Landing page with adaptive design and country recognizing from database
- 1. Front-End.
- 2. Admin panel design. Loading IP database.
- 3. Admin panel authentication.
- 4. Loading IP CSV-file to database.
- 5. Cache IP database to memory.
- 6. Get IP4 from IP6 address.
- 7. Get Country by IP.
- 8. MVC controller code.
- 9. Layout page and Views.
Below you can see one my small MVC project for one special Landing with adaptive design. In this project I work as full stack programmer, including job of html layout engineer, therefore firstly I describe front-end of this project. This project need to work extremely fast, therefore I create it without any script, only on CSS.
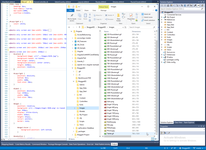
1. Front-End.
What is adaptive design? My CSS file contains six different parts, six different html markup.
1: html, body {
2: padding: 0px;
3: font-family: Cuprum;
4: max-width:100%;
5: overflow-x:hidden;
6: }
7:
8:
9: #copyright p {
10: color: white;
11: }
12:
13: @media only screen and (max-width: 320px) {
14: #content {
15: background-image: url("../Images/Phone.jpg");
...:
123: }
124:
125: @media only screen and (min-width: 320px) and (max-width: 480px) {
126: #content {
...:
235: }
236:
237: @media only screen and (min-width: 480px) and (max-width: 768px) {
238: #content {
239: background-image: url("../Images/Tablet.gif");
...:
352: }
353:
354: @media only screen and (min-width: 768px) and (max-width: 1920px) {
355: #content {
...:
470: }
471:
472: @media screen and (min-width: 1920px) and (max-width: 2560px) {
473: #content {
...:
580: }
581:
582: @media screen and (min-width: 2560px) {
583: #content {
...:
694: }
695: }
Each html markup has own background with different positioning of html-elements, for example this is different view with width less than 480 and more than 480.
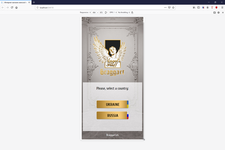
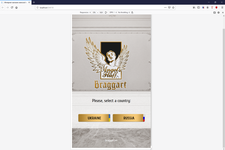
In line 200-207 I arrange buttons in vertically, in 316-323 I arrange buttons horizontally.
...:
125: @media only screen and (min-width: 320px) and (max-width: 480px) {
126: #content {
...:
200: #twobutton {
201: position: relative;
202: top: 330px;
203: display: inline-block;
204: width: 230px;
205: height: 140px;
206: /*border: 1px solid red;*/
207: }
...:
209: #selectukraine {
210: background: url(../Images/480-UkraineSelect.gif) no-repeat left top;
211: width: 230px;
212: height: 58px;
213: border-radius: 8px;
214: cursor: pointer;
215: float: left;
216: }
217:
218: #selectrussia {
219: background: url(../Images/480-RussiaSelect.gif) no-repeat left top;
220: width: 230px;
221: height: 58px;
222: border-radius: 8px;
223: cursor: pointer;
224: float: right;
225: margin-top: 20px;
226: }
...:
236:
237: @media only screen and (min-width: 480px) and (max-width: 768px) {
316: #twobutton {
317: position: relative;
318: top: 400px;
319: display: inline-block;
320: width: 475px;
321: height: 62px;
322: /*border: 1px solid red;*/
323: }
...:
327: #selectukraine {
328: background: url(../Images/768-UkraineSelect.gif) no-repeat left top;
329: width: 230px;
330: height: 58px;
331: border-radius: 8px;
332: cursor: pointer;
333: float: left;
334: }
335:
336: #selectrussia {
337: background: url(../Images/768-RussiaSelect.gif) no-repeat left top;
338: width: 230px;
339: height: 58px;
340: border-radius: 8px;
341: cursor: pointer;
342: float: right;
343: }
Each button has rollover, I define it in this way:
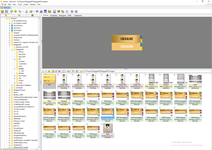
227:
228: #selectrussia:hover {
229: background-position: left bottom;
230: }
231:
232: #selectukraine:hover {
233: background-position: left bottom;
234: }
2. Admin panel design. Loading IP database.
For this project I have created simple Admin panel in classic ASP.NET.
