<< return Tsc Compiler "Module" and "outFile/outDir" Options.
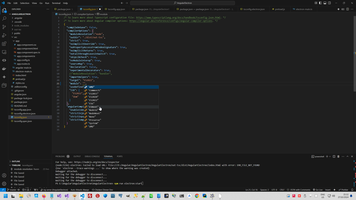
module Value | Description | Suitable for | Notes |
ES2015 (or es2015) | Generates ES2015 (ES6) modules. | Older projects or environments that support ES2015 modules. | Less common in modern Angular projects. |
ES2020 (or es2020) | Generates ES2020 modules. | Projects targeting environments supporting ES2020 or later. | A good choice if you need some ES2020 features and your target environment supports it. |
ES2022 (or es2022) | Generates ES2022 modules. | Projects targeting environments supporting ES2022 or later. | A good modern choice for most Angular projects if you're not using a bundler-specific module format. |
ESNext | Generates modules using the latest ECMAScript features. This is the most current standard. | Modern projects, especially those using bundlers like Webpack or Vite. | Webpack or Vite will bundle this down to a compatible version for browsers. Avoid using this directly for browser environments. |
commonjs | Generates CommonJS modules (Node.js style). | Primarily Node.js server-side code, or projects explicitly using CommonJS. | Not typically used for client-side Angular applications unless building a server-side component. |
amd | Generates Asynchronous Module Definition (AMD) modules. | Older projects or environments that specifically require AMD modules. | Rarely used in modern web development. |
system | Generates SystemJS modules. | Projects using the SystemJS module loader. | Less common in modern Angular projects. |
umd | Generates Universal Module Definition (UMD) modules, which can work in various environments (browser, Node.js, AMD, etc.). | Libraries intended for wide use across different environments. | Not commonly needed for Angular applications themselves, more often for creating reusable libraries. |
Important Considerations for Angular (more about other AngularOptions):
- • Bundlers: Modern Angular projects almost always use a bundler (Webpack, Vite, or others). The bundler handles module resolution and transpilation, often making the choice of module in tsconfig.json less critical. Webpack and Vite typically work best with ESNext because it allows them to leverage the latest JavaScript features and optimize the output effectively.
- • moduleResolution: node: This setting always needs to be node in your main tsconfig.json and all secondary ones unless you are explicitly using a different module resolution strategy defined by your bundler.
- • Angular CLI: The Angular CLI manages the build process. It generally handles module transformations automatically, so you usually don't have to fine-tune the module setting unless you encounter specific issues or have non-standard build configurations.
If you're using outFile to bundle your Electron main.ts into a single main.js file and want to use tsc without a bundler like Webpack, then "commonjs" is usually the best choice.
Main choice outFile/outDir:
- outFile bundles everything into a single file, which doesn't work well with ES modules or more modern module systems. tsc with outFile only supports "AMD" and "System" if you need to bundle your output into a single file. Between those two, "System" is generally the more modern and preferred choice when using outFile, though using outFile itself isn't ideal for larger projects or those using modern JavaScript features.
- "module": • "commonjs": Why commonjs isn't an option with outFile. The CommonJS format (used by Node.js) relies on synchronous require() calls. The outFile option bundles everything into one file, however, within a single bundled file, there's no way to do these synchronous require() calls to modules that are embedded within the bundle. That's why tsc only allows "AMD" and "System" which use a module format that can work with bundled code. If you want to use "commonjs", then do not use outFile and use a bundler or tsc without outFile.
- "module": • "System": The "System" module format is designed to be a universal module loader, capable of handling various module formats (including CommonJS, AMD, and ES modules). While SystemJS itself is less widely used directly today in web development, the "module": "System" option in tsc generates code that's structurally closer to ES modules and could potentially be easier to adapt to a bundler or more modern setup later.
- "module": • "AMD" (Asynchronous Module Definition): AMD was created for asynchronous module loading in browsers but is less common in modern web development due to better native browser support for es modules, better network connectivity, and improvements in general that make it a less popular choice. It's usually not necessary or the most efficient choice.
