- Javascript documentation
- ECMAScript ES6 vs Typescript
- React vs Angular
- (2023) CloudflareWorker and Supabase
- (2022) NativeScript, AndroidBooks
- (2022) JS, Css
- (2022) Typescript, Webpack
- (2022) Angular, RxJs, Firebase, MongoDb
- (2022) Node, NestJs, Electron, Pwa, Telegram
- (2022) React, Redux, GraphQL, NextJs
- (2022) Angular/Typescript, JS books

<SITEMAP> <MVC> <ASP> <NET> <DATA> <KIOSK> <FLEX> <SQL> <NOTES> <LINUX> <MONO> <FREEWARE> <DOCS> <ENG> <CHAT ME> <ABOUT ME> < THANKS ME> |