KWMC - description the features of a typical ASP.NET MVC project.
This is part of my ASP.NET MVC Handbook..
- Project packages
- Use Dynamic route
- Use FluentMigrator to deploy Model POCO classes to Database engine
- Use AutoMapper to create projection (View) from POCO classes
- Use Autofac as dependency resolver
- Use Hangfire to start periodic task
- Use CustomAttribute to store metadata about model field
- Use attributes as common controller code
- Use UIHint attribute to define template for EditorFor and DisplayFor helpers
- Save ModelState with Redirection
- Use attributes as common controller code
- Use jQuery Unobtrusive Validation, custom attributes for validation and validation service/controller
- Use Owin Authentication
- Use RoleBasedAccess - special security model
- Use SqlDependency/SqlServiceBroker to send notification to SignalR hub
- Use SignalR to notify other user about events
- Use Ajax/WebApi Syns/Async request/response.
- Use SiteMap to provide forms title and available menu/step
- Define common code as Function and Helpers (directly in View and in the codeBehind)
- Use special services with dependency injection.
- Use Database to store all project resources. Use Middleware (Module) to handle request to resource
- Create middleware as separate project and register all modules in main project
- Project store UserCulture to Database
- Define IEnumerable extension interface to Hierarchy LinqToSQL and LinqToObject
- Use Google reCaptcha ASP.NET MVC (clearly in View and as filter attribute)
- More efficiency - use (1) GzipCompression, (2) Cache, (3) manual MemoryDispose, (4) Bundle scripts and CSS.
- Use DragAndDrop JS with custom XHR processing
- Use Bootstrap template
- Use BootsrapModal window
- This project support SmartCustmDataTable, SCEditor, Chats, various DataPicker and a lot of other futures
- Use third-party web services - (1) Open Weather data, (2) Azure Earth Map, (3) Azure storage for saving files, (4) WebService about vehicle model, (5) Bitly.com to shorten URL for SMS
- This project has full web interface to Mail/SMS system. Use SendGrid-csharp library from Twilio.
- Use Google JS API
- Detect mobile device
- 1. Project packages
- 2. First interesting future is dynamic route. You can select PropertyArea and PropertyArea will be add to URL.
- 3. Second interesting future of this project is FluentMigrator github, quickstart. This ORM allow deploy Model POCO classes to Database engine and despite it has 20-30 times less popularity than EF, however some projects use it. More about ORM - ???????????????????????? ?????????????? ???????????? ?? ????????????..
- 3. AutoMapper https://automapper.org/ allow create Projection (View) from POCO classes. This project actively used AutoMapper. This is common implementation of this my function Amazing extension function CopyLinqDataMembersByName to expand Linq-to-SQL..
- 5. Next interesting future of this project is Autofac https://autofac.org/, github. NET Core use embedded dependency resolver, this project made on .NET Framework, therefore is use Autofac as dependency resolver.
- 6. Use Hangfire https://www.hangfire.io/ to define periodic task. Alternative way see in page Blazor tests of Quartz jobs.
- 7. Use CustomAttribute to store metadata about model field.
- 8. Use UIHint attribute to define template for EditorFor and DisplayFor helpers.
- 9. Save ModelState with Redirection. More Store state in ViewState/ViewBag/ViewData/TempData, Maintain ModelState with redirectToAction.
- 10. Use attributes as common controller code
- 11. Use jQuery Unobtrusive Validation, custom attributes for validation and validation service/controller. More about validation see in page jquery.validate.unobtrusive.js.
- 12. Use Owin Authentication. Practical Example to receive OWIN token SetUp OWIN providers.
- 13. RoleBasedAccess - special security model realized by attributes and helpers. For example I'm administrator of this system, but have not access to control perking place.
- 14. Use SqlDependency/SqlServiceBroker to send notification to SignalR hub. More - Enabling Query Notifications. At common I have my own implementation this function since 2005 year ????????????-???????????? ?????? ???????????????? ?????????????????????? ???? ???????????????????? ???????????? ?? SQL-?????????????? ???? ?????????????????? ???????? ??????????.
- 15. Use SignalR to notify other user about events. More How to debug SignalR application in Net Core 3.1, Use SignalRSwaggerGen to create Swagger specification of SignalR hub, Use Fiddler as reverse proxy to isolate microservice for trace request from Redux frontend and to Amazon DynamoDB and debug SignalR hub.
