3.3. My typical VB.NET desktop application. Fill TreeView and ListView without binding.
I have many descriptions how to fill TreeView with React, for example Multi Languages Spell Checker for webmaster. Part 3. Directory observer on NET Reactive extension., but in this page I describe simplest way to create TreeView nodes.
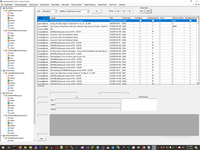
Firstly need to link ImageList to TreeView:
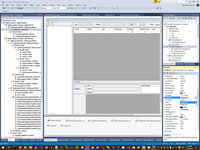
And after that you can fill treeview by the same way:
265:
266: Sub BuildTreeView()
267: For Each Top As v_GetUsers1 In VGetUsers1BindingSource.DataSource
268: Dim TopNode = New TreeNode With {.Text = Top.UserName, .Tag = Top.UserID, .ImageIndex = 1}
269: TreeView1.Nodes.Add(TopNode)
270: For Each Box As p_GetMailBox1_Result In db1.p_GetMailBox1(Top.UserID).ToList
271: Dim BoxNode As New TreeNode With {.Text = Box.login, .Tag = Box.idmailbox, .ImageIndex = 2}
272: TopNode.Nodes.Add(BoxNode)
273: Dim BoxType1 As New TreeNode With {.Text = "Inbox", .ImageIndex = 3}
274: BoxNode.Nodes.Add(BoxType1)
275: Dim BoxType2 As New TreeNode With {.Text = "Outbox", .ImageIndex = 4}
276: BoxNode.Nodes.Add(BoxType2)
277: Dim BoxType3 As New TreeNode With {.Text = "Sent", .ImageIndex = 5}
278: BoxNode.Nodes.Add(BoxType3)
279: Dim BoxType4 As New TreeNode With {.Text = "Deleted", .ImageIndex = 6}
280: BoxNode.Nodes.Add(BoxType4)
281: Dim BoxType5 As New TreeNode With {.Text = "Drafts", .ImageIndex = 7}
282: BoxNode.Nodes.Add(BoxType5)
283: Dim BoxType6 As New TreeNode With {.Text = "Filed", .ImageIndex = 8}
284: BoxNode.Nodes.Add(BoxType6)
285: Dim BoxType7 As New TreeNode With {.Text = "Search", .ImageIndex = 9}
286: BoxNode.Nodes.Add(BoxType7)
287: Next
288: Next
Filling Listview without binding is pretty simple too:
265:
220:
221: Sub FillAttachmentListListView(Attachments As IEnumerable(Of MimeKit.MimeEntity))
222: AttachmentListListView.Items.Clear()
223: If Attachments.Count = 0 Then
224: AttachmentListListView.BackColor = SystemColors.Control
225: Else
226: AttachmentListListView.BackColor = SystemColors.Window
227: For Each One As MimeKit.MimePart In Attachments
228: If One.IsAttachment Then
229: Dim AttItem As New ListViewItem(One.FileName, 0)
230: AttItem.ToolTipText = One.ContentType.ToString
231: AttachmentListListView.Items.Add(AttItem)
232: End If
233: Next
234: 'AttachmentListListView.Columns(AttachmentListListView.Columns.Count - 1).Width = -2
235: AttachmentListListView.Refresh()
236: End If
Also in ListView I usually create own draw module, if I moving cursor out of ListView, selected row is showing as necessary.
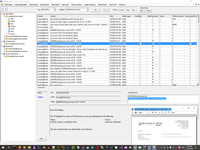
Ti rich this need firstly set particular parameters to ListView
18: AttachmentListListView.OwnerDraw = True
And after that handles particular event:
239: Dim AttachmentListListViewLostFocusItem As Integer = -1
240: Private Sub AttachmentListListView_Leave(sender As Object, e As EventArgs) Handles AttachmentListListView.Leave
241: AttachmentListListViewLostFocusItem = AttachmentListListView.FocusedItem.Index
242: End Sub
243:
244: Private Sub AttachmentListListView_DrawItem(sender As Object, e As DrawListViewItemEventArgs) Handles AttachmentListListView.DrawItem
245: If e.Item.Selected Then
246: If AttachmentListListViewLostFocusItem = e.Item.Index Then
247: e.Item.ForeColor = Color.Black
248: e.Item.BackColor = Color.LightBlue
249: AttachmentListListViewLostFocusItem = -1
250: ElseIf AttachmentListListView.Focused Then
251: e.Item.ForeColor = SystemColors.HighlightText
252: e.Item.BackColor = SystemColors.Highlight
253: End If
254: Else
255: e.Item.ForeColor = AttachmentListListView.ForeColor
256: e.Item.BackColor = AttachmentListListView.BackColor
257: End If
258: e.DrawBackground()
259: e.DrawText()
260: End Sub
Comments (
)

Link to this page:
//www.vb-net.com/Samantha/FillView.htm
|