ServiceWorker (SW01)
1: <!DOCTYPE html>
2: <html lang="en">
3: <head>
4: <meta charset="UTF-8">
5: <title>Service Worker Test</title>
6: </head>
7: <body>
8: <h3>Service Worker Test</h3>
9: <p>This page is cached by a Service Worker.</p>
10: <div id="dynamic-content"></div>
11: <img src="Logo-1.png" alt="Test Image">
12: <script src="SW03.js"></script>
13: <script src="SW01.js"></script>
14: </body>
15: </html>
1: if ('serviceWorker' in navigator) {
2: console.log('[Main Script] Service Worker supported');
3:
4: window.addEventListener('load', () => {
5: console.log('[Main Script] Page fully loaded. Registering Service Worker...');
6: navigator.serviceWorker.register('SW02.js')
7: .then(registration => {
8: console.log('Service worker registered:', registration);
9:
10: // Listen for controller change
11: navigator.serviceWorker.addEventListener('controllerchange', () => {
12: console.log('[Main Script] Service Worker is now controlling the page');
13: });
14:
15: // If the Service Worker is already controlling the page
16: if (navigator.serviceWorker.controller) {
17: console.log('[Main Script] Service Worker is already controlling the page');
18: }
19: })
20: .catch(error => {
21: console.error('Service worker registration failed:', error);
22: });
23: });
24: } else {
25: console.error('[Main Script] Service Worker not supported');
26: }
Service workers run in a separate thread and process (or context) from the web page. They have their own lifecycle and event model. It's not traditional multi-threading like Web Workers because they have a separate execution context and manage their own events and lifecycles. They can enhance user experience by caching and enabling background tasks without blocking the main thread and preventing memory leaks, ensuring smooth and responsive user interface interactions. They exist independent of web page, even when page is closed. They can receive push notifications. They can perform background fetch, background synchronization and other background operations. -------------Server--------------------------------- # npx http-server # Starting up http-server, serving ./ # http-server version: 10.1.1 # http-server settings: # CORS: disabled # Cache: 3600 seconds # Connection Timeout: 120 seconds # Directory Listings: visible # AutoIndex: visible # Serve GZIP Files: false # Serve Brotli Files: false # Default File Extension: none # Available on: # http://10.0.2.16:8080 # http://192.168.0.13:8080 # http://127.0.0.1:8080 # Hit CTRL-C to stop the server -------------BROWSER--------------------------------- app.js loaded! SW03.js:1:9 DOM fully loaded and parsed ServiceWorkerRegistration { installing: ServiceWorker, waiting: null, active: null, navigationPreload: NavigationPreloadManager, scope: "http://localhost:8080/", updateViaCache: "imports", onupdatefound: null, pushManager: PushManager } active: ServiceWorker { scriptURL: "http://localhost:8080/SW02.js", state: "activated", onstatechange: null, … } installing: null navigationPreload: NavigationPreloadManager { } onupdatefound: null pushManager: PushManager { } scope: "http://localhost:8080/" updateViaCache: "imports" waiting: null
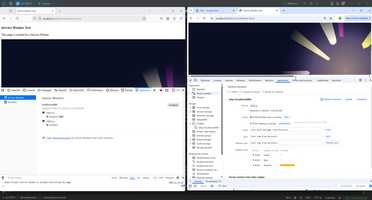
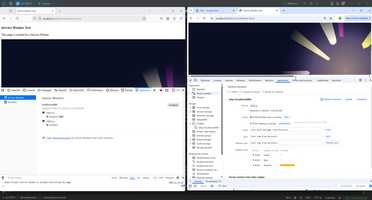
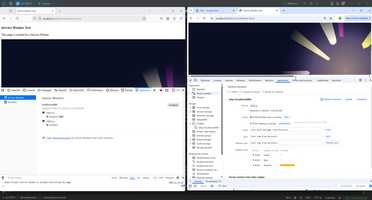
ServiceWorker context:
ES6 context:
- (2025) Basic workflow to working with Images (CSP, CORS), (Temporary URL as blob, Encoding image as data:image), (Fetch, Browser FileReader, Node-Fetch), (ArrayBuffer, TypedArray, DataView, HexConversion), DataStream (Concatenate Chunks, Convert data inside stream), (Image server, Image Proxy server). #Browser #FrontLearning #ES6
- (2024) Notes about JS Closures. #ES6
- (2024) Notes about Javascript asynchronous programming. #ES6
- (2022) Modern Javascript books #ES6 #Doc
- (2021) JS learning start point #ES6
- (2021) Maximilian Schwarzmüller Javascript lecture #ES6
- (2021) Javascript interview question from Happy Rawat #ES6
- (2021) Javascript tests #ES6
- (2016) New unique features of Javascript (updated). #ES6
Comments (
)

Link to this page:
http://www.vb-net.com/JavascriptES6/SW01.htm
|