- 16. Use Ajax/WebApi Syns/Async request/response. More The ways to receive complex parameters in ASP.NET, How classic ASP.NET programmers has realized WEB.API since 2005 year, Set Up ASP.NET API Endpoints to open database on internet
- 17. Use SiteMap to provide forms title and available menu/step. This is full difference SiteMap provider I usually use ( Manage site with huge number of page, ???????????? SEO SiteMap, Scott Mitchell Custom SiteMap provider)
- 18. Define common code as Function and Helpers (directly in View and in the codeBehind). Usually Helpers produced HTML, but function is a simple string processing. More ?????????????? ?????? ?????????????????? ?????? ?? ???????????????? MVC, How to create Razor html-helper in VB.NET.
- 19. This project user hundreds of common classes (like GenerateRandomPassword, UTF DateTimeConverter, ReadStream and so on) and special services with dependency injection.
- 20. Use Database to store all project resources. Use Middleware (Module) to handle request to resource. More Processing resources in ASP.NET.
- 22. Create middleware as separate project and register all modules in main project.
- 23. Project store UserCulture to Database
- 24. Define IEnumerable extension interface to Hierarchy LinqToSQL and LinqToObject. More Yield/Iterator/IEnumerable - ?? ???? ???????? ???????????????????? ?????? ???????????? ?????????? ???????????????????? ?? ?????????, Serialize Table to CSV with Iterator and Yeld, Generic VB.NET function for loading ExcelFile to DataBase (EF6 CodeFirst) with Reflection and avoiding Linq-to-Entity Linq.Expressions (use Linq-to-Object IEnumerable) , Collection.Generic.List (of T), IQueryable vs IEnumerable.
- 25. Use Google reCaptcha ASP.NET MVC (clearly in View and as filter attribute). I don't use this library, because I have a lot of own implementation of this function, for example look to this my page from 2006 year FeedBack-??????????????????. And also for critical application I have my own Virtual Keyboard (to protect from KeyLogger), look for example to this page ???????????????????????? Web-????????????????????.
- 26. More efficiency - use (1) GzipCompression, (2) Cache, (3) manual MemoryDispose, (4) Bundle scripts and CSS. Bundle definition in this project has more than 300 code lines. More about GzipCompression - 3 Way of GzipCompression.
- 27. Use DragAndDrop JS with custom XHR processing. More about XHR handles Inject OnBegin/OnSuccess/OnFailure custom Javascript code into ASP.NET Ajax tag by Bundle of jquery.unobtrusive-ajax.min.js, more about various JS Image processing Cropper ?????????????? ??????????..
- 28. There are a lot of site with free booBootstrap templates like https://themewagon.com/, but this project use SmartAdmin template from https://wrapbootstrap.com/. Also below you can see list of function of SmartAdmin template. This is official documentation for SmartAdmin bootstrap template. And there are a lot of other useful description of this template SmartAdmin Responsive WebApp.
- 29. Use BootsrapModal window. JS Modal is not a simple future, look to this my page for example My solution with Bootstrap modal window and Classic ASP.NET.
- 30. This project support SmartCustmDataTable, SCEditor, Chats, various DataPicker and a lot of other JS futures. More - ?????????????????????? (datapicker), ?????????????????????? ???????? ???? jQuery / MS AJAX / JavaScript / Flex / MONO, FCKEditor (text formatter).
- 31. Use third-party web services - (1) Open Weather data, (2) Azure Earth Map, (3) Azure storage for saving files, (4) WebService about vehicle model, (5) Bitly.com to shorten URL for SMS
- 32. This project has full web interface to Mail system. Use SendGrid-csharp library from Twilio.
- 33. Use Google JS API https://developers.google.com/apis-explorer/, https://github.com/google/google-api-javascript-client. More example of using Google API - How to build application server based on push notification from Firebase, MongoDB - noSQL-database for irregular JSON data, Parse Yotube response by Newtonsoft.Json, Send mails via Gmail.
- 34. Detect mobile device. Mobile device can be detected by many case, first method is define @media in CSS Landing page with adaptive design and country recognizing from database, second method is applied in this site - to analyze HTTP_USER_AGENT, the same way I use in my projects, for example ???????? ?????? ??????????????????????, and third method I used in my ancient site in ASP (ASP without .NET) - setup COOKIE by JS and analyze this COOKIE.
This is package list of this project and below I make small describe about some interesting future of this project and pattern of using some of this packages.