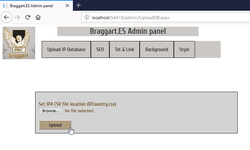
1: <%@ Master Language="VB" AutoEventWireup="false" CodeBehind="M1.master.vb" Inherits="BraggartES.M1" %>
2:
3: <%@ Import Namespace="System.Web.Optimization" %>
4:
5: <!DOCTYPE html>
6: <html>
7: <head runat="server">
8: <title>Braggart.ES Admin panel</title>
9: <asp:ContentPlaceHolder ID="head" runat="server">
10: </asp:ContentPlaceHolder>
11: <asp:PlaceHolder runat="server">
12: <%: Styles.Render("~/Content/css") %>
13: <%: Scripts.Render("~/bundles/modernizr") %>
14: <%: Scripts.Render("~/bundles/jquery") %>
15: </asp:PlaceHolder>
16: <style>
17: ul {
18: list-style-type: none;
19: margin: 0;
20: padding: 0;
21: overflow: hidden;
22: background-color: #cbc7c4;
23: width: 550px;
24: }
25:
26: li {
27: float: left;
28: border-left: 1px;
29: border-style: solid;
30: border-width: 1px;
31: border-collapse: collapse;
32: }
33:
34: li a {
35: display: block;
36: color: #202221;
37: text-align: center;
38: padding: 16px;
39: text-decoration: none;
40: }
41:
42: li a:hover {
43: background-color: #af9d80;
44: }
45:
46: .box {
47: width: 400px;
48: margin: 100px;
49: background-color: lightgray;
50: border: 1px;
51: border-style: solid;
52: padding: 10px;
53: }
54:
55: .button {
56: background-color: antiquewhite;
57: width: 100px;
58: height: 30px;
59: cursor: pointer;
60: }
61:
62: .button:hover {
63: background-color: #af9d80;
64: }
65: </style>
66:
67: </head>
68: <body>
69:
70:
71:
72: <form id="form1" runat="server" style="width: 100%; float: left;">
73: <asp:ImageButton ID="ImageButton1" runat="server" Style="float: left; padding-right: 20px;" ImageUrl="~/Images/Adm.png" PostBackUrl="~/" />
74: <div id="AdminMenu" runat="server">
75: <div style="width:550px;text-align:center;margin-left: 100px;">
76: <asp:LinkButton ID="LinkButton1" runat="server" Style="padding-left: 100px;padding-right: 100px;background-color: #cbc7c4; color: #202221; text-decoration: none; text-align: center; font-size: 25px;" PostBackUrl="~/Admin/Default.aspx">Braggart.ES Admin panel</asp:LinkButton>
77: </div>
78: <div style="height: 15px"></div>
79: <ul>
80: <li>
81: <asp:HyperLink ID="HyperLink1" runat="server" NavigateUrl="~/Admin/UploadDB.aspx">Upload IP Database</asp:HyperLink>
82: </li>
83: <li>
84: <asp:HyperLink ID="HyperLink2" runat="server" NavigateUrl="~/Admin/SeoKey.aspx">SEO</asp:HyperLink>
85: </li>
86: <li>
87: <asp:HyperLink ID="HyperLink3" runat="server" NavigateUrl="~/Admin/TxtAndLink.aspx">Txt & Link</asp:HyperLink>
88: </li>
89: <li>
90: <asp:HyperLink ID="HyperLink4" runat="server" NavigateUrl="~/Admin/Background.aspx">Background</asp:HyperLink>
91: </li>
92: <li>
93: <asp:HyperLink ID="HyperLink5" runat="server" NavigateUrl="~/Admin/Style.aspx">Style</asp:HyperLink>
94: </li>
95: </ul>
96: </div>
97: <div style="clear: both"></div>
98: <div>
99: <asp:ContentPlaceHolder ID="ContentPlaceHolder1" runat="server">
100: </asp:ContentPlaceHolder>
101: </div>
102: <asp:PlaceHolder runat="server">
103: <%: Scripts.Render("~/bundles/jquery") %>
104: </asp:PlaceHolder>
105: </form>
106: </body>
107: </html>
1: <%@ Page Title="" Language="vb" AutoEventWireup="false" MasterPageFile="~/Admin/M1.Master" CodeBehind="UploadDB.aspx.vb" Inherits="BraggartES.UploadDB" %>
2:
