Node Learning
I like writing with Node API, for example with this code I make support my site by years, and finally I had time to publish it to Github https://github.com/Alex-1557/Node-FindReplace. And this is description of my first huge commercial project with Node and Express.
- Developer workflow. Developer workflow mean (1)install nodemon to automatic reload server, (2) setup correct launch.json on VS Code config and (3) setup script on package.json
- Common Express pipeline. (1) define various environment constant in .env with package dotenv, (2) define route endpoint in./routes/index.js, (3) define Express and function StartServer with all parameters for Express.USE(), (4) define Error handling middleware, (5) startServer.
- add API endpoint. To add endpoint need to do 3 point - (1) add endpoint to Index.js, (2) and method to API controller and (3) add DTO object, (4) ValidateDTO function.
- Strip gateway. Strip controller has 3 method:
- MySQL Query. For working with SQL I simple add a query with "?" instead parameters and pass parameters to query. In this case I also used uid generator.
- Pack complex object (like firebase access object) with Node Buffer.From function
- Various additional protection. Define in config JWT secret key and Static protection key and check it.
- GraphQL request
- Various Axios request to 3-rd party API
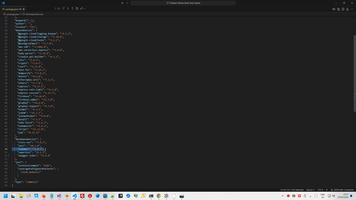
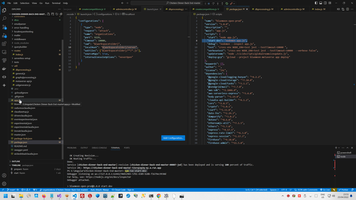
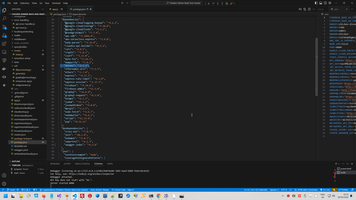
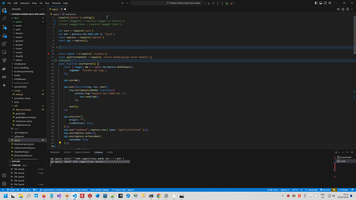
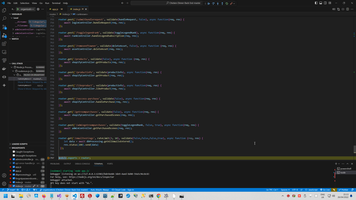
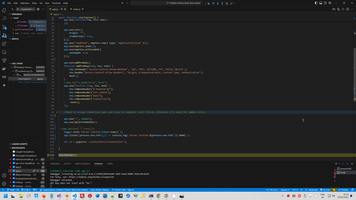
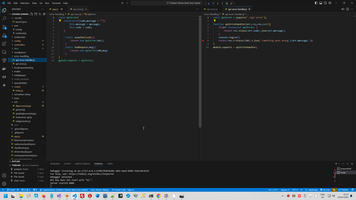
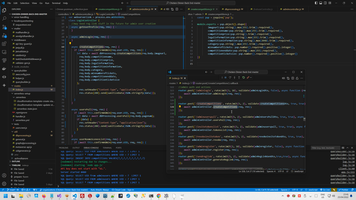
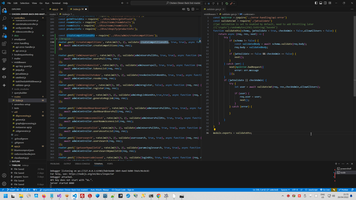
1: router.post('/webhook', async function(req, res) {