1: <?xml version="1.0" encoding="utf-8"?>
2: <packages>
3: <package id="AjaxMin" version="5.14.5506.26202" targetFramework="net461" />
4: <package id="Antlr" version="3.5.0.2" targetFramework="net45" />
5: <package id="Antlr3.Runtime" version="3.5.1" targetFramework="net45" />
6: <package id="Aurochses.Data" version="1.2.2" targetFramework="net461" />
7: <package id="AutoAutoMapper" version="0.2.0.0" targetFramework="net461" />
8: <package id="Autofac" version="4.8.1" targetFramework="net461" />
9: <package id="Autofac.Mvc5" version="4.0.2" targetFramework="net45" />
10: <package id="Autofac.Owin" version="4.2.0" targetFramework="net461" />
11: <package id="Autofac.WebApi2" version="4.2.0" targetFramework="net461" />
12: <package id="Autofac.WebApi2.Owin" version="4.0.0" targetFramework="net461" />
13: <package id="AutoMapper" version="6.1.1" targetFramework="net461" />
14: <package id="bootstrap" version="3.3.7" targetFramework="net45" />
15: <package id="BundleTransformer.Core" version="1.9.160" targetFramework="net45" />
16: <package id="BundleTransformer.MicrosoftAjax" version="1.9.160" targetFramework="net461" />
17: <package id="EntityFramework" version="6.1.3" targetFramework="net45" />
18: <package id="FontAwesome" version="4.7.0" targetFramework="net45" />
19: <package id="Foolproof" version="0.9.4518" targetFramework="net45" />
20: <package id="Hangfire" version="1.6.19" targetFramework="net461" />
21: <package id="Hangfire.Autofac" version="2.3.1" targetFramework="net45" />
22: <package id="Hangfire.Core" version="1.6.19" targetFramework="net461" />
23: <package id="Hangfire.SqlServer" version="1.6.19" targetFramework="net461" />
24: <package id="JetBrains.Annotations" version="8.0.5.0" targetFramework="net461" />
25: <package id="jQuery" version="3.1.1" targetFramework="net45" />
26: <package id="jQuery.FileUpload" version="9.18.0" targetFramework="net45" />
27: <package id="jQuery.mvc.LightBox" version="1.0.2" targetFramework="net45" />
28: <package id="jQuery.UI.Combined" version="1.12.1" targetFramework="net45" />
29: <package id="jQuery.Validation" version="1.16.0" targetFramework="net45" />
30: <package id="Microsoft.AspNet.Identity.Core" version="2.2.1" targetFramework="net461" />
31: <package id="Microsoft.AspNet.Identity.Owin" version="2.2.1" targetFramework="net461" />
32: <package id="Microsoft.AspNet.Mvc" version="5.2.3" targetFramework="net461" />
33: <package id="Microsoft.AspNet.Mvc.Futures" version="5.0.0" targetFramework="net45" />
34: <package id="Microsoft.AspNet.Razor" version="3.2.3" targetFramework="net45" />
35: <package id="Microsoft.AspNet.SignalR" version="2.2.2" targetFramework="net45" />
36: <package id="Microsoft.AspNet.SignalR.Core" version="2.2.2" targetFramework="net45" />
37: <package id="Microsoft.AspNet.SignalR.JS" version="2.2.2" targetFramework="net45" />
38: <package id="Microsoft.AspNet.SignalR.SystemWeb" version="2.2.2" targetFramework="net45" />
39: <package id="Microsoft.AspNet.Web.Optimization" version="1.1.3" targetFramework="net45" />
40: <package id="Microsoft.AspNet.WebApi" version="5.2.3" targetFramework="net461" />
41: <package id="Microsoft.AspNet.WebApi.Client" version="5.2.3" targetFramework="net461" />
42: <package id="Microsoft.AspNet.WebApi.Core" version="5.2.3" targetFramework="net461" />
43: <package id="Microsoft.AspNet.WebApi.Owin" version="5.2.0" targetFramework="net461" />
44: <package id="Microsoft.AspNet.WebApi.WebHost" version="5.2.3" targetFramework="net461" />
45: <package id="Microsoft.AspNet.WebPages" version="3.2.3" targetFramework="net45" />
46: <package id="Microsoft.jQuery.Unobtrusive.Ajax" version="3.2.3" targetFramework="net45" />
47: <package id="Microsoft.jQuery.Unobtrusive.Validation" version="3.2.3" targetFramework="net45" />