3: <asp:Content ID="Content1" ContentPlaceHolderID="head" runat="server">
4: </asp:Content>
5:
6: <asp:Content ID="Content2" ContentPlaceHolderID="ContentPlaceHolder1" runat="server">
7: <div class="box" style="width:600px">
8:
9: <asp:Label ID="lErr1" runat="server" Text="" ForeColor="Red"></asp:Label>
10: <br />
11: <asp:Label ID="Label1" runat="server" Text="Set IP4 CSV file location (IPCountry.csv)" ForeColor="#643d09"></asp:Label>
12: <br />
13: <asp:FileUpload ID="Ip4FileUpload" runat="server" Width="500px" ForeColor="#643D09" />
14:
15: <%-- <br />
16: <br />
17: <asp:Label ID="Label2" runat="server" Text="Set IP6 CSV file location (IPV6-COUNTRY.CSV)" ForeColor="#643d09"></asp:Label>
18: <br />
19: <asp:FileUpload ID="Ip6FileUpload" runat="server" Width="500px" ForeColor="#643D09" />--%>
20: <br />
21: <br />
22: <asp:Button ID="UploadButton" runat="server" Text="Upload" class="button" />
23:
24: </div>
25: </asp:Content>
1: <%@ Page Title="" Language="vb" AutoEventWireup="false" MasterPageFile="~/Admin/M1.Master" CodeBehind="UploadDB.aspx.vb" Inherits="BraggartES.UploadDB" %>
2:
3: <asp:Content ID="Content1" ContentPlaceHolderID="head" runat="server">
4: </asp:Content>
5:
6: <asp:Content ID="Content2" ContentPlaceHolderID="ContentPlaceHolder1" runat="server">
7: <div class="box" style="width:600px">
8:
9: <asp:Label ID="lErr1" runat="server" Text="" ForeColor="Red"></asp:Label>
10: <br />
11: <asp:Label ID="Label1" runat="server" Text="Set IP4 CSV file location (IPCountry.csv)" ForeColor="#643d09"></asp:Label>
12: <br />
13: <asp:FileUpload ID="Ip4FileUpload" runat="server" Width="500px" ForeColor="#643D09" />
14:
15: <%-- <br />
16: <br />
17: <asp:Label ID="Label2" runat="server" Text="Set IP6 CSV file location (IPV6-COUNTRY.CSV)" ForeColor="#643d09"></asp:Label>
18: <br />
19: <asp:FileUpload ID="Ip6FileUpload" runat="server" Width="500px" ForeColor="#643D09" />--%>
20: <br />
21: <br />
22: <asp:Button ID="UploadButton" runat="server" Text="Upload" class="button" />
23:
24: </div>
25: </asp:Content>
3. Admin panel authentication .
For this project I decide simple authentication with define user in config file and to work without user database. I restrict access to admin user.
24: <system.web>
25: <customErrors mode="Off" />
26: <compilation debug="true" targetFramework="4.5.1" />
27: <httpRuntime targetFramework="4.5.1" maxRequestLength="20000000" executionTimeout="300" />
28: <authentication mode="Forms"></authentication>
29: <membership>
30: <providers>
31: <clear />
32: <remove name="AspNetSqlMembershipProvider" />
33: </providers>
34: </membership>
35: </system.web>
36: <location path="~/Admin">
37: <system.web>
38: <authentication mode="Forms">
39: <forms loginUrl="~/Admin/Login.aspx" timeout="2880" defaultUrl="~/Admin/Default.aspx">
40: <credentials passwordFormat="Clear">
41: <user name="XXXXXXXXXXXX" password="YYYYYYYYYYY" />
42: </credentials>
43: </forms>
44: </authentication>
45: <authorization>
46: <deny users="?" />
47: </authorization>
48: <membership>
49: <providers>
50: <clear />
51: <remove name="AspNetSqlMembershipProvider" />
52: </providers>
53: </membership>
54: </system.web>
55: </location>
Each page before beginning must check user authentication.
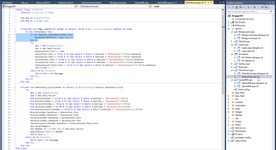
And this is my login page.