2: await stripeController.stripeWebhook(req, res);
3: });
4: router.get('/getbillinginfo', validate(false), async function(req, res) {
5: await stripeController.getSubscriptionInfo(req, res);
6: });
7:
8: router.get('/cancelsubscription', validate(false), async function(req, res) {
9: await stripeController.cancelSubscription(req, res);
10: });
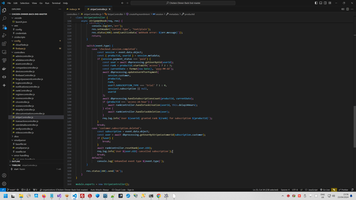
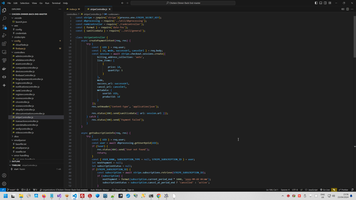
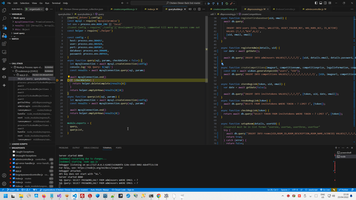
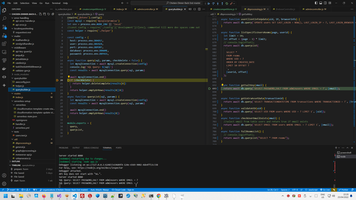
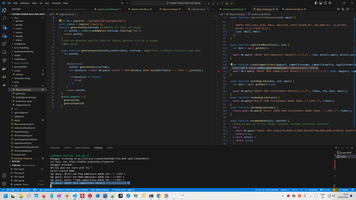
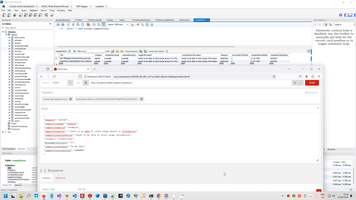
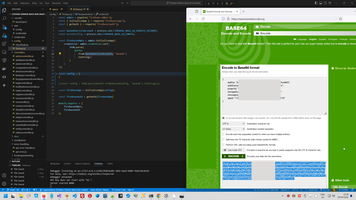
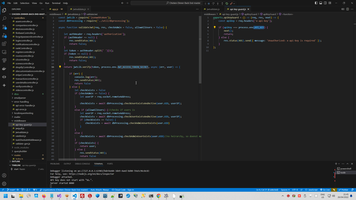
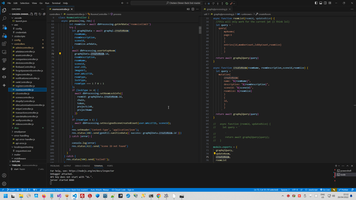
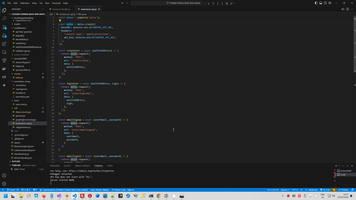
And now I decide to improve my skills and take lecture online from Maximilian Schwarzmüller:
1. Node.JS and Express
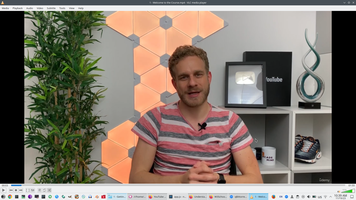
1 - Module Introduction.mp4 2 - Executing TypeScript Code with Node.js.mp4 3 - Setting up a Project.mp4 4 - Finished Setup & Working with Types (in Node + Express Apps).mp4 5 - Adding Middleware & Types.mp4 6 - Working with Controllers & Parsing Request Bodies.mp4 7 - More CRUD Operations.mp4 8 - Wrap Up.mp4
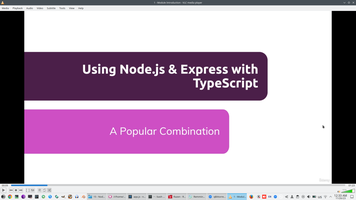
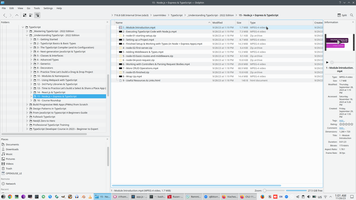
Node used only JS, not TS.
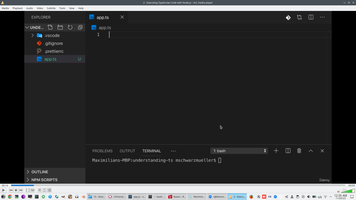
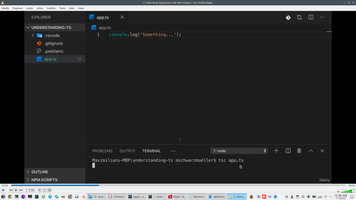
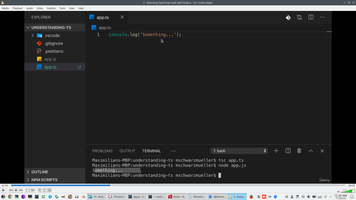
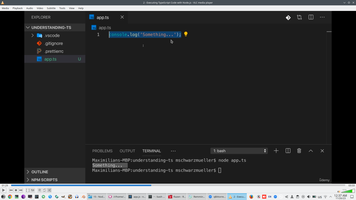
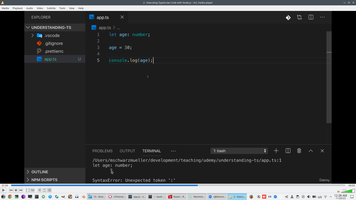
Ts_node allow excute TS, its cool tool for development, but not applicable to production with Express server
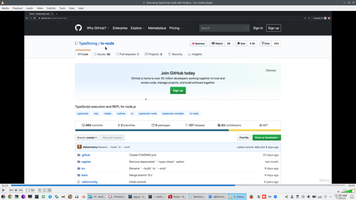
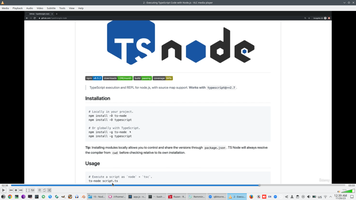
Initialize Node project and setup correct parameters on TsConfig.