48: <package id="Microsoft.NETCore.Platforms" version="1.1.0" targetFramework="net461" />
49: <package id="Microsoft.Owin" version="3.1.0" targetFramework="net45" />
50: <package id="Microsoft.Owin.Host.SystemWeb" version="3.1.0" targetFramework="net45" />
51: <package id="Microsoft.Owin.Security" version="3.1.0" targetFramework="net45" />
52: <package id="Microsoft.Owin.Security.Cookies" version="3.1.0" targetFramework="net461" />
53: <package id="Microsoft.Owin.Security.Facebook" version="3.1.0" targetFramework="net461" />
54: <package id="Microsoft.Owin.Security.Google" version="3.1.0" targetFramework="net461" />
55: <package id="Microsoft.Owin.Security.OAuth" version="2.1.0" targetFramework="net461" />
56: <package id="Microsoft.Owin.Security.Twitter" version="3.1.0" targetFramework="net461" />
57: <package id="Microsoft.Web.Infrastructure" version="1.0.0.0" targetFramework="net45" />
58: <package id="Microsoft.Win32.Primitives" version="4.3.0" targetFramework="net461" />
59: <package id="Modernizr" version="2.8.3" targetFramework="net45" />
60: <package id="morelinq" version="2.6.0" targetFramework="net461" />
61: <package id="MvcDisplayTemplates" version="4.0.0" targetFramework="net45" />
62: <package id="MvcSiteMapProvider.MVC5" version="4.6.22" targetFramework="net45" />
63: <package id="MvcSiteMapProvider.MVC5.Core" version="4.6.22" targetFramework="net45" />
64: <package id="MvcSiteMapProvider.Web" version="4.6.22" targetFramework="net45" />
65: <package id="NETStandard.Library" version="1.6.1" targetFramework="net461" />
66: <package id="Newtonsoft.Json" version="10.0.3" targetFramework="net45" />
67: <package id="Owin" version="1.0" targetFramework="net45" />
68: <package id="reCaptcha.AspNet.Mvc" version="1.2.3.0" targetFramework="net461" />
69: <package id="Select.HtmlToPdf" version="17.1.0" targetFramework="net45" />
70: <package id="Select2.js" version="4.0.3" targetFramework="net45" />
71: <package id="System.AppContext" version="4.3.0" targetFramework="net461" />
72: <package id="System.Collections" version="4.3.0" targetFramework="net461" />
73: <package id="System.Collections.Concurrent" version="4.3.0" targetFramework="net461" />
74: <package id="System.Console" version="4.3.0" targetFramework="net461" />
75: <package id="System.Diagnostics.Debug" version="4.3.0" targetFramework="net461" />
76: <package id="System.Diagnostics.DiagnosticSource" version="4.3.0" targetFramework="net461" />
77: <package id="System.Diagnostics.Tools" version="4.3.0" targetFramework="net461" />
78: <package id="System.Diagnostics.Tracing" version="4.3.0" targetFramework="net461" />
79: <package id="System.Globalization" version="4.3.0" targetFramework="net461" />
80: <package id="System.Globalization.Calendars" version="4.3.0" targetFramework="net461" />
81: <package id="System.IO" version="4.3.0" targetFramework="net461" />
82: <package id="System.IO.Compression" version="4.3.0" targetFramework="net461" />
83: <package id="System.IO.Compression.ZipFile" version="4.3.0" targetFramework="net461" />
84: <package id="System.IO.FileSystem" version="4.3.0" targetFramework="net461" />
85: <package id="System.IO.FileSystem.Primitives" version="4.3.0" targetFramework="net461" />
86: <package id="System.Linq" version="4.3.0" targetFramework="net461" />
87: <package id="System.Linq.Expressions" version="4.3.0" targetFramework="net461" />
88: <package id="System.Net.Http" version="4.3.0" targetFramework="net461" />
89: <package id="System.Net.Primitives" version="4.3.0" targetFramework="net461" />
90: <package id="System.Net.Sockets" version="4.3.0" targetFramework="net461" />
91: <package id="System.ObjectModel" version="4.3.0" targetFramework="net461" />
92: <package id="System.Reflection" version="4.3.0" targetFramework="net461" />