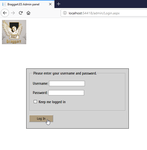
1: <%@ Page Title="" Language="vb" AutoEventWireup="false" MasterPageFile="~/Admin/M1.Master" CodeBehind="Login.aspx.vb" Inherits="BraggartES.Login" %>
2:
3: <asp:Content ID="Content1" ContentPlaceHolderID="head" runat="server">
4: </asp:Content>
5: <asp:Content ID="Content2" ContentPlaceHolderID="ContentPlaceHolder1" runat="server">
6: <div style="">
7: <div class="box">
8: <span class="failureNotification">
9: <asp:Literal ID="FailureText" runat="server"></asp:Literal>
10: </span>
11: <asp:ValidationSummary ID="LoginUserValidationSummary" runat="server" CssClass="failureNotification"
12: ValidationGroup="LoginUserValidationGroup" />
13: <div class="accountInfo">
14: <fieldset class="login">
15: <legend>Please enter your username and password.</legend>
16: <p>
17: <asp:Label ID="UserNameLabel" runat="server" AssociatedControlID="UserName">Username:</asp:Label>
18: <asp:TextBox ID="UserName" runat="server" CssClass="textEntry"></asp:TextBox>
19: <asp:RequiredFieldValidator ID="UserNameRequired" runat="server" ControlToValidate="UserName"
20: CssClass="failureNotification" ErrorMessage="User Name is required." ToolTip="User Name is required."
21: ValidationGroup="LoginUserValidationGroup">*</asp:RequiredFieldValidator>
22: </p>
23: <p>
24: <asp:Label ID="PasswordLabel" runat="server" AssociatedControlID="Password">Password:</asp:Label>
25: <asp:TextBox ID="Password" runat="server" CssClass="passwordEntry" TextMode="Password"></asp:TextBox>
26: <asp:RequiredFieldValidator ID="PasswordRequired" runat="server" ControlToValidate="Password"
27: CssClass="failureNotification" ErrorMessage="Password is required." ToolTip="Password is required."
28: ValidationGroup="LoginUserValidationGroup">*</asp:RequiredFieldValidator>
29: </p>
30: <p>
31: <asp:CheckBox ID="RememberMe" runat="server" />
32: <asp:Label ID="RememberMeLabel" runat="server" AssociatedControlID="RememberMe" CssClass="inline">Keep me logged in</asp:Label>
33: </p>
34: </fieldset>
35: <p class="submitButton">
36: <asp:Button ID="LoginButton" runat="server" CommandName="Login" Text="Log In" ValidationGroup="LoginUserValidationGroup" class="button" />
37: </p>
38: </div>
39: </div>
40: </div>
41: </asp:Content>
1: Imports System.Xml
2:
3: Public Class Login
4: Inherits System.Web.UI.Page
5:
6: Protected Sub LoginButton_Click(sender As Object, e As EventArgs) Handles LoginButton.Click
7: Dim RDR As XmlTextReader
8: RDR = New XmlTextReader(Server.MapPath("~/web.config"))
9: Dim XML As XmlDocument = New XmlDocument()
10: XML.Load(RDR)
11: Dim Name As String = XML.SelectNodes("//user")(0).Attributes("name").Value
12: Dim Pass As String = XML.SelectNodes("//user")(0).Attributes("password").Value
13: If UserName.Text = Name And Password.Text = Pass Then
14: Dim ticket As New FormsAuthenticationTicket(1, Name, Now, Now.AddYears(1), True, "", "/Admin")
15: Dim EncryptTicket As String = FormsAuthentication.Encrypt(ticket)
16: Dim AUCook As HttpCookie = New HttpCookie(FormsAuthentication.FormsCookieName, EncryptTicket)
17: AUCook.Domain = ConfigurationManager.AppSettings.Item("Domain")
18: AUCook.Expires = Now.AddYears(1)
19: Response.Cookies.Add(AUCook)
20: Response.Redirect("~/Admin/Default.aspx")
21: End If
22: End Sub
23:
24: End Class
4. Loading IP CSV-file to database.
For this project I pay database in https://www.ip2location.com/
After that I define a database in MS SQL. Firstly I plain upload IP4 and IP6 database, but little bit later I decides to limit myself only IP4 database, but there is definition to both tables (IP6 database has more precision than support MS SQL, therefore I store data in binary form).