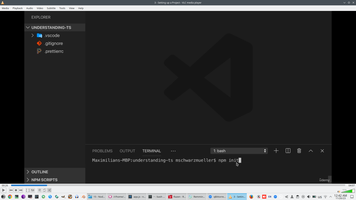
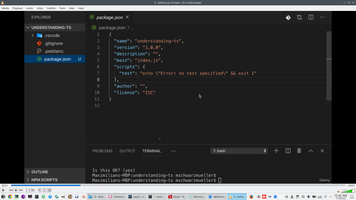
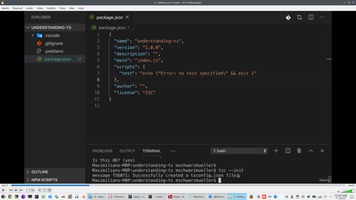
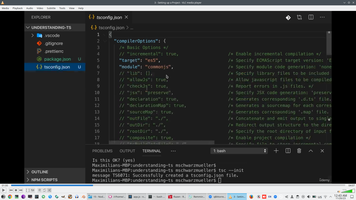
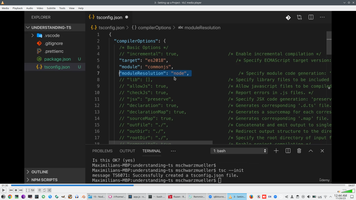
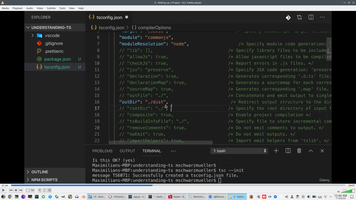
This way is possible, but with Experimental mode, we don't use it, just use TS
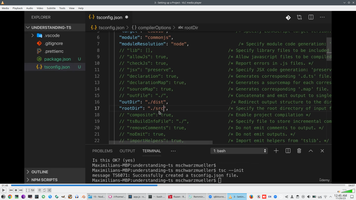
Install Express server and Body-Parser (to parse request). Install NodeMon to support HotReload.
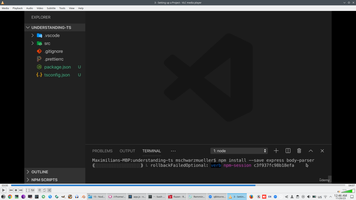
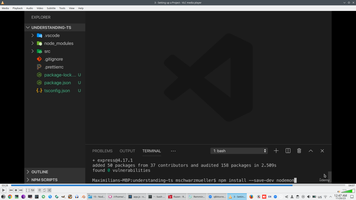
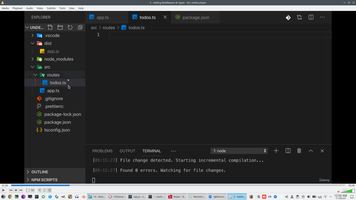
We listen port 3000, But Express require @Types, because currently we work without additional Library. So, Install @Types for Node.JS
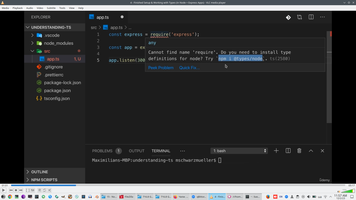
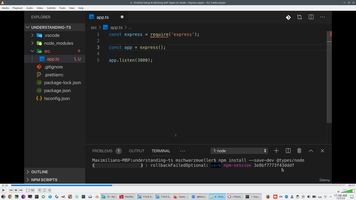
Configure Typescript - ModuleResolution is Node and no lobrary.
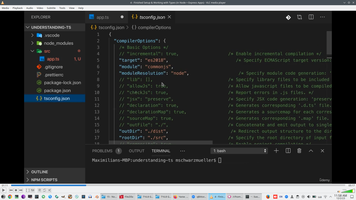
This is JS way, not TS, because Express has no types
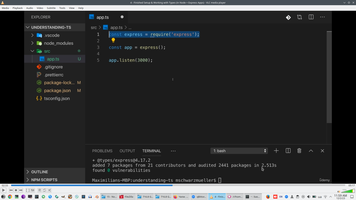
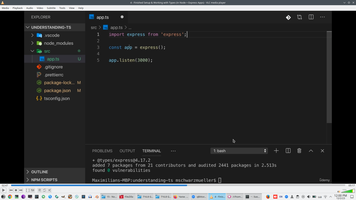
And now Express has Types
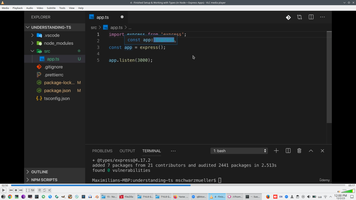
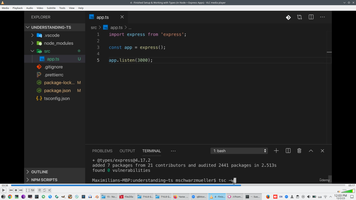
Js result of TS compilation
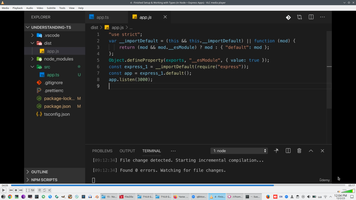
Npm Start will start Nodemon
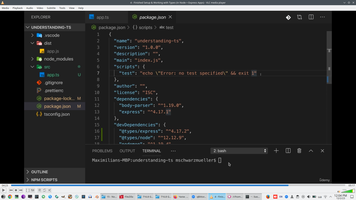
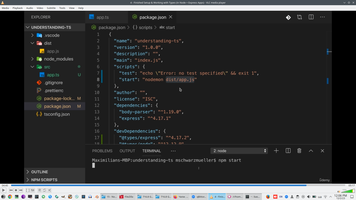
We start Nodemon and switch to second Bash
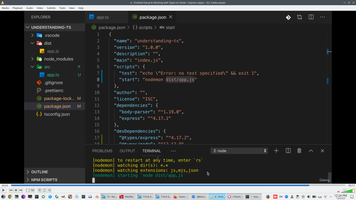