93: <package id="System.Reflection.Extensions" version="4.3.0" targetFramework="net461" />
94: <package id="System.Reflection.Primitives" version="4.3.0" targetFramework="net461" />
95: <package id="System.Resources.ResourceManager" version="4.3.0" targetFramework="net461" />
96: <package id="System.Runtime" version="4.3.0" targetFramework="net461" />
97: <package id="System.Runtime.Extensions" version="4.3.0" targetFramework="net461" />
98: <package id="System.Runtime.Handles" version="4.3.0" targetFramework="net461" />
99: <package id="System.Runtime.InteropServices" version="4.3.0" targetFramework="net461" />
100: <package id="System.Runtime.InteropServices.RuntimeInformation" version="4.3.0" targetFramework="net461" />
101: <package id="System.Runtime.Numerics" version="4.3.0" targetFramework="net461" />
102: <package id="System.Security.Cryptography.Algorithms" version="4.3.0" targetFramework="net461" />
103: <package id="System.Security.Cryptography.Encoding" version="4.3.0" targetFramework="net461" />
104: <package id="System.Security.Cryptography.Primitives" version="4.3.0" targetFramework="net461" />
105: <package id="System.Security.Cryptography.X509Certificates" version="4.3.0" targetFramework="net461" />
106: <package id="System.Text.Encoding" version="4.3.0" targetFramework="net461" />
107: <package id="System.Text.Encoding.Extensions" version="4.3.0" targetFramework="net461" />
108: <package id="System.Text.RegularExpressions" version="4.3.0" targetFramework="net461" />
109: <package id="System.Threading" version="4.3.0" targetFramework="net461" />
110: <package id="System.Threading.Tasks" version="4.3.0" targetFramework="net461" />
111: <package id="System.Threading.Timer" version="4.3.0" targetFramework="net461" />
112: <package id="System.ValueTuple" version="4.4.0" targetFramework="net461" />
113: <package id="System.Xml.ReaderWriter" version="4.3.0" targetFramework="net461" />
114: <package id="System.Xml.XDocument" version="4.3.0" targetFramework="net461" />
115: <package id="Twitter.Bootstrap" version="3.0.1.1" targetFramework="net45" />
116: <package id="WebActivatorEx" version="2.2.0" targetFramework="net45" />
117: <package id="WebGrease" version="1.6.0" targetFramework="net45" />
118: <package id="WebMarkupMin.Core" version="2.4.0" targetFramework="net45" />
119: <package id="WebMarkupMin.Mvc" version="1.1.0" targetFramework="net45" />
120: <package id="WebMarkupMin.Web" version="1.1.1" targetFramework="net45" />
121: </packages>
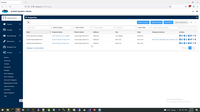
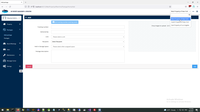
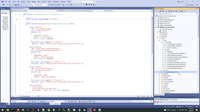
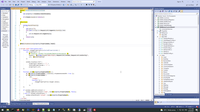
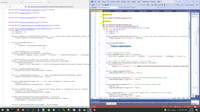
All controllers has special attributes HidePropertySelector and form accuracy handled property attribute missing.
It is interesting that whole controllers, models and view was be accuracy placed to Area, not in main site folder as usually. Each controller in Area has additionally registration.
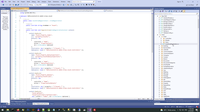
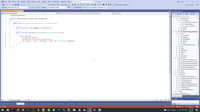
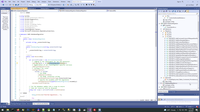
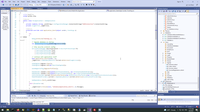
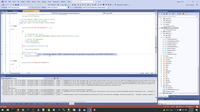
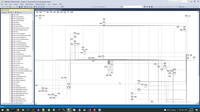
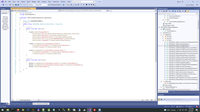
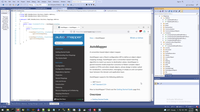
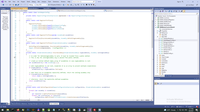
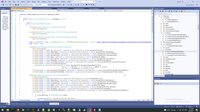
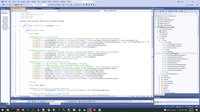
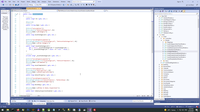
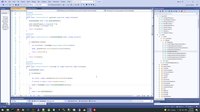
AutoMapper support M:1, 1:M, M:M data relation. More about data relation ???????????????????? ????'?????????? ?????????? 1:1, 1:M, M:M ?? ???????? ???? EF CodeFirst., FinancialBroker - MDI application with EF code first database.
