(<< Back <<) Typescript Examples (<< Back <<)
9. Drag-and-drop example
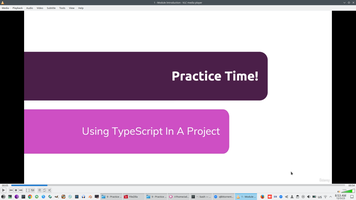
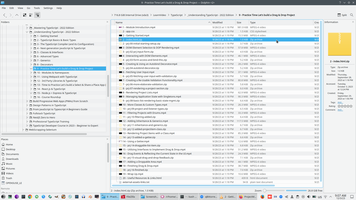
1 - Module Introduction.mp4 2 - Getting Started.mp4 3 - DOM Element Selection & OOP Rendering.mp4 4 - Interacting with DOM Elements.mp4 5 - Creating & Using an Autobind Decorator.mp4 6 - Fetching User Input.mp4 7 - Creating a Re-Usable Validation Functionality.mp4 8 - Rendering Project Lists.mp4 9 - Managing Application State with Singletons.mp4 10 - More Classes & Custom Types.mp4 11 - Filtering Projects with Enums.mp4 12 - Adding Inheritance & Generics.mp4 13 - Rendering Project Items with a Class.mp4 14 - Using a Getter.mp4 15 - Utilizing Interfaces to Implement Drag & Drop.mp4 16 - Drag Events & Reflecting the Current State in the UI.mp4 17 - Adding a Droppable Area.mp4 18 - Finishing Drag & Drop.mp4 19 - Wrap Up.mp4
Start from empty HTML and empty TS, for the moment al code will be stored to one file, splitting code to modules is next lecture
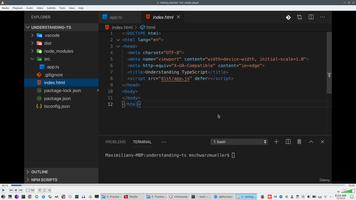
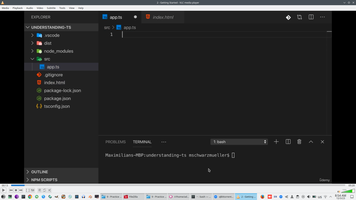
Replace Html ad CSS, we will use shadow DOM, Using templates and slots
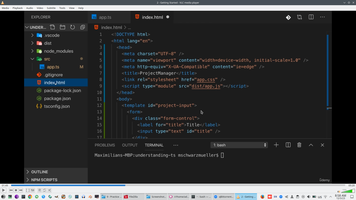
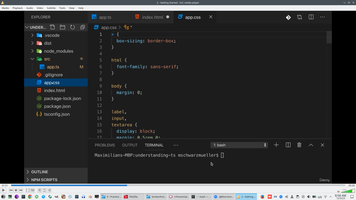
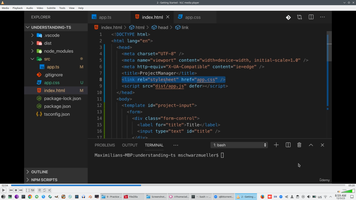
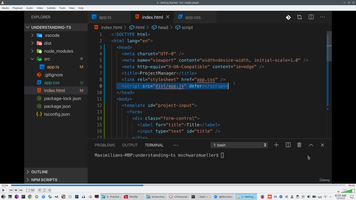
Modern browser understand <template> html tag, browser don;t show it but tag can reach from JS https://developer.mozilla.org/en-US/docs/Web/HTML/Element/template
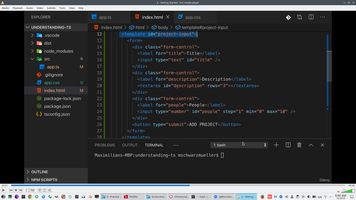
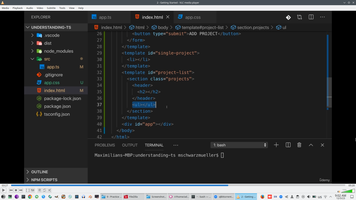
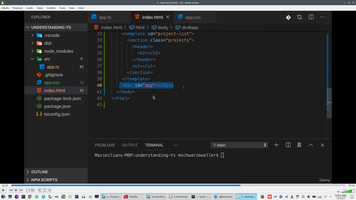
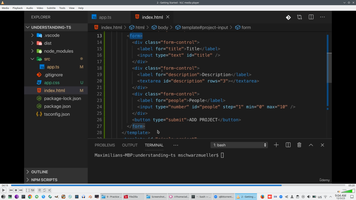
So, we will use 3 templates on this project - project-input, single-project, project-list
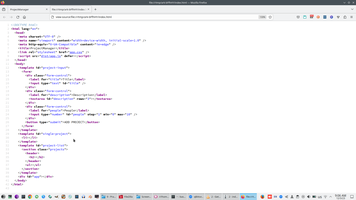
On the first Bash we do "NPM START" in another "TSC -W", browser will read port 3001 and TS compiler will track changing source code
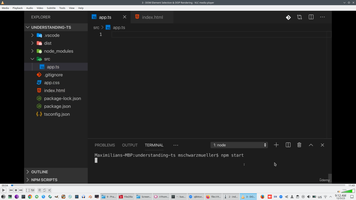
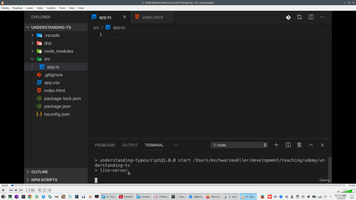
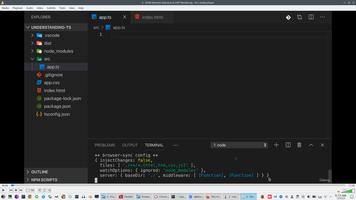
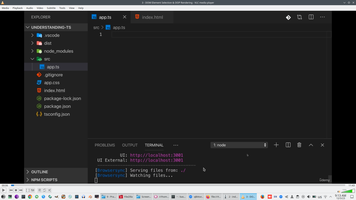
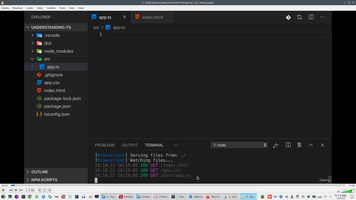
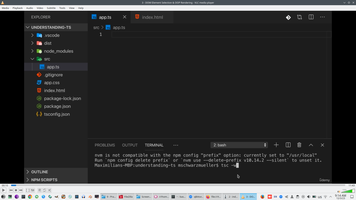
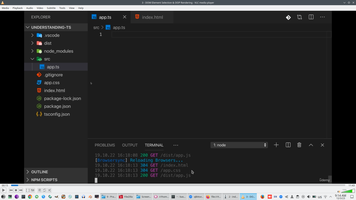
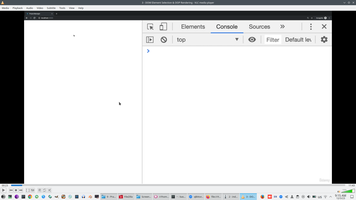
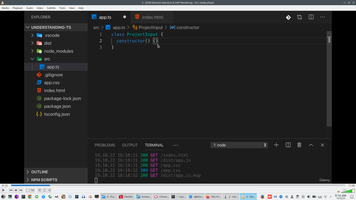
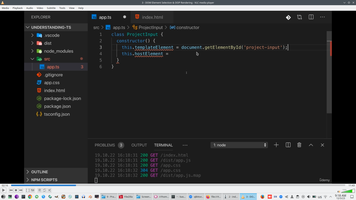
HtmlTemplate element globally available, because DOM library connected
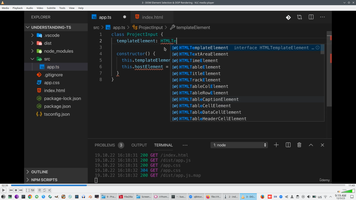
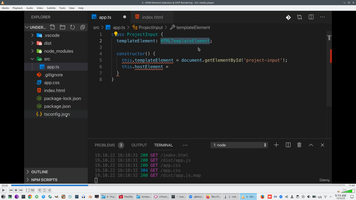