1: CREATE TABLE [dbo].[IP4](
2: [I] [int] IDENTITY(1,1) NOT NULL,
3: [IP_FROM] [bigint] NOT NULL,
4: [IP_TO] [bigint] NOT NULL,
5: [COUNTRY_CODE] [char](2) NOT NULL,
6: CONSTRAINT [PK_IP4] PRIMARY KEY CLUSTERED
7: (
8: [I] ASC
9: )WITH (PAD_INDEX = OFF, STATISTICS_NORECOMPUTE = OFF, IGNORE_DUP_KEY = OFF, ALLOW_ROW_LOCKS = ON, ALLOW_PAGE_LOCKS = ON) ON [PRIMARY]
10: ) ON [PRIMARY]
11:
12: GO
13:
14: CREATE TABLE [dbo].[IP6](
15: [I] [int] IDENTITY(1,1) NOT NULL,
16: [IP_FROM] [varbinary](39) NOT NULL,
17: [IP_TO] [varbinary](39) NOT NULL,
18: [COUNTRY_CODE] [char](2) NOT NULL,
19: CONSTRAINT [PK_IP6] PRIMARY KEY CLUSTERED
20: (
21: [I] ASC
22: )WITH (PAD_INDEX = OFF, STATISTICS_NORECOMPUTE = OFF, IGNORE_DUP_KEY = OFF, ALLOW_ROW_LOCKS = ON, ALLOW_PAGE_LOCKS = ON) ON [PRIMARY]
23: ) ON [PRIMARY]
24:
25: GO
And this is a form to uploading ip2location CSV-file to my database. For this task I have to filtered data and stored IP only for Ukraine and Russia.
1: <%@ Page Title="" Language="vb" AutoEventWireup="false" MasterPageFile="~/Admin/M1.Master" CodeBehind="UploadDB.aspx.vb" Inherits="BraggartES.UploadDB" %>
2:
3: <asp:Content ID="Content1" ContentPlaceHolderID="head" runat="server">
4: </asp:Content>
5:
6: <asp:Content ID="Content2" ContentPlaceHolderID="ContentPlaceHolder1" runat="server">
7: <div class="box" style="width:600px">
8:
9: <asp:Label ID="lErr1" runat="server" Text="" ForeColor="Red"></asp:Label>
10: <br />
11: <asp:Label ID="Label1" runat="server" Text="Set IP4 CSV file location (IPCountry.csv)" ForeColor="#643d09"></asp:Label>
12: <br />
13: <asp:FileUpload ID="Ip4FileUpload" runat="server" Width="500px" ForeColor="#643D09" />
14: <br />
15: <br />
16: <asp:Button ID="UploadButton" runat="server" Text="Upload" class="button" />
17:
18: </div>
19: </asp:Content>
1: Imports System.IO
2:
3: Public Class UploadDB
4: Inherits System.Web.UI.Page
5:
6: Protected Sub Page_Load(ByVal sender As Object, ByVal e As System.EventArgs) Handles Me.Load
7: If Not IsPostBack Then
8: If Not Request.IsAuthenticated Then
9: Response.Redirect("Login.aspx")
10: End If
11: End If
12: End Sub
13:
14: Protected Sub UploadButton_Click(sender As Object, e As EventArgs) Handles UploadButton.Click
15: Dim Ip4CurRowNumber As Integer = 1
16: Try
17: Dim db1 As New BrgUaEntities
18: db1.Database.ExecuteSqlCommand("Truncate table dbo.IP4")
19: Dim Ip4Buffer(Ip4FileUpload.PostedFile.InputStream.Length) As Byte
20: Ip4FileUpload.PostedFile.InputStream.Seek(0, IO.SeekOrigin.Begin)
21: Ip4FileUpload.PostedFile.InputStream.Read(Ip4Buffer, 0, Convert.ToInt32(Ip4FileUpload.PostedFile.InputStream.Length))
22: Dim Ip4Stream As IO.Stream = New IO.MemoryStream(Ip4Buffer)
23: Dim Ip4RDR = New StreamReader(Ip4Stream)
24: While True
25: Dim Ip4Str1 = Ip4RDR.ReadLine
26: If Ip4Str1 Is Nothing Then Exit While
27: Dim Ip4Arr1() As String = Ip4Str1.Split(",")
28: If Ip4Arr1.Length = 4 Then
29: If Ip4Arr1(2).Replace("""", "") = "UA" Or Ip4Arr1(2).Replace("""", "") = "RU" Then
30: Ip4CurRowNumber += 1
31: db1.IP4.Add(New IP4 With {
32: .IP_FROM = Ip4Arr1(0).Replace("""", ""),
33: .IP_TO = Ip4Arr1(1).Replace("""", ""),
34: .COUNTRY_CODE = Ip4Arr1(2).Replace("""", "")
35: })
36: db1.SaveChanges()
37: End If
38: End If
39: End While
40: lErr1.Text = "RU & UA :" & Ip4CurRowNumber & " rows uploaded"