- If you're not using outFile and want the TypeScript compiler to generate separate .js files for each .ts file, then you should be using "module": "ES2022" for most projects (and definitely for your Angular app's TypeScript code) along with "moduleResolution": "bundler" and a bundler like Webpack. If you decide to follow this approach (which is recommended) then you should also modify your scripts section and add scripts to compile the main electron file and use Webpack. If you choose this path then modify the angular project's tsconfig.json file to have different compilerOptions so that both the frontend Angular code and the Electron main process are compiled correctly. Create a new tsconfig.electron.json that extends the main tsconfig.json that sets the "module" to commonjs or amd to resolve the error and ensure that the moduleResolution is not set to bundler
- • "module": "commonjs": This emits modules following the CommonJS format, which is the standard module system used in Node.js. It relies on the require() function to import modules and module.exports to export them. This format is very common when writing server-side Javascript and in Electron's main process.
- • "module": "AMD" (Asynchronous Module Definition): This emits modules using the Asynchronous Module Definition format, designed for asynchronous loading of modules in browsers. It uses the define() function to define modules and require() to load dependencies. AMD was more popular in the past when asynchronous module loading was crucial due to slower network speeds and larger JavaScript files but is not used very often today.
- • "module": "ES2022" (ECMAScript Modules): This emits modules using the modern ECMAScript module (ESM) syntax, introduced in ES6 (ES2015). It uses import and export statements, which are now supported natively in modern browsers and Node.js. This is currently the preferred format for new projects and frontend projects in general and it is the default for new Angular projects.
The key difference between "module": "AMD" / "commonjs" / "ES2022" (or other similar module system) lies in how modules (separate JavaScript files that encapsulate code) are defined, imported, and exported in the JavaScript code generated by the TypeScript compiler (tsc).
Electron context:
- (2024) Electron video player with cashing and CSP policy #Electron #Video
- (2024) My workable project template for Angular Electron #Angular #Electron
- (2024) Node PostProcessor step for Angular and Electron. #Angular #Electron #NodeBackend
- (2022) Electron learning #FrontLearning #Electron
- ...
- (2024) Browser Window Options in Electron application.
- (2024) Important Parameters in Electron application.
- (2024) Core Features and Concepts in Electron application.
- (2024) Crucial Programming Techniques in Electron application.
- (2024) Main Process vs. Renderer Process in Electron application.
- (2024) Inter-Process Communication in Electron application.
- (2024) Asynchronous Operations in Electron application.
- (2024) Databases in Electron application.
- (2024) MySQL and PostgreSQL integration in Electron application.
- (2024) LocalStorage in Electron application.
- (2024) Native Modules in Electron application.
- (2024) Electron APIs in Electron application.
- (2024) Multi-window Applications in Electron application.
- (2024) Packaging and Distribution in Electron application.
- (2024) Node Integration in Electron application.
- (2024) Memory Leaks in Electron application.
- (2024) Code Security in Electron application.
- (2024) Browser Automation in Electron application.
- (2024) Testing in Electron application.
- (2024) Monetization in Electron application.
AngularElectron context:
Front context:
- (2025) My new project with WebRTC, what is WebRTC? #Android #Front
- (2024) Encapsulate HTML, CSS, and JS to WebComponent. Shadow DOM. Example of my WebComponent in this blog. #Front
- (2024) My lecture about Javascript (Ukrainian language). #Front
- (2019) Inject OnBegin/OnSuccess/OnFailure custom Javascript code into ASP.NET Ajax tag by Bundle of jquery.unobtrusive-ajax.min.js #Front #JavascriptProjects
- (2017) My site with peer-to-peer communication based on Mizu-Voip library. #Front #Css #Voip
- (2017) My solution with Bootstrap modal window and Classic ASP.NET. #AspNetClassic #Front #Css
- (2016) SPA-page на Classic ASP.NET та jQuery. #Front #AspNetClassic
- (2015) Cropper світлин сайту. #Front #AspNetMvc #Css
- (2015) Перемикач мови для сайту. #Front #AspNetMvc
- (2012) Календарики (datapicker), применяемые мною на jQuery / MS AJAX / JavaScript / Flex / MONO. #Front
- (2012) Заполнение связанных списков на MS AJAX и jQuery. #Front #AspNetClassic
- (2012) Применение jQuery-UI-dialog в ASP.NET #Front #AspNetClassic
- (2011) Как с помощью jQuery сделать флеш-ролик резиновым #Front #Flex
- (2011) AJAX подсказка/автозаполнение на jQuery #Front
- (2010) Flex, Java, Angular, Android. #SectionFront
- (2009) Язва-скрипт в ASP.NET. #Front
- (2009) Счетчики на Web-страничках. #AspNetClassic #Front
Comments (
)

Link to this page:
http://www.vb-net.com/AngularElectron/TscCompilerOptions.htm
|