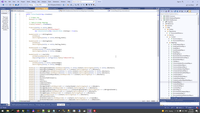
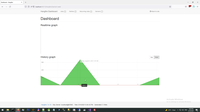
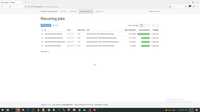
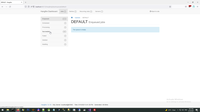
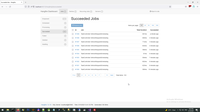
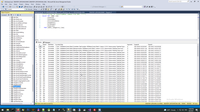
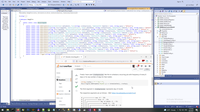
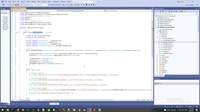
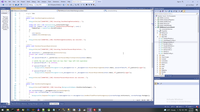
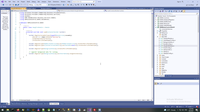
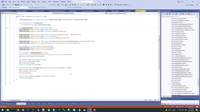
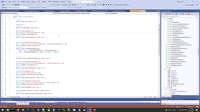
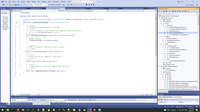
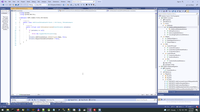
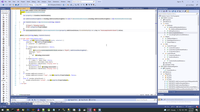
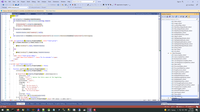
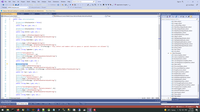
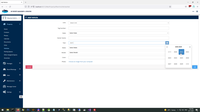
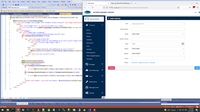
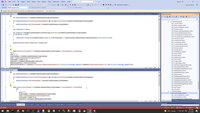
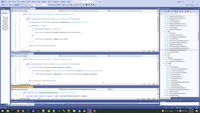
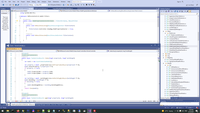
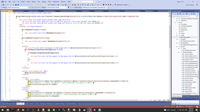
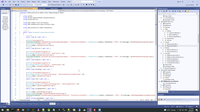
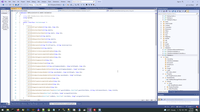
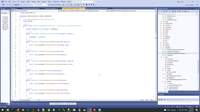
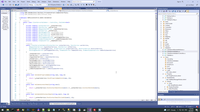
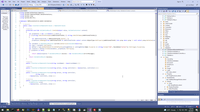
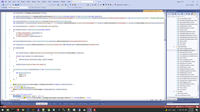
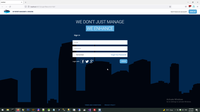
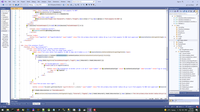
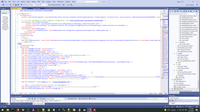
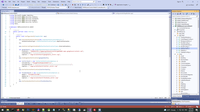
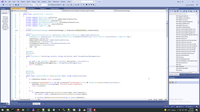
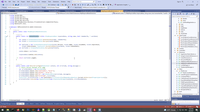
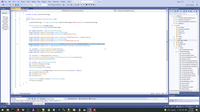
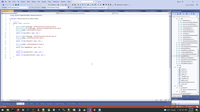
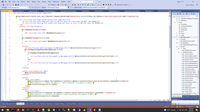
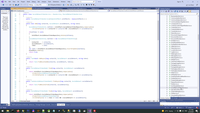
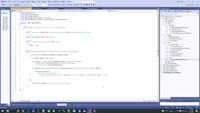
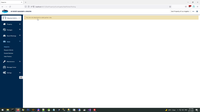
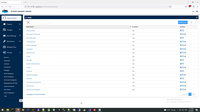
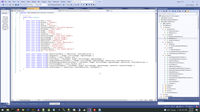
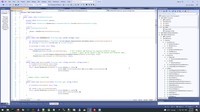
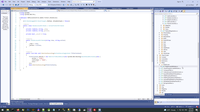
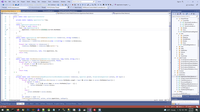
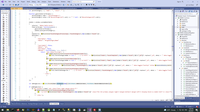
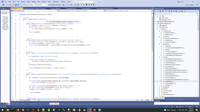
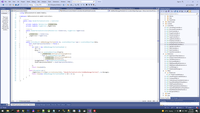
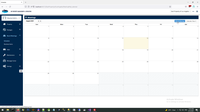
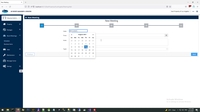
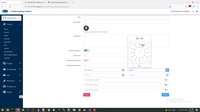
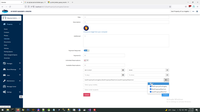
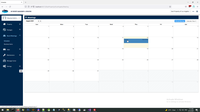
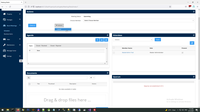
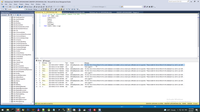