41: Catch ex As Exception
42: lErr1.Text = "Row=" & Ip4CurRowNumber & ":" & ex.Message
43: End Try
44: End Sub
45: End Class
5. Cache IP database to memory.
Initially I use ORM Linq-to-SQL, but little bit later I have rebuild this site to EF. Below you can see a screen with EF6 and three magic string to upload IP-database to site memory.
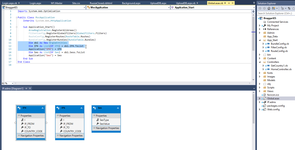
It is reasonable, because database is not huge.
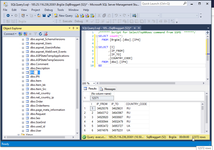
6. Get IP4 from IP6 address.
If I decide to use only IP6, I create this module to analyze any IP addr adn select IP4 from app possible IPs.
31: Public Shared Function GetIP4Address() As String
32: Dim IP4Address As String = String.Empty
33:
34: For Each IPA As IPAddress In Dns.GetHostAddresses(HttpContext.Current.Request.UserHostAddress)
35: If IPA.AddressFamily.ToString() = "InterNetwork" Then
36: IP4Address = IPA.ToString()
37: Exit For
38: End If
39: Next
40:
41: Return IP4Address
42:
43: End Function
44: End Class
7. Get Country by IP.
Pay attention to calculation in string 16 - this is more than integer !
1: Imports System.Net
2: Imports BraggartES
3: Friend Class GetCountry
4:
5: Public Shared Function FromIP4(Ip As String) As String
6: Dim IP4 As List(Of IP4)
7: If HttpContext.Current.Application("IP4") Is Nothing Then
8: Dim db1 As New BrgUaEntities
9: HttpContext.Current.Application("IP4") = db1.IP4.ToList
10: End If
11: IP4 = HttpContext.Current.Application("IP4")
12: Dim IpArr1() As String = Ip.Split(".")
13: Dim Res As Integer = 0
14: If IpArr1.Length = 4 Then
15: If Integer.TryParse(IpArr1(0), Res) And Integer.TryParse(IpArr1(1), Res) And Integer.TryParse(IpArr1(2), Res) And Integer.TryParse(IpArr1(3), Res) Then
16: Dim IpValue As Int64 = CLng(IpArr1(0)) * 256 * 256 * 256 + CLng(IpArr1(1)) * 256 * 256 + CLng(IpArr1(2)) * 256 + CLng(IpArr1(3))
17: Dim Z = (From X In IP4 Select X Where X.IP_FROM <= IpValue And X.IP_TO >= IpValue).ToList
18: If Z.Count > 0 Then
19: Return Z(0).COUNTRY_CODE
20: Else
21: Return "NO"
22: End If
23: Else
24: Return "NO"
25: End If
26: Else
27: Return "NO"
28: End If
29: End Function
30:
8. MVC controller code
In string 59-62 I define a model to communicate with view.