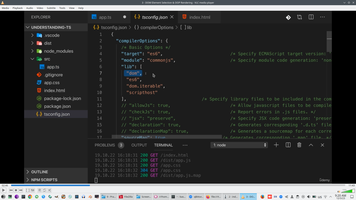
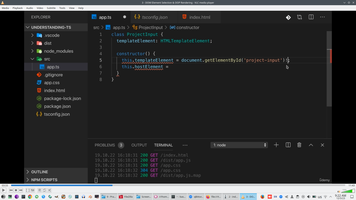
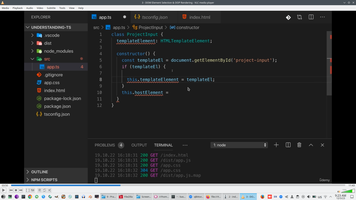
Use explamation mark
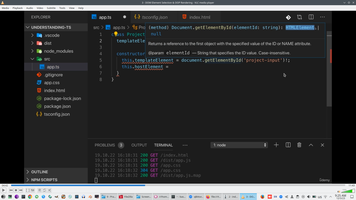
Casting to HtmlTemplateElement
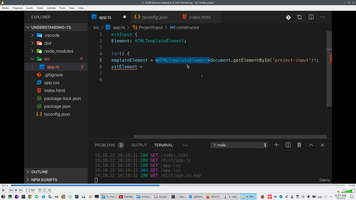
Alternative second syntax of casting is 'AS'
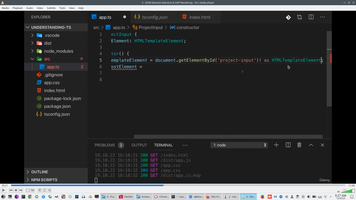
Idea is when we instantiated this class, immediately rendered <template> to <div>
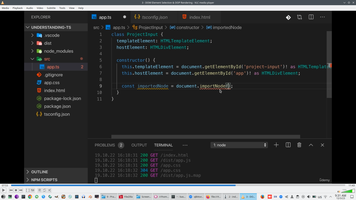
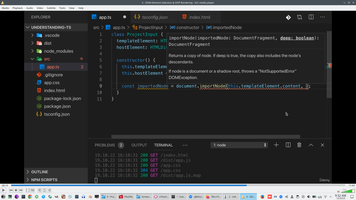
So, now we have imported full document fragment structure
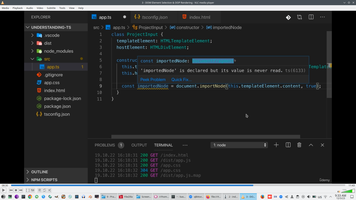
Atach will contains rendering logic
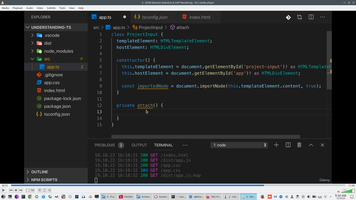
Document.InsertAdjacent method provided by Browser
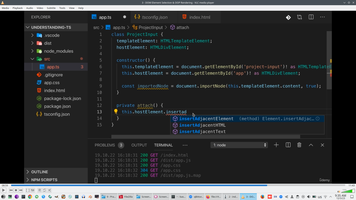
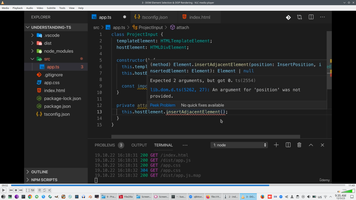
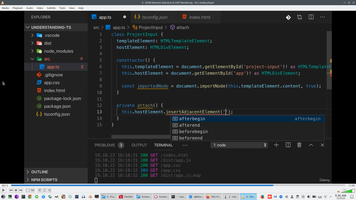
But firstly we need to reach <form> element
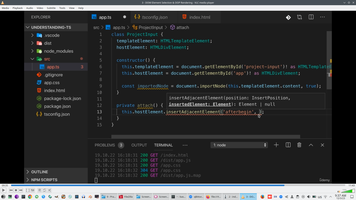
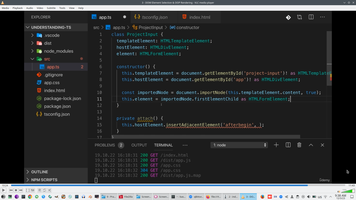
And attach 7lt;form> element to <div> (host element)
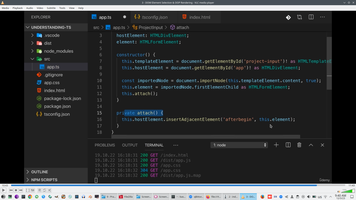
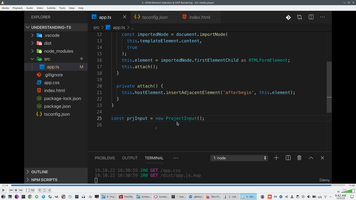
Try how form will be attached from <template> and rendered
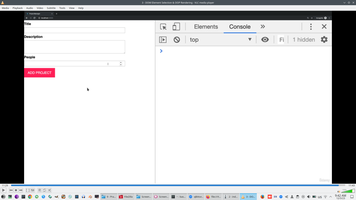
We have a style
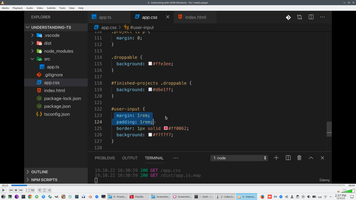
before we attach element to DOM we will set element ID to bind it to style
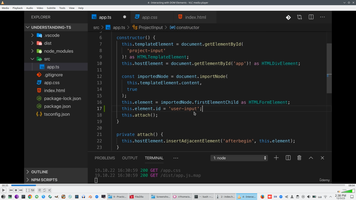
Now site looks match better
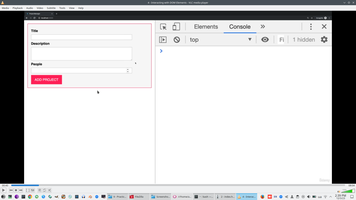
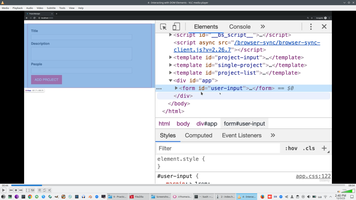
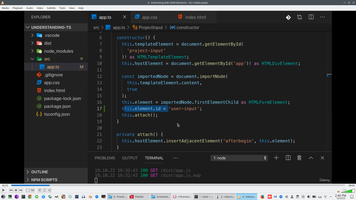
Declare 3 new variable
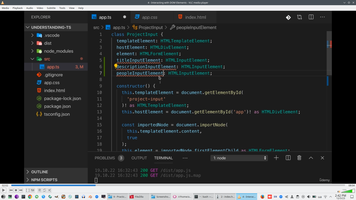
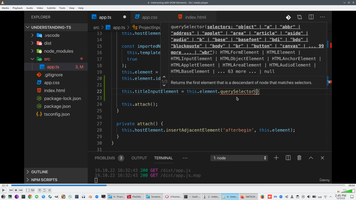
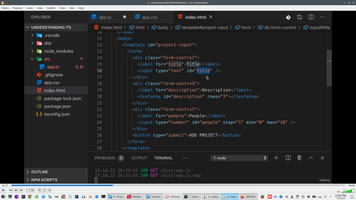
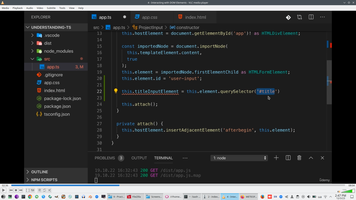
And provide access to user input
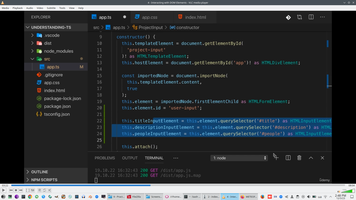
Add EventHandler to form submit
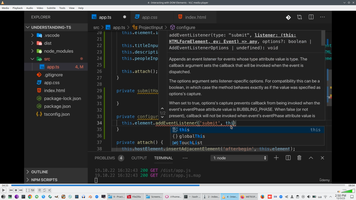
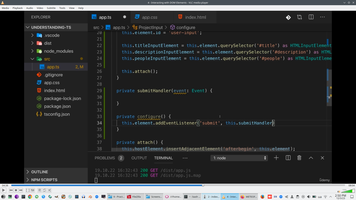
parameter event:Event on Eventhandler
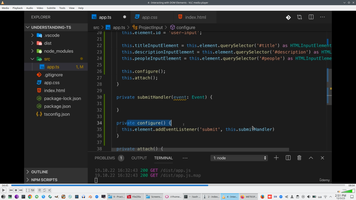
PreventDefault firstly, to prevent browser submission HttpRequest to server
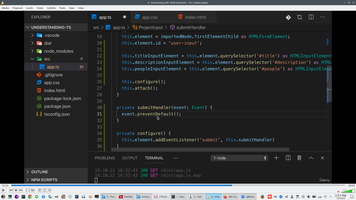
This cause because This is different on AddEventListeners and class
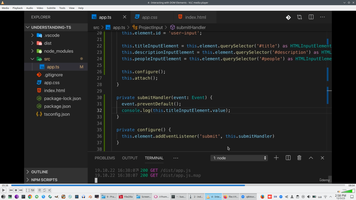
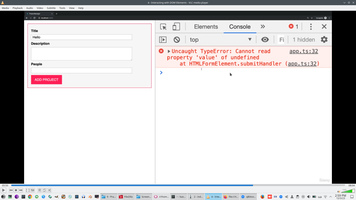
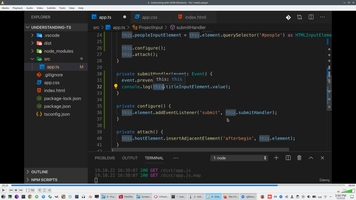
Therefore we need to use bind(), we need pass this from addEventListener to submitHandler method of ProjectInput class, bind() is vanilla JS method.