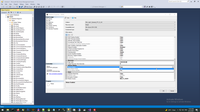
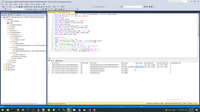
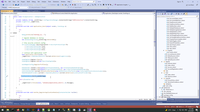
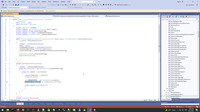
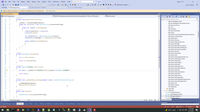
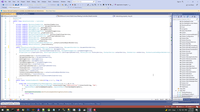
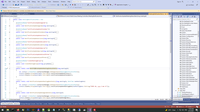
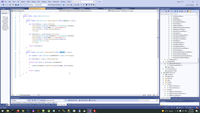
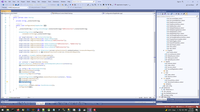
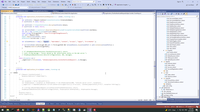
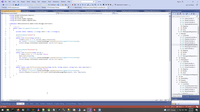
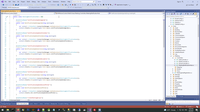
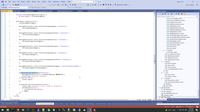
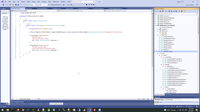
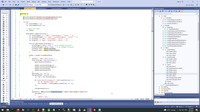
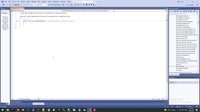
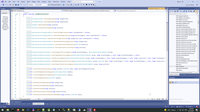
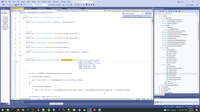
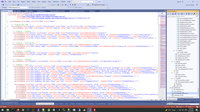
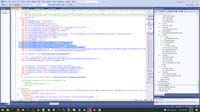
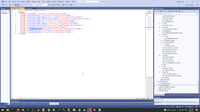
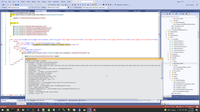
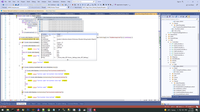
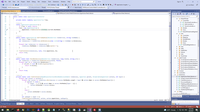
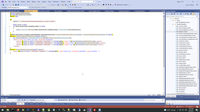
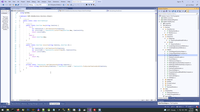
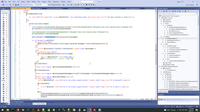
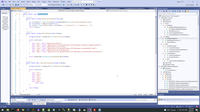
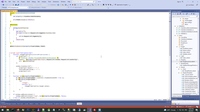
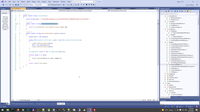
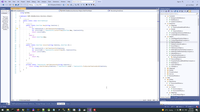
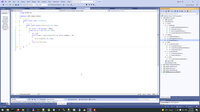
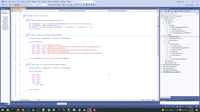
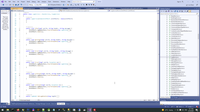
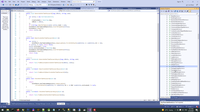
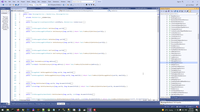
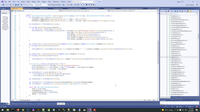
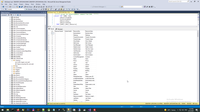
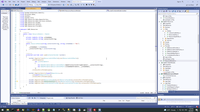
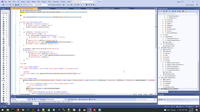
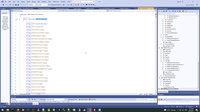
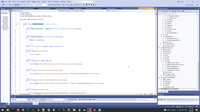
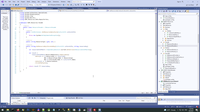
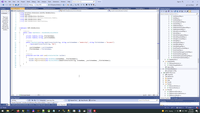
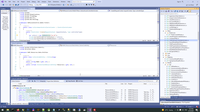
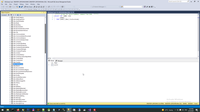
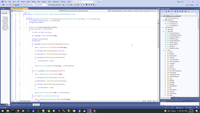
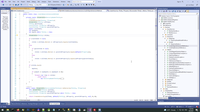
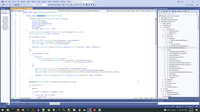
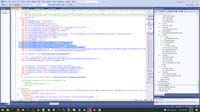
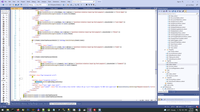
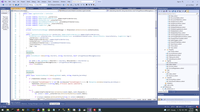
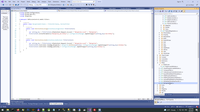
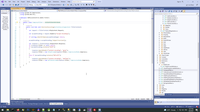
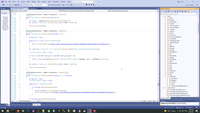
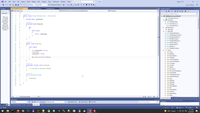
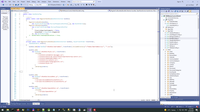
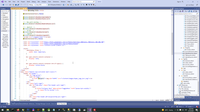
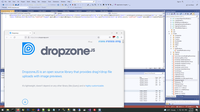
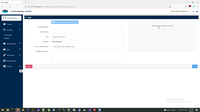
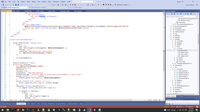
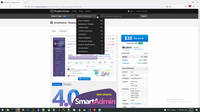
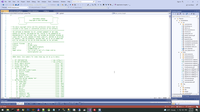
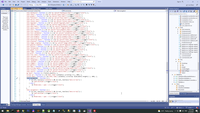
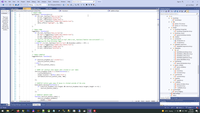
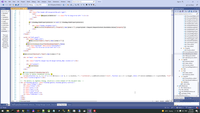
Other useful site, related to Bootstrap: Bootstrap grid system, BootstrapSnippetPack, Bootstrap 3 Snippets, mdbootstrap, getbootstrap, bootsrap column generator, bootstrap builder
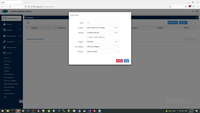
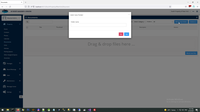
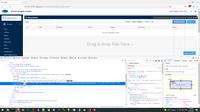
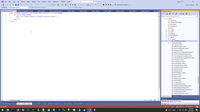
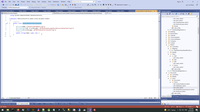
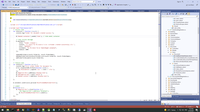
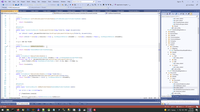
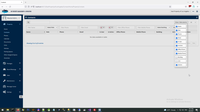
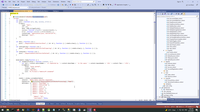
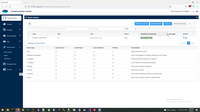
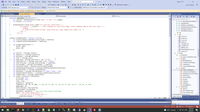
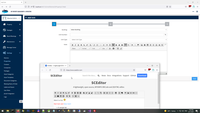
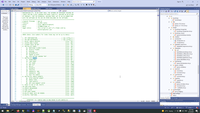
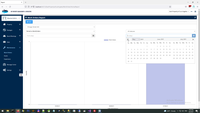
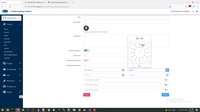
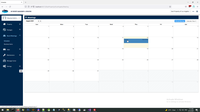
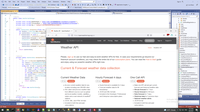
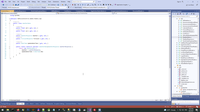
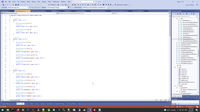
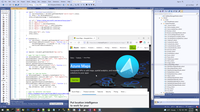
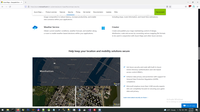
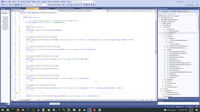
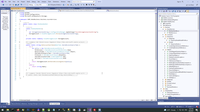
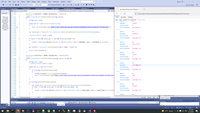
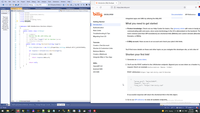
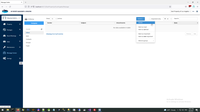
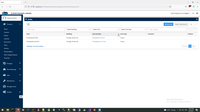
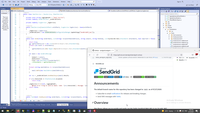
More about Email processing. Use Twilio to send SMS, My typical VB.NET desktop application. Parse message body by MailKit/MimeKit and save attachment, ASP.NET Core Backend with MailKit/IMAP (Async/Await, Monitor, ConcurrentQueue, CancellationTokenSource)
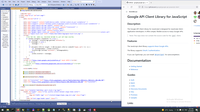
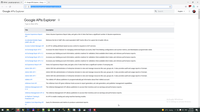
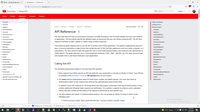
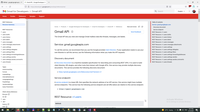
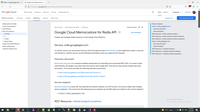
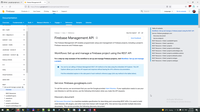
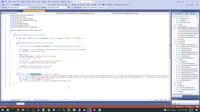

|