1: Public Class HomeController
2: Inherits System.Web.Mvc.Controller
3:
4: Dim db1 As BrgUaEntities
5: Dim Seo As List(Of Seo)
6:
7: Function Index() As ActionResult
8: If HttpContext.Application("Seo") Is Nothing Then
9: db1 = New BrgUaEntities
10: Seo = db1.Seos.ToList
11: Else
12: Seo = HttpContext.Application("Seo")
13: End If
14: ViewBag.Title = (From X In Seo Select X Where X.SeoType = "PageTitle").First.SeoValue
15: ViewBag.Description = (From X In Seo Select X Where X.SeoType = "PageDescription").First.SeoValue
16: ViewBag.Keywords = (From X In Seo Select X Where X.SeoType = "PageKeywords").First.SeoValue
17: '
18: Dim RequestIP4 As String = GetCountry.GetIP4Address
19: Dim Country As String = "NO"
20: If Not String.IsNullOrEmpty(RequestIP4) Then
21: Country = GetCountry.FromIP4(RequestIP4)
22: End If
23: '
24: Dim Model = New MvcModel
25: 'Country = "NO"
26: Select Case Country
27: Case "NO"
28: Model.Label = (From X In Seo Select X Where X.SeoType = "UnknounCountry").First.SeoValue
29: If CBool(ConfigurationManager.AppSettings("RussiaReady")) Then
30: Model.RussiaLink = (From X In Seo Select X Where X.SeoType = "RussiaLink").First.SeoValue
31: Else
32: Model.RussiaLink = "/Home/Russia"
33: End If
34: Model.UkraineLink = (From X In Seo Select X Where X.SeoType = "UkraineLink").First.SeoValue
35: Return View("SelectCountry", Model)
36: Case "RU"
37: Model.Label = (From X In Seo Select X Where X.SeoType = "RussiaText").First.SeoValue
38: If CBool(ConfigurationManager.AppSettings("RussiaReady")) Then
39: Model.Link = (From X In Seo Select X Where X.SeoType = "RussiaLink").First.SeoValue
40: Else
41: Model.Link = "/Home/Russia"
42: End If
43: Return View("Russia", Model)
44: Case "UA"
45: Model.Label = (From X In Seo Select X Where X.SeoType = "UkraineText").First.SeoValue
46: Model.Link = (From X In Seo Select X Where X.SeoType = "UkraineLink").First.SeoValue
47: Return View("Ukraine", Model)
48: Case Else
49: Return View("Index", Model)
50: End Select
51: End Function
52:
53: Function Russia() As ActionResult
54: Return View("RussiaClosed")
55: End Function
56:
57: End Class
58:
59: Public Class MvcModel
60: 'Ukraine & Russia
61: Public Property Link As String
62: 'Ukraine & Russia & SelectCountry & RussiaClosed
63: Public Property Label As String
64: 'SelectCountry
65: Public Property UkraineLink As String
66: Public Property RussiaLink As String
67: End Class
9. Layout page and Views.
This is layout page with CUPRUM font definition and title setting in admin system and stored in DB.
1: <!DOCTYPE html>
2: <html>
3: <head>
4: <meta charset="utf-8" />
5: <meta name="viewport" content="width=device-width, initial-scale=1.0">
6: <meta name="description" content="@ViewBag.Description">
7: <meta name="keywords" content="@ViewBag.Keywords">
8: <title>@ViewBag.Title</title>
9: <link href="https://fonts.googleapis.com/css?family=Cuprum" rel="stylesheet">
10: @Styles.Render("~/Content/css")
11: @Scripts.Render("~/bundles/modernizr")
12: </head>
13: <body>
14: <div id="content">
15: >@@RenderBody()
16: <div id="copyright">
17: <p>Braggart.es</p>
18: </div>
19: </div>
20: </body>
21: </html>
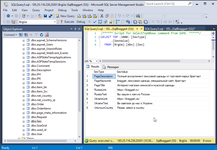
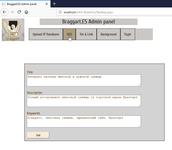
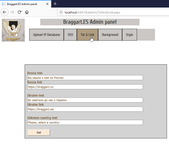
And this is some another view of this smallest site.
1: @Code
2: Layout = "~/Views/Shared/_Layout.vbhtml"
3: @ModelType MvcModel
4: End Code
5:
6: <form action="@Model.Link">
7: <input id="angel" type="submit" value="" />
8: </form>
9:
10: <div id="label">@Model.Label</div>
11:
12: <div id="onebutton">
13: <form action="@Model.Link">
14: <input id="goukraine" type="submit" value="" />
15: </form>
16: </div>
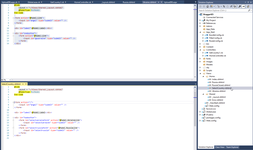
This site is extremely small, another my sites has hundreds of forms, please see for example:
- ?????????????? ???????????? ????????????????
- ?????????????????????? ?????????????? ?????????????????? SHEL-AUTO.RU ???? web-???????????????? EMEX.RU.
- Social network for Canada with printed version of calendar to communities.
but this site is so instructive, therefore I decide to describe it.

|