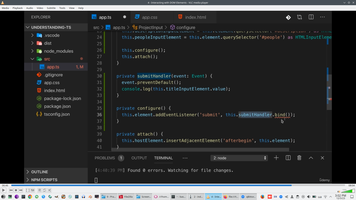
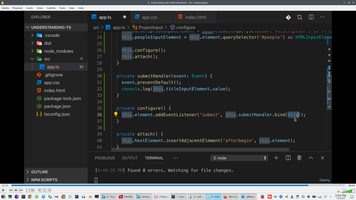
And currently all working fine, we have access in submitHandler to user input
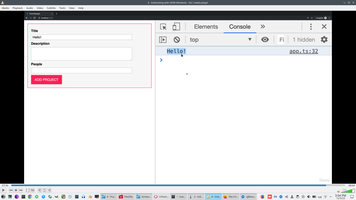
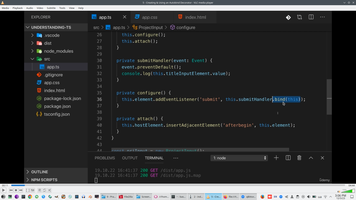
We will create AutoBind decorator to all project
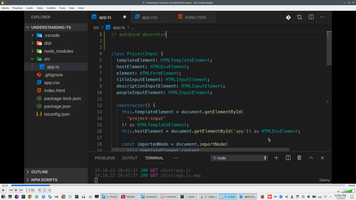
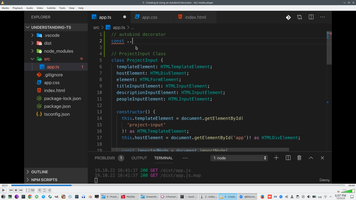
Decorator interface for method
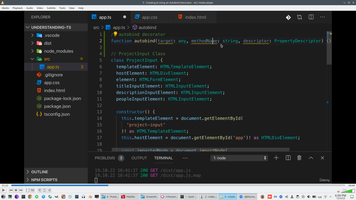
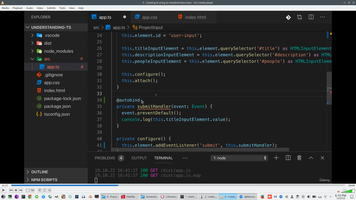
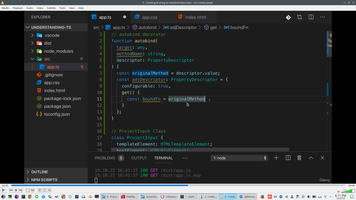
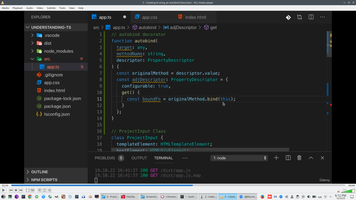
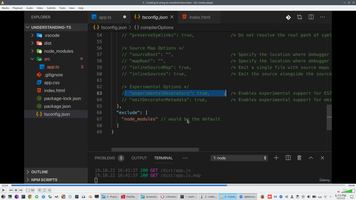
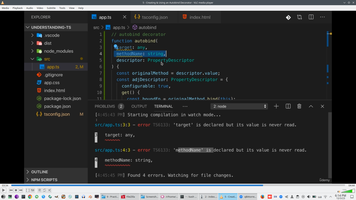
But firstly turn on decorator future on TS config
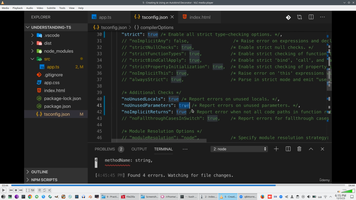
Or delete TS error "this parameter never used"
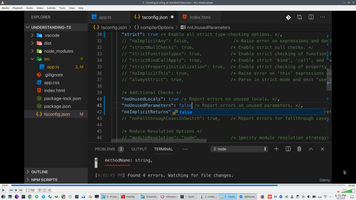
This two variable never used, musk it with underscore "_"
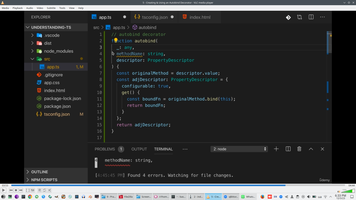
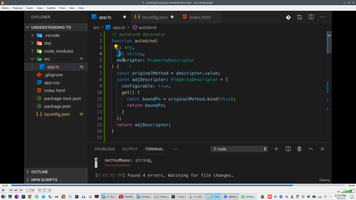
Now we replace implicit bind to decorator
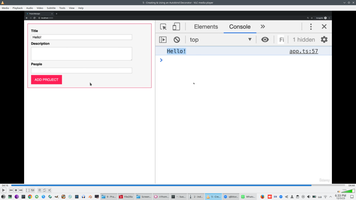
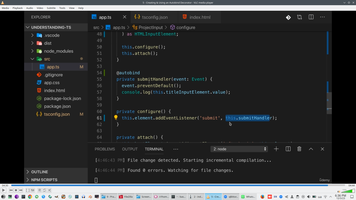
gatherUserInput will processing input after submit
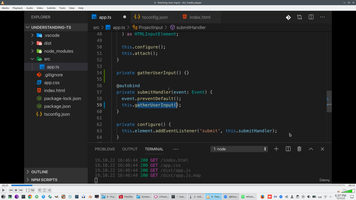
Function should return a Tuple with all 3 user input value
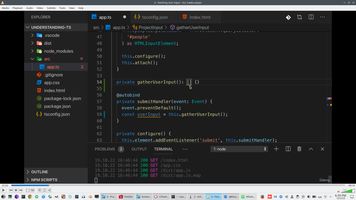
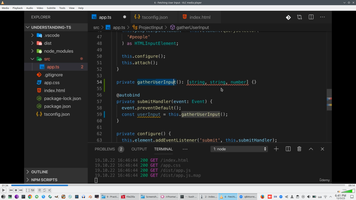
Trivial way is checking for non-empty value, but we need more smart and scalable solution
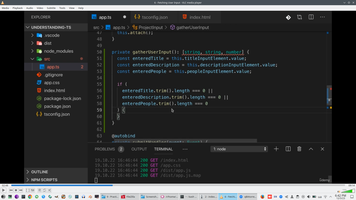
Convert string to number with ParseFloat or '+'
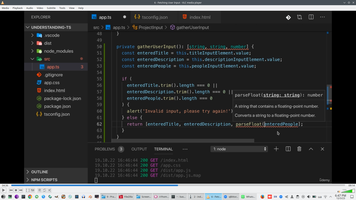
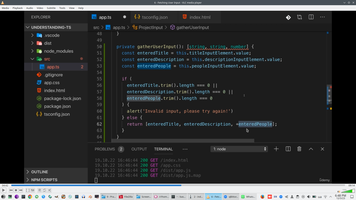
How to fix wrong input branch, we have no correct Tuple for return
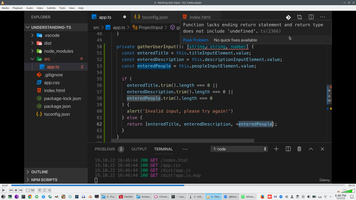
return; get undefined as function result
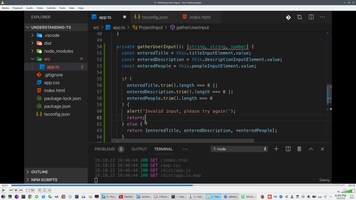
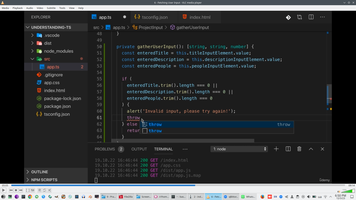
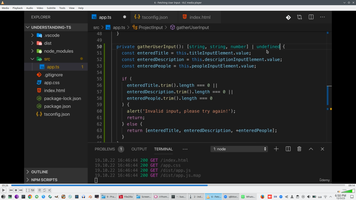
Or we need to set Void instead undefined
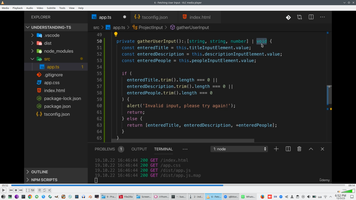
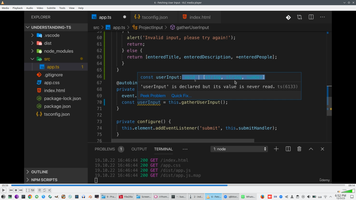
Can we check function result as InstanceOf?
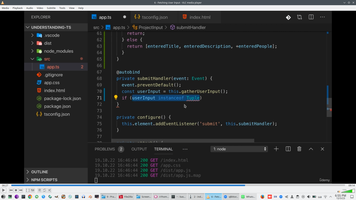
On runtime we have no way to check Tuple, because JS known nothing about Tuple, for JS level Tuple just simple Array
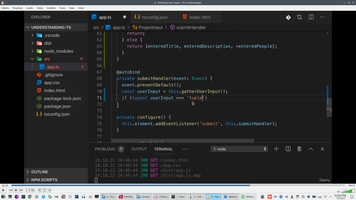
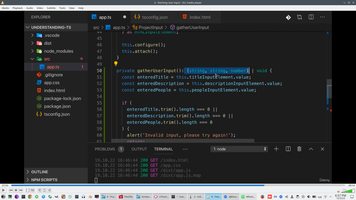
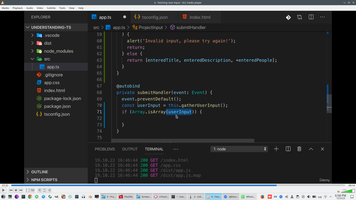
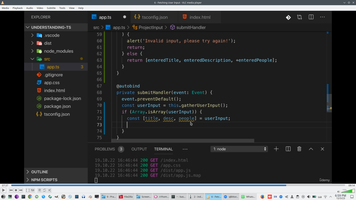
So, checking void or correct result of user input will be Array.IsArray
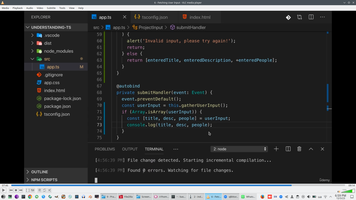
And check how function gatherUserInput return correct Tuple
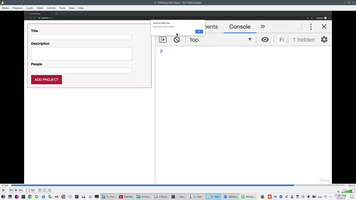
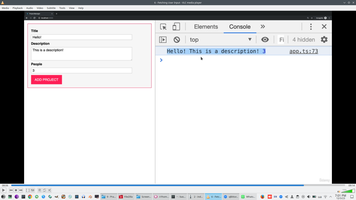
Destructive assignment tuple to to 3 variables
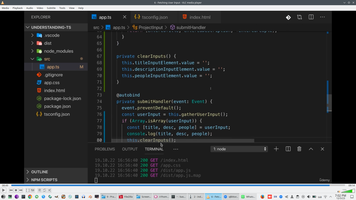
Invalid input with '===' and '||' now will replace to call validator
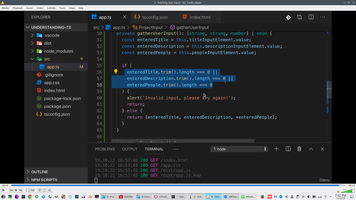
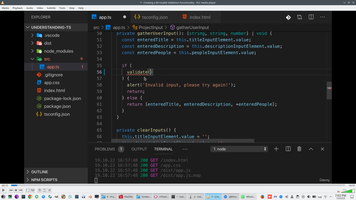
or combine of 3 validate functions with '&&'
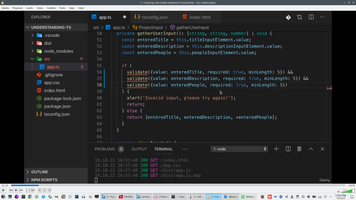
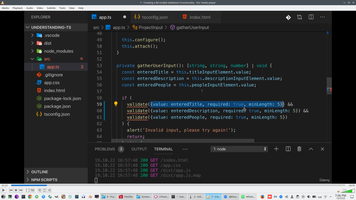
Interface of universal validator parameters
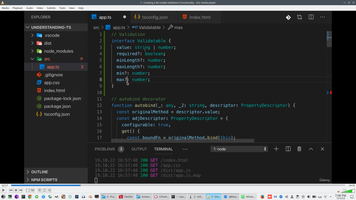
Define optional parameter by unity type with '| undefined' or by mark parameter name with question mark '?'
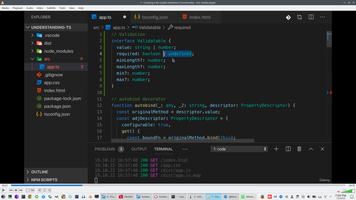
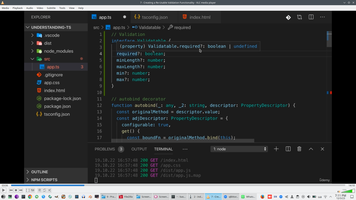
Pass object with interface Validatable to function Validate
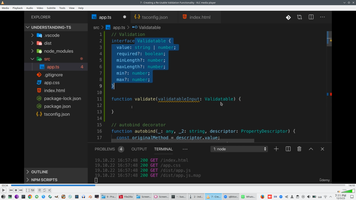
Validation logic - we combine result with '&&'
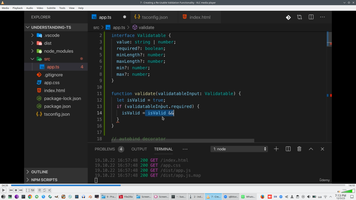
Problem that don't sure what exactly we need to validate - string or number, in this case we need to use TypeGuard
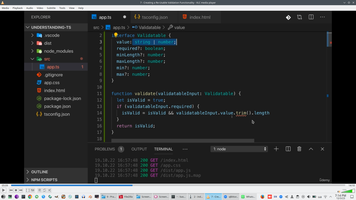
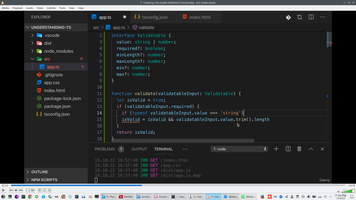
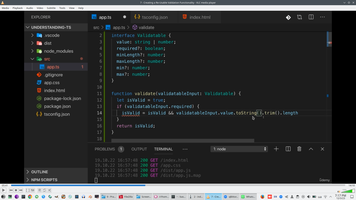
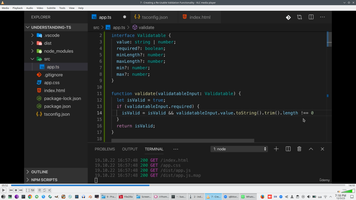
Main developer mistakes - empty string (if length is zero) != null and != undefined
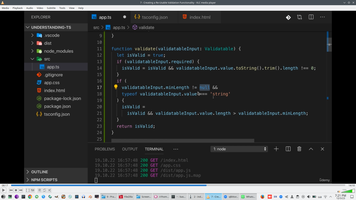
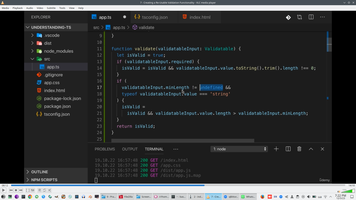
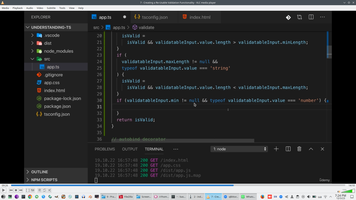
Testing validation - construct validation object and pass it as parameter to validation function, combine Validation with '&&' or with ! and '||'
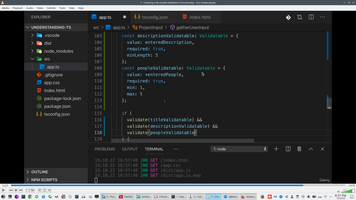
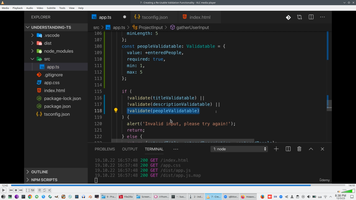
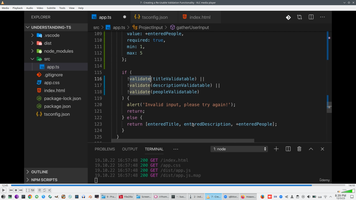
For number Html5 allow set min and max numbers
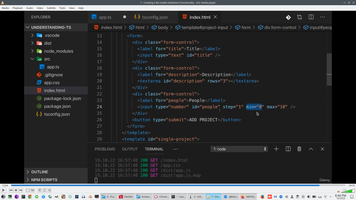
Currently Submit handler doing nothing, only console.log
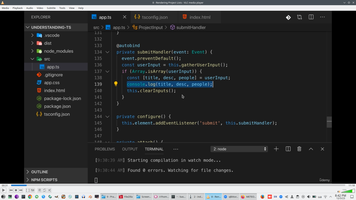
But we need to render ProjectList, Section element is common Html-element supported by Browser - https://developer.mozilla.org/en-US/docs/Web/HTML/Element/section
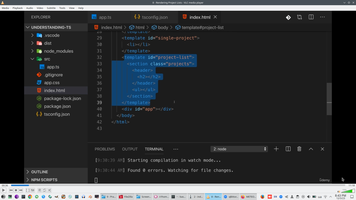
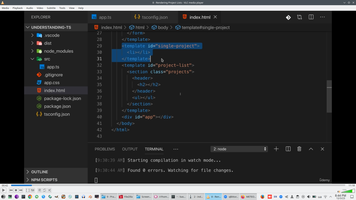
So, start class ProjectList, what will support rendering list
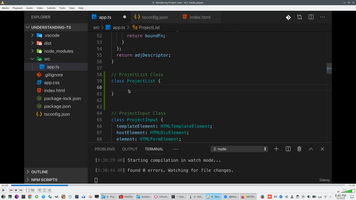
We can access to all needed HtmlElement
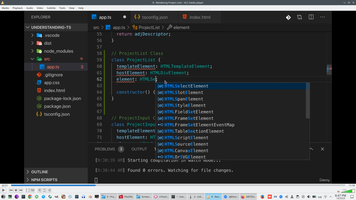
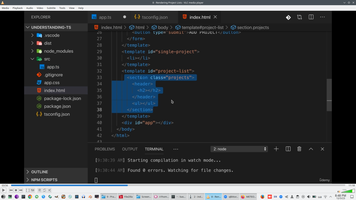
And add to constructor one parameter - with literal type active/finished and autoDeclaration
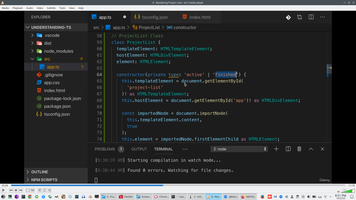
Dynamic element ID
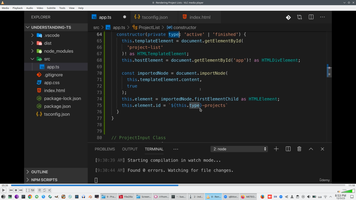
Attach method will be rendered this list to the DOM
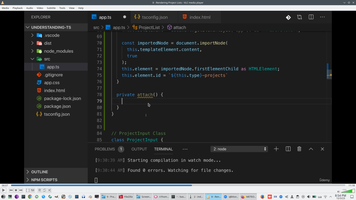
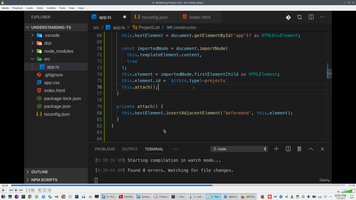
RenderContent will fill <h2> and <ul> tags
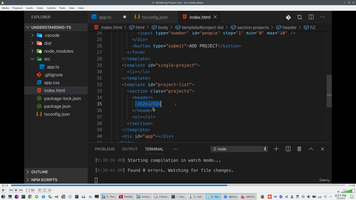
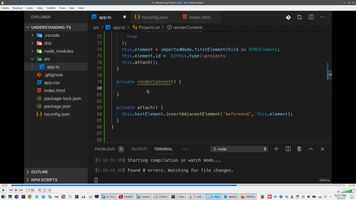
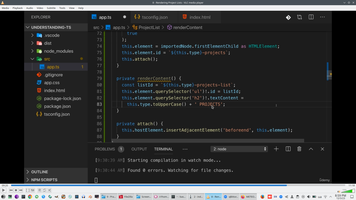
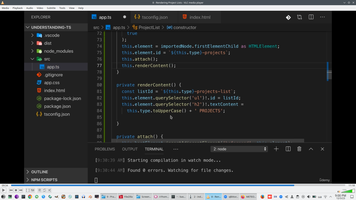
Instantiate project list
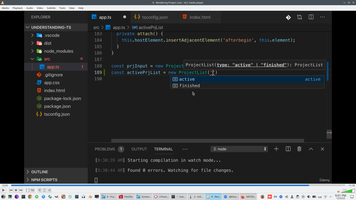
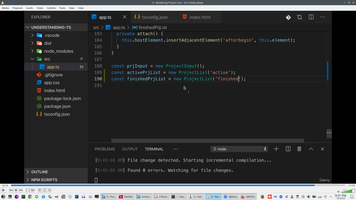
And checking how project list rendering from template to DOM
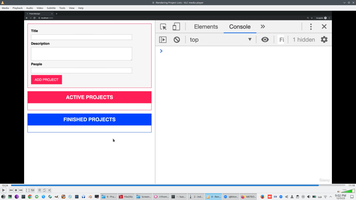
In this place we need to create new project section and attach project section to active Tab
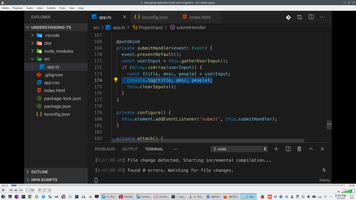
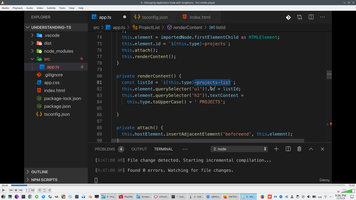
Trivial idea is Get Active Tab and add new project to ActiveTab
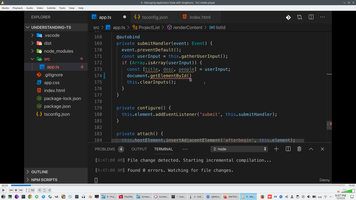
But we create more powerful code, we create method AddProject
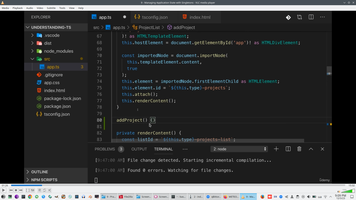
We need to pass reference for ProjectInput to constructor and call AddProject on the instance
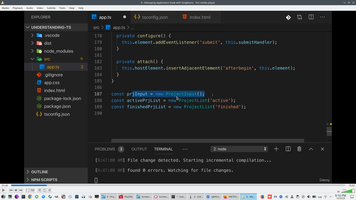
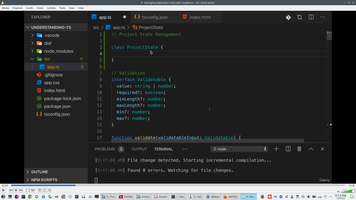
This will be Global Project state, usually we used this way on Angular or Redux, at start point this is Array of Any, this is not final point.
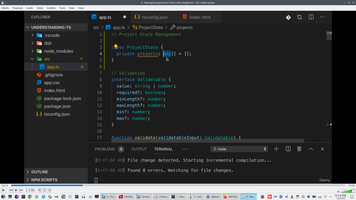
AddProject is public method, because there are no qualificator
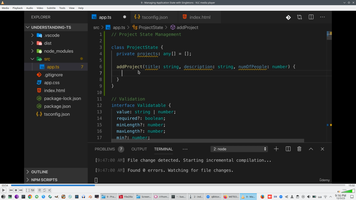
And push new project to teh ProjectState object
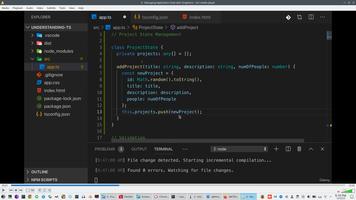
Create private constructor and static Instance variable, and GeInstance method, because ProjectStaite state is Singleton class
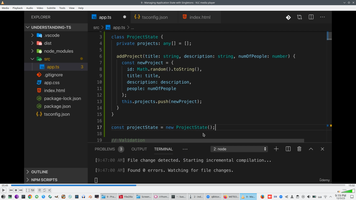
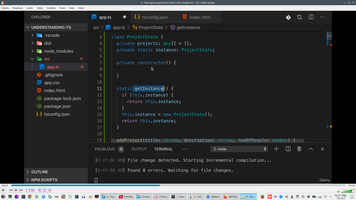
And call project state with NewProject
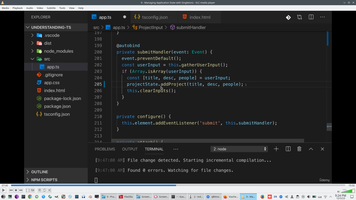
Create Listeners class, Project state need to collecting Listeners associated with each project
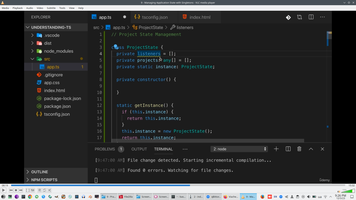
Listener should be a pointer to function
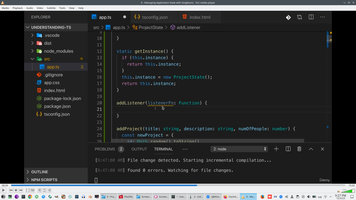
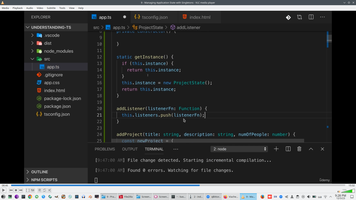
Idea is execute Listeners as function
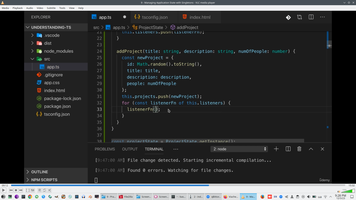
Slice() create copy of Array
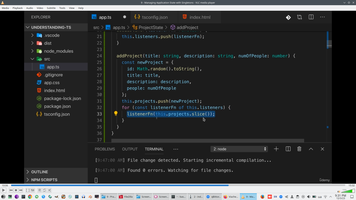
We want to pass listeners (if project state changed), this will be callback (func on VB)
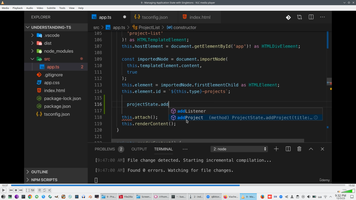
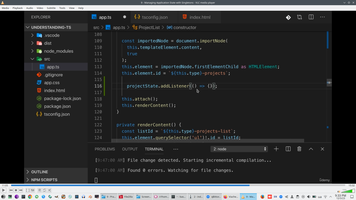
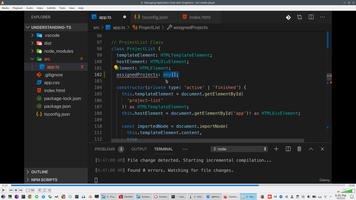
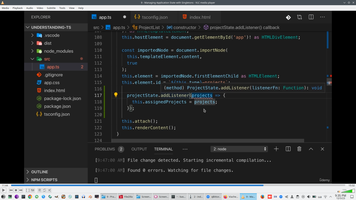
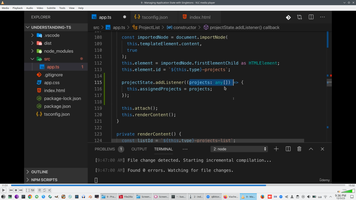
Add new <li> to <ul>
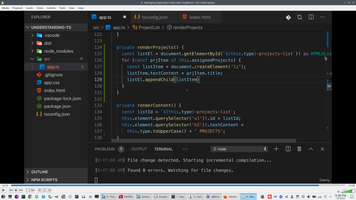
Currently new project added to both list (new and finished)
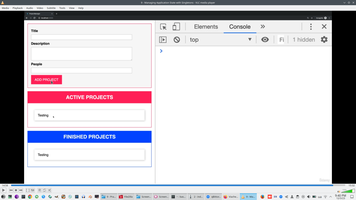
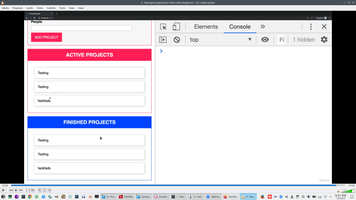
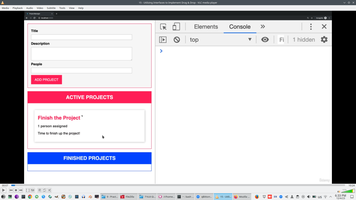
Full code huge, part of lecture skipped
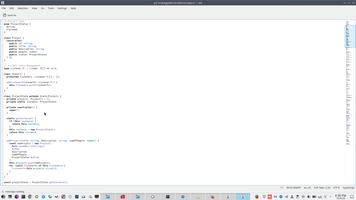
Visual update and change project status from Active to Finished
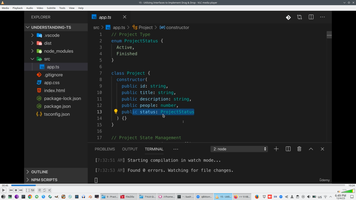
Start create two interface - Draggable and DragTarget
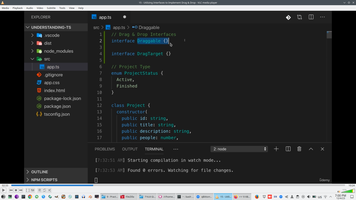
This interface will be add to any class what can be draggable - ProjectItem class
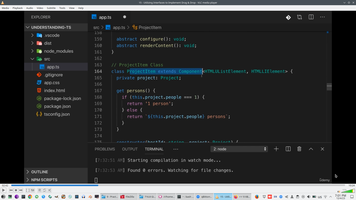
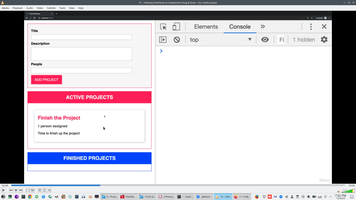
And the boxes class what save draggable element
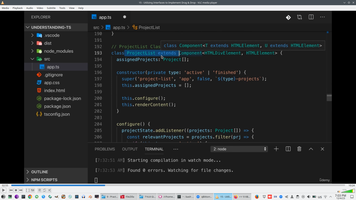
Draggable interface need to implement two handlers - DragStartHandler and DragEndHandler
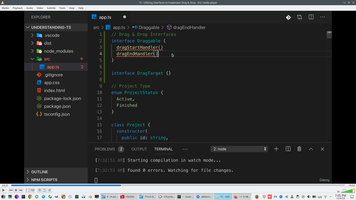
DragTarget interface need to implement 3 handler - DragOverHandler, DropHandler, DragLeaveHandler
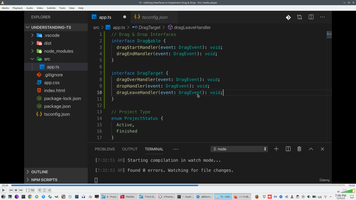
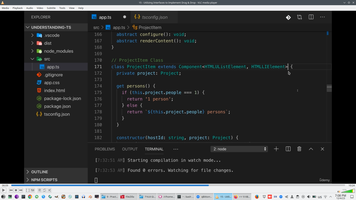
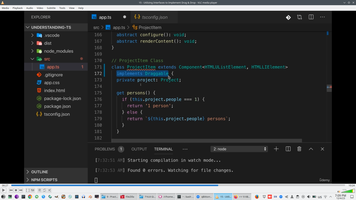
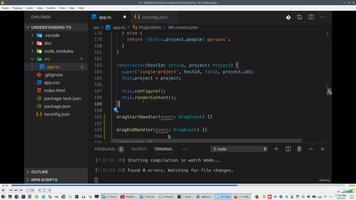
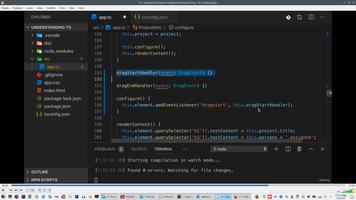
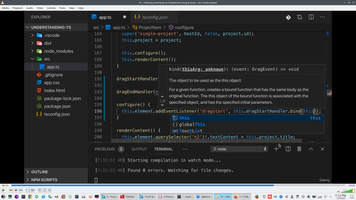
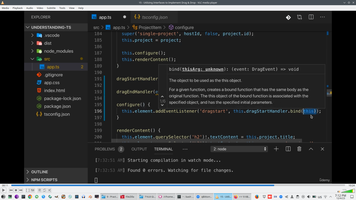
Bind this handler with Decorator @AutoBind
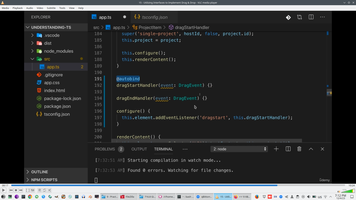
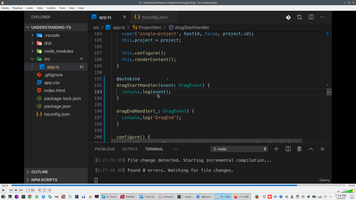
Without draggable attribute on Html drag-and-drop don't working
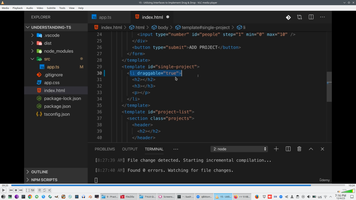
And now item can draggable, we can see coordinates
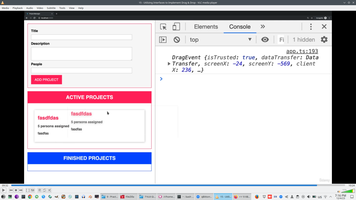
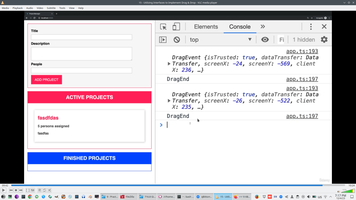
Change style for more visible draggable element
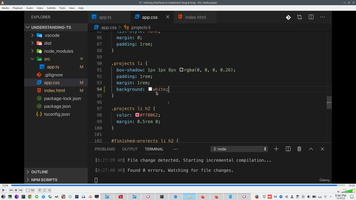
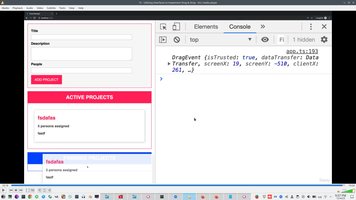
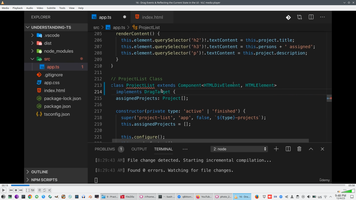
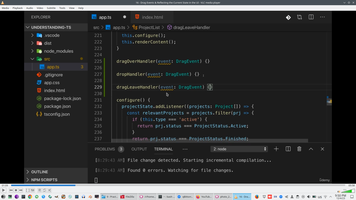
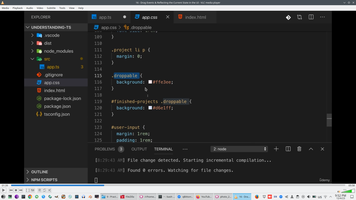
Add style Droppable to <ul>
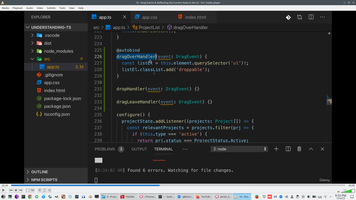
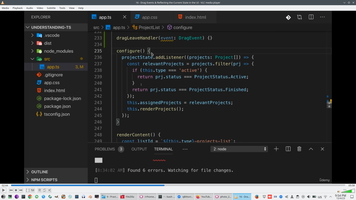
All JS Drag events
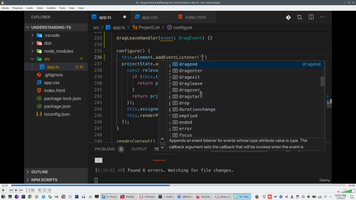
Implement other handlers
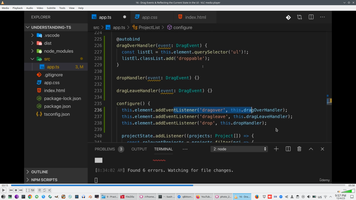
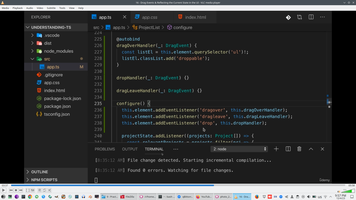
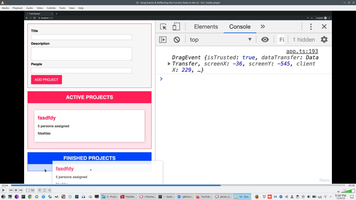
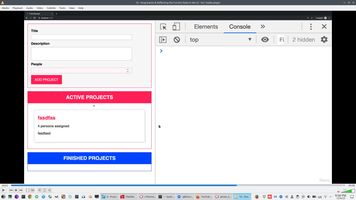
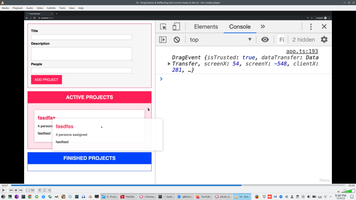
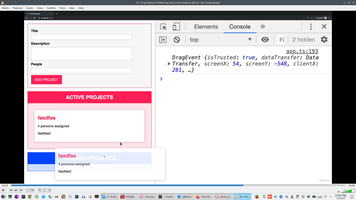
And remove style Droppable from <ul> when drag-and-drop finished
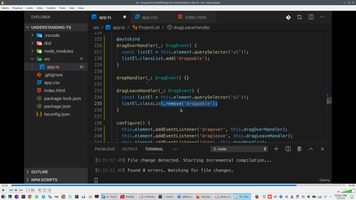
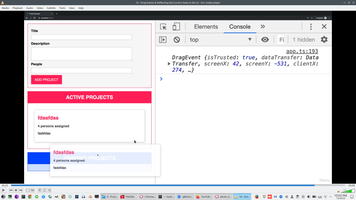
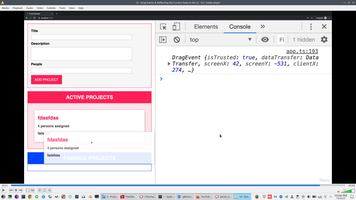
13. Google Map example + Axios HttpRequest library
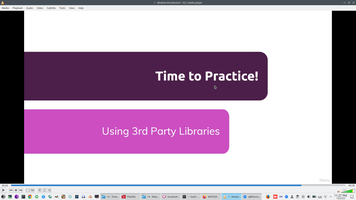
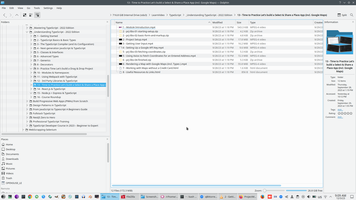
Project setup, we want receive search string from user input and than pass string to Google Map and receive GeoCoordinates from Google and finally show Google Map
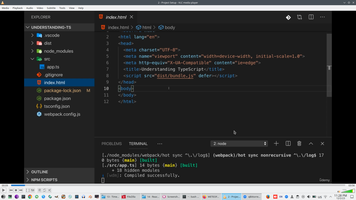
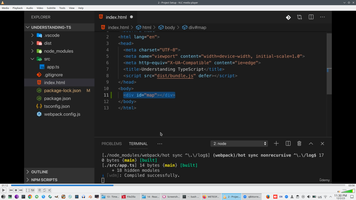
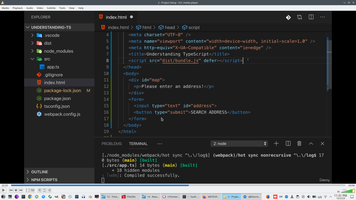
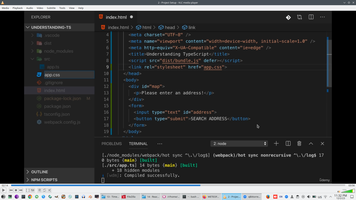
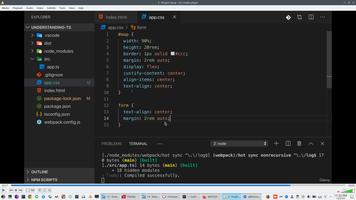
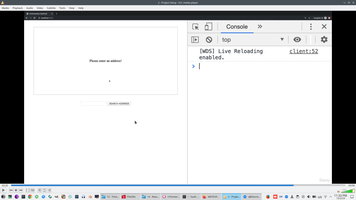
Make form submission firstly
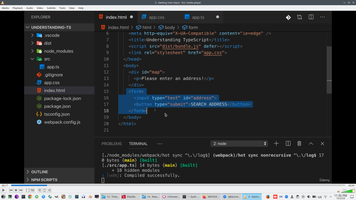
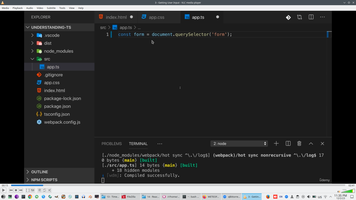
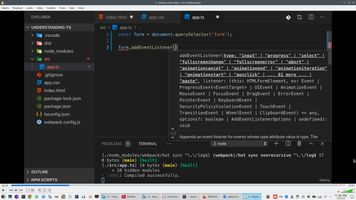
PreventDefault + Exclamation mark "!"
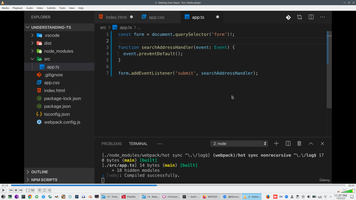
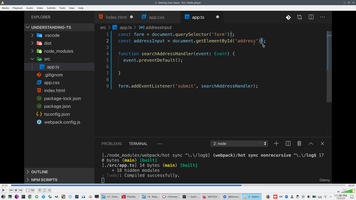
HtmlInputElement to access for Value
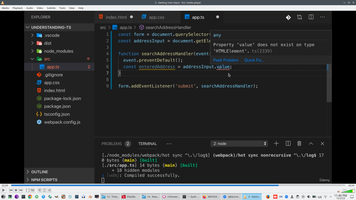
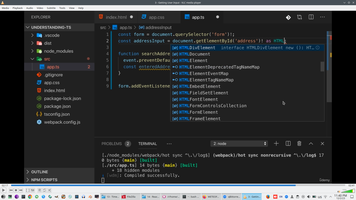
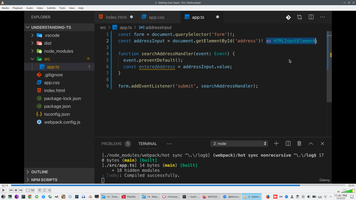
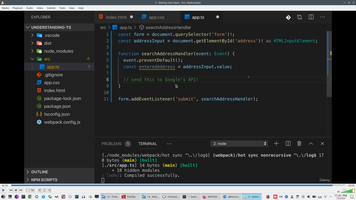
We will provide API key to URL request and receive coordinates from Google Maps
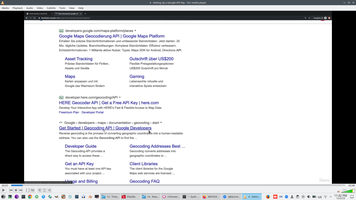
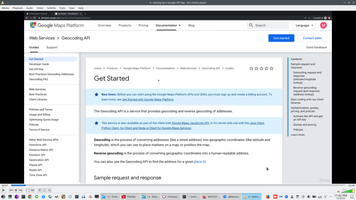
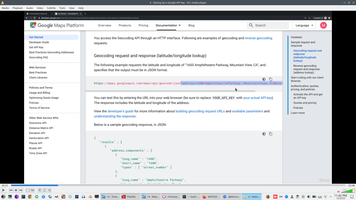
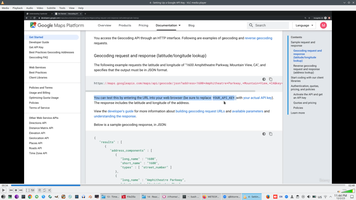
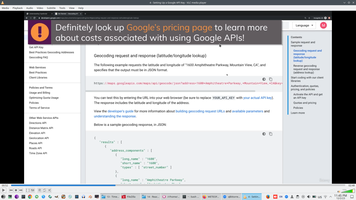
Setup Google API and receive Google Map key. Google map meed credit card.
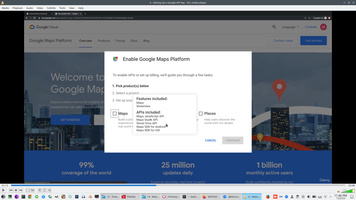
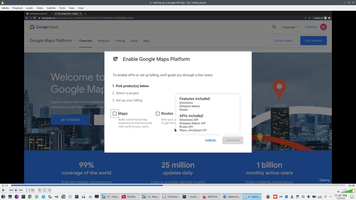
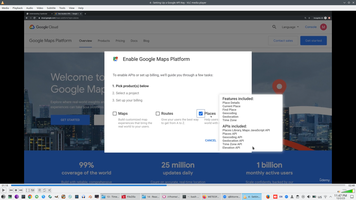
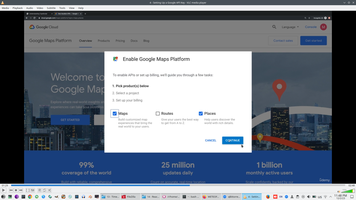
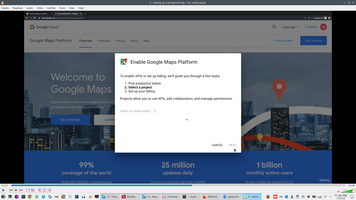
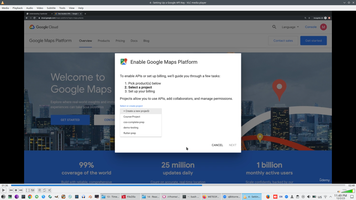
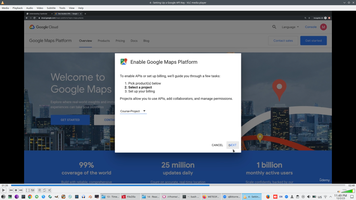
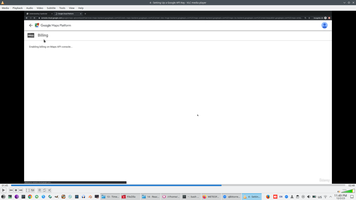
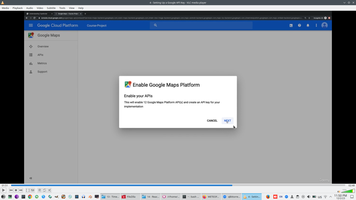
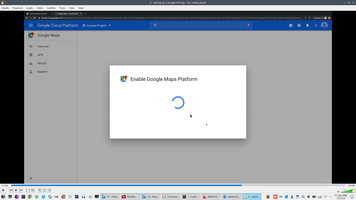
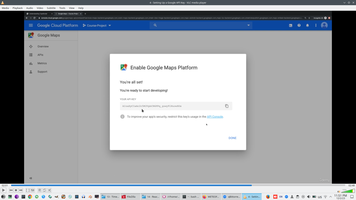
Pass key to project
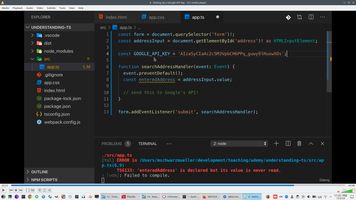
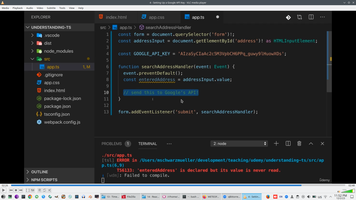
We can simple use JS Fetch API
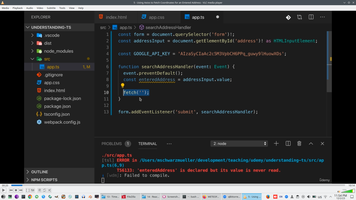
But we use Axios library. Install it.
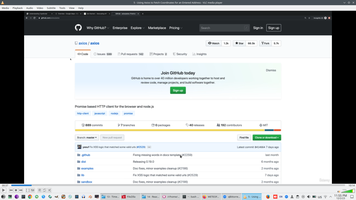
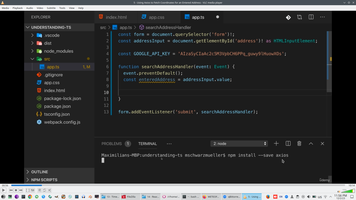
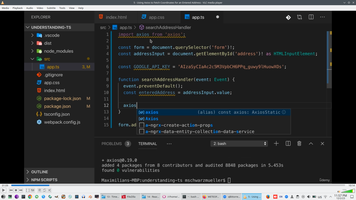
Axios already has index.d.ts a and don't need install @types
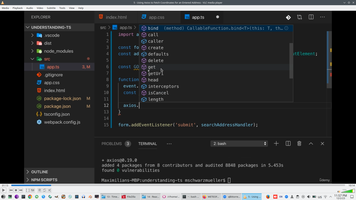
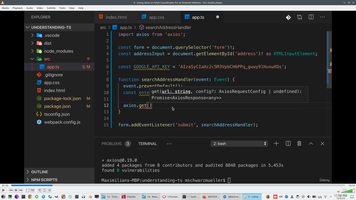
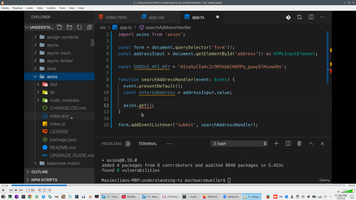
We can see all request and response types and in index.d.ts
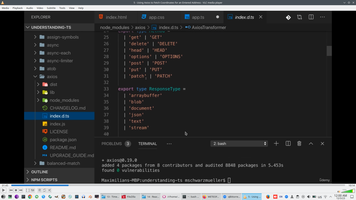
Pass URL from Google to project and add API key
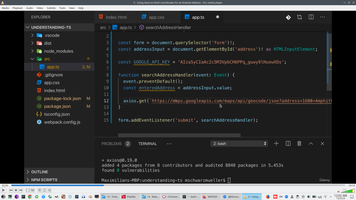
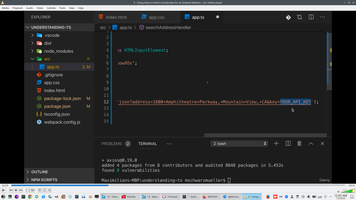
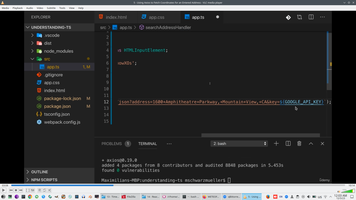
We must use EncodeUrl because API key can contains special characters
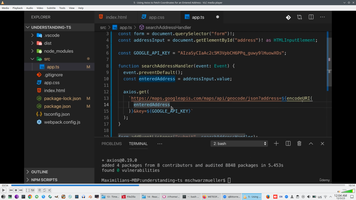
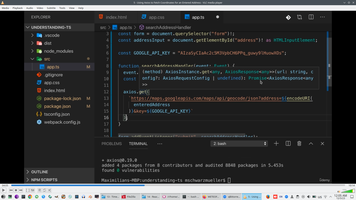
Axois Get return Promise, therefore we need to use then and catch
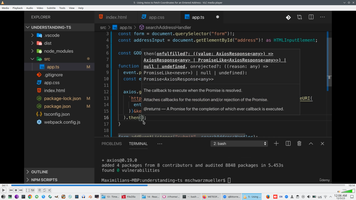
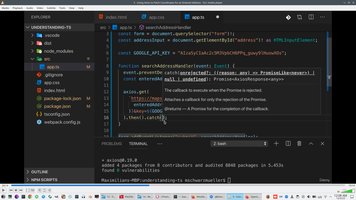
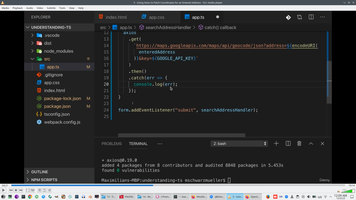
Request to Google ready, time to try
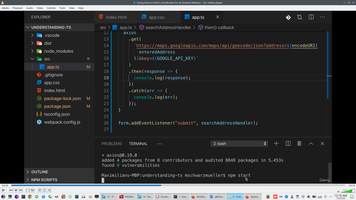
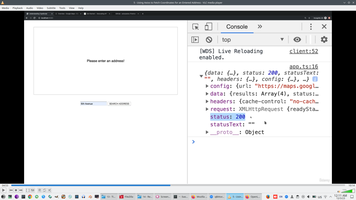
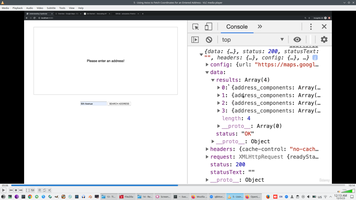
We can see array of result points, usually first is best
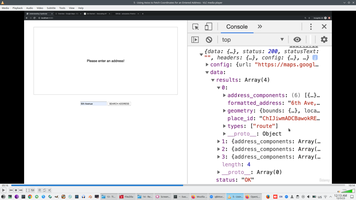
We need extract Latitude and Longitude from first array item
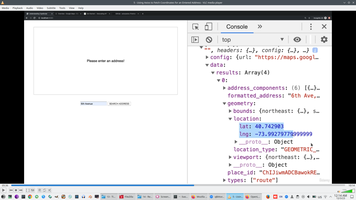
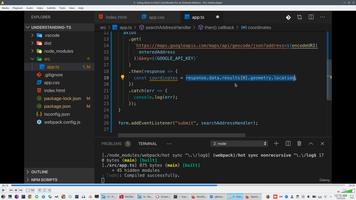
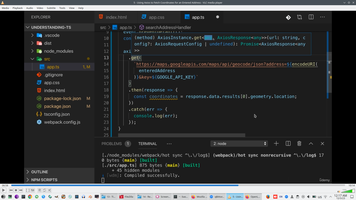
For Promise this is simple, this is not Observable, but Axois is generic library and we need to define structure of Google response
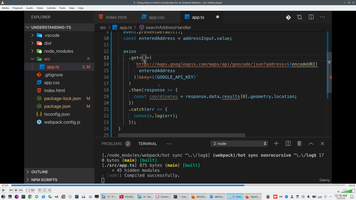
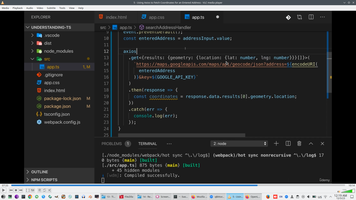
Define type GoogleGeoCodingResponse and make result Axois.Get result typed
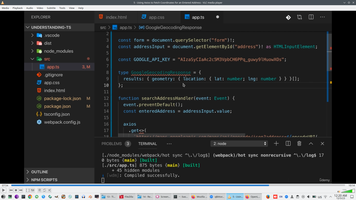
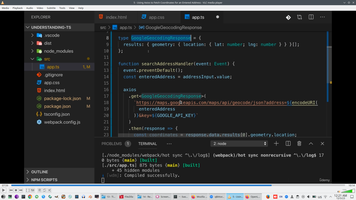
Look to All status what Google can return
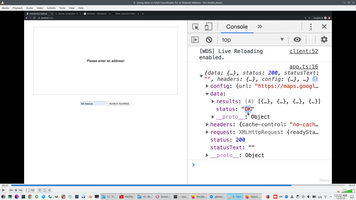
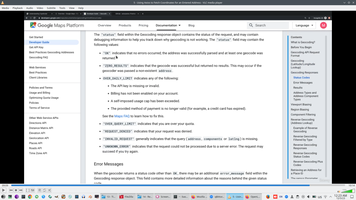
Define status as union type with Literal type and add that status to GoogleGeoCodingResponse. We have 7 types of response, but no one interesting for us, except OK
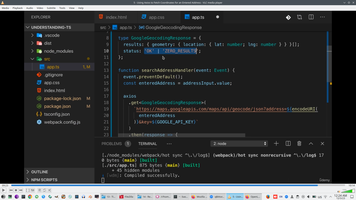
Throw new Error if status different than 'OK'
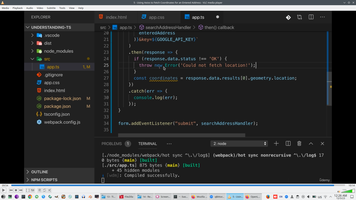
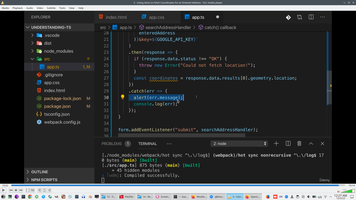
Grab script what can show Map and Delete InitMap callback
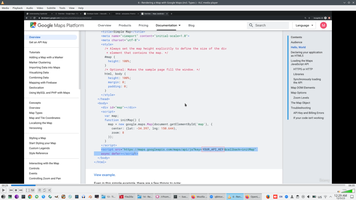
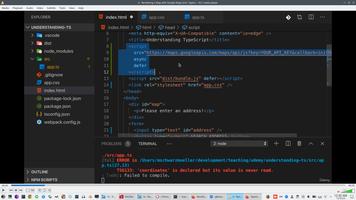
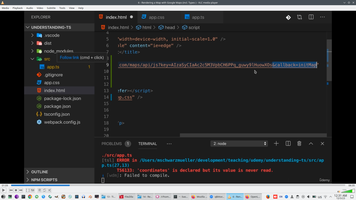
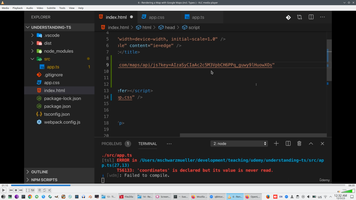
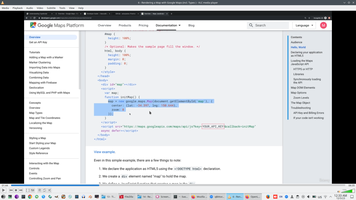
And call Show Google Map on Than branch
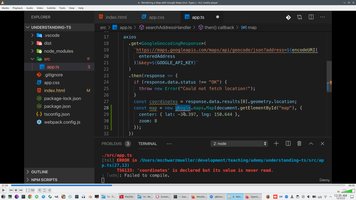
Google not defined on TS code, therefore we need Declare var Google:any;
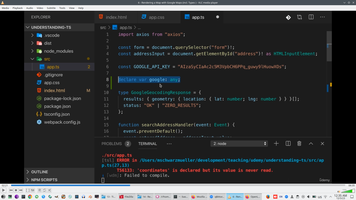
Look to Google how to render Marker and set marker with needed coordinates
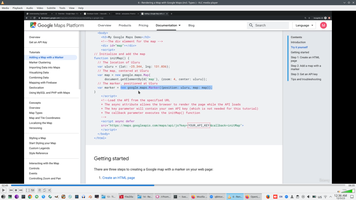
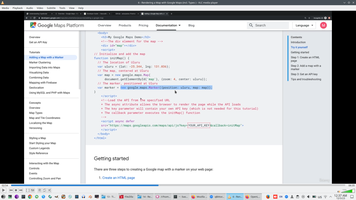
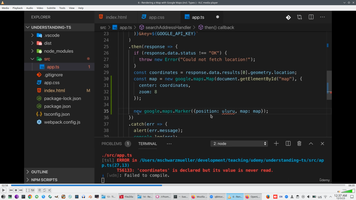
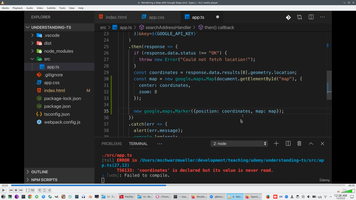
Check how project working
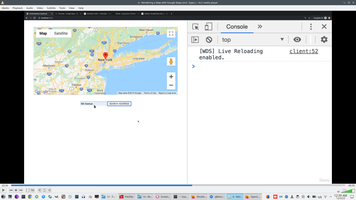
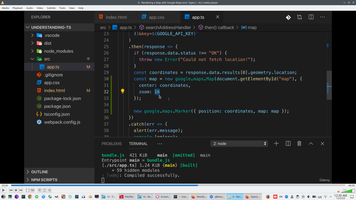
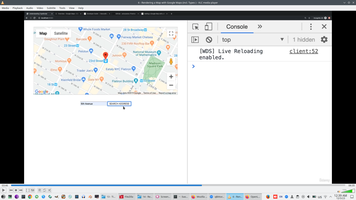
Check how error captured on catch branch, change Maps to Map.
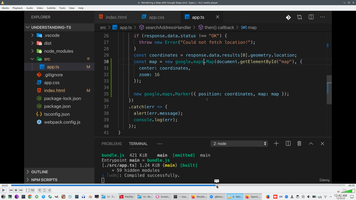
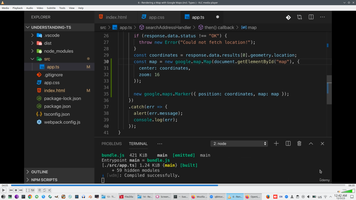
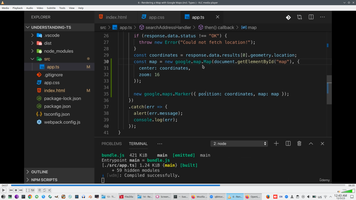
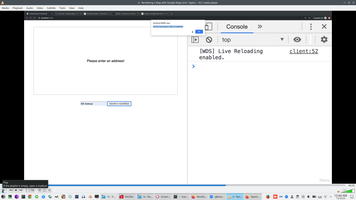
To use all Google API correctly we can install @types/GoogleMap
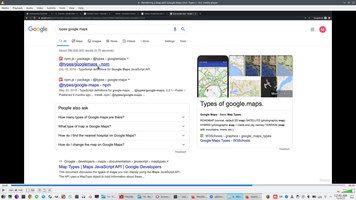
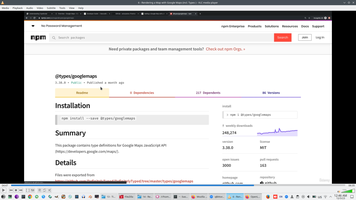
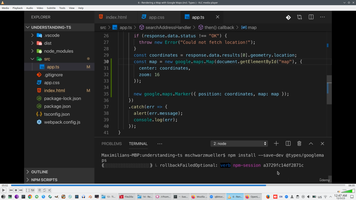
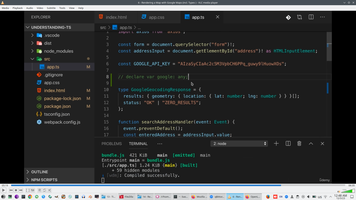
And now we can see errors of call Google API on compilation time
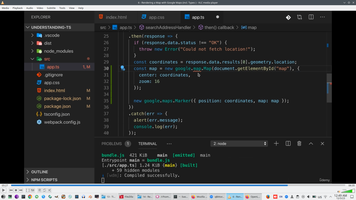
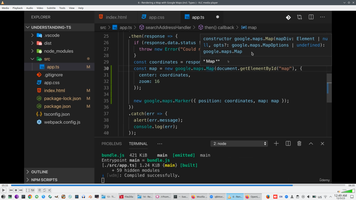
Best practice is fixing used library on config (--save-dev on installation)
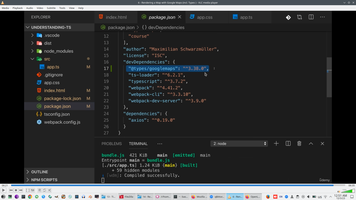
There are open alternatives to Google - without Credit card and money
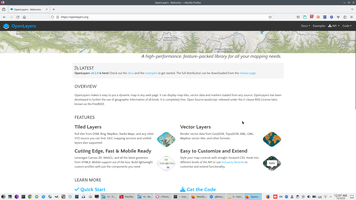
Related pages
- Angular documentation
- Typescript documentation
- Javascript documentation
- ECMAScript ES6 vs Typescript
- React vs Angular
- (2023) CloudflareWorker and Supabase
- (2022) NativeScript, AndroidBooks
- (2022) JS, Css
- (2022) Typescript, Webpack
- (2022) Angular, RxJs, Firebase, MongoDb
- (2022) Node, NestJs, Electron, Pwa, Telegram
- (2022) React, Redux, GraphQL, NextJs
- (2022) Angular/Typescript, JS books

<SITEMAP> <MVC> <ASP> <NET> <DATA> <KIOSK> <FLEX> <SQL> <NOTES> <LINUX> <MONO> <FREEWARE> <DOCS> <ENG> <CHAT ME> <ABOUT ME> < THANKS ME> |