This is JS way, we don't need it, switch to TS Import directive
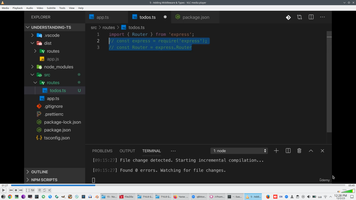
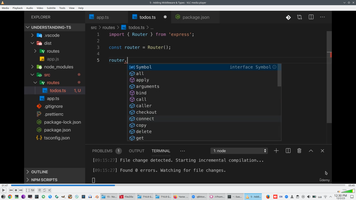
Get/Post doing nothing, but Patch require ID on URL Path
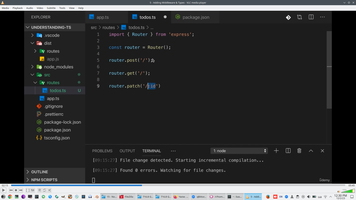
Import Routes to main app, we handle only Routes from Todos, other API not be handled.
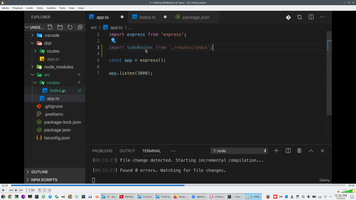
And registered MiddleWare with App.Use. This is Typical request Handler for MiddleWare to call Regular Middleware function
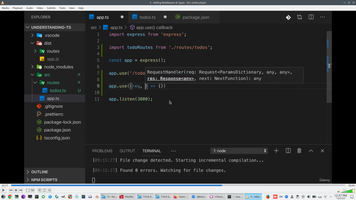
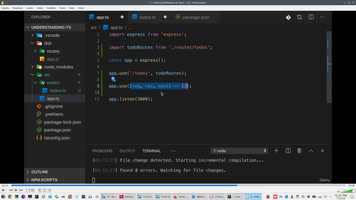
Request Handler with 4 parameters is Middleware with Error Handling
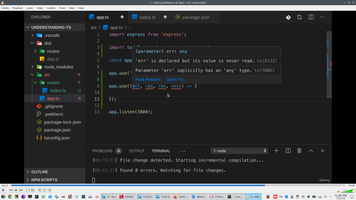
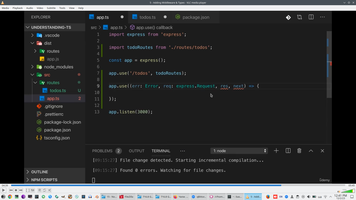
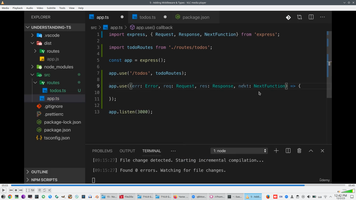
So, this is our Error Handler to catch Any error Request and with return Status 500
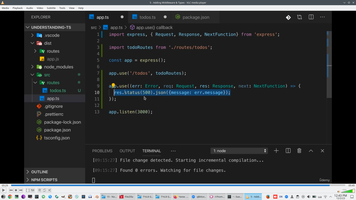
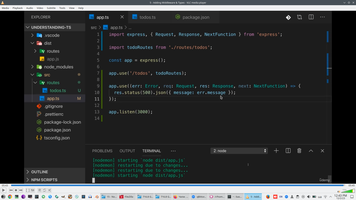
Create TodoS API, controller with Logic of application API
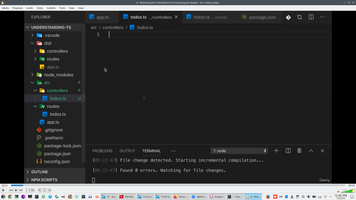
This is common Request processing function
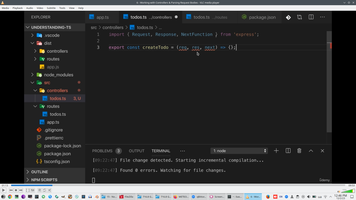
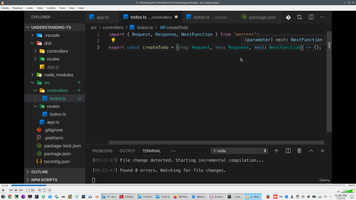
This of shortcut of Request processing handler
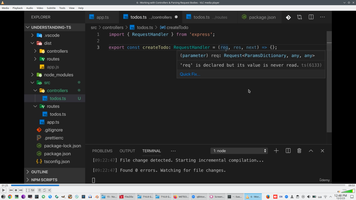
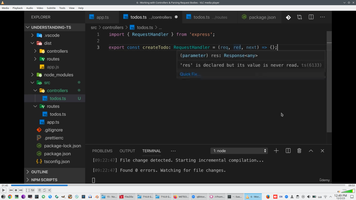
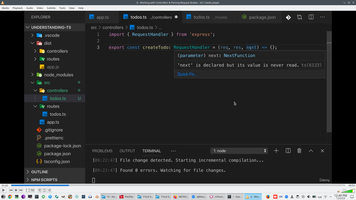
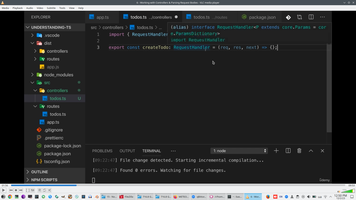
Create Models Folder with Model ToDo and Import this Model
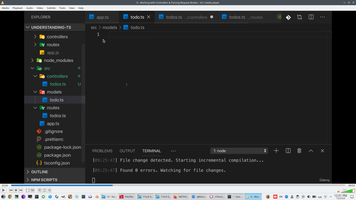
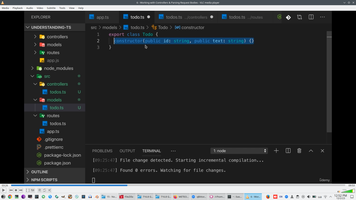
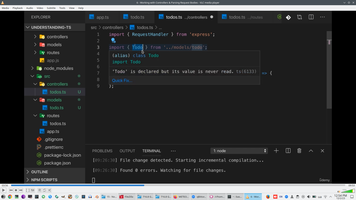
Declare TODOS array as asrray of models ToDo
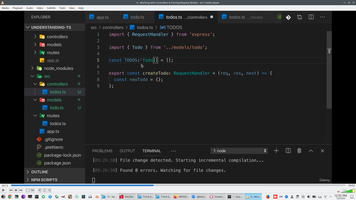
Initialize key of Array with Random ID, cast body as Text and Push new data to TODOS Array, we don't store anything to DB currently
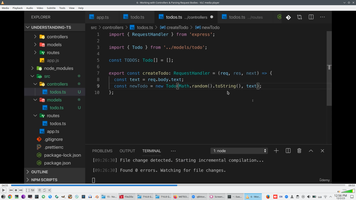
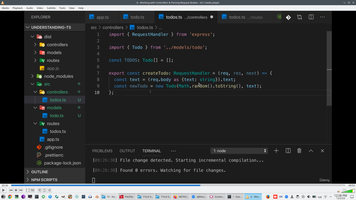
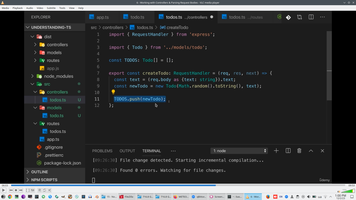
Import controller function to main App and pass reference to CreateToDo function to Post handler
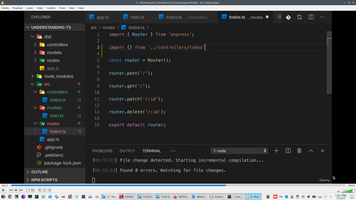
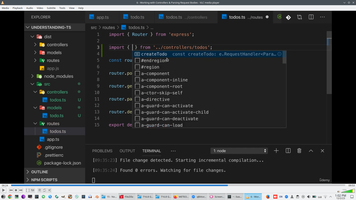
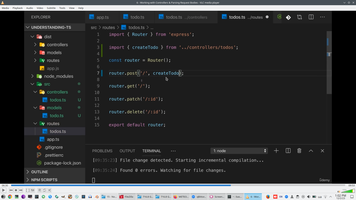
Not execute function with '()' only pass reference to it
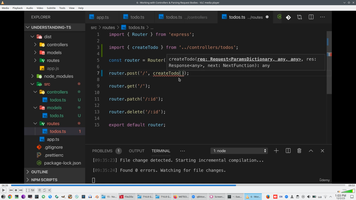
Create Response JSON on controller with Status code
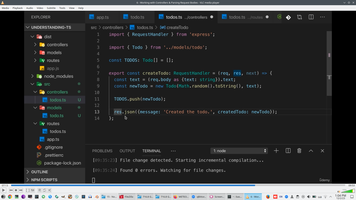
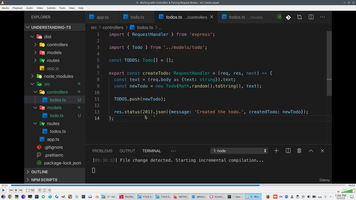
Import JSON processing function Body-Parser
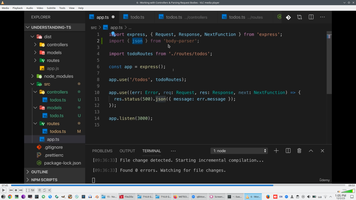
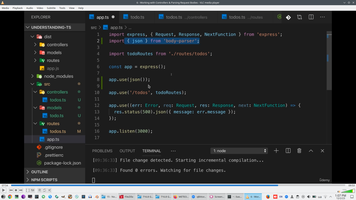
And register JSON as Middleware, Now JSON processor will working for any Request and extract Key from JSON Body
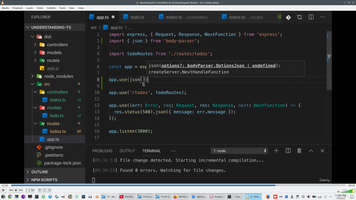
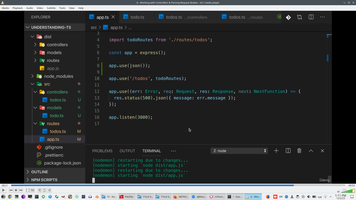
And currently we expect Text property on any JSON body on any our correct Request (Path Todos, port 3000).
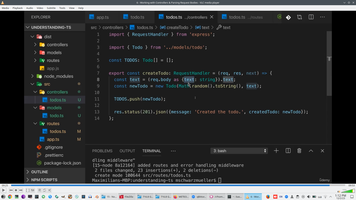
Check API and new ToDo created correctly.
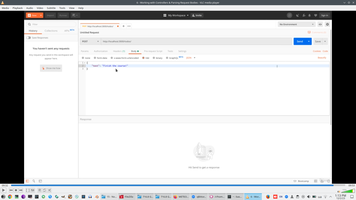
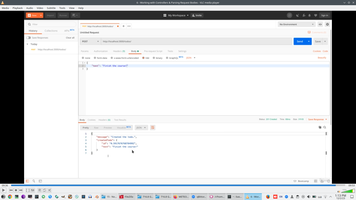
This is our current final JS code processed on Express server
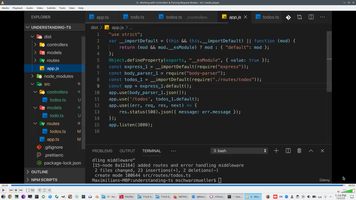
Create CRUD operation, next controller Entry Point is GetTodos and UpdateTodo
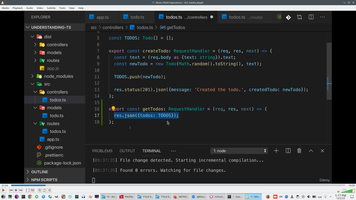
Import GetToDo and check.
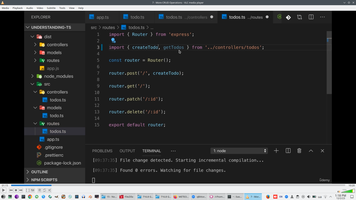
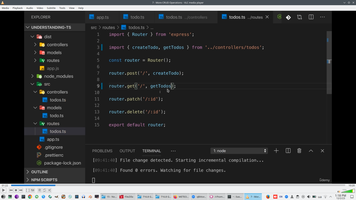
After code changing we have empty array because server restarted, but after restart all working fine
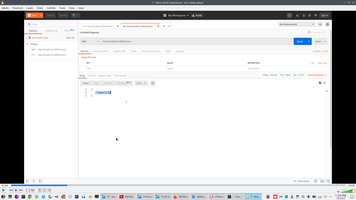
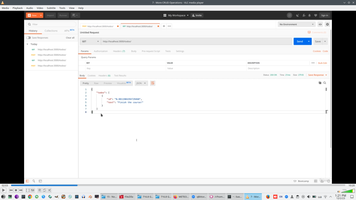
Create Delete method on Controller, ID is mandatory segment of URL
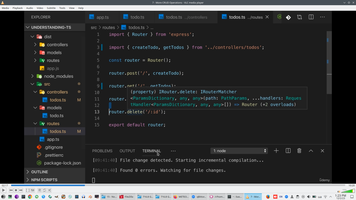
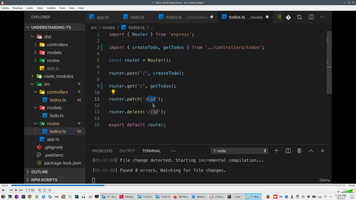
But TS don't know anything about mandatory URL segment
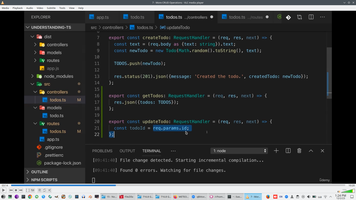
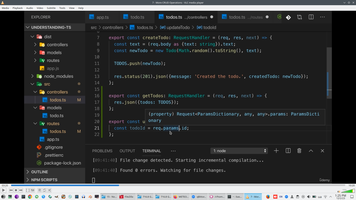
Therefore we need to define parameter type of RequestHandler by using Generic, what kind of body receive RequestHandler
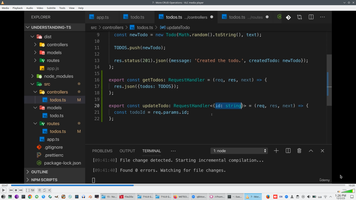
And now we can see that body contains exactly ID, exactly the key what we define on Route
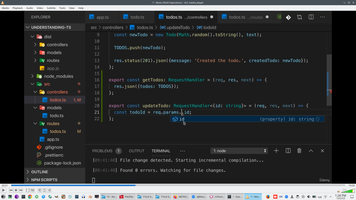
Extract value of Text property
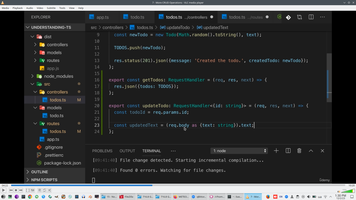
We need to create Predicate function to JS Filter with ID from URL as key
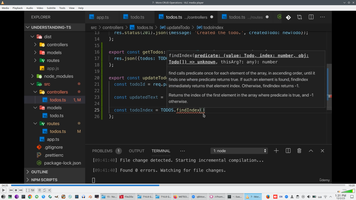
If expected Key is missing on Request we Throw Error And Error Handler will Catch this error
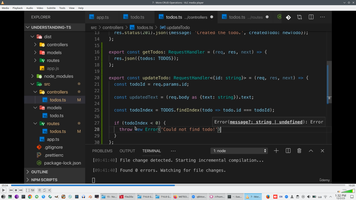
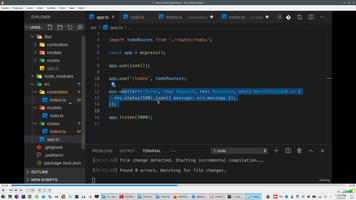
Convert Response body as JSON and pass reference to this function to Router
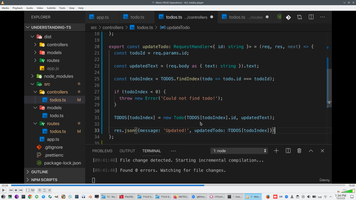
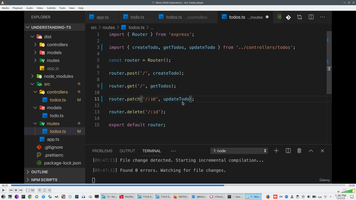
Check, All CRUD Api working fine!
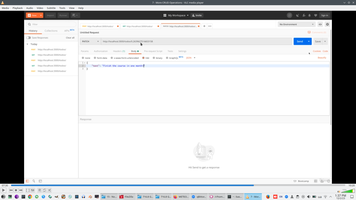
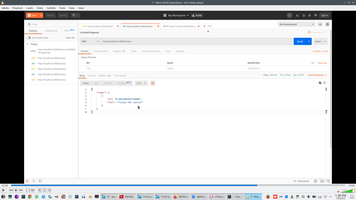
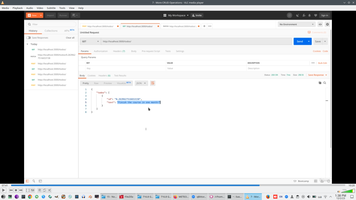
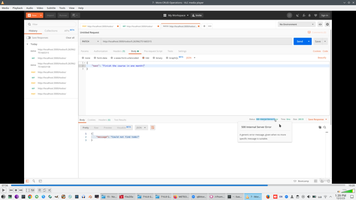
2. DigitalOcean
- Containerizing a Node.js Application for Development With Docker Compose(October 29, 2022)
- From Containers to Kubernetes with Node.js eBook(May 8, 2020)
- How To Code in Node.js eBook(December 14, 2020)
- How To Automate Your Node.js Production Deployments with Shipit on CentOS 7(February 26, 2020)
- How To Build a Concurrent Web Scraper with Puppeteer, Node.js, Docker, and Kubernetes(August 19, 2020)
- How To Build a Discord Bot with Node.js(January 18, 2022)
- How To Build a GraphQL Server in Node.js Using GraphQL-yoga and MongoDB(December 12, 2019)
- How To Build a Media Processing API in Node.js With Express and FFmpeg.wasm(October 16, 2021)
- How To Build a Media Processing API in Node.js With Express and FFmpeg.wasm(October 16, 2021)
- How To Build a Telegram Quotes Generator Bot With Node.js, Telegraf, Jimp, and Pexels(November 24, 2021)
- How To Create a Node.js Module(December 2, 2019)
- How To Create a Web Server in Node.js with the HTTP Module(April 10, 2020)
- How To Create an HTTP Client with Core HTTP in Node.js(October 7, 2020)
- How To Debug Node.js with the Built-In Debugger and Chrome DevTools(June 24, 2020)
- How To Develop a Node.js TCP Server Application using PM2 and Nginx on Ubuntu 16.04(July 24, 2018)
- How To Handle Asynchronous Tasks with Node.js and BullMQ(January 13, 2023)
- How To Improve Your Development Efficiency with Visual Studio Code(December 12, 2019)
- How To Install and Use the Yarn Package Manager for Node.js(August 10, 2021)
- How To Install Node.js on CentOS 8(April 1, 2020)
- How To Install Node.js on Debian 8(December 7, 2016)
- How To Install Node.js on Rocky Linux 8(August 2, 2022)
- How To Install Node.js on Ubuntu 20.04(January 23, 2023)
- How To Install Node.js on Ubuntu 22.04(April 28, 2022)
- How To Write and Run Your First Program in Node.js(March 18, 2022)
- How To Install the Deno JavaScript Runtime on Ubuntu 20.04(August 27, 2021)
- How To Integrate MongoDB with Your Node Application(February 14, 2019)
- How To Launch Child Processes in Node.js(July 31, 2020)
- How To Read and Write CSV Files in Node.js Using Node-CSV(April 22, 2022)
- How To Scale Node.js Applications with Clustering(February 10, 2023)
- How To Scrape a Website Using Node.js and Puppeteer(August 13, 2020)
- How To Secure MongoDB on Ubuntu 20.04(March 4, 2021)
- How To Set Up a GraphQL API Server in Node.js(June 13, 2022)
- How To Set Up a Node.js Application for Production on Ubuntu 20.04(March 31, 2021)
- How To Test a Node.js Module with Mocha and Assert(March 16, 2020)
- How To Upload a File to Object Storage with Node.js(November 24, 2017)
- How To Use EJS to Template Your Node Application(May 5, 2021)
- How To Use Multithreading in Node.js(August 5, 2022)
- How to Use Node.js and Github Webhooks to Keep Remote Projects in Sync(August 31, 2018)
- How To Use SQLite with Node.js on Ubuntu 22.04(October 19, 2022)
- How To Use the Node.js REPL(March 18, 2022)
- How To Use Visual Studio Code for Remote Development via the Remote-SSH Plugin(August 5, 2021)
- How To Use Vue.js Environment Modes with a Node.js Mock Data Layer(February 11, 2021)
- How To Work with Files Using Streams in Node.js(March 16, 2022)
- How To Work with Files using the fs Module in Node.js(September 9, 2020)
- How To Work With Zip Files in Node.js(December 16, 2021)
- How To Use Server-Side Rendering with Nuxt.js(February 16, 2022)
- How To Write Asynchronous Code in Node.js(February 5, 2020)
- How To Write End-to-End Tests in Node.js Using Puppeteer and Jest(March 15, 2022)
- How To Use node-cron to Run Scheduled Jobs in Node.js(December 1, 2021)
- Understanding the DOM Tree and Nodes(November 8, 2017)
- Using Buffers in Node.js(May 1, 2020)
- Using Event Emitters in Node.js(May 29, 2020)
- What is Node.js?(June 24, 2021)
3. EggHead
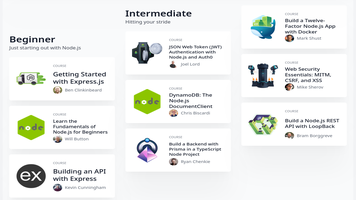
- Getting Started with Express.jsBen Clinkinbeard
- Learn the Fundamentals of Node.js for BeginnersWill Button
- Building an API with ExpressKevin Cunningham
- JSON Web Token (JWT) Authentication with Node.js and Auth0Joel Lord
- DynamoDB: The Node.js DocumentClientChris Biscardi
- Build a Backend with Prisma in a TypeScript Node ProjectRyan Chenkie
- Build a Twelve-Factor Node.js App with DockerMark Shust
- Web Security Essentials: MITM, CSRF, and XSSMike Sherov
- Build a Node.js REST API with LoopBackBram Borggreve
- Get Started with AI-Driven App Development Using the OpenAI Node.js SDKColby Fayock
- Build a REST API with Express 5 and node 14Jamund Ferguson
4. Related page:
- (2023) CloudflareWorker and Supabase
- (2022) NativeScript, AndroidBooks
- (2022) JS, Css
- (2022) Typescript, Webpack
- (2022) Angular, RxJs, Firebase, MongoDb
- (2022) Node, NestJs, Electron, Pwa, Telegram
- (2022) React, Redux, GraphQL, NextJs
- (2022) Angular/Typescript, JS books

<SITEMAP> <MVC> <ASP> <NET> <DATA> <KIOSK> <FLEX> <SQL> <NOTES> <LINUX> <MONO> <FREEWARE> <DOCS> <ENG> <CHAT ME> <ABOUT ME> < THANKS ME> |