<< Multi Languages Spell Checker for webmaster. Part 3. Directory observer on NET Reactive extension. <<
- Multi Languages Spell Checker for webmaster. Part 1. Install and tune NHunspell engine.
- Multi Languages Spell Checker for webmaster. Part 2. Main MDI-form.
- Multi Languages Spell Checker for webmaster. Part 3. Directory observer on NET Reactive extension.
- Multi Languages Spell Checker for webmaster. Part 4. TheArtOfDev.HtmlRenderer.
- Multi Languages Spell Checker for webmaster. Part 5. Multilang asynchronous spell checker with NHunspell.
- Multi Languages Spell Checker for webmaster. Part 6. TextEditor with highlighting, searching, line numbering and FTP-uploading.
- Multi Languages Spell Checker for webmaster. Part 7. Other project components.
Sorry, no time for more details description, only code.
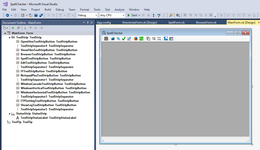
1: Imports System.Reactive.Concurrency
2: Imports System.Reactive.Linq
3: Imports System.Threading
4: Imports System.Collections.Generic
5: Imports System.ComponentModel
6: Imports System.Data
7: Imports System.Drawing
8: Imports System.Linq
9: Imports System.Text
10: Imports System.Windows.Forms
11: Imports System.IO
12:
13:
14: Partial Public Class DirectoriesForm
15:
16: Private Observer As IDisposable
17:
18: Private Directories As IObservable(Of String)
19:
20: Private BufferedDirectories As IObservable(Of IList(Of String))
21:
22: Private Sub DirectoriesForm_Load(sender As Object, e As EventArgs) Handles Me.Load
23: TreeViewDirectories.DrawMode = TreeViewDrawMode.OwnerDrawText
24: FolderBrowseStyleComboBox.SelectedIndex = 0
25: FolderBrowseTypeComboBox.SelectedIndex = 0
26: Me.butStop.Enabled = False
27: End Sub
28:
29:
30: Private Sub butOpen_Click(sender As Object, e As EventArgs) Handles butOpen.Click
31: Dim FB As New FolderBrowserDialog
32: FB.Description = "Select folder to observe"
33: If FB.ShowDialog = DialogResult.OK Then
34: Dim SyncContext = SynchronizationContext.Current
35: FolderBrowseStyleComboBox.Enabled = False
36: FolderBrowseTypeComboBox.Enabled = False
37: SearchPatternTextBox.Enabled = False
38: TreeViewDirectories.Nodes.Clear()
39: FileCount = 0
40: FolderCount = 0
41: If FolderBrowseStyleComboBox.Text = "Flat" Then
42:
43: ' Observe the enumeration of all directories using the winforms thread.
44: Directories = GetAllContents(FB.SelectedPath, SearchPatternTextBox.Text, FolderBrowseTypeComboBox.Text).
45: ToObservable(Scheduler.ThreadPool).ObserveOn(SyncContext)
46:
47: If Observer Is Nothing Then
48: ' Observe on the single directories.
49: Observer = Directories.Subscribe(AddressOf outputDirectory)
50: 'Observer = Directories.Finally(Sub() MsgBox("Finish"))
51: End If
52:
53: ElseIf FolderBrowseStyleComboBox.Text = "Tree" Then
54: ' Observe the enumeratino of all directories, but buffered in groups
55: ' of 1000 entries each second, and then observe that group on the winforms thread.
56:
57: BufferedDirectories = Observable.
58: Interval(TimeSpan.FromSeconds(1), Scheduler.ThreadPool).
59: Zip(
60: GetAllContents(FB.SelectedPath, SearchPatternTextBox.Text, FolderBrowseTypeComboBox.Text).
61: ToObservable(Scheduler.ThreadPool).
62: Buffer(1000),
63: Function(a, b) b
64: ).
65: ObserveOn(SyncContext)
66:
67: If Observer Is Nothing Then
68: ' observe on the buffered directories.
69: Observer = BufferedDirectories.Subscribe(AddressOf outputDirectories)
70: 'Dim Z As IList(Of String) = BufferedDirectories.Finally(Sub() MsgBox("Finish"))
71: End If
72: End If
73: butStop.Enabled = True
74: End If
75: End Sub
76:
77: ' Clear out the running observer
78: Private Sub butStop_Click(sender As Object, e As EventArgs) Handles butStop.Click
79: If Observer IsNot Nothing Then
80: Observer.Dispose()
81: Observer = Nothing
82: butStop.Enabled = False
83: FolderBrowseStyleComboBox.Enabled = True
84: FolderBrowseTypeComboBox.Enabled = True
85: SearchPatternTextBox.Enabled = True
86: End If
87: End Sub
88:
89: ' We check to see if the handle is created because when
90: ' the form is disposing this may still be trying to observe.
91: Private Sub outputDirectory(path As String)
92: If TreeViewDirectories.IsHandleCreated Then
93: TreeViewDirectories.Nodes.Add(path)
94: End If
95: End Sub
96:
97: Private TreeNodes As New Dictionary(Of String, TreeNode)()
98: ' We check to see if the handle is created because when
99: ' the form is disposing this may still be trying to observe.
100: Private Sub outputDirectories(paths As IEnumerable(Of String))
101: If TreeViewDirectories.IsHandleCreated Then
102: Try
103: TreeViewDirectories.BeginUpdate()
104: For Each path__1 In paths
105: Dim sb = New StringBuilder()
106: Dim pieces = path__1.Split(Path.DirectorySeparatorChar)
107: Dim parent As TreeNode = Nothing
108: For i As Integer = 0 To pieces.Length - 1
109: Dim child As TreeNode = Nothing
110: sb.Append(pieces(i))
111: sb.Append(Path.DirectorySeparatorChar)
112: If Not TreeNodes.TryGetValue(sb.ToString(), child) Then
113: If parent IsNot Nothing Then
114: child = parent.Nodes.Add(pieces(i))
115: Else
116: child = TreeViewDirectories.Nodes.Add(pieces(i))
117: End If
118: TreeNodes(sb.ToString()) = child
119: End If
120: parent = child
121: Next
122: Next
123: Finally
124: TreeViewDirectories.EndUpdate()
125: End Try
126: End If
127: End Sub
128:
129: Shared FileCount As Integer
130: Shared FolderCount As Integer
131: 'This is another thread than form
132: Private Iterator Function GetAllContents(path As String, SearchPattern As String, SearchType As String) As IEnumerable(Of String)
133: If SearchType <> "FolderOnly" Then
134: Dim Files As String() = Nothing
135: Try
136: Files = Directory.GetFiles(path, SearchPattern, SearchOption.TopDirectoryOnly)
137: Catch generatedExceptionName As IOException
138: Catch generatedExceptionName As UnauthorizedAccessException
139: End Try
140: If Files IsNot Nothing Then
141: For Each One In Files
142: FileCount += 1
143: If FileCount Mod 10 = 0 Then UpdateFileLabel(FileCount.ToString)
144: Yield One
145: Next
146: End If
147: End If
148:
149: Dim Subdirs As String() = Nothing
150: Try
151: Subdirs = Directory.GetDirectories(path)
152: Catch generatedExceptionName As IOException
153: Catch generatedExceptionName As UnauthorizedAccessException
154: End Try
155: If Subdirs IsNot Nothing Then
156: For Each subdir In Subdirs
157: Debug.Print(subdir.ToString)
158: FolderCount += 1
159: If FolderCount Mod 10 = 0 Then UpdateFolderLabel(FolderCount.ToString)
160: Yield subdir
161: For Each grandchild In GetAllContents(subdir, SearchPattern, SearchType)
162: FolderCount += 1
163: If FolderCount Mod 10 = 0 Then UpdateFolderLabel(FolderCount.ToString)
164: Debug.Print(grandchild.ToString)
165: Yield grandchild
166: Next
167: Next
168: End If
169: Debug.Print("Finish " & path)
170: End Function
171:
172: Public Sub UpdateFolderLabel(ProgressTXT As String)
173: InvokeOnUiThreadIfRequired(Sub() FolderCountLabel.Text = ProgressTXT)
174: InvokeOnUiThreadIfRequired(Sub() StatusStrip1.Refresh())
175: End Sub
176:
177: Public Sub UpdateFileLabel(ProgressTXT As String)
178: InvokeOnUiThreadIfRequired(Sub() FileCountLabel.Text = ProgressTXT)
179: InvokeOnUiThreadIfRequired(Sub() StatusStrip1.Refresh())
180: End Sub
181:
182: Dim SelectedNode As TreeNode
183: Private Sub treeViewDirectories_AfterSelect(sender As Object, e As TreeViewEventArgs) Handles TreeViewDirectories.AfterSelect
184: If SelectedNode Is Nothing Then
185: SelectedNode = TreeViewDirectories.TopNode
186: TreeViewDirectories.SelectedNode = TreeViewDirectories.TopNode
187: Else
188: SelectedNode = TreeViewDirectories.SelectedNode
189: End If
190: If FolderBrowseStyleComboBox.Text <> "Flat" Then
191: TreeViewDirectories.SelectedNode.Expand()
192: End If
193: Dim X As MainForm = ParentForm
194: X.SetNewFile(e.Node.FullPath)
195: End Sub
196:
197: Private Sub UpToolStripButton_Click(sender As Object, e As EventArgs) Handles UpToolStripButton.Click
198: If TreeViewDirectories.SelectedNode Is Nothing Then
199: SelectedNode = TreeViewDirectories.TopNode
200: TreeViewDirectories.SelectedNode = TreeViewDirectories.TopNode
201: TreeViewDirectories.SelectedNode.Expand()
202: End If
203: TreeViewDirectories.SelectedNode = TreeViewDirectories.SelectedNode.PrevVisibleNode
204: End Sub
205:
206: Private Sub DownToolStripButton_Click(sender As Object, e As EventArgs) Handles DownToolStripButton.Click
207: If TreeViewDirectories.SelectedNode Is Nothing Then
208: SelectedNode = TreeViewDirectories.TopNode
209: TreeViewDirectories.SelectedNode = TreeViewDirectories.TopNode
210: TreeViewDirectories.SelectedNode.Expand()
211: End If
212: TreeViewDirectories.SelectedNode = TreeViewDirectories.SelectedNode.NextVisibleNode
213: End Sub
214:
215: Dim FilesTxt As List(Of String)
216: Private Sub LoadFileListToolStripButton_Click(sender As Object, e As EventArgs) Handles LoadFileListToolStripButton.Click
217: Application.DoEvents()
218: Dim X As New OpenFileDialog
219: X.Title = "Browse Text Files"
220: X.DefaultExt = "txt"
221: X.Filter = "xml files (*.xml)|*.xml|All files (*.*)|*.*"
222: X.CheckFileExists = True
223: X.CheckPathExists = True
224: X.FileName = "E:\vb-net\sitemap.xml"
225: If X.ShowDialog = DialogResult.OK Then
226: FolderBrowseStyleComboBox.Text = "Flat"
227: FilesTxt = File.ReadLines(X.FileName).ToList
228: FileCountLabel.Text = FilesTxt.Count - 1
229: FolderBrowseStyleComboBox.Enabled = False
230: FolderBrowseTypeComboBox.Enabled = False
231: SearchPatternTextBox.Enabled = False
232: TreeViewDirectories.Nodes.Clear()
233: FileCount = 0
234: FolderCount = 0
235: Dim FileName As String
236: Dim Match As RegularExpressions.Match
237: For i As Integer = 0 To FilesTxt.Count - 1
238: Match = RegularExpressions.Regex.Match(FilesTxt(i), "<url><loc>.+</loc></url>")
239: If Match.Success Then
240: FileName = FilesTxt(i).Replace("<url><loc>", "").Replace("</loc></url>", "")
241: FileName = FileName.Replace("//www.vb-net.com/", "E:\vb-net\")
242: FileName = FileName.Replace("/", "\")
243: outputDirectory(FileName)
244: End If
245: Next
246: End If
247: End Sub
248:
249: Private Sub TreeViewDirectories_DrawNode(sender As Object, e As DrawTreeNodeEventArgs) Handles TreeViewDirectories.DrawNode
250: Dim TW As TreeView = CType(sender, TreeView)
251: If TW.SelectedNode Is Nothing Then
252: e.Graphics.DrawString(e.Node.FullPath, Me.Font, Brushes.Black, e.Bounds.X, e.Bounds.Y)
253: Else
254: If e.Node Is TW.SelectedNode Then
255: e.Graphics.DrawString(e.Node.FullPath, Me.Font, Brushes.Red, e.Bounds.X, e.Bounds.Y)
256: Else
257: e.Graphics.DrawString(e.Node.FullPath, Me.Font, Brushes.Black, e.Bounds.X, e.Bounds.Y)
258: End If
259:
260: End If
261: End Sub
262: End Class
Comments (
)

Link to this page:
//www.vb-net.com/SpellChecker/DirectoryObserver